Mastering the Factory Pattern: Common Pitfalls in Java
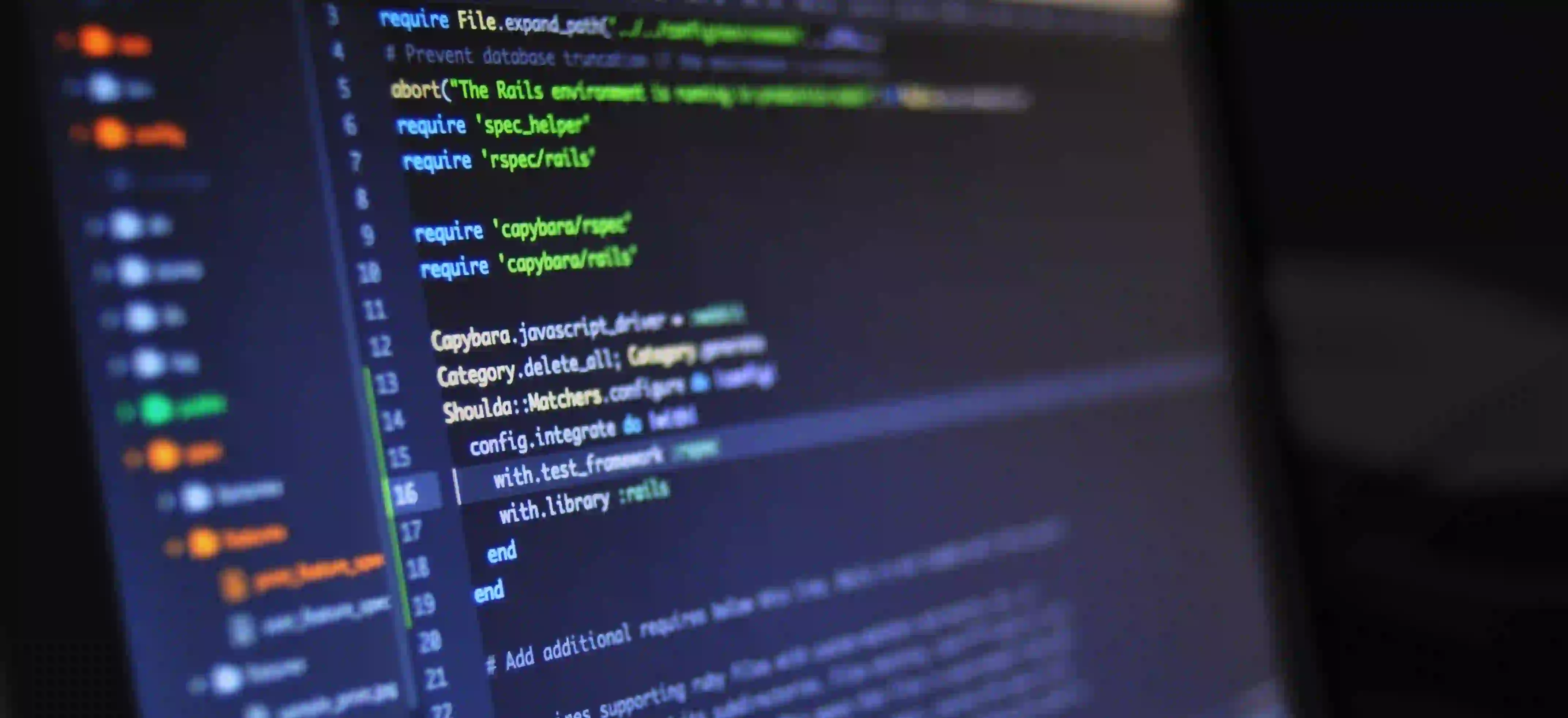
Mastering the Factory Pattern: Common Pitfalls in Java
In the realm of object-oriented programming, design patterns have become an essential component of software development. Among these, the Factory Pattern stands out as a versatile approach to object creation. This pattern abstracts the instantiation process, making it easier to manage and extend. However, even seasoned developers can fall prey to common pitfalls when implementing the Factory Pattern in Java. In this blog post, we dive deep into the intricacies of the Factory Pattern, highlight its benefits, and pinpoint the common mistakes developers make. By the end, you will be equipped to apply this design pattern more effectively in your Java projects.
What is the Factory Pattern?
The Factory Pattern is categorized as a creational design pattern. Essentially, it provides an interface for creating objects in a superclass, but allows subclasses to alter the type of created objects. This separation can promote loose coupling and lead to code that is easier to read and maintain.
Benefits of the Factory Pattern
-
Loose Coupling: One of the primary advantages of using the Factory Pattern is that it decouples the client code from the concrete instances it works with. This means that changes to object creation don’t directly impact the usage of the object.
-
Centralized Object Creation: Factory classes centralize the logic of object instantiation, making it easier to manage and maintain.
-
Ease of Extension: If new types of objects need to be added, associated changes can be confined to the factory and not spread across the entire codebase.
Factory Method Pattern Example
Let’s consider an example in Java to better grasp the idea of the Factory Pattern.
// Step 1: Create an interface for the product
public interface Product {
void use();
}
// Step 2: Implement Concrete Products
public class ConcreteProductA implements Product {
public void use() {
System.out.println("Using product A");
}
}
public class ConcreteProductB implements Product {
public void use() {
System.out.println("Using product B");
}
}
// Step 3: Create a Factory Class
public abstract class Factory {
public abstract Product createProduct();
}
// Step 4: Implement Concrete Factories
public class ConcreteFactoryA extends Factory {
public Product createProduct() {
return new ConcreteProductA();
}
}
public class ConcreteFactoryB extends Factory {
public Product createProduct() {
return new ConcreteProductB();
}
}
// Main class to test the factories
public class Client {
public static void main(String[] args) {
Factory factoryA = new ConcreteFactoryA();
Product productA = factoryA.createProduct();
productA.use(); // Outputs: Using product A
Factory factoryB = new ConcreteFactoryB();
Product productB = factoryB.createProduct();
productB.use(); // Outputs: Using product B
}
}
In this demonstration, we illustrate the Factory Pattern's structure. Notice how the Client
class operates independently of the specific Product
implementations. This promotes flexibility in code maintenance and enhancement.
Common Pitfalls in Implementing the Factory Pattern
While the Factory Pattern offers substantial benefits, it is important to be aware of certain pitfalls that can lead to inefficiencies or increased complexity. Here are some of the most prevalent mistakes:
1. Over-Engineering the Factory
One common mistake is overcomplicating the factory design. Some developers may try to handle too many variations in a single factory. Consequently, they end up with extended classes that become cumbersome.
Solution: Keep it simple! If there are multiple products, consider using multiple smaller factories instead of a monolithic one. Each factory can focus on a specific product family, thus promoting single responsibility.
public interface Shape {
void draw();
}
public class Circle implements Shape {
public void draw() {
System.out.println("Drawing a Circle");
}
}
public class Rectangle implements Shape {
public void draw() {
System.out.println("Drawing a Rectangle");
}
}
public abstract class ShapeFactory {
public abstract Shape createShape();
}
// Concrete Factories
public class CircleFactory extends ShapeFactory {
public Shape createShape() {
return new Circle();
}
}
public class RectangleFactory extends ShapeFactory {
public Shape createShape() {
return new Rectangle();
}
}
2. Lack of Flexibility
Another pitfall is a lack of flexibility in the factory design. If the factory is tightly integrated with specific product types, adding new product types can become a monumental task.
Solution: Use interfaces and abstract classes to guide your implementation. This allows you to easily introduce new product types without modifying existing code.
3. Ignoring Dependency Injection
Some developers neglect to leverage dependency injection (DI) when implementing the Factory Pattern. This decision often leads to a tightly coupled codebase.
Solution: Use DI frameworks like Spring, which can facilitate the creation of factory beans to instantiate your objects dynamically.
This approach not only keeps your component configurations clean but also enhances testing by allowing mock objects to be injected where needed.
4. Insufficient Error Handling
Factories may throw unexpected exceptions if the creation process isn't handled correctly. Skipping error handling can cause your application to be brittle.
Solution: Implement robust error handling mechanisms, such as try-catch blocks, to gracefully manage object creation failures.
public Product createProduct(String type) {
if (type.equals("A")) {
return new ConcreteProductA();
} else if (type.equals("B")) {
return new ConcreteProductB();
} else {
throw new IllegalArgumentException("Unknown product type");
}
}
5. Forgetting to Document
Lastly, a common mistake is not documenting the purpose and functionality of the factory class. Clear documentation is crucial for maintainability, especially in larger teams.
Solution: Always comment on the functionality of your factory classes and methods to support future developers looking to understand the intent behind the design.
The Closing Argument
Mastering the Factory Pattern in Java requires an understanding of both its strengths and potential pitfalls. By avoiding over-engineering, maintaining flexibility, leveraging dependency injection, ensuring proper error handling, and documenting your code, you can create an effective and maintainable implementation of the Factory Pattern.
As you implement this design pattern in your next Java project, bear these insights in mind. If you wish to delve deeper into Java design patterns and their applications, consider exploring Refactoring Guru for extensive resources.
By consistently applying these principles, you will position yourself not only as a competent developer but also as an advocate for robust, scalable software design. Happy coding!