Mastering Java Strategy Pattern: Common Pitfalls Explained
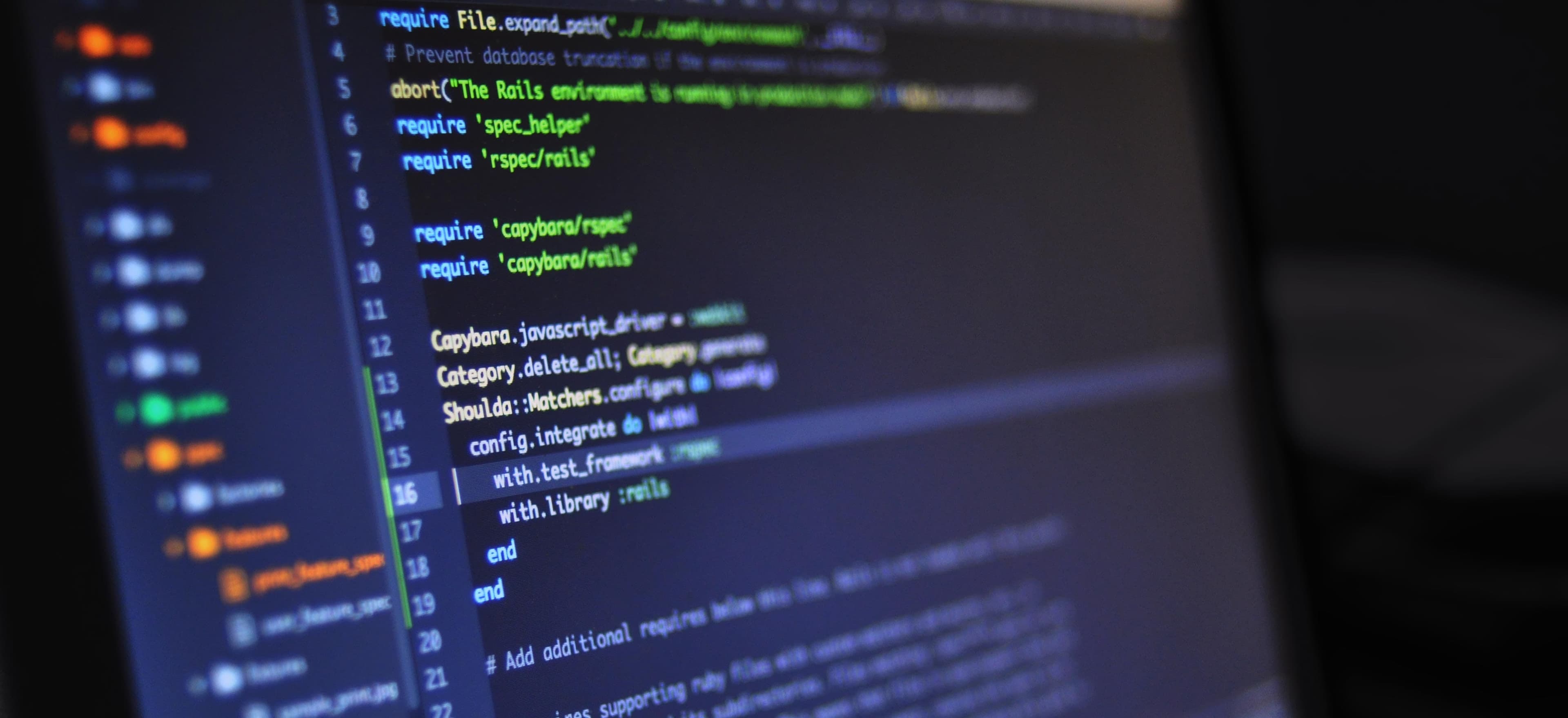
- Published on
Mastering Java Strategy Pattern: Common Pitfalls Explained
Java is a versatile programming language that boasts several design patterns, one of which is the Strategy Pattern. This pattern is particularly useful for defining a family of algorithms, encapsulating each one, and making them interchangeable. With the adoption of this pattern, your code can remain flexible and adhere to the Open/Closed Principle—a key aspect of the SOLID principles of object-oriented design.
What Is the Strategy Pattern?
The Strategy Pattern enables an object to select an algorithm from a family of algorithms at runtime. It promotes the separation of concerns, allowing developers to develop various strategies without altering the context in which these strategies are applied.
Why Use the Strategy Pattern?
- Flexibility: Clients can choose which algorithm to use at runtime.
- Encapsulation: Each algorithm implementation can reside in its own class, enhancing readability and maintainability.
- Reusability: Different contexts can reuse the strategies.
Basic Structure
// Strategy Interface
public interface SortingStrategy {
void sort(int[] array);
}
// Concrete Strategy A
public class BubbleSort implements SortingStrategy {
public void sort(int[] array) {
// Simple bubble sort algorithm
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
swap(array, j, j + 1);
}
}
}
}
private void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
// Concrete Strategy B
public class QuickSort implements SortingStrategy {
public void sort(int[] array) {
quickSort(array, 0, array.length - 1);
}
private void quickSort(int[] array, int low, int high) {
if (low < high) {
int pivotIndex = partition(array, low, high);
quickSort(array, low, pivotIndex - 1);
quickSort(array, pivotIndex + 1, high);
}
}
private int partition(int[] array, int low, int high) {
int pivot = array[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (array[j] <= pivot) {
i++;
swap(array, i, j);
}
}
swap(array, i + 1, high);
return i + 1;
}
private void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
// Context
public class SortingContext {
private SortingStrategy strategy;
public SortingContext(SortingStrategy strategy) {
this.strategy = strategy;
}
public void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
public void sortArray(int[] array) {
strategy.sort(array);
}
}
Commentary on the Code
-
Strategy Interface: We define a common interface
SortingStrategy
that mandates the implementation of the sort method. This abstraction allows us to define any sorting algorithm. -
Concrete Strategies:
BubbleSort
andQuickSort
are artifacts of distinct sorting algorithms. Each implementation encapsulates the sorting algorithm, fulfilling the single responsibility principle. -
Context Class: The
SortingContext
class is useful for clients wishing to execute a specific strategy without knowing the details of the algorithm being applied. It keeps a reference to theSortingStrategy
.
Common Pitfalls with the Strategy Pattern
While the Strategy Pattern can greatly simplify your code, pitfalls often arise during its implementation. Here are eight common pitfalls to watch out for:
1. Overcomplicating Simple Problems
Many developers may use the Strategy Pattern for trivial cases where a simple if-else statement would suffice. You shouldn't complicate your project unnecessarily.
2. Ignoring Cohesion
When mixing too many strategies, the code's cohesion can suffer. Make sure that related strategies belong in the same context for clarity.
3. Neglecting Encapsulation
Another common error is failing to properly encapsulate the individual strategies, which can result in unexpected behaviors. Design each strategy to be self-contained and independent.
4. Tight Coupling with Context
Avoid tightly coupling your strategies to the context. This can make unit testing and future modifications difficult. Strive for loose coupling using dependency injection frameworks like Spring.
5. Loss of Performance
In certain situations, using a strategy may introduce performance overhead. This can occur due to additional abstraction layers. It’s best to profile code to ensure that performance remains acceptable.
6. Poor Naming Conventions
Name your strategies meaningfully to clarify their purpose. Avoid generic names that could confuse developers about the algorithm's function. For example, instead of just naming a class SortStrategy
, consider MergeSortStrategy
.
7. Forgetting Strategy Changes
When context changes trigger strategy changes, often developers forget to update or reconfigure strategies that depend on the new context. This oversight can produce bugs.
8. Underestimating Testing Requirements
An often neglected aspect is adequate testing. Each strategy should be tested in isolation. Adopting a test-driven development strategy can help ensure that each part functions correctly before integration.
Best Practices in Implementing the Strategy Pattern
-
Use Dependency Injection: A good practice is to leverage Dependency Injection (DI) frameworks like Spring. They help manage dependencies and promote easy swapping of algorithms.
-
Document: Clear documentation on your strategy implementations can save time and confusion for your peers. Ensure that you explain the algorithm and its expected usage.
-
Adopt Descriptive Interface Names: Besides clear class and method names, consider providing examples of how to use the strategies within your documentation.
-
Keep Strategies Cohesive: Ensure that strategies follow a singular responsibility. This simplifies understanding and future enhancements.
-
Unit Testing: Create robust unit tests for every strategy to guarantee they behave as intended under various conditions.
The Closing Argument
The Java Strategy Pattern is a powerful design tool that, when mastered, can significantly enhance your programming prowess. However, it comes with its share of pitfalls, as illustrated above. By understanding these common mistakes and adhering to best practices, developers can utilize the Strategy Pattern to create flexible, maintainable, and efficient Java applications.
If you're interested in further exploring Java design patterns, check out resources like Refactoring Guru and The Source Making, where you can gain deeper insights into design patterns and best practices in software engineering.
References
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma et al.
- SOLID Principles in Java: A Practical Guide
By understanding the nuances and common pitfalls of the Strategy Pattern, you can become increasingly adept at crafting clean, efficient, and flexible code that stands the test of time. Happy coding!