Avoiding Common Java Pitfalls: Tips for Every Developer
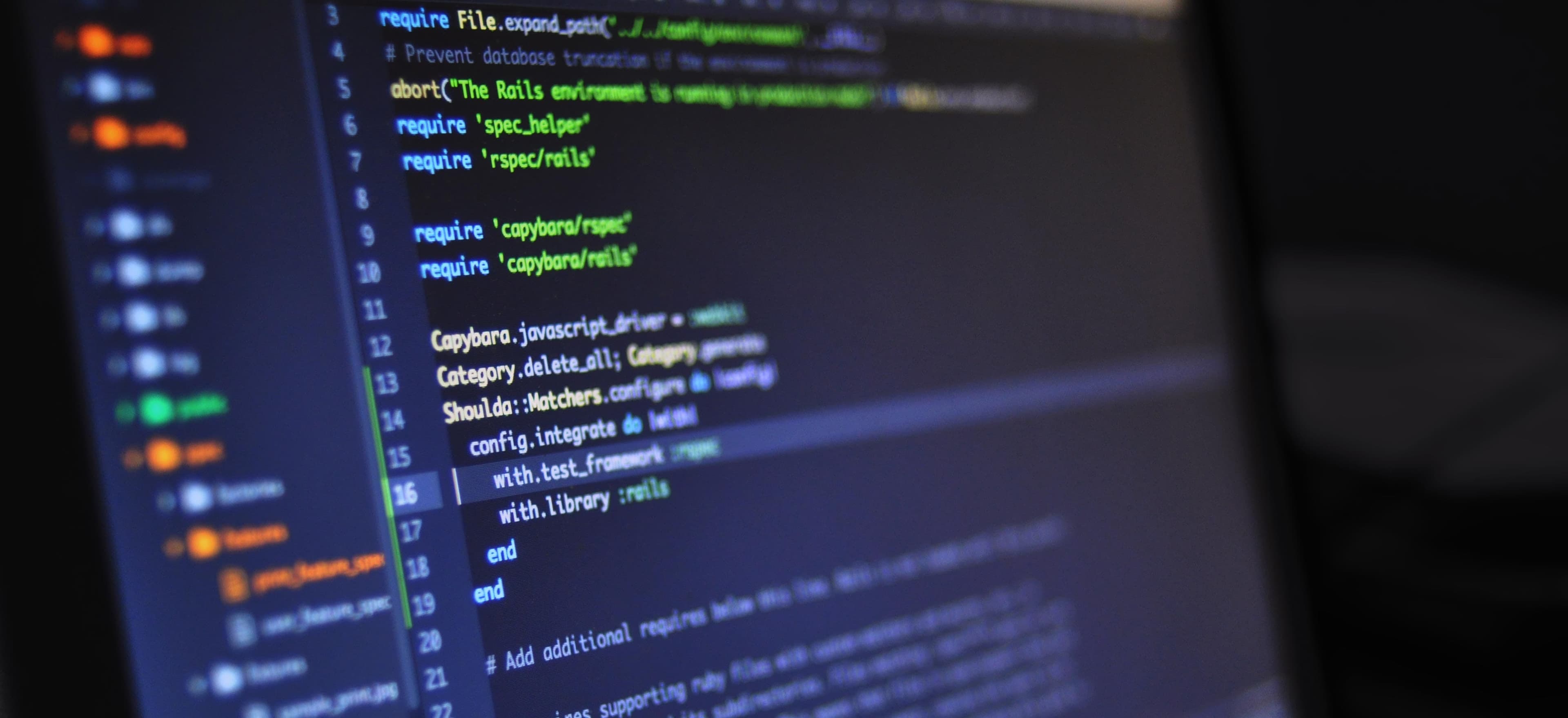
- Published on
Avoiding Common Java Pitfalls: Tips for Every Developer
Java is a powerful, versatile programming language widely used in various applications, from mobile to web development. However, like any programming language, Java is not without its common pitfalls. Understanding and avoiding these pitfalls can save developers time and effort, reduce bugs, and improve overall code quality. In this blog post, we’ll delve into some common Java mistakes and provide actionable tips for every developer.
1. NullPointerException: A Constant Frustration
One of the most notorious pitfalls in Java is the NullPointerException
. This exception occurs when your code attempts to use an object reference that has not been initialized or has been set to null
. Here’s an example:
public class Example {
public static void main(String[] args) {
String str = null;
System.out.println(str.length()); // Throws NullPointerException
}
}
Why It Happens
The root cause of this issue often lies in unchecked object initialization or not verifying whether an object is null
before accessing its methods or properties.
Avoiding It
- Use Optional: Java 8 introduced the
Optional
class, which provides a more robust way to handle null references. This allows you to express the "absence of a value" without throwing exceptions.
import java.util.Optional;
public class Example {
public static void main(String[] args) {
Optional<String> optionalStr = Optional.ofNullable(null);
System.out.println(optionalStr.orElse("Default Value")); // Outputs: Default Value
}
}
- Null Checks: Always perform null checks before accessing an object’s members.
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("String is null");
}
For more information, check out the Documentation for Optional.
2. Memory Leaks
Memory management in Java is generally handled by the garbage collector, but developers can still create memory leaks, particularly with long-lived object references.
How It Happens
A common scenario is maintaining references in collections that are never cleared. For instance:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private List<Object> list = new ArrayList<>();
public void addObject(Object obj) {
list.add(obj); // This will keep all the references until the program terminates
}
}
Preventive Measures
- Clear Collections: Make sure to clear collections when they are no longer needed.
public void clearObjects() {
list.clear(); // Clear references when done
}
- Weak References: Use
WeakReference
if you want to allow the garbage collector to reclaim objects.
import java.lang.ref.WeakReference;
public class Example {
private WeakReference<Object> weakRef;
public void createWeakReference(Object obj) {
weakRef = new WeakReference<>(obj);
}
}
3. Using the == Operator with Objects
In Java, using the ==
operator for object comparison may lead to unexpected results since it checks for reference equality rather than value equality.
Example of Pitfall
String str1 = new String("Hello");
String str2 = new String("Hello");
System.out.println(str1 == str2); // Outputs: false
Correct Method
For strings and most objects, always override the .equals()
method, or use it directly.
System.out.println(str1.equals(str2)); // Outputs: true
Why It Matters
Relying on ==
can lead to subtle bugs, especially when comparing object instances. Always use .equals()
unless you specifically need to check for reference equality.
4. Not Handling Exceptions Properly
Java has a rich exception handling mechanism, but many developers overlook its importance, leading to crashes and bugs.
Mistake Example
public void fileOperation() {
try {
// Some file operation
} catch (Exception e) {
// Ignoring the exception
}
}
Best Practices
- Specific Exceptions: Catch specific exceptions rather than a general Exception.
try {
// File operation
} catch (IOException e) {
e.printStackTrace();
}
- Finally Block: Use the
finally
block to close resources.
try {
// Code that may throw an exception
} finally {
// Close resources (like Streams, Files)
}
- Logging: Log exceptions to retain details for debugging.
private static final Logger logger = Logger.getLogger(Example.class.getName());
try {
// risky operation
} catch (IOException e) {
logger.log(Level.SEVERE, "Exception occurred", e);
}
5. Misusing Static Variables
Static variables share state across all instances of a class, which can lead to unintended consequences. If not managed correctly, they can disrupt the expected behavior of class instances.
Example of Misuse
public class Counter {
private static int count = 0;
public Counter() {
count++;
}
public static int getCount() {
return count;
}
}
When to Use
Use static variables when you truly need shared state across instances. If each instance requires a separate state, favor instance variables.
Key Takeaways
Java is an excellent language for building robust applications, but avoiding pitfalls is crucial for writing clean, maintainable code. By keeping these common pitfalls in mind, developers can streamline their workflow, minimize bugs, and produce high-quality software.
For more in-depth knowledge, consider exploring resources like the Java Tutorials or participating in forums like Stack Overflow to grasp various Java concepts.
Happy coding!
Checkout our other articles