Common Security Pitfalls in Java Application Development
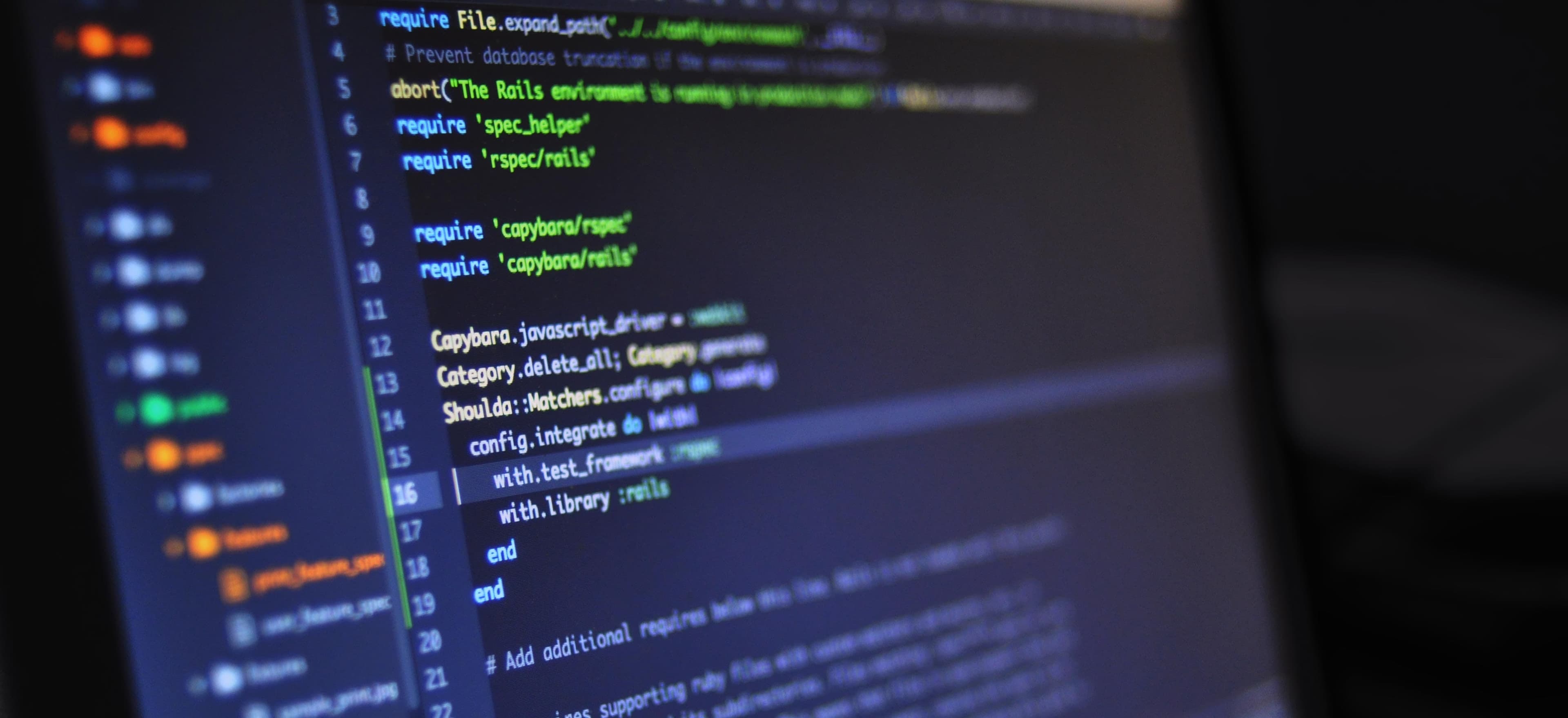
- Published on
Common Security Pitfalls in Java Application Development
Java is one of the most widely used programming languages for application development, largely due to its versatility, portability, and robustness. However, with great power comes great responsibility. As developers, it's critical to understand and mitigate the security risks that can arise during the software development life cycle (SDLC). In this post, we will explore common security pitfalls in Java application development and how to avoid them.
1. Ignoring Input Validation
Why It Matters
Input validation is the first line of defense against malicious attacks. Unchecked user input can lead to various types of vulnerabilities, including SQL injection, Cross-Site Scripting (XSS), and Remote Code Execution.
Code Example
import java.util.regex.*;
public class UserInput {
public static void validateInput(String userInput) {
String regex = "^[a-zA-Z0-9]*$"; // Allow only alphanumeric characters
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(userInput);
if (!matcher.matches()) {
throw new IllegalArgumentException("Invalid input!");
}
// Process input
}
}
Commentary
In this example, we use a regex to ensure that user input only consists of alphanumeric characters. If invalid input is detected, an exception is thrown, safeguarding against potentially harmful data reaching the application logic.
2. Hardcoded Credentials
Why It Matters
Storing credentials in source code is a risk that can lead to unauthorized access to sensitive data. It's especially dangerous if the code is ever stored in a version control system.
Code Example
import java.util.Properties;
import java.io.InputStream;
import java.io.IOException;
public class Configuration {
public static Properties loadProperties() throws IOException {
Properties properties = new Properties();
// Avoid hardcoding credentials
try (InputStream input = Configuration.class.getClassLoader().getResourceAsStream("config.properties")) {
properties.load(input);
}
return properties;
}
}
Commentary
In this updated code snippet, we load sensitive information such as credentials from an external properties file instead of hardcoding them. This approach not only improves security but also allows for easier updates and configuration changes without modifying the source code.
3. Inadequate Authentication and Authorization Mechanisms
Why It Matters
Weak authentication can lead to unauthorized access, risking data integrity and confidentiality. Similarly, improper authorization can grant users access to functionalities they should not possess.
Code Example
import java.util.HashMap;
import java.util.Map;
public class Authenticator {
private static Map<String, String> userDatabase = new HashMap<>();
static {
// Example user info
userDatabase.put("admin", "admin123");
}
public static boolean authenticate(String username, String password) {
if (userDatabase.containsKey(username)) {
return userDatabase.get(username).equals(password);
}
return false; // Invalid user
}
}
Commentary
In this simplistic authentication example, user credentials are checked against a hardcoded map. For production applications, it's essential to employ more robust mechanisms such as OAuth2 or JWT for secure authentication and authorization.
4. Lack of Secure Communication
Why It Matters
Sending sensitive information over unencrypted channels can lead to eavesdropping and data breaches. It is vital to always encrypt data in transit and at rest.
Code Example
import javax.net.ssl.*;
import java.io.*;
import java.net.*;
public class SecureConnection {
public static void main(String[] args) {
try {
SSLContext sslContext = SSLContext.getInstance("TLS");
// Initialize with default secure settings
sslContext.init(null, null, new java.security.SecureRandom());
SSLSocketFactory ssf = sslContext.getSocketFactory();
try (SSLSocket socket = (SSLSocket) ssf.createSocket("example.com", 443)) {
// Secure communication with the server
socket.startHandshake();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In this Java code snippet, we establish a secure SSL connection to a remote server. Utilizing TLS (Transport Layer Security) ensures encrypted communications. Understandably, any application transferring sensitive information should utilize secure protocols.
5. Failing to Implement Proper Exception Handling
Why It Matters
Improper exception handling can expose sensitive information about the application and its environment. It's essential to manage exceptions carefully to avoid leaking valuable information.
Code Example
public class ExceptionExample {
public void dangerousOperation() {
try {
// Code that can throw an exception
} catch (Exception e) {
// Log the exception without exposing the stack trace
System.err.println("An internal error occurred. Please contact support.");
}
}
}
Commentary
In this example, instead of printing the full stack trace, which could reveal sensitive internal information, we notify the user with a generic error message. Always log exceptions meaningfully without compromising security.
6. Outdated Libraries and Frameworks
Why It Matters
Using outdated third-party libraries can expose applications to known vulnerabilities. Regularly updating libraries ensures that applications are protected against exploits that target older versions.
Action Step
Developers should regularly review their dependency management files (like Maven's pom.xml
or Gradle's build.gradle
) and employ tools such as OWASP Dependency-Check or Snyk to identify vulnerabilities in dependencies.
The Bottom Line
Java application development is a powerful tool for creating robust software solutions. Nonetheless, neglecting security considerations can result in severe consequences. By remaining vigilant against common security pitfalls, such as input validation, credential management, authentication, secure communication, exception handling, and library updates, developers can create safer applications.
For further information on securing Java applications, check out these comprehensive resources from the OWASP Foundation or the Java Platform Security Guide.
Be proactive about security; it's not just an afterthought—it's an integral part of software development. Together, we can build applications that are not just functional but also secure.