Common Log4j2 Logging Pitfalls in Java Applications
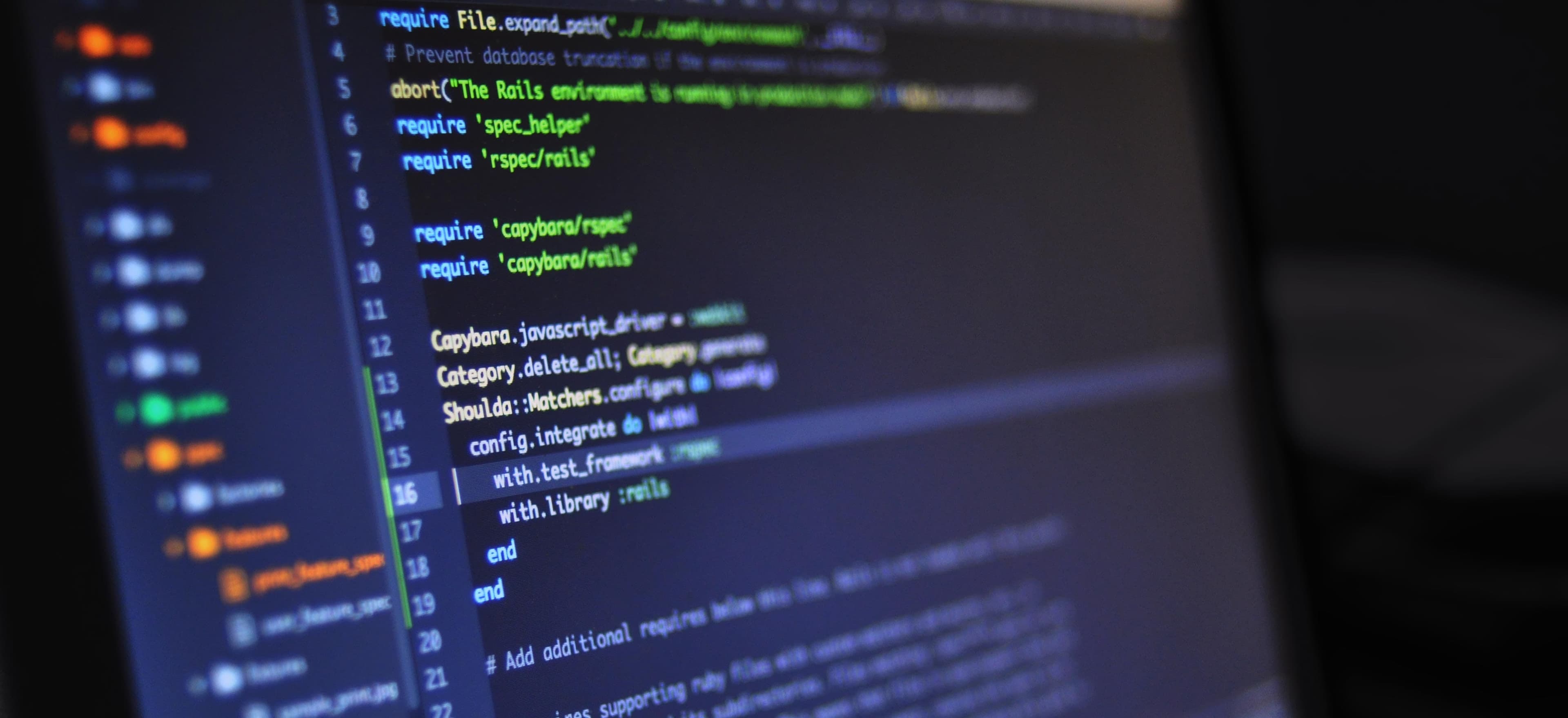
- Published on
Common Log4j2 Logging Pitfalls in Java Applications
Logging is a critical feature in any application, providing developers with the ability to trace events and understand the flow of their applications. Among the various logging frameworks available, Log4j2 has gained widespread popularity due to its flexibility and performance. However, like any tool, it has its pitfalls. In this post, we’ll explore common logging pitfalls encountered while using Log4j2 in Java applications and how to avoid them.
1. Ignoring Log Levels
One of the most common pitfalls is misunderstanding or misusing log levels. Log4j2 defines several log levels, such as TRACE, DEBUG, INFO, WARN, ERROR, and FATAL. Each level signifies the severity of the log message.
Example
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class ExampleClass {
private static final Logger logger = LogManager.getLogger(ExampleClass.class);
public void performAction() {
logger.debug("This is a debug message");
logger.info("This is an info message");
logger.error("This is an error message");
}
}
Why It Matters
Using debugging logs in a production environment can lead to performance bottlenecks and information overload, making it challenging to identify real issues. To avoid this, set the appropriate level for production and development environments. Check your log4j2.xml
configuration file or the logging settings before deploying your application.
2. Ineffective Logging Messages
Another pitfall is poor message quality. Logging is not just about recording information; it's about communication. If your log messages are unclear or lack context, troubleshooting becomes unnecessarily complicated.
Example
logger.info("Processed order");
Why It Matters
This message lacks context. Instead of simply stating that an order was processed, include relevant details.
Improved Example
logger.info("Processed order with ID: {} for customer: {}", orderId, customerId);
By including orderId
and customerId
, you provide valuable context, making it easier to trace an issue. Ensure your log messages are informative and structured.
3. Logging Sensitive Data
Logging sensitive information such as usernames, passwords, and personal identification numbers can be a critical security flaw. One common mistake is the lack of filtering or masking capabilities.
Example
logger.info("User logged in with username: {}", username);
The Risk
While it seems harmless, disclosing usernames can aid malicious actors in crafting targeted attacks. Avoid logging sensitive information altogether or use masking.
Safer Approach
logger.info("User logged in with username: {}", maskSensitiveData(username));
Implementing a Masking function
public String maskSensitiveData(String data) {
return data.replaceAll("(?<=\\w{2}).", "*"); // Masking with asterisks but keeping first two characters
}
By applying simple strategies such as this, you can enhance your application's security.
4. Overusing Exception Logging
Another common pitfall is logging exceptions inappropriately. Developers sometimes log exceptions without enough context. Simply catching and logging an error can lead to loss of critical information.
Example
try {
riskyOperation();
} catch (Exception e) {
logger.error("An error occurred", e);
}
Why It Matters
While this logs the error, it doesn't explain what the operation was, which can make diagnosing the issue difficult.
Improved Example
try {
riskyOperation();
} catch (Exception e) {
logger.error("Failed to perform riskyOperation. The error is: {}", e.getMessage(), e);
}
Providing Context
Adding context helps in understanding why the exception occurred, making it easier to debug.
5. Not Configuring Asynchronous Logging
Many developers overlook the performance gains made possible by asynchronous logging. Logging can create significant performance overhead, especially in high-traffic applications.
Example Configuration
To enable asynchronous logging in Log4j2, your log4j2.xml
should look like this:
<Configuration status="WARN">
<Appenders>
<Async name="AsyncLogger">
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{YYYY-MM-dd HH:mm:ss} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
</Async>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="AsyncLogger"/>
</Root>
</Loggers>
</Configuration>
Why It Matters
By processing log events asynchronously, your application can continue its tasks without waiting for the logging process to complete. This can significantly improve your application's performance.
6. Ignoring External Configurations
Hardcoding log configurations can lead to inconsistencies across environments. Many developers fail to externalize configurations, making it tedious to change settings without changing code.
Recommended Practice
Instead of hardcoding, use external configuration files. Log4j2's versatility allows you to alter configurations using different file formats such as XML, JSON, or YAML.
Example of External Configuration
You can add a log4j2.xml
file and specify its location in your application start-up.
-Dlog4j.configurationFile=/path/to/log4j2.xml
Why It Matters
By doing so, you will facilitate logging configuration changes based on different environments with minimal effort. This ensures responsiveness to any changes needed without redeploying the application.
In Conclusion, Here is What Matters
Logging is an essential element of software development, but it comes with its challenges, particularly when using frameworks like Log4j2. Understanding common pitfalls such as log level misuse, ineffective log messages, logging sensitive data, inappropriate exception logging, neglecting asynchronous features, and hardcoding configurations can lead to more maintainable and secure applications.
For more detailed insights into Log4j2 configurations, visit Apache's official documentation and explore the Log4j2 GitHub repository for best practices.
By being aware of these pitfalls and taking proactive steps, developers can significantly enhance their applications' reliability and security. Remember, effective logging can make all the difference between a headache and smooth troubleshooting.
Checkout our other articles