Mastering Apache Kafka: Common Java Integration Pitfalls
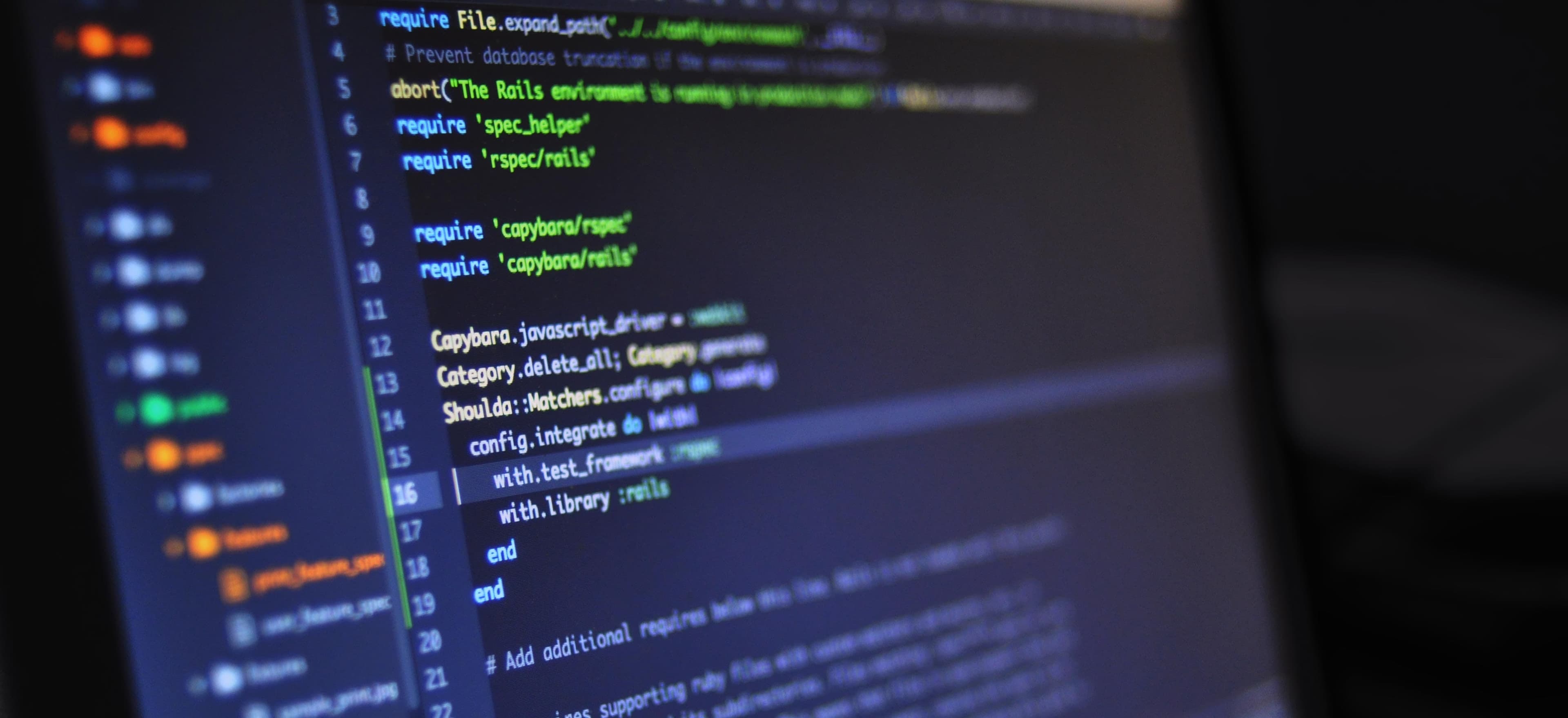
- Published on
Mastering Apache Kafka: Common Java Integration Pitfalls
Apache Kafka is an industry-standard tool for building real-time data pipelines and streaming applications. Its high throughput, scalability, and durability make it an appealing choice for developers seeking to manage data in motion. However, integrating Kafka with Java applications can come with its own set of challenges. In this post, we will explore common pitfalls and how to overcome them to ensure smooth Kafka integration with your Java applications.
Understanding Apache Kafka
Before diving into specifics, let's establish a foundational understanding of what Apache Kafka is. Kafka is a distributed event streaming platform capable of handling trillions of events a day. Key components of Kafka include:
- Producers: Applications that publish (write) messages to one or more Kafka topics.
- Consumers: Applications that subscribe to (read) messages from one or more topics.
- Brokers: Kafka servers that store data and serve client requests.
- Topics: Categories to which messages are published.
For a deeper understanding of Kafka’s architecture, consider this helpful resource: Apache Kafka Documentation.
Pitfall 1: Not Configuring Kafka Properly
One of the most common mistakes developers make is neglecting to apply the appropriate configurations. Kafka offers various settings that influence performance, message durability, and resource utilization.
Example Configuration
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("acks", "all");
Why This Matters
- Bootstrap Servers: Specifies the Kafka broker(s) to connect to. This is crucial for initial communication.
- Key and Value Serializer: Determines how keys and values are converted into bytes for transmission. Choosing the right serializer is essential for compatibility.
- Acknowledgment Settings (
acks
): This setting determines how many broker acknowledgments must be received before considering a request complete. Settingacks
to "all" guarantees that all replicas receive the message, ensuring high durability.
Failure to configure these settings can lead to performance bottlenecks or lost messages.
Pitfall 2: Ignoring Error Handling
In a production environment, error handling should never be an afterthought. Kafka integrates smoothly with Java exceptions, but mishandling these errors can cause your application to crash or lose valuable data.
Example Error Handling
try {
producer.send(new ProducerRecord<>(topic, key, value)).get();
} catch (ExecutionException e) {
System.err.println("Error sending message: " + e.getMessage());
// Add proper logging and handling here
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
Why This Matters
Proper error handling ensures that you gracefully manage unexpected situations. Failing to catch exceptions can lead to application downtime. Implementing logging and retry mechanisms can help you maintain the resilience of your applications.
Pitfall 3: Mishandling Consumer Offsets
One of the significant challenges with Kafka consumers is managing offsets. Offsets represent the position of a consumer in a topic and determine which messages have been processed.
Example Consumer Configuration
props.put("enable.auto.commit", "false");
Why This Matters
Setting enable.auto.commit
to false
allows you to manage offsets manually. This is crucial because:
- You avoid losing messages in case of failures.
- You gain control over when offsets are committed, allowing you to reprocess messages if necessary.
However, this adds complexity. You must ensure that offsets are committed only after successful message processing.
Pitfall 4: Lack of Monitoring
Monitoring your Kafka consumers and producers is essential for diagnosing performance issues and ensuring reliability.
Monitoring Tools
Consider using Kafka Manager or Confluent Control Center for comprehensive monitoring capabilities.
Why This Matters
Without proper monitoring, you may overlook important metrics such as:
- Consumer lag: The difference between the highest offset produced and the offset being consumed.
- Error rates: The percentage of messages failing to process.
- Throughput and latency: Metrics indicating the speed and efficiency of your system.
Setting up alerts for these metrics enables proactive action towards potential issues.
Pitfall 5: Neglecting Security
Security is often sidelined in many Kafka applications, yet it's a critical element for any production system. Kafka supports various security mechanisms such as SSL, SASL, and ACLs (Access Control Lists).
Example Security Configuration
props.put("security.protocol", "SASL_PLAINTEXT");
props.put("sasl.mechanism", "PLAIN");
Why This Matters
Implementing security configurations protects your data both in transit and at rest. Given the sensitivity of the data often managed by Kafka, neglecting these configurations can expose your application to vulnerabilities.
The Last Word
Integrating Apache Kafka with Java can feel overwhelming at first, but avoiding the common pitfalls outlined in this post will set you on a path to success. As with any technology, understanding the hows and whys behind the code you write makes a world of difference. Make sure to focus on proper configuration, robust error handling, manual offset management, monitoring practices, and security measures. By doing so, you can harness the power of Kafka effectively and efficiently.
For further reading, visit:
- Kafka for Java Developers: Getting Started
- Best Practices for Securing Apache Kafka
Mastering the integration of Apache Kafka into your Java applications may take time, but the benefits far outweigh the initial learning curve. Now, start applying these lessons in your projects! Happy coding!