Common JavaFX Pitfalls: Avoiding GUI Development Mistakes
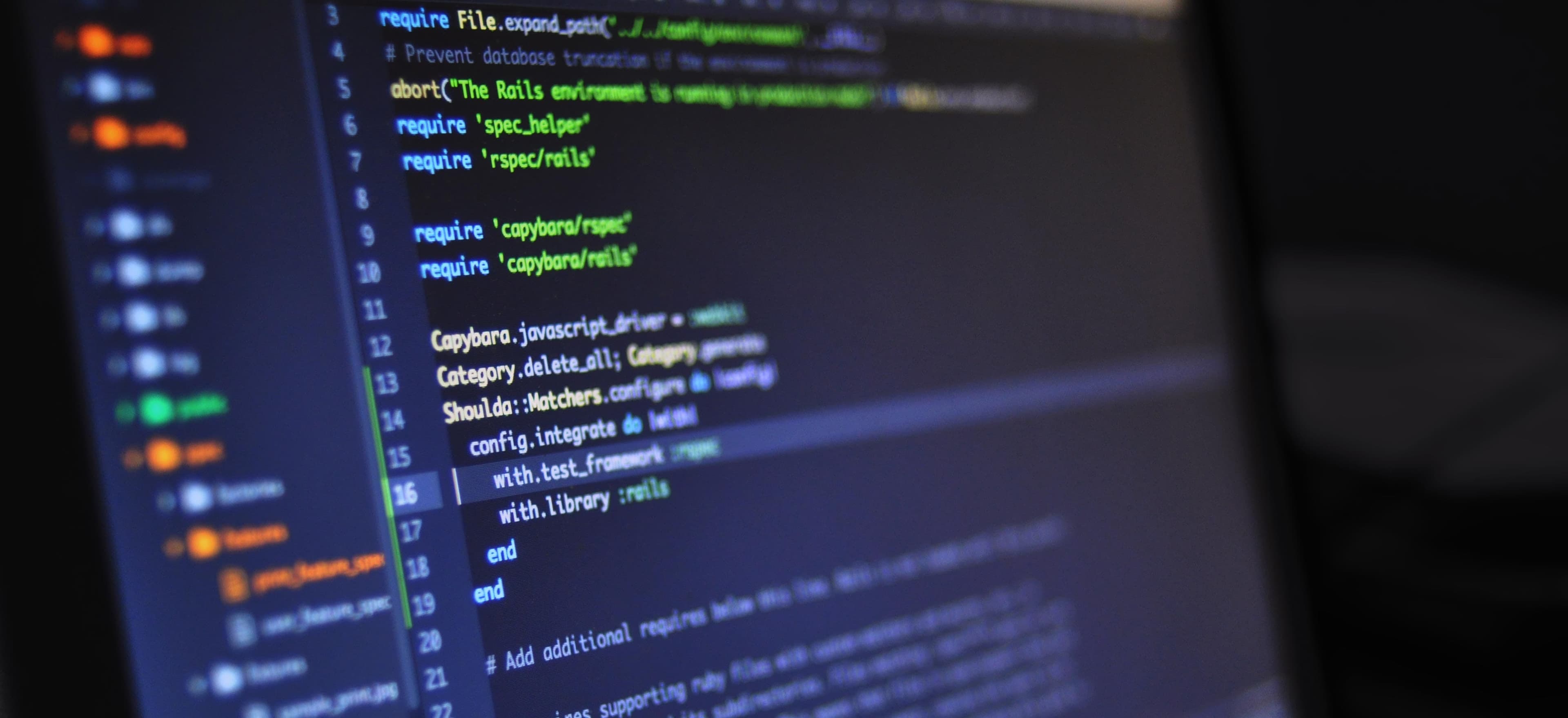
- Published on
Common JavaFX Pitfalls: Avoiding GUI Development Mistakes
JavaFX is a powerful tool for building graphical user interfaces (GUIs) in Java applications. With its rich set of features and capabilities, it can help developers create highly responsive and visually appealing applications. However, like any technology, there are pitfalls that developers often encounter. In this blog post, we will cover some common JavaFX mistakes and how to avoid them, ensuring you can build high-quality applications efficiently.
Table of Contents
- 1. Understanding the JavaFX Application Lifecycle
- 2. Improper Use of the JavaFX Thread
- 3. Ignoring FXML Best Practices
- 4. Unoptimized Scene Graph Management
- 5. Neglecting CSS for Styling
- 6. Overcomplicating Event Handling
- 7. Inefficient Resource Management
- 8. Conclusion
1. Understanding the JavaFX Application Lifecycle
Before diving into coding, it is crucial to have a clear understanding of the JavaFX application lifecycle. JavaFX applications go through a specific sequence of stages—initialization, UI construction, and event handling.
Key Points:
init()
Method: Used for initializing resources or configurations before the primary stage is created.start(Stage stage)
: The main entry point for the GUI, where you will set up your scene graph.stop()
Method: Called to clean up resources when the application is closed.
Why It Matters: Misunderstanding the lifecycle can lead to resource leaks, unintended application behavior, or failure to initialize components properly.
Example:
@Override
public void init() throws Exception {
// Initialize resources here
System.out.println("Initializing application resources.");
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("JavaFX Application");
// Set up scene and show stage here
}
For more on the lifecycle of a JavaFX application, check out Oracle's Documentation.
2. Improper Use of the JavaFX Thread
JavaFX is single-threaded by design. This means that all UI updates must occur on the JavaFX Application Thread. If you attempt to modify UI elements from any other thread, you will encounter exceptions.
Key Points:
- Always use the
Platform.runLater()
method to update the UI from any background thread.
Example:
new Thread(() -> {
String updateMessage = "Background task complete.";
Platform.runLater(() -> {
// Update any UI elements here safely
label.setText(updateMessage);
});
}).start();
Why It Matters: This avoids concurrency issues and ensures that your UI updates seamlessly, leading to a smoother user experience.
3. Ignoring FXML Best Practices
FXML enables separation of design and logic in JavaFX applications, but improper use can lead to a messy codebase. It's essential to decorate your FXML files and controller classes properly.
Key Points:
- Use
@FXML
to link your FXML components to Java controllers. - Keep your FXML files clean and well-organized.
Example:
<Button fx:id="myButton" text="Click Me" onAction="#handleButtonClick"/>
In the corresponding controller:
@FXML
private Button myButton;
@FXML
private void handleButtonClick() {
// Button click handler
}
Why It Matters: Using FXML correctly not only enhances readability but also makes maintaining the code more manageable.
4. Unoptimized Scene Graph Management
When working with JavaFX, you'll interact with the scene graph frequently. Overpopulating or deeply nesting your scene graph can lead to performance issues.
Key Points:
- Avoid unnecessary layers and groups.
- Use layout managers effectively to minimize complexity.
Example:
VBox layout = new VBox();
layout.getChildren().addAll(new Button("Button 1"), new Button("Button 2"));
Why It Matters: A simpler scene graph results in better rendering performance, improving your application's responsiveness.
5. Neglecting CSS for Styling
JavaFX allows for extensive styling using CSS, yet many developers overlook this powerful feature, opting instead for manual styling in code.
Key Points:
- Use CSS for easy management and consistency across UI components.
Example:
.button {
-fx-background-color: #009688;
-fx-text-fill: white;
}
In your Java code, include:
scene.getStylesheets().add("style.css");
Why It Matters: CSS enables you to manage complex designs while keeping your Java code clean. For a deeper dive into JavaFX CSS styling, visit JavaFX CSS Reference Guide.
6. Overcomplicating Event Handling
Event handling in JavaFX should be straightforward. However, developers sometimes add unnecessary complexity by overusing custom events or listeners.
Key Points:
- Keep your event handling simple and direct.
- Use lambda expressions for cleaner implementations.
Example:
myButton.setOnAction(event -> {
System.out.println("Button clicked!");
});
Why It Matters: Simplified event handling makes your code easier to read, test, and maintain.
7. Inefficient Resource Management
JavaFX applications often include images, styles, or other resources. Failing to manage these resources efficiently can lead to increased memory usage and poor performance.
Key Points:
- Load resources lazily, and utilize caching effectively.
Example:
Image image = new Image("file:resources/image.png", false);
ImageView imageView = new ImageView(image);
Why It Matters: Efficient resource management prevents memory leaks and optimizes your application's performance.
8. Conclusion
JavaFX offers a wealth of possibilities for creating stunning graphical applications. However, if you are not aware of common pitfalls such as improper thread handling, overcomplicated event management, or resource mismanagement, you may run into challenges.
By understanding the lifecycle of JavaFX applications, engaging with FXML properly, utilizing CSS for styling, and applying efficient event and resource management techniques, you can significantly improve the quality of your GUI applications.
For further reading, consider exploring additional resources on the JavaFX Documentation or diving into some tutorials on JavaFX best practices.
Happy coding!