Resolving Common JMS Messaging Issues in Java
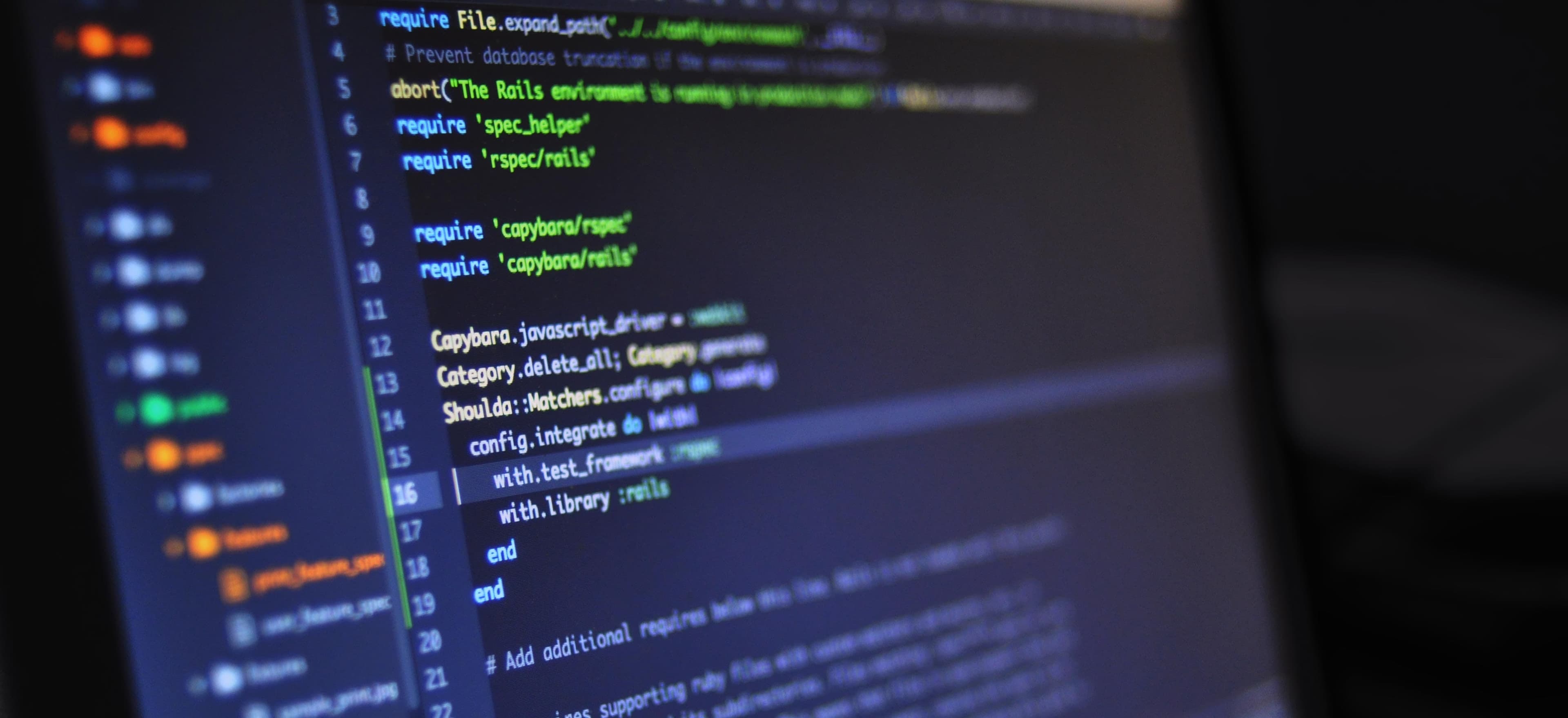
- Published on
Resolving Common JMS Messaging Issues in Java
Java Message Service (JMS) is a powerful feature in Java that enables communication between different components of a distributed application through messaging. However, while working with JMS, developers often encounter a variety of issues that can affect message production and consumption. This blog post will discuss some common JMS messaging issues and provide guidance on resolving them.
What is JMS?
Before diving into the issues, it's crucial to understand what JMS is. JMS is an API that allows Java applications to create, send, receive, and read messages. It’s a part of the Java EE platform and provides a way for resources to communicate with each other in a loosely coupled manner, which is significant for building distributed systems.
Common JMS Messaging Issues
1. Connection Issues
One of the most common issues encountered when working with JMS is connection problems. This can occur for several reasons, such as:
-
Incorrect Connection URL: Make sure that the endpoint you are trying to connect to is correctly specified. A slight mistake in the URL can prevent the connection.
-
Network Issues: Check for issues in the network that may be blocking the connection.
-
Broker Down: The JMS broker may be down or overloaded.
Sample Code to Handle Connection
import javax.jms.Connection;
import javax.jms.ConnectionFactory;
import javax.jms.JMSException;
public class JMSConnectionManager {
private Connection connection;
public void createConnection(ConnectionFactory connectionFactory) {
try {
connection = connectionFactory.createConnection();
connection.start();
System.out.println("Connection established successfully.");
} catch (JMSException e) {
System.err.println("Failed to create JMS connection: " + e.getMessage());
}
}
public void closeConnection() {
if (connection != null) {
try {
connection.close();
System.out.println("Connection closed successfully.");
} catch (JMSException e) {
System.err.println("Failed to close JMS connection: " + e.getMessage());
}
}
}
}
Why This Code Matters: The code establishes a connection to the JMS broker and ensures that you handle exceptions properly. It demonstrates how to both open and close connections efficiently, which is crucial for maintaining performance and resource management.
2. Message Delivery Delays
Message delivery delays can occur due to several factors, including:
-
Network Latency: High latency can lead to slow message delivery.
-
Configuration Errors: Misconfigured queues or topics can prevent messages from being processed promptly.
-
Subscription Issues: If the consumers are not available, messages may pile up in the queue.
Troubleshooting Steps
- Verify network performance using ping and traceroute commands.
- Check JMS configuration on the broker console for discrepancies.
3. Transaction Management Issues
JMS supports transactions, which is designed to ensure message delivery and processing integrity. However, improper transaction handling can cause issues such as:
-
Messages Not Being Consumed: If a transaction is not committed, messages may remain in the queue forever.
-
Duplicate Messages: If a transaction fails and is retried without proper management, duplicates can occur.
Sample Code for Transaction Management
import javax.jms.*;
public class JMSTransactionExample {
private Connection connection;
private Session session;
public void processMessages(ConnectionFactory connectionFactory, Destination destination) throws JMSException {
connection = connectionFactory.createConnection();
session = connection.createSession(true, Session.SESSION_TRANSACTED);
MessageProducer producer = session.createProducer(destination);
MessageConsumer consumer = session.createConsumer(destination);
try {
TextMessage message = session.createTextMessage("Hello, world!");
producer.send(message);
System.out.println("Message sent.");
TextMessage receivedMessage = (TextMessage) consumer.receive(1000);
System.out.println("Received: " + receivedMessage.getText());
session.commit(); // Commit the transaction
System.out.println("Transaction committed.");
} catch (JMSException e) {
session.rollback(); // Rollback the transaction on error
System.err.println("Transaction rolled back: " + e.getMessage());
} finally {
consumer.close();
producer.close();
session.close();
connection.close();
}
}
}
Why Transaction Handling is Important: This code emphasizes the importance of managing transactions effectively in JMS. Using sessions allows us to either commit or rollback changes depending on success or failure, thereby preventing message loss or duplication.
4. Message Formats and Serialization Issues
When sending messages, it's important to ensure that the data format matches what the consumer expects. Serialization issues can arise from:
-
Mismatched Message Types: Ensure that the producer and consumer agree on the message type.
-
Invalid Serialization: If your objects are not Serializable, attempting to send them will raise exceptions.
Code to Demonstrate Serialization
import javax.jms.*;
public class MessageProducerExample {
public void sendObjectMessage(Session session, Destination destination) {
try {
ObjectMessage objectMessage = session.createObjectMessage();
MyCustomObject myObject = new MyCustomObject("Sample Data");
objectMessage.setObject(myObject);
session.createProducer(destination).send(objectMessage);
System.out.println("Object message sent successfully.");
} catch (JMSException e) {
System.err.println("Failed to send object message: " + e.getMessage());
}
}
public static class MyCustomObject implements java.io.Serializable {
private String data;
public MyCustomObject(String data) {
this.data = data;
}
public String getData() {
return data;
}
}
}
Why Serialization Matters: It's crucial to make sure that the classes being sent as messages implement Serializable
. This sample demonstrates how to send custom objects, elaborating the serialization aspect in ensuring smooth messaging across different parts of an application.
5. Consumer Issues
Sometimes, consumers may fail to process messages due to:
-
Long Processing Times: If a consumer takes too long to process a message, other messages may be delayed.
-
Exception Handling: Uncaught exceptions in consumers can cause them to stop consuming messages.
Best Practices for Consumers
- Use asynchronous consumption techniques.
- Implement proper exception handling to avoid consumer shutdown.
Relevant Links for Further Learning
For more detailed knowledge on JMS, you may explore:
- Oracle's JMS Documentation
- Effective Java Messaging from Baeldung.
Final Thoughts
Managing JMS messaging effectively involves understanding and resolving various potential issues that can arise in application communication. By adhering to best practices, including connection management, transaction handling, serialization considerations, and ensuring efficient consumer processing, developers can ensure a smooth messaging experience.
Remember, while JMS is an excellent tool for enabling communication in a Java enterprise setup, understanding its nuances and challenges is key to building robust applications.
Lessons Learned
In summary, while JMS can introduce complexity into your Java applications, a clear understanding of common issues and how to address them can significantly enhance the reliability of your messaging system. Thoroughly monitoring, testing, and understanding the configuration will help you mitigate the pitfalls mentioned above.
Do you have any other JMS-related challenges? Let’s discuss solutions in the comments below!