The Hidden Complexity of Java ClassLoaders Explained
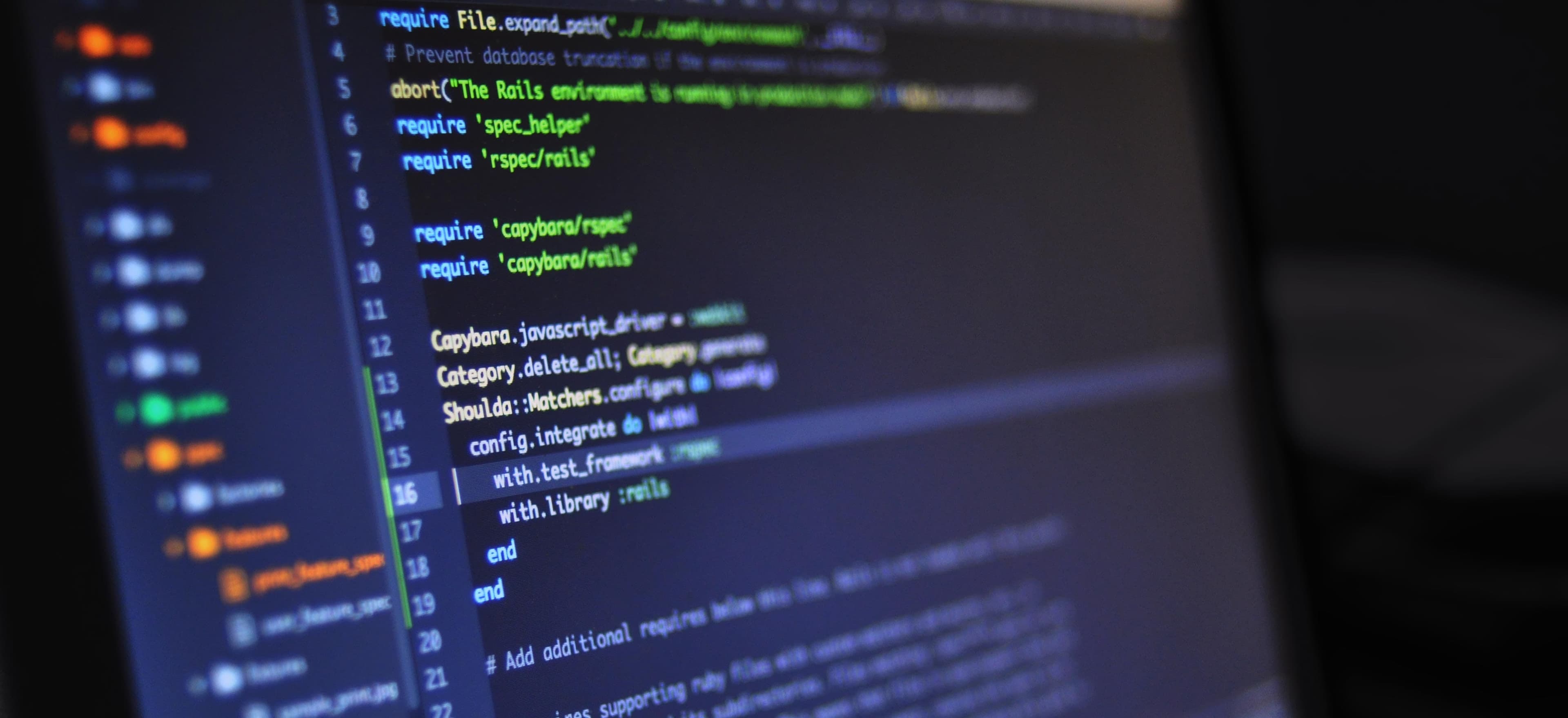
- Published on
The Hidden Complexity of Java ClassLoaders Explained
Java is a powerful, versatile programming language widely used in enterprise development and large-scale applications. At its core, the language brings with it a robust runtime environment that delivers significant features and functionalities. One of the most complex yet vital components of this environment is the ClassLoader. In this blog post, we'll unravel the hidden complexities of Java ClassLoaders, exploring their types, functionalities, and practical implications for developers.
What is a ClassLoader?
In simple terms, a ClassLoader in Java is a part of the Java Runtime Environment that loads classes into memory. It is responsible for dynamically loading classes when they are needed during the execution of a program. This process occurs primarily through the use of bytecode, which Java compiles from its source code.
The Role of ClassLoaders
ClassLoaders serve several key roles:
- Dynamic Loading: Classes can be loaded at runtime without any need for prior compilation.
- Namespace Management: Each ClassLoader provides a namespace, ensuring that classes with the same name can exist in different contexts without conflict.
- Memory Management: ClassLoaders help manage memory more efficiently by cleaning up classes that are no longer needed.
The Hierarchy of ClassLoaders
Java ClassLoaders operate within a hierarchy, which consists of three primary types:
-
Bootstrap ClassLoader:
- This is the root of the class loading hierarchy. It is part of the Java Virtual Machine (JVM) and loads the core classes such as
java.lang.*
,java.util.*
, and other essential classes that come with the Java Runtime Environment (JRE). - It's worth noting that the Bootstrap ClassLoader is implemented in native code and does not extend the
java.lang.ClassLoader
class.
- This is the root of the class loading hierarchy. It is part of the Java Virtual Machine (JVM) and loads the core classes such as
-
Extension ClassLoader:
- This loader is responsible for loading classes from the extension directories, typically found in the
jre/lib/ext
folder. - It provides additional libraries to Java applications and enhances modularity.
- This loader is responsible for loading classes from the extension directories, typically found in the
-
System/Application ClassLoader:
- The System ClassLoader is responsible for loading application classes from the classpath, and it's the most commonly used ClassLoader in many Java applications.
- The classpath can include directories, JAR files, and ZIP archives, making this loader flexible.
Here’s a simple representation of the ClassLoader hierarchy:
Bootstrap ClassLoader
|
Extension ClassLoader
|
System/Application ClassLoader
Understanding this hierarchy is critical for developers, as it helps debug class-loading-related issues more effectively.
Custom ClassLoader: An Example
While Java provides default ClassLoaders, there are scenarios where custom ClassLoaders are necessary. For instance, when loading classes from a non-standard source (like a remote server) or when developing modular applications.
Code Snippet: Creating a Custom ClassLoader
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class CustomClassLoader extends ClassLoader {
private String path;
public CustomClassLoader(String path) {
this.path = path;
}
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
byte[] classData = loadClassData(name);
if (classData == null) {
throw new ClassNotFoundException("Class not found: " + name);
}
return defineClass(name, classData, 0, classData.length);
}
private byte[] loadClassData(String className) {
String filePath = path + File.separator + className.replace('.', File.separatorChar) + ".class";
try (InputStream inputStream = new FileInputStream(filePath)) {
byte[] data = new byte[inputStream.available()];
inputStream.read(data);
return data;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
// Example usage
public static void main(String[] args) {
try {
CustomClassLoader loader = new CustomClassLoader("path/to/classes");
Class<?> cls = loader.loadClass("com.example.MyClass");
System.out.println("Class Loaded: " + cls.getName());
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Explanation:
- Extending ClassLoader: A custom ClassLoader extends the default
ClassLoader
class. - findClass Method: This method attempts to locate and load the required class.
- loadClassData Method: It reads the class file from the file system, converting it into a byte array.
The code snippet above demonstrates how we can programmatically load classes from a specified directory. Understanding how to implement a Custom ClassLoader is essential for extending Java's capabilities based on specific application needs.
ClassLoader and the Java Memory Model
It's important to discuss how ClassLoaders interact with the Java Memory Model. When a ClassLoader loads a class, it creates a unique representation of that class in the Java heap. This representation is tied to the ClassLoader that loaded it. As a result, classes loaded by different ClassLoaders can coexist without conflict, which is especially useful for web applications that might load the same library under different contexts.
Practical Implications for Developers
Here are some implications and best practices developers should consider:
- Avoid ClassLoader Confusion: Be cautious about loading the same classes in different ClassLoaders. It may lead to classcast exceptions during runtime.
- Use for Modular Architecture: Leverage Custom ClassLoaders in modular applications or plugin architectures where you need different versions of the same library.
- Diagnosing Issues: When experiencing
ClassNotFoundException
, check your ClassLoader hierarchy. It often holds clues to loading issues.
ClassLoader Performance Considerations
Custom ClassLoaders may introduce performance overhead if not implemented correctly. It's crucial to cache loaded classes to avoid repeated loading:
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Check cache first
Class<?> cachedClass = classCache.get(name);
if (cachedClass != null) {
return cachedClass;
}
byte[] classData = loadClassData(name);
if (classData == null) {
throw new ClassNotFoundException("Class not found: " + name);
}
Class<?> clazz = defineClass(name, classData, 0, classData.length);
classCache.put(name, clazz); // Cache the loaded class
return clazz;
}
In this refined version of the findClass
method, we’re implementing a caching mechanism that will store loaded class references. This small modification can result in substantial performance gains by preventing unnecessary reloading of classes.
Final Considerations
The intricacies of Java ClassLoaders may seem daunting at first, but a solid understanding is crucial for any serious Java developer. From managing class loading hierarchies to implementing Custom ClassLoaders, these components provide a foundation for more robust application architectures.
For more detailed explorations of Java and related technologies, consider visiting Java Official Documentation or Baeldung's Java Tutorials. These resources offer additional insights and examples, aiding your journey toward mastering advanced Java concepts.
By comprehending how ClassLoaders work, developers can enhance the performance, security, and modularity of their Java applications, ultimately leading to more maintainable and efficient code. Happy coding!