Common JavaMail API Pitfalls and How to Avoid Them
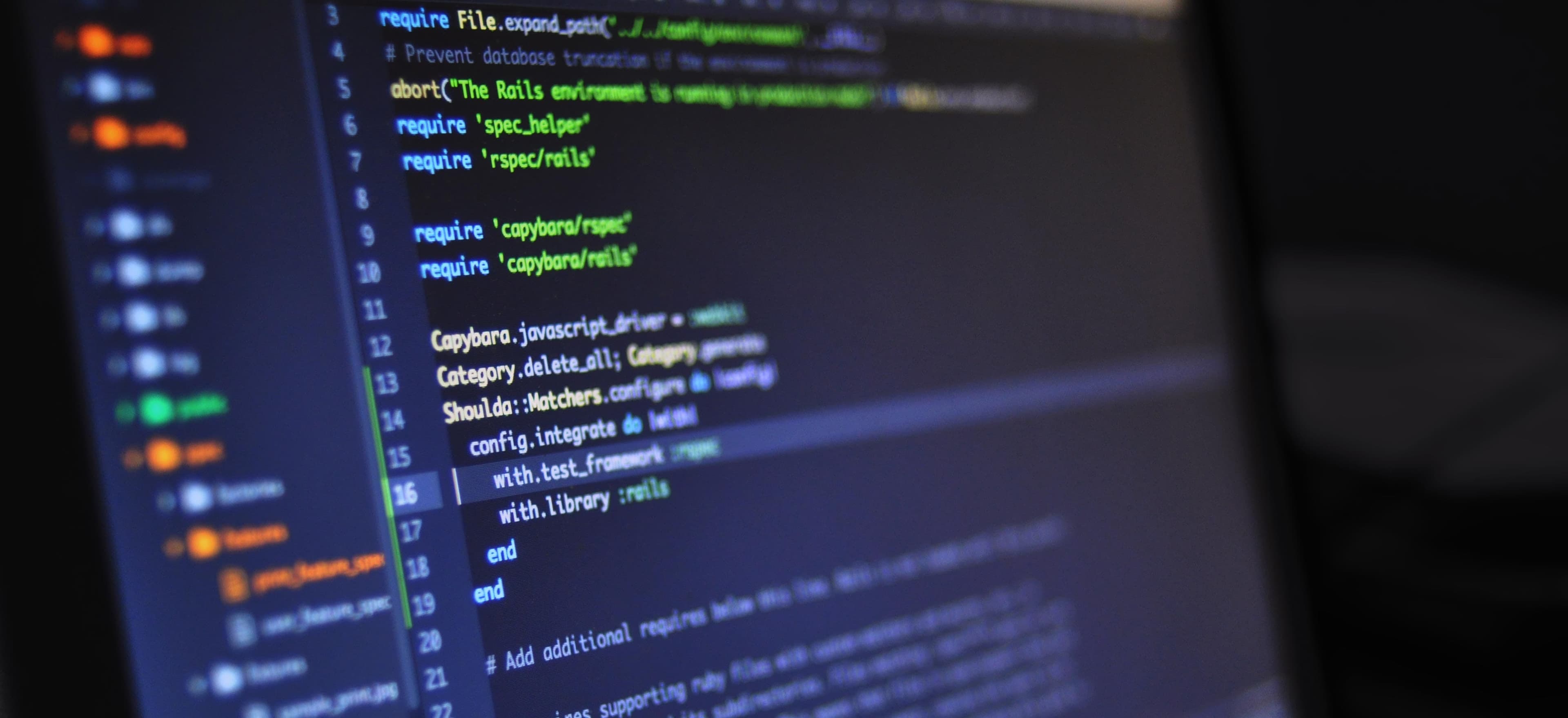
- Published on
Common JavaMail API Pitfalls and How to Avoid Them
The JavaMail API is a powerful tool for sending and receiving email for Java applications. It is widely used for creating applications that manage email services. However, working with JavaMail can lead to common pitfalls that many developers encounter. In this blog post, we will discuss these pitfalls and how you can effectively avoid them.
Understanding JavaMail API
Before diving into the pitfalls, let's establish what the JavaMail API is. The API allows you to send and receive emails from Java applications. It abstracts the complexities of email communication and provides a simple interface to work with multiple email protocols (SMTP, POP3, IMAP).
The essential setup for using JavaMail involves:
- Dependency Management: You need the JavaMail API included in your project.
- Proper Configuration: Correctly configuring the properties like SMTP server address, authentication, etc.
Example: Simple Email Sending
import javax.mail.*;
import javax.mail.internet.*;
import java.util.Properties;
public class EmailSender {
public static void sendEmail(String to, String subject, String body) {
// Setting up SMTP server properties
Properties properties = System.getProperties();
properties.put("mail.smtp.host", "smtp.example.com");
properties.put("mail.smtp.port", "587");
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
// Authenticating the SMTP session
Session session = Session.getInstance(properties, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("username", "password");
}
});
try {
// Constructing the email message
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress("from@example.com"));
message.addRecipient(Message.RecipientType.TO, new InternetAddress(to));
message.setSubject(subject);
message.setText(body);
// Sending the email
Transport.send(message);
System.out.println("Email sent successfully.");
} catch (MessagingException mex) {
mex.printStackTrace();
}
}
}
In this code, we define a method sendEmail
that takes recipient information and sends an email. The properties define SMTP communication settings; these must be accurately configured to ensure email delivery.
Common Pitfalls
Now that you're familiar with basic email sending, let’s address some common pitfalls developers face when using the JavaMail API.
1. Misconfigured SMTP Properties
Pitfall: One of the most frequent mistakes is misconfiguring SMTP server properties. This could be the server address, port, or authentication requirements.
Solution: Always verify your SMTP settings with your email provider. Ensure you’re using the correct server address and authentication methods. Example:
properties.put("mail.smtp.port", "587"); // Use 587 for TLS, 465 for SSL
properties.put("mail.smtp.auth", "true"); // Essential for authentication
2. Forgetting to Handle Exceptions
Pitfall: Neglecting to handle exceptions appropriately can lead to your application crashing silently.
Solution: Ensure robust error handling. This not only helps in debugging but also improves user experience. Use logging frameworks like SLF4J, or simply print stack traces for development purposes.
try {
// Sending email code
} catch (MessagingException mex) {
System.out.println("Error occured: " + mex.getMessage());
// Consider using a dedicated logger
}
3. Using Incorrect Email Format
Pitfall: Sending emails with incorrect formats can lead to undelivered emails or hard bounces.
Solution: Validate email addresses before sending. You can use regex or libraries designed for email validation.
public static boolean isValidEmail(String email) {
String regex = "^[A-Za-z0-9+_.-]+@(.+)$";
return email.matches(regex);
}
4. Not Using TLS/SSL
Pitfall: Failing to enable TLS/SSL for email communication can expose sensitive information.
Solution: Always use SSL/TLS when sending emails. In your properties, ensure to enable it as shown earlier.
5. Not Including Proper Email Headers
Pitfall: Missing important headers can lead to issues with email deliverability, spam filters, or organizational policies.
Solution: Pay attention to necessary headers like "From", "To", "Subject", and consider adding "Reply-To" and custom headers as needed.
message.setFrom(new InternetAddress("from@example.com", "MyApp"));
message.setReplyTo(InternetAddress.parse("support@example.com"));
6. Ignoring Character Encoding
Pitfall: Sending emails with improper character encoding can result in garbled text, especially for non-ASCII characters.
Solution: When setting the body of the email, specify a character set, such as UTF-8.
message.setText(body, "UTF-8");
7. Not Cleaning Up Resources
Pitfall: Resources like sessions and transports should be properly closed to avoid memory leaks.
Solution: Use Transport.close()
when you’re done with sending emails.
8. Over-Relying on Sending Emails
Pitfall: Relying solely on the JavaMail API for all forms of notifications could lead to issues if email services go down.
Solution: Consider implementing a fallback mechanism, such as logging notifications or alternative communication methods (SMS, push notifications).
In Conclusion, Here is What Matters
In summary, the JavaMail API is a robust solution for managing email communication in Java applications. However, by being aware of common pitfalls, you can ensure a smoother development experience and more reliable email delivery.
To enhance your email functionalities further, explore the JavaMail API documentation for advanced features. Additionally, consider integrating your application with services like SendGrid or Mailgun for scalable email solutions.
With these best practices and insights, you can effectively harness the power of the JavaMail API while avoiding the common pitfalls that plague many developers. Happy coding!