Mastering JNI: Common Pitfalls and How to Avoid Them
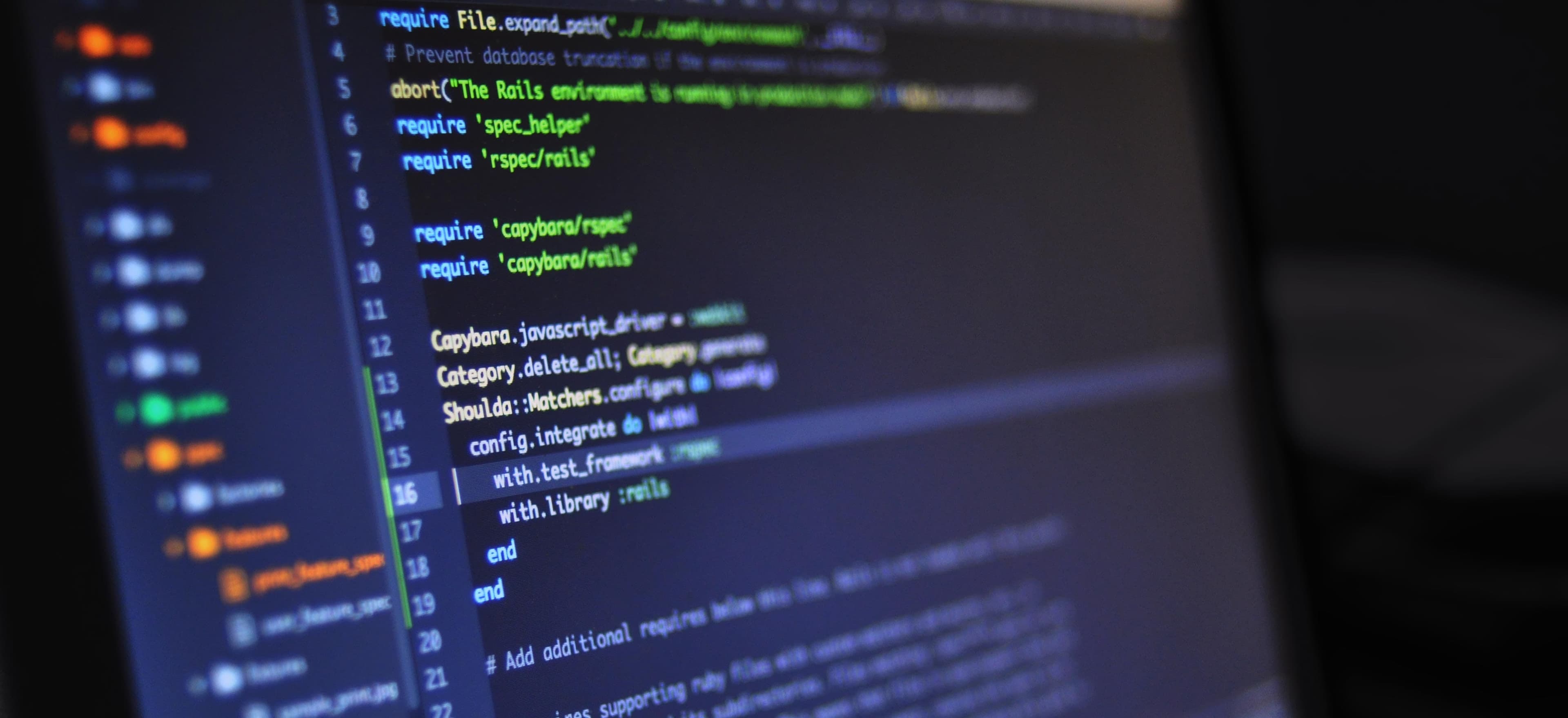
- Published on
Mastering JNI: Common Pitfalls and How to Avoid Them
Java Native Interface (JNI) is a powerful feature that allows Java code to interface with native applications and libraries written in languages like C or C++. While JNI provides great flexibility, it also introduces complexity and potential pitfalls. In this blog post, we will explore common issues developers encounter when using JNI and how to avoid them.
What is JNI?
JNI is a programming framework that enables Java code running in the Java Virtual Machine (JVM) to call and be called by native applications and libraries. It is particularly useful for performance-critical applications where developers need to take advantage of existing C/C++ codebases or require direct access to system resources.
Why Use JNI?
- Performance: JNI allows you to leverage optimized native libraries for computationally intensive tasks.
- Legacy Integration: Many systems still rely on existing C/C++ code; JNI enables integration without rewriting the entire application.
- Platform-Specific Features: JNI facilitates access to platform-specific features and capabilities that are not exposed through the Java Standard Library.
Common Pitfalls in JNI
While JNI can be a powerful tool, it comes with its own set of challenges. Here are some of the most common pitfalls developers face when writing JNI code.
1. Memory Management Issues
Problem
In C/C++, memory management is manual. If you forget to free memory allocated with malloc
or new
, you create memory leaks, which can lead to performance issues and crashes.
Solution
Always ensure to free memory once you are done using it. Use a structured approach to manage memory allocation and deallocation. For example:
#include <jni.h>
#include <stdlib.h>
JNIEXPORT jstring JNICALL Java_MyClass_getString(JNIEnv *env, jobject obj) {
char *nativeString = (char *)malloc(20 * sizeof(char));
strcpy(nativeString, "Hello from C!");
jstring result = (*env)->NewStringUTF(env, nativeString);
free(nativeString); // Free allocated memory
return result; // Return jstring
}
In this example, memory is allocated for a native string, but it’s crucial to free it before returning the result. This helps prevent memory leaks.
2. Exception Handling
Problem
JNI can throw exceptions that may not be obvious if the native code does not handle or throw them properly. If an exception occurs and is not cleared, it can lead to inconsistent states.
Solution
Check for exceptions after calling JNI functions. Use methods like (*env)->ExceptionCheck
and (*env)->ExceptionClear
to manage exception statuses:
JNIEXPORT void JNICALL Java_MyClass_nativeMethod(JNIEnv *env, jobject obj) {
jclass cls = (*env)->FindClass(env, "SomeClass");
if (cls == NULL) {
// Clear the exception before throwing a new one
(*env)->ExceptionClear(env);
// Handle the error
}
}
By managing exceptions properly, you ensure that your JNI interface remains robust, leading to fewer runtime errors.
3. Type Mismatches
Problem
JNI requires meticulous type matching between Java and C/C++. A mismatch can lead to unexpected behavior and difficult-to-diagnose bugs.
Solution
Always ensure types match between Java definitions and JNI declarations. Using the appropriate JNI functions to convert types is essential. For instance, when working with arrays, always double-check the size and type:
JNIEXPORT void JNICALL Java_MyClass_processArray(JNIEnv *env, jobject obj, jintArray inputArray) {
jsize length = (*env)->GetArrayLength(env, inputArray);
jint *arrayElements = (*env)->GetIntArrayElements(env, inputArray, NULL);
// process the array
for (int i = 0; i < length; i++) {
// Manipulate elements
}
(*env)->ReleaseIntArrayElements(env, inputArray, arrayElements, 0); // Important to free resources
}
This snippet demonstrates how to safely handle an array passed from Java to C/C++.
4. Performance Overhead
Problem
JNI incurs some performance overhead due to the crossing of the Java-Native boundary. Frequent calls between Java and native code can slow down your application.
Solution
Minimize JNI calls by batching operations. If you frequently need to call native code, consider aggregating multiple calls into one:
// Java code
public native void bulkProcess(int[] data); // One JNI call instead of multiple
By calling bulkProcess
, you avoid the overhead associated with multiple JNI calls.
5. Compiling and Loading Issues
Problem
Compiling native code can be a hassle, especially when it comes to ensuring that the correct native library is available at runtime.
Solution
Use a consistent build system (like CMake, Gradle with the native plugin, etc.) and ensure the path to your native library is included in the Java Library Path:
java -Djava.library.path=/path/to/native/libs YourJavaClass
Key Takeaways
Mastering JNI is a valuable skill for Java developers, especially when performance is key or when working with existing native code. By being aware of common pitfalls and implementing appropriate strategies, you can leverage JNI effectively while minimizing errors.
For more comprehensive coverage of JNI, consider checking the official Java Documentation for best practices. Also, familiarize yourself with additional error handling techniques to further enhance the robustness of your JNI code.
By keeping in mind the above pitfalls and solutions, you are well on your way to becoming proficient in JNI and unlocking powerful capabilities for your Java applications. Happy coding!