Common Pitfalls in Java Internationalization and Localization
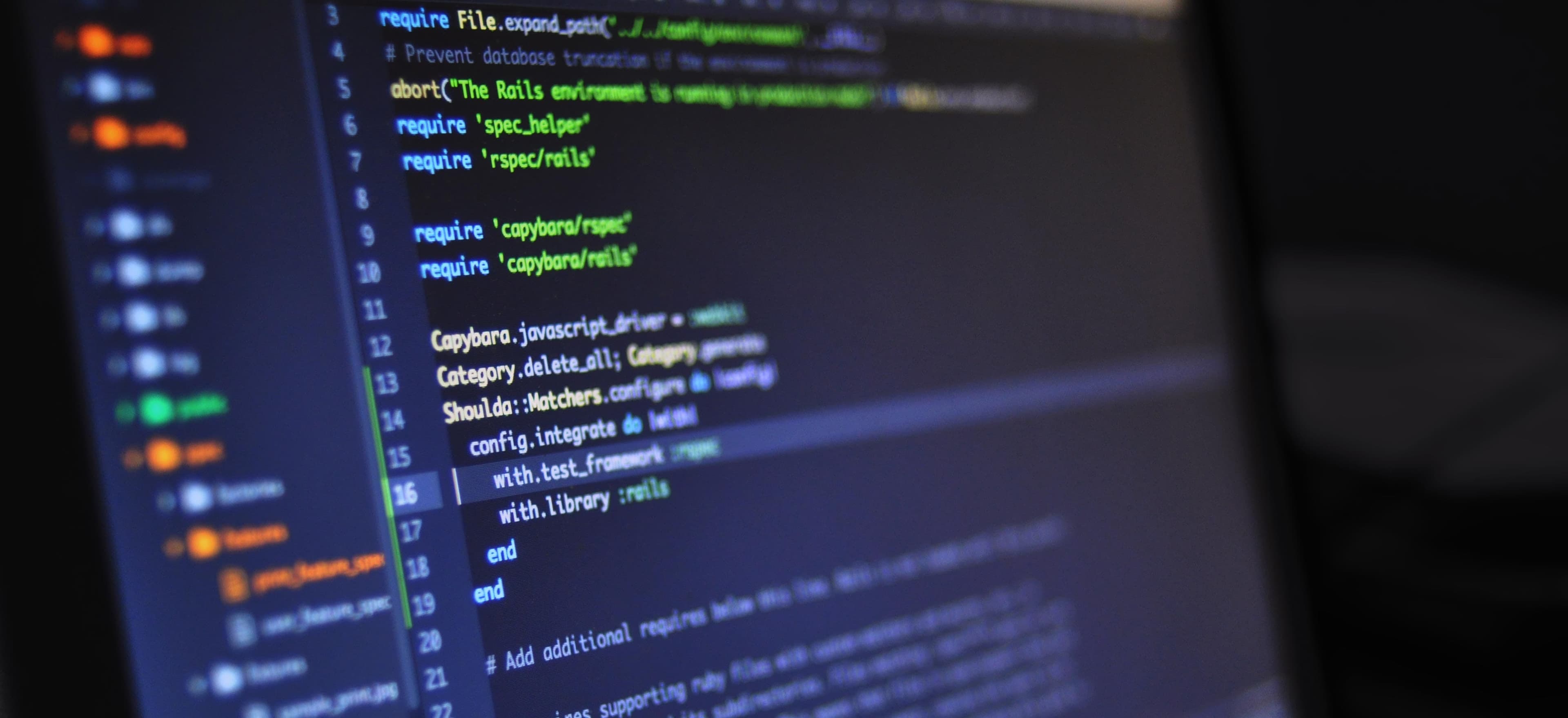
- Published on
Common Pitfalls in Java Internationalization and Localization
Internationalization (i18n) and localization (l10n) are vital practices in software development, especially for businesses aiming to reach global markets. Java, being a powerful, widely-used programming language, offers robust support for i18n and l10n. However, even seasoned developers can encounter pitfalls that hinder effective implementations. In this post, we will explore common mistakes and how to avoid them to create a seamless user experience across different languages and cultures.
Understanding Internationalization and Localization
Before diving into the pitfalls, let’s clarify the concepts:
-
Internationalization (i18n): This is the process of designing a software application to support various languages and cultural formats without needing engineering changes. It involves abstracting out local-specific elements, such as text, dates, currencies, and other locale-specific data.
-
Localization (l10n): This is the adaptation of your application for a specific region or language by translating text and adjusting formats and functionalities to meet local needs.
Common Pitfalls in Java Internationalization and Localization
1. Hard-Coding Strings
Pitfall: One of the most common mistakes is hard-coding text strings directly into the source code.
Why It's a Problem
Hard-coded strings make it difficult to translate applications into multiple languages, as updates require changes in the code itself.
Solution
Use Java’s ResourceBundle
to manage externalized strings. Here’s a simple example:
import java.util.Locale;
import java.util.ResourceBundle;
public class InternationalizationExample {
public static void main(String[] args) {
Locale locale = new Locale("fr", "FR");
ResourceBundle messages = ResourceBundle.getBundle("Messages", locale);
System.out.println(messages.getString("greeting"));
}
}
In this example, the ResourceBundle
retrieves localized strings based on the current locale. This allows you to maintain all translations separately, enhancing flexibility and maintainability.
2. Neglecting Locale-Specific Formats
Pitfall: Failing to consider locale-specific formats for dates, numbers, and currencies can lead to confusion for end-users.
Why It's a Problem
Different regions have varied conventions, which if ignored, could result in misunderstandings.
Solution
Utilize the java.text
package for formatting localized data. For instance:
import java.text.NumberFormat;
import java.util.Locale;
public class LocaleSpecificFormatting {
public static void main(String[] args) {
double amount = 12345.678;
// Format as USA currency
Locale usaLocale = Locale.US;
NumberFormat usCurrencyFormatter = NumberFormat.getCurrencyInstance(usaLocale);
System.out.println("US: " + usCurrencyFormatter.format(amount));
// Format as French currency
Locale franceLocale = Locale.FRANCE;
NumberFormat frCurrencyFormatter = NumberFormat.getCurrencyInstance(franceLocale);
System.out.println("FR: " + frCurrencyFormatter.format(amount));
}
}
By leveraging NumberFormat
, you can automatically adapt the presentation to fit user expectations across different regions.
3. Incomplete Message Handling
Pitfall: Omitting critical messages in resource bundles can lead to disjointed user experiences.
Why It's a Problem
If certain strings are not localized, users might encounter unexpected behaviors or errors that are confusing, especially if terms are left untranslated.
Solution
Conduct thorough reviews of resource files to ensure every user-facing message is included and translated. Implementing automated tests to verify string completeness can be highly beneficial.
4. Ignoring Pluralization and Variants
Pitfall: Failing to account for plural forms and linguistic variants can result in awkward or incorrect messaging.
Why It's a Problem
Different languages handle plurals in unique ways. For example, in English, “1 apple” versus “2 apples.” However, languages like Russian have more complex rules.
Solution
Java's MessageFormat
class can specify plural forms and variants appropriately, allowing you to create dynamically adjusted messages. Here’s an example:
import java.text.MessageFormat;
import java.util.ResourceBundle;
public class PluralizationExample {
public static void main(String[] args) {
ResourceBundle messages = ResourceBundle.getBundle("Messages");
int apples = 3; // This could be dynamic based on user input.
String message = MessageFormat.format(messages.getString("appleCount"), apples);
System.out.println(message);
}
}
In your resource bundles, you can define keys like:
appleCount=You have {0} apple(s).
This ensures that the output adapts based on how many apples the user has.
5. Failing to Test in Different Locales
Pitfall: Developers often test applications solely in their default or native locale.
Why It's a Problem
Neglecting to test in various locales can lead to bugs and display issues specific to certain languages.
Solution
Regularly test your application using different locale configurations. Automated tools can simulate users from various locales. Tools like Internationalization Checks in Java can help identify potential issues early in the development cycle.
6. Overlooking Cultural Sensitivities
Pitfall: Certain images, symbols, or colors may have disparate meanings across cultures, and ignorance of these differences can create offense.
Why It's a Problem
Using an element that feels normal in one culture might be considered inappropriate in another.
Solution
Conduct research into the cultures of your target audience and potentially engage local experts for guidance on what may or may not work. Additionally, consider allowing users to customize certain elements of their experience.
Bringing It All Together
Internationalization and localization can significantly enhance your application's reach and user experience. By avoiding the common pitfalls discussed, such as hard-coded strings, incomplete message translations, and overlooking cultural nuances, Java developers can create applications that speak to users in their language and cultural context.
Leveraging Java's rich libraries and packages like ResourceBundle
and MessageFormat
can streamline the i18n and l10n processes, making applications more adaptable and user-friendly.
For further reading on Java internationalization, check out Java Internationalization (i18n) Overview for comprehensive guidelines and best practices. Happy coding!
This blog post detailed the common pitfalls in Java internationalization and localization, offering clear solutions backed by code snippets while maintaining a user-focused approach.