Common JPA Mistakes That Developers Must Avoid
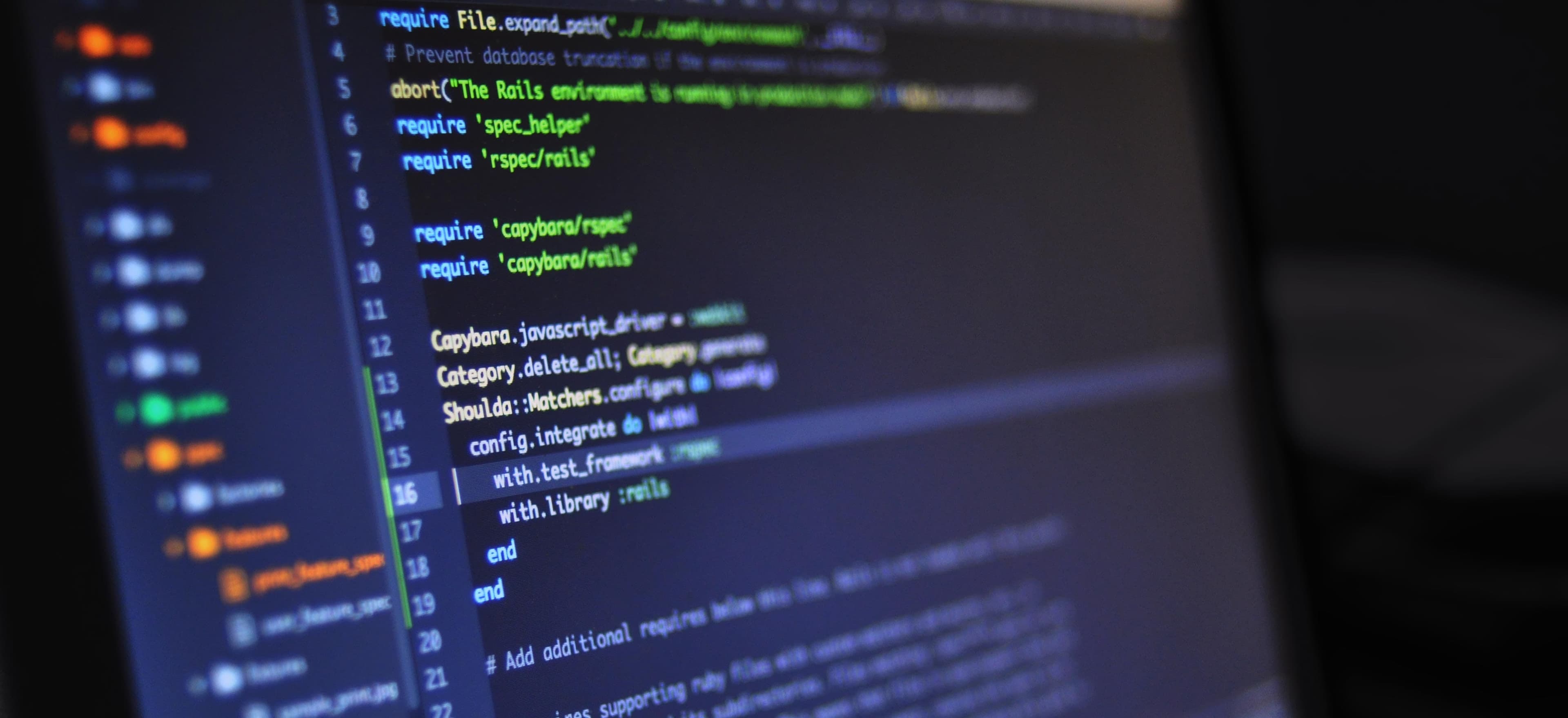
- Published on
Common JPA Mistakes That Developers Must Avoid
Java Persistence API (JPA) is an essential part of Java Enterprise Edition (Java EE) and is widely used for database operations in enterprise-level applications. While JPA simplifies the interaction with relational databases through an object-oriented approach, there are several common mistakes that developers often encounter. In this blog post, we will explore the pitfalls related to JPA and how to avoid them, ensuring that your applications remain efficient, maintainable, and bug-free.
Understanding JPA: A Quick Overview
Before diving into common mistakes, it's essential to grasp the purpose of JPA. JPA is a specification that allows developers to map Java objects to database tables. It provides an interface for performing CRUD (Create, Read, Update, Delete) operations without getting mired in SQL syntax. Through the use of Object-Relational Mapping (ORM), it allows an abstraction layer over databases.
The Anatomy of an Entity
To understand how mistakes can occur, let's take a look at a simple JPA entity:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
private String email;
// Getters and Setters
}
In this example, we define a User
entity with fields id, name, and email. By understanding this structure, we can now dive into the mistakes that developers often make.
Top Common JPA Mistakes
1. Not Using Transactions Properly
One of the most fundamental mistakes is neglecting to use transactions appropriately. JPA requires transactions to be encapsulated in a boundary. This ensures that either all operations succeed or none do.
Why It's Important: Failing to manage transactions can lead to inconsistent data states. For example:
EntityManager entityManager = entityManagerFactory.createEntityManager();
entityManager.getTransaction().begin();
User user = new User();
user.setName("John Doe");
entityManager.persist(user);
entityManager.getTransaction().commit();
2. Forgetting to Close EntityManager
When using JPA, managing resources is crucial. Developers often forget to close the EntityManager
. This can lead to memory leaks and exhaustion of database connections.
How to Avoid It: Always close the EntityManager
when it is no longer needed, ideally using a try-with-resources statement:
try (EntityManager entityManager = entityManagerFactory.createEntityManager()) {
entityManager.getTransaction().begin();
// Your operations
entityManager.getTransaction().commit();
} // Automatically closed
3. Misusing Fetch Types
JPA allows you to define the fetch strategy: FetchType.LAZY
or FetchType.EAGER
. The mistake often made is using FetchType.EAGER
by default, which loads related entities immediately and can lead to performance issues.
Example:
@Entity
public class Order {
@OneToMany(fetch = FetchType.LAZY)
private List<Item> items; // Favor lazy loading
}
Why: Fetching large amounts of data can slow down your application significantly. Lazy loading improves performance as it only fetches data when needed.
4. Ignoring Null Values
Another common oversight is neglecting to check for null values before performing operations. This can lead to unexpected null pointer exceptions.
How to Avoid It: Always validate input before using it:
public void addItem(Item item) {
if (item == null) {
throw new IllegalArgumentException("Item cannot be null");
}
// Proceed with adding item to order
}
5. Not Implementing Equals and HashCode Properly
When working with collections of entities, failing to correctly implement equals
and hashCode
can lead to unpredictable behavior, especially when using sets or maps.
Why It's Important: If two entities are considered equal but do not have the same hash code, you might experience issues while retrieving them from collections.
Example:
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof User)) return false;
User user = (User) o;
return id.equals(user.id);
}
@Override
public int hashCode() {
return Objects.hash(id);
}
6. Using Detached Entities
Using detached entities can lead to unexpected errors. When an entity is detached from the EntityManager
, it is no longer synchronized with the database. Working with detached entities requires care.
How to Avoid It: Always ensure that the EntityManager
is aware of the entity before performing operations on it:
User user = entityManager.find(User.class, userId);
user.setName("Updated Name");
entityManager.merge(user); // Reattach to EntityManager
7. Not Leveraging Named Queries and JPQL
Many developers tend to use native queries rather than taking advantage of JPA’s powerful JPQL (Java Persistence Query Language) and named queries.
Why Use JPQL: Named queries offer several benefits such as better readability, optimization, and easier maintenance.
Example:
@NamedQuery(name="User.findByName", query="SELECT u FROM User u WHERE u.name = :name")
List<User> findByName(@Param("name") String name);
8. Not Handling Concurrency Issues
In multi-threaded applications, concurrency can be problematic. Not anticipating concurrent updates can lead to data inconsistencies.
How to Avoid It: Employ optimistic and pessimistic locking strategies. Here's an example of optimistic locking using a version field:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
@Version
private Long version; // Version field for optimistic locking
}
The Last Word
Avoiding these common JPA mistakes is crucial for developing efficient and robust Java applications. Understanding the nuances of JPA can lead to more maintainable code and a better performance outlook for your applications.
Additional Resources:
- Java Persistence API Documentation
- JPA Best Practices
- Understanding JPA Fetch Types
By keeping these best practices in mind, you can elevate your JPA development skills and create applications that stand the test of time. Happy coding!