Common Pitfalls When Deploying Java Apps on WildFly
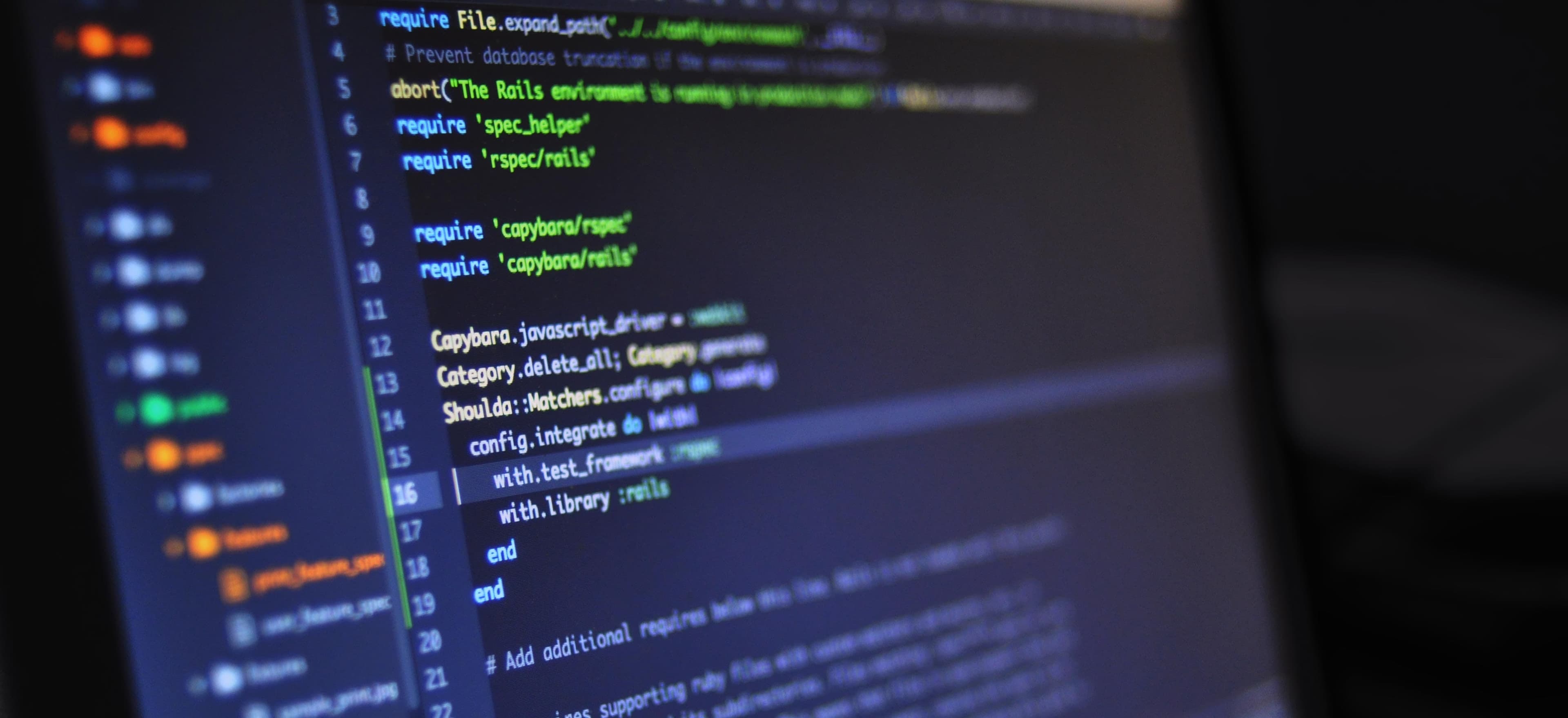
- Published on
Common Pitfalls When Deploying Java Apps on WildFly
Deploying Java applications on WildFly can be a rewarding endeavor, but it also comes with its unique set of challenges. In this blog post, we will explore some common pitfalls that developers encounter when deploying their Java applications on WildFly. We will provide practical advice and code snippets to help guide you through the process.
Diving Into the Subject to WildFly
WildFly is an open-source application server derived from JBoss AS. It is built to support enterprise applications, offering a robust set of features that include clustering, concurrency, and a management console, among others. Its flexibility and performance capabilities make it a popular choice among Java developers. However, certain mistakes can derail your deployment if you're not careful.
Pitfall #1: Misconfigured Data Sources
Data sources are crucial for any application that interacts with a database. In WildFly, this means configuring a datasource in the management console or standalone.xml
. A common pitfall is misconfiguring your connection settings, leading to runtime errors.
Example Configuration
Here’s a snippet showing how to configure a datasource in standalone.xml
:
<datasource jndi-name="java:/myDS" pool-name="MyDS_Pool">
<connection-url>jdbc:mysql://localhost:3306/mydb</connection-url>
<driver>mysql</driver>
<security>
<user-name>myUser</user-name>
<password>myPassword</password>
</security>
</datasource>
Commentary
- Connection URL: Make sure your connection URL is correct. The format must align with the driver specifications.
- Driver Configuration: Confirm that the MySQL driver (or any other driver's JAR) is placed in the
modules
directory and properly mapped in themodule.xml
file. - JNDI Name: The JNDI name used in the application must match the JNDI entry defined in your datasource.
Getting these settings right avoids common pitfalls related to database connectivity.
Pitfall #2: Inadequate Resource Management
WildFly supports various resource types, but failing to properly manage your resources can lead to memory leaks and application crashes. A common error is not releasing connections or resources after usage.
Code Snippet
try (Connection connection = dataSource.getConnection()) {
// Perform data operations
} catch (SQLException e) {
e.printStackTrace();
}
Commentary
Using the try-with-resources statement ensures that the Connection
is closed automatically when done. Failing to close resources explicitly can lead to resource exhaustion, a common issue in high-traffic applications.
Pitfall #3: Overlooking Application Configurations
WildFly allows for advanced configurations through various deployment descriptors like web.xml
, jboss-web.xml
, and others. However, neglecting to set these configurations properly can lead to unexpected behavior in your app.
Example:
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.example.MyServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
Commentary
- Load-on-Startup: The load-on-startup element indicates the order in which servlets are initialized. Set this value only when necessary.
- Role Mapping: Ensure that your security constraints are adequately defined in your descriptor to prevent unauthorized access to certain parts of your application.
Properly configuring these files is crucial for the smooth running of your app.
Pitfall #4: Incompatible Libraries
One common pitfall is deploying applications with incompatible library versions. WildFly operates with specific versions of Java EE APIs and third-party libraries. Using libraries that clash with those in WildFly can lead to ClassNotFoundException
or NoClassDefFoundError
.
Solution
To avoid this issue, ensure the libraries packaged in your WAR or EAR files do not shadow WildFly's libraries. Utilize the jboss-deployment-structure.xml
to exclude WildFly's modules:
<jboss-deployment-structure>
<deployment>
<exclusions>
<module name="javax.api"/>
</exclusions>
</deployment>
</jboss-deployment-structure>
Commentary
By excluding conflicting modules, you can prevent potential issues when components have overlapping dependencies. Always consult the WildFly documentation for compatibility matrix and prerequisites before deploying your apps.
Pitfall #5: Neglecting Logging and Monitoring
Logging issues often go overlooked. Without proper logging and monitoring configurations, diagnosing problems in production becomes a daunting task.
Example Logging Configuration
Configure logging in standalone.xml
:
<logger category="com.example">
<level name="DEBUG"/>
</logger>
Commentary
- Debug Level: Use appropriate logging levels (DEBUG, INFO, ERROR) according to your environment. DEBUG level logging can be excessive in production.
- Log Management: Employ an external logging framework like Log4j or SLF4J for more flexibility in managing logs.
Utilizing a robust logging strategy lays the groundwork for maintaining application health.
Pitfall #6: Ignoring Performance Tuning
WildFly comes with various configuration options that can enhance the performance of your application. Not tuning these settings can result in underperforming applications.
Key Areas to Tune:
- Thread Pools: Adjust the number of threads available for handling requests based on your application load.
- Cache Settings: If using JPA or Hibernate, configure cache strategies to reduce database load.
Example Configuration
In standalone.xml
, you can configure thread pools like so:
<subsystem xmlns="urn:jboss:domain:threads:2.3">
<thread-factory name="MyThreadFactory" group-name="my-group"/>
<bounded-queue-thread-pool name="MyExecutor" max-threads="20" keepalive-time="60"/>
</subsystem>
Commentary
Improperly configured thread pools can either lead to wasted resources or request timeouts. Always monitor your application to adjust these settings based on real usage patterns.
Final Thoughts
Deploying Java applications on WildFly can be straightforward, provided you avoid these common pitfalls. Pay attention to datasource configurations, resource management, application descriptors, library versions, logging, and performance tuning. By implementing best practices around these areas, you can ensure a smoother deployment experience.
For further reading, you might find the following resources valuable:
- WildFly User Guide
- JDBC Data Sources in WildFly
- Best Practices for Logging in Java Applications
By equipping yourself with the knowledge and tools available, you can enhance your experience deploying Java applications on WildFly, making your life significantly easier—one deployment at a time.