Common JDBC Pitfalls and How to Avoid Them
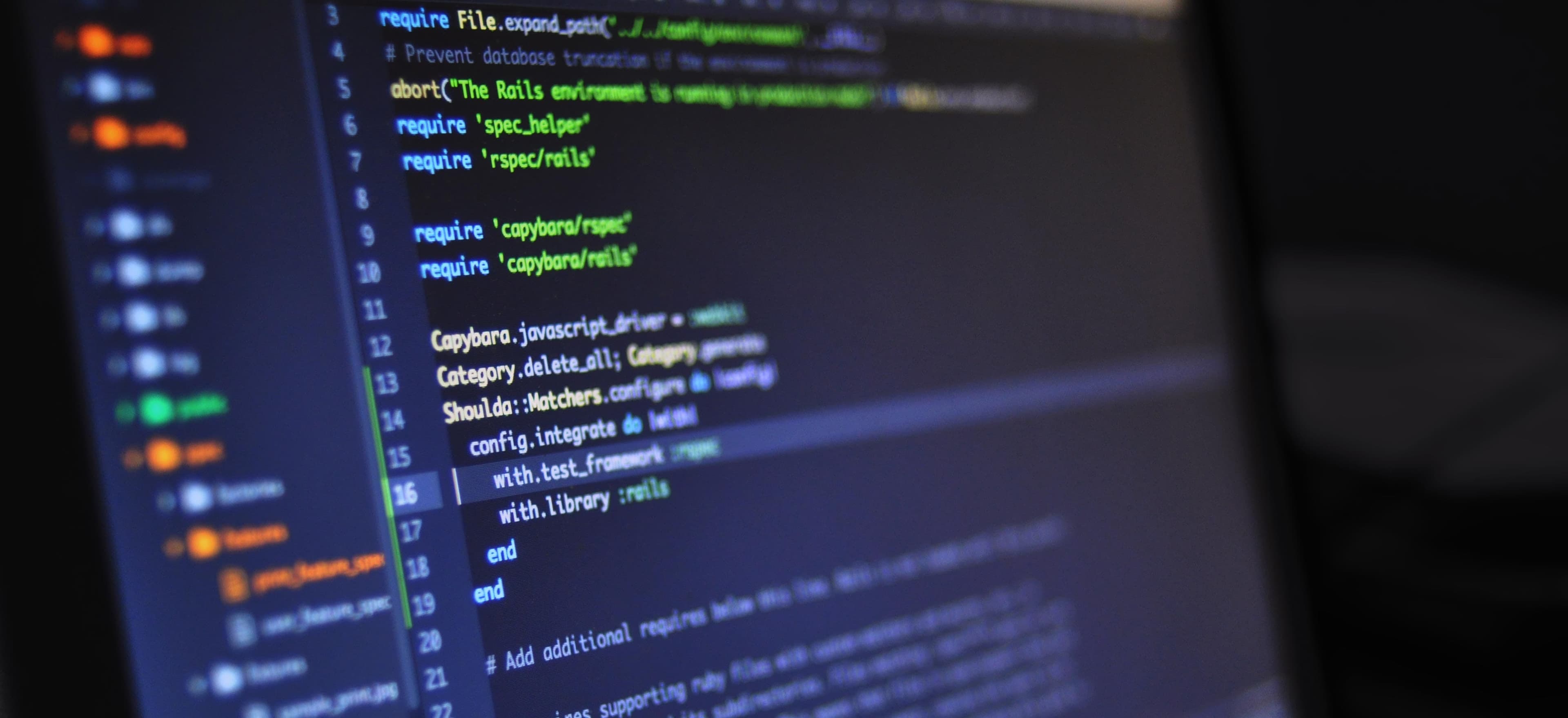
- Published on
Common JDBC Pitfalls and How to Avoid Them
Java Database Connectivity (JDBC) is a Java-based API that enables Java applications to interact with a database. Although JDBC simplifies the process of managing database connections, executing SQL commands, and processing results, there are common pitfalls that developers can encounter. This blog post will cover these pitfalls, explain why they occur, and provide practical solutions to avoid them.
The Java Database Connectivity Overview
Before diving into the common pitfalls, it's essential to understand what JDBC encompasses. JDBC provides the methods to connect to a database, send SQL statements, and retrieve results. It consists of two main packages:
java.sql
- This package contains classes and interfaces that provide standard APIs for database access.javax.sql
- This provides additional features such as connection pooling and distributed transactions.
For a more detailed overview of JDBC, visit Oracle JDBC Documentation.
Pitfall 1: Not Closing JDBC Resources
Description
One major pitfall occurs when developers forget to close JDBC resources such as Connection
, Statement
, and ResultSet
. Leaving these resources open leads to memory leaks and can exhaust the database connection pool.
How to Avoid It
Implementing a try-with-resources
statement is the best way to ensure resources are automatically closed when they are no longer needed.
Example Code
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class JdbcExample {
public void fetchRecords() {
String url = "jdbc:mysql://localhost:3306/mydb";
String username = "myuser";
String password = "mypass";
String query = "SELECT * FROM users";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement stmt = conn.prepareStatement(query);
ResultSet rs = stmt.executeQuery()) {
while (rs.next()) {
System.out.println("User ID: " + rs.getInt("id"));
System.out.println("User Name: " + rs.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In the example above, all resources are automatically closed when the try
block exits, whether normally or abruptly due to an exception. This prevents memory leaks and helps maintain application performance.
Pitfall 2: Using Plain JDBC for Complex Queries
Description
Many developers use plain JDBC for complex database operations, which can lead to verbose and hard-to-read code.
How to Avoid It
Utilize frameworks like JDBC Template or JPA (Java Persistence API) to handle complex queries more efficiently. This allows you to keep the code clean and maintainable.
Example Code with Spring JDBC Template
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
import java.util.List;
import java.util.Map;
public class UserDao {
private JdbcTemplate jdbcTemplate;
public UserDao() {
DriverManagerDataSource datasource = new DriverManagerDataSource();
datasource.setDriverClassName("com.mysql.cj.jdbc.Driver");
datasource.setUrl("jdbc:mysql://localhost:3306/mydb");
datasource.setUsername("myuser");
datasource.setPassword("mypass");
this.jdbcTemplate = new JdbcTemplate(datasource);
}
public List<Map<String, Object>> fetchAllUsers() {
String query = "SELECT * FROM users";
return jdbcTemplate.queryForList(query);
}
}
Commentary
By using JdbcTemplate
, the code becomes less prone to errors. It handles resource management and reduces boilerplate code, allowing developers to focus more on business logic.
Pitfall 3: Not Handling SQL Exceptions Properly
Description
Many developers catch SQLException
and log it without providing any meaningful context. This can make debugging frustrating and time-consuming.
How to Avoid It
Always provide a descriptive message or context along with the exception for better traceability.
Example Code
public void saveUser(User user) {
String query = "INSERT INTO users (id, name) VALUES (?, ?)";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement stmt = conn.prepareStatement(query)) {
stmt.setInt(1, user.getId());
stmt.setString(2, user.getName());
stmt.executeUpdate();
} catch (SQLException e) {
System.err.println("Unable to save user to the database. User ID: " + user.getId());
e.printStackTrace();
}
}
Commentary
By providing context, the error is easier to diagnose. Properly handling exceptions not only aids in debugging but also improves the reliability of your application.
Pitfall 4: Inefficient SQL Queries
Description
Another common pitfall is writing inefficient SQL queries that do not utilize indexes properly or retrieve more data than necessary.
How to Avoid It
Always use EXPLAIN statements to analyze query performance and optimize them as needed. Make effective use of indexes, and avoid SELECT *
when fetching data.
Example Code
public List<Map<String, Object>> fetchActiveUsers() {
String query = "SELECT id, name FROM users WHERE active = 1";
return jdbcTemplate.queryForList(query);
}
Commentary
In the above example, we fetch only relevant columns and enforce a condition. This minimizes the load on the database and improves performance.
Pitfall 5: Hardcoding Configuration Values
Description
Hardcoding database connection details, like username and password, directly in the source code can lead to security issues.
How to Avoid It
Externalize configuration values using a properties file or environment variables.
Example Code (Using properties
File)
# database.properties
db.url=jdbc:mysql://localhost:3306/mydb
db.username=myuser
db.password=mypass
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class Config {
private Properties props = new Properties();
public Config() {
try {
props.load(new FileInputStream("database.properties"));
} catch (IOException e) {
e.printStackTrace();
}
}
public String getDbUrl() {
return props.getProperty("db.url");
}
public String getDbUsername() {
return props.getProperty("db.username");
}
public String getDbPassword() {
return props.getProperty("db.password");
}
}
Commentary
By externalizing configuration, you make it easier to change values across different environments without altering the code. This practice increases security and maintainability.
The Closing Argument
JDBC is a powerful tool for database interaction in Java applications, but it comes with its challenges. By being aware of common pitfalls and implementing best practices, you can improve the reliability and performance of your applications.
Make sure to implement the strategies discussed in this blog post, such as properly closing resources, using frameworks for complex queries, adequately handling exceptions, optimizing SQL, and externalizing configuration values.
For further reading on best practices and performance tuning of JDBC, check out the Official Oracle JDBC Documentation and explore more on Java Best Practices.
By following these guidelines, you create clean, efficient, and manageable Java applications with reliable database interactions. Happy coding!