Common Pitfalls in REST Assured: Troubleshooting Tips
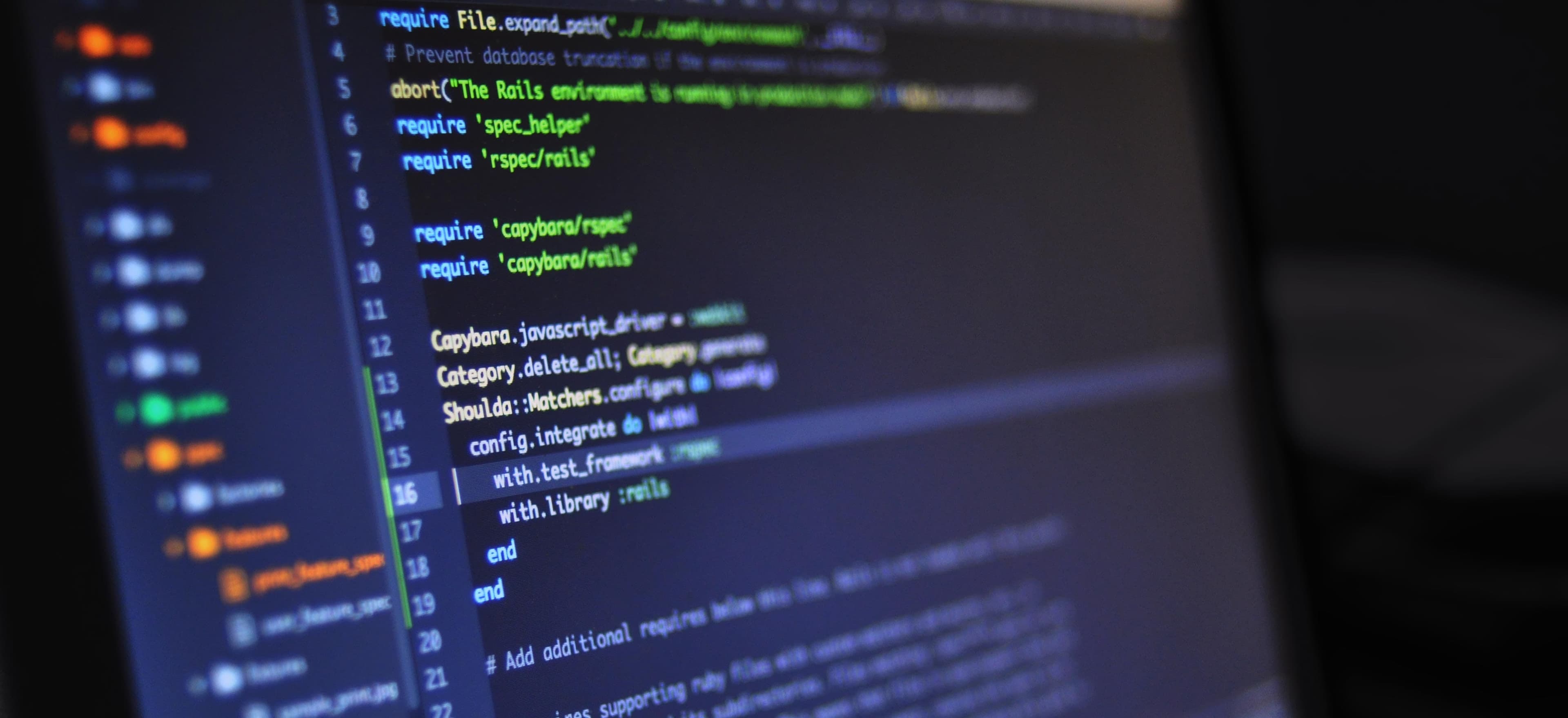
- Published on
Common Pitfalls in REST Assured: Troubleshooting Tips
Testing APIs is crucial, especially with the growing need for robust web services. REST Assured, a powerful Java library for testing RESTful APIs, significantly facilitates this process. However, like any other tool, it has its pitfalls. In this blog post, we will explore common issues encountered when using REST Assured and provide troubleshooting tips to help you overcome these challenges.
Understanding REST Assured
REST Assured is a domain-specific language (DSL) for writing tests for RESTful APIs. It enables developers to validate HTTP responses effectively using a fluent API, making tests readable and maintainable. Its integration with frameworks like JUnit and TestNG makes it even more versatile in the testing ecosystem.
Why Use REST Assured?
- Simplicity: It allows writing tests in a clear and concise manner.
- Rich Features: With built-in support for JSON/XML verification, response specification, authentication, etc.
- Seamless Integration: Works well with existing Java testing frameworks.
However, while its capabilities are vast, new users might find themselves caught in common pitfalls. Let's delve into some of these issues.
Common Pitfalls and Troubleshooting Tips
1. Incorrect Base URI
A common mistake is setting the base URI incorrectly. Ensure that you've defined the correct endpoint for your tests.
RestAssured.baseURI = "http://example.com/api";
Why? If the base URI is incorrect, your requests will fail, leading to confusion and wasted time.
2. Ignoring Response Status Codes
Many developers overlook the response status codes, assuming that receiving a response means success.
Response response = RestAssured.given()
.when()
.get("/endpoint")
.then()
.statusCode(200) // Ensure the status code is what you expect
.extract()
.response();
Why? Responses can return 200, but if the body is not as expected, your test could still fail. Always verify both the status code and the body content.
3. Not Validating Response Body
A successful API call does not guarantee accurate data. Make sure to validate your JSON/XML responses.
response.then().assertThat()
.body("name", equalTo("John Doe")); // Verifying JSON response
Why? A correctly defined response does not mean data correctness. Validating ensures your API behavior aligns with expectations.
4. Not Handling Headers Properly
Headers can often be a source of confusion, especially if not set or validated properly during requests.
given()
.header("Authorization", "Bearer token")
.when()
.get("/secure-endpoint")
.then()
.statusCode(200);
Why? APIs often require specific headers (like Authorization) for successful operations. Failing to include these can lead to 403 Forbidden or other errors.
5. Failure to Handle Different Content Types
REST Assured automatically determines content types based on the response. However, in some cases, applying explicit content-type settings resolves issues.
given()
.contentType("application/json") // Explicitly setting the content type
.when()
.post("/submit")
.then()
.statusCode(201);
Why? Not specifying content types, especially in POST or PUT requests, can lead to unexpected API behavior.
6. Using the Wrong HTTP Method
Be cautious about using incorrect HTTP methods (GET vs POST vs PUT) when making requests.
// For creating resources, use POST
given()
.body(newUser) // newUser being a JSON-like object
.when()
.post("/users")
.then()
.statusCode(201);
Why? Each HTTP method serves a different purpose, and incorrect usage can lead to unsuccessful operations or API errors.
7. Not Using Timeouts
Network calls can sometimes hang. If you don't set timeouts, your tests might run indefinitely.
RestAssured.given()
.config(RestAssured.config().httpClient(httpClientConfig()
.setParam("http.connection.timeout", 10000) // Set connection timeout to 10 seconds
.setParam("http.socket.timeout", 10000)))
.when()
.get("/endpoint");
Why? Setting timeouts helps prevent tests from hanging, ensuring faster feedback on your code.
8. Overlooking SSL Configuration
When dealing with HTTPS endpoints, users often neglect SSL configurations, leading to connection problems.
RestAssured.useRelaxedHTTPSValidation(); // Allow self-signed certificates
Why? If you're testing a development environment with self-signed certificates, you need this configuration to prevent connection failures.
9. Not Using Assertions Effectively
Assertions help validate the expected outcome of your tests. Avoid making vague assertions.
response.then().assertThat()
.contentType("application/json")
.body("status", equalTo("success"))
.body("data.id", notNullValue()); // Verify nested response fields
Why? Assertions help you pinpoint failures in your test results, providing actionable feedback.
Learning Resources
If you want to dive deeper into REST Assured, you can explore its official documentation and community forums for shared experiences and solutions to common problems. Additionally, check out Baeldung's REST Assured Tutorial for a comprehensive guide on using REST Assured effectively.
Bringing It All Together
While REST Assured makes API testing straightforward, it's essential to be aware of common pitfalls and learn how to troubleshoot them effectively. By following the tips outlined in this article, you'll be well on your way to robust and reliable API tests. Remember, the key to successful API testing is clarity and precision—both in your request formation and in your response validation.
By harnessing REST Assured with these insights, you can ensure a smoother testing process, allowing you to focus on building quality applications. Happy testing!