Common Java Socket Issues and How to Fix Them
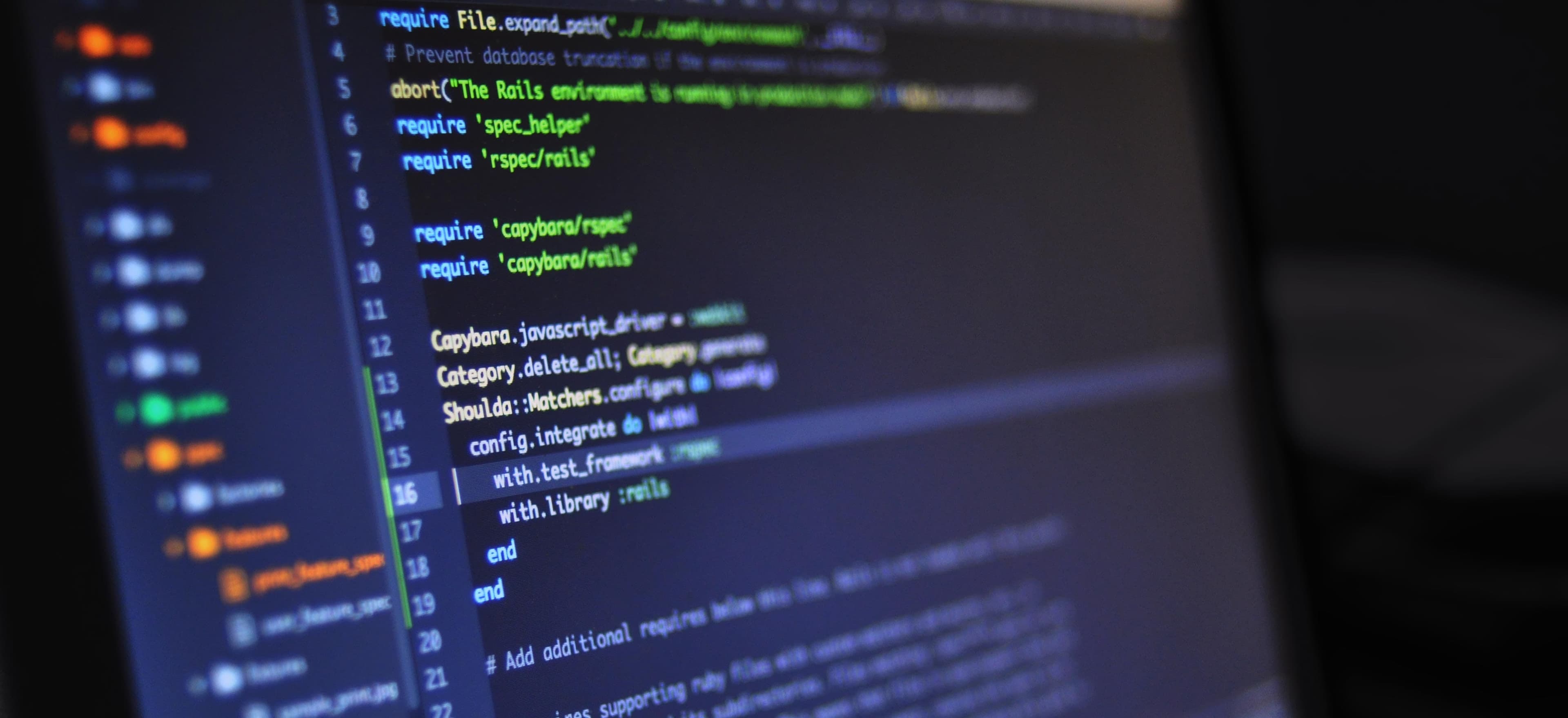
- Published on
Common Java Socket Issues and How to Fix Them
Java's socket programming is a powerful yet intricate aspect of software development. Whether you're developing a simple client-server application or a complex multi-threaded server, understanding socket issues can significantly impact performance and reliability. In this article, we will explore common Java socket problems and how to solve them, ensuring you can create robust network applications.
Understanding Java Sockets
Java sockets enable communication between two networked devices. They provide a means for data exchange using Transmission Control Protocol (TCP) or User Datagram Protocol (UDP). The Java API for sockets is straightforward but requires an understanding of the underlying principles, including handling connection timeouts, data integrity, and thread safety.
Common Socket Issues
1. Connection Timeout
One of the most prevalent issues developers face is connection timeout. This occurs when a client attempts to reach a server but does not receive a response within the expected timeframe.
Resolution:
You can address connection timeouts through careful socket configuration. By setting a timeout on the socket itself, you can ensure that your application does not hang indefinitely.
import java.io.IOException;
import java.net.Socket;
public class TimeoutExample {
public static void main(String[] args) {
try {
// Creating a Socket with a 5-second timeout
Socket socket = new Socket();
socket.connect(new InetSocketAddress("example.com", 80), 5000);
System.out.println("Connected successfully");
socket.close();
} catch (IOException e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
Here, the connect
method's timeout parameter is critical. It prevents your program from waiting too long if the server is down or unreachable.
2. Port Already in Use
Another common error is the "port already in use" exception. This occurs when a server attempts to bind to a port that is already occupied by another process.
Resolution:
Make sure to close sockets properly or use a different port. Check for lingering processes with the following command:
- Windows:
netstat -ano | findstr :<port>
- Linux/Mac:
lsof -i :<port>
To address this issue in Java:
import java.io.IOException;
import java.net.ServerSocket;
public class PortBindingExample {
public static void main(String[] args) {
int port = 8080;
try (ServerSocket serverSocket = new ServerSocket(port)) {
System.out.println("Server is listening on port " + port);
// Await client connections here
} catch (IOException e) {
System.err.println("Port is already in use: " + e.getMessage());
}
}
}
In this snippet, if the port is busy, an exception will be caught and properly logged.
3. Data Corruption and Integrity Issues
Data integrity is paramount in socket communication. Data corruption might happen when sending or receiving serialized objects across a network.
Resolution:
Utilize Java's built-in serialization and deserialization mechanisms carefully by ensuring that both client and server use the same class versions. Implement checksums for added integrity.
import java.io.*;
import java.net.Socket;
public class DataIntegrityExample {
public static void main(String[] args) {
try (Socket socket = new Socket("example.com", 1234);
ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream());
ObjectInputStream in = new ObjectInputStream(socket.getInputStream())) {
String message = "Hello from client!";
out.writeObject(message);
out.flush();
String response = (String) in.readObject();
System.out.println("Received: " + response);
} catch (IOException | ClassNotFoundException e) {
System.err.println("Error in sending or receiving data: " + e.getMessage());
}
}
}
In this example, we employ ObjectInputStream
and ObjectOutputStream
to send and receive serializable objects. Pay attention to matching versions between your client and server classes to ensure proper deserialization.
4. Blocking Calls and Threading Issues
Blocking calls can lead to performance bottlenecks, especially in multi-threaded applications where users expect responsive interactions. A thread can block while waiting for data, leading to sluggish application behavior.
Resolution:
Use non-blocking I/O (NIO) for scalable applications. By utilizing the java.nio.channels
package, you can avoid blocking calls by using selectors.
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
public class NonBlockingExample {
public static void main(String[] args) {
try {
Selector selector = Selector.open();
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.bind(new InetSocketAddress(8080));
serverSocketChannel.configureBlocking(false);
serverSocketChannel.register(selector, serverSocketChannel.validOps());
while (true) {
selector.select(); // Blocks until at least one channel is ready
var selectedKeys = selector.selectedKeys();
for (var key : selectedKeys) {
if (key.isAcceptable()) {
SocketChannel client = serverSocketChannel.accept();
client.configureBlocking(false);
System.out.println("Accepted connection from: " + client.getRemoteAddress());
}
}
selectedKeys.clear();
}
} catch (IOException e) {
System.err.println("Error in non-blocking server: " + e.getMessage());
}
}
}
This non-blocking server listens for incoming connections without holding up resources. The Selector
allows your application to respond to multiple channels efficiently, improving performance.
5. Firewall and Security Issues
Sockets can be affected by firewalls that block port access. This is particularly the case in production environments, where strict security policies are commonplace.
Resolution:
Make sure the port you are using isn’t blocked by the firewall on either the client or server side. You can check the firewall settings on Windows and adjust accordingly. On Linux, use iptables
to configure access.
Always test your application in an environment that mirrors your production settings, including firewall and security measures.
A Final Look
Java socket issues can be daunting, but understanding them provides a foundation for building efficient network applications. By managing connection timeouts, choosing the right ports, ensuring data integrity, employing non-blocking I/O, and taking security measures into account, you can develop robust systems.
For further insights into advanced socket programming, consider reading resources such as the Java Networking Tutorial, which provides an in-depth exploration of these fundamental concepts.
Implement these solutions in your project to enhance both performance and reliability. Happy coding!