Maven vs Gradle: Choosing the Best Build Tool for Your Project
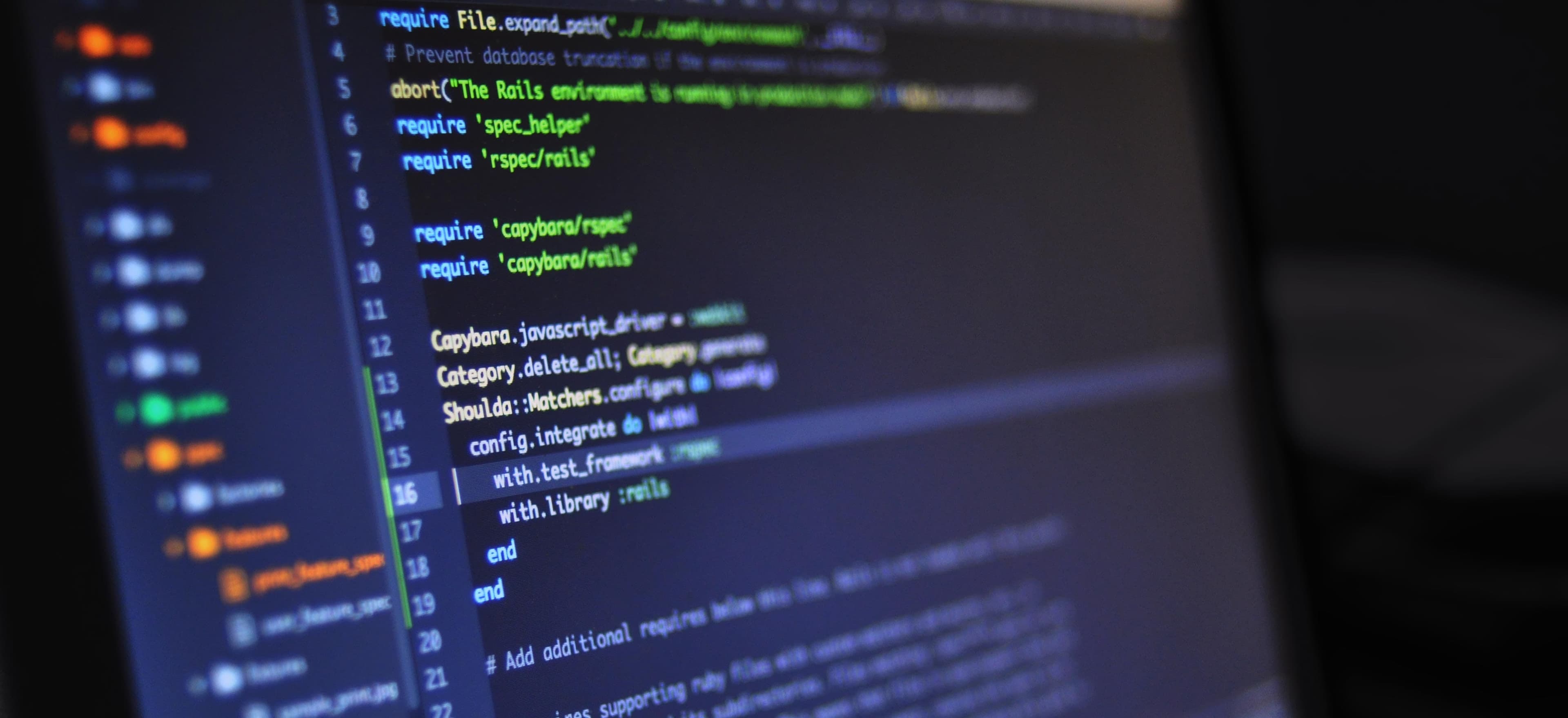
- Published on
Maven vs Gradle: Choosing the Best Build Tool for Your Project
When it comes to Java projects, build tools are essential in managing dependencies, compiling code, packaging applications, and more. Two of the most popular tools in the Java ecosystem are Maven and Gradle. This blog post will delve into each tool's features, advantages, and disadvantages, helping you make an informed decision on which one is best suited for your needs.
What is Maven?
Maven is a build automation tool used primarily for Java projects. It uses XML files for configuration and offers a comprehensive project management framework. Maven's primary benefit lies in its standardization; it follows a consistent directory structure and build lifecycle. This means almost any Java developer can jump into a Maven project and understand its structure.
Key Features of Maven
- Convention over Configuration: Maven simplifies project setup by enforcing a standard project directory structure.
- Dependency Management: Maven handles dependencies smoothly, automatically resolving and downloading them from the repository.
- Plugins: A rich ecosystem of plugins extends Maven's functionality.
Example of a Simple Maven Project
To illustrate how Maven works, here's a simple configuration for a Java project (pom.xml):
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
</dependencies>
</project>
Why Maven?
Maven’s clarity comes from its structured approach. Developers can easily understand a project by simply looking at the pom.xml
file. However, it's often criticized for verbosity and inflexibility, especially when custom configurations are needed.
What is Gradle?
Gradle is a modern build automation tool that combines concepts from both Maven and Ant. It uses Groovy or Kotlin DSL for its configurations, enabling both flexibility and ease of expression. Gradle is designed to manage multi-project builds, which is essential for larger applications.
Key Features of Gradle
- Dynamic Language Support: Gradle allows you to utilize its Groovy or Kotlin DSL, making configurations more readable and concise.
- Incremental Builds: Gradle can compile only the parts of the project that have changed, resulting in faster builds.
- Dependency Management: Similar to Maven, Gradle also simplifies managing dependencies but with more advanced features.
Example of a Simple Gradle Project
Here’s a simple build.gradle
file for a Gradle project:
plugins {
id 'java'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.slf4j:slf4j-api:1.7.30'
}
Why Gradle?
The flexibility of Gradle's DSL allows for custom logic in your build scripts. If your project requires complex customizations, Gradle is likely the better choice. However, this flexibility can also introduce complexity, especially for new developers.
Maven vs Gradle: A Feature Comparison
1. Ease of Use
- Maven: Its structured XML files are easy to understand but can become unwieldy for more complex configurations. However, its adherence to conventions means less room for error.
- Gradle: Offers a more expressive syntax through Groovy/Kotlin, which might reduce boilerplate code but requires familiarity with its syntax and conventions.
2. Performance
- Maven: Every build compiles all files, which can be slower for large projects.
- Gradle: Utilizes incremental builds and caching, allowing it to significantly reduce build times.
3. Build Speed
- Maven: Configuration is straightforward, but dependency resolution can slow down builds.
- Gradle: Optimized for performance with features such as the Gradle Daemon and build caching.
4. Community and Ecosystem
- Maven: Has a mature and stable ecosystem with a wide range of plugins.
- Gradle: Growing community and libraries. While not as extensive as Maven’s, it continues to evolve.
In Conclusion, Here is What Matters: Which Tool Should You Choose?
-
Choose Maven if your project is small to medium-sized and you're working within a team that values standardization and simplicity. Its robust dependency management and convention over configuration model can significantly speed up onboarding for new team members.
-
Choose Gradle if you're undertaking a larger project or require more customizations. Gradle’s flexibility and performance make it suitable for complex systems where rapid build times are a requirement.
Ultimately, both Maven and Gradle have their unique strengths and weaknesses, and the best choice ultimately depends on your project requirements.
Further Reading
For more insights into reproducible builds and the role of build tools, check out Jenkins Documentation and Gradle User Manual.
Choosing between Maven and Gradle doesn't have to be daunting. Understanding your project’s needs will guide you to the right build tool, allowing you to focus on what really matters — delivering high-quality software efficiently.