Mastering Functional Programming: Common Pitfalls in Java
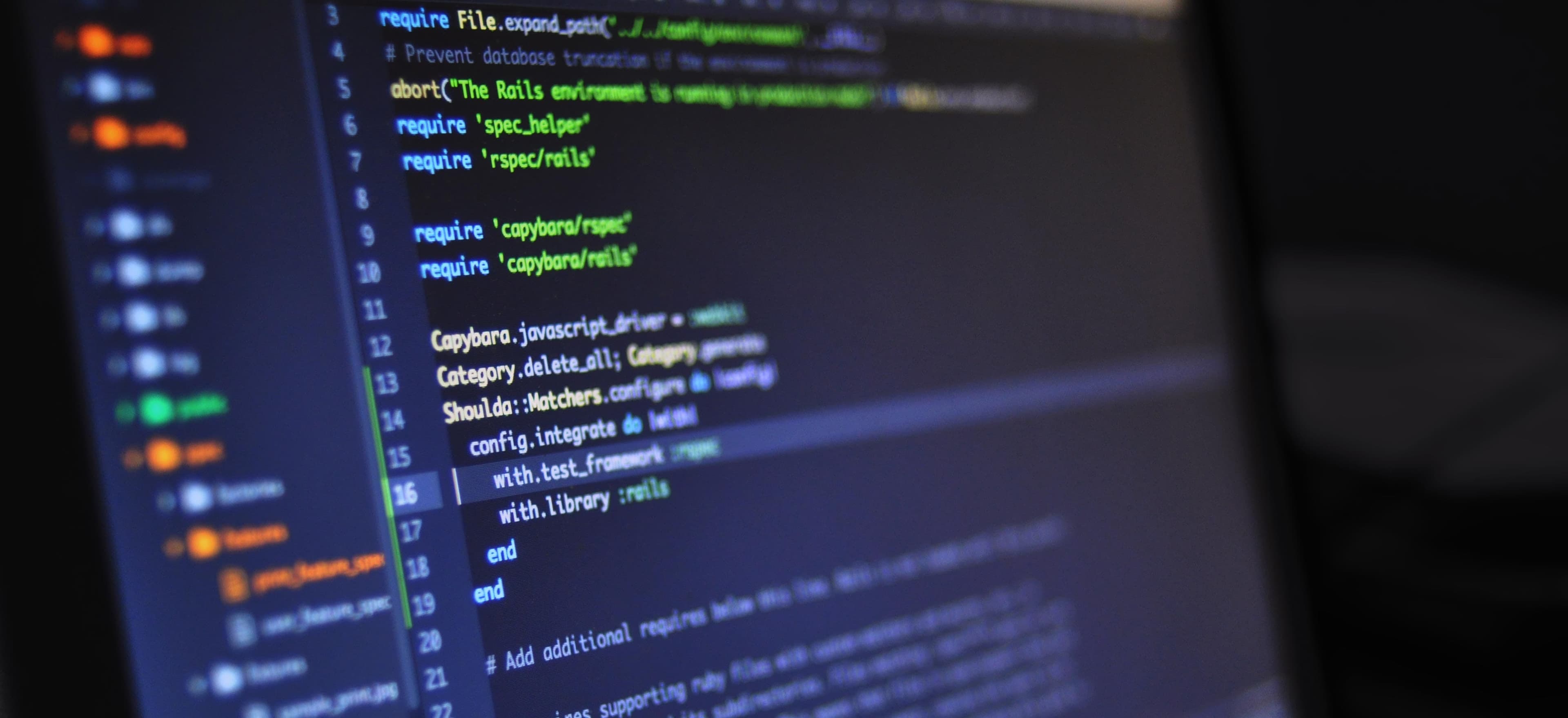
- Published on
Mastering Functional Programming: Common Pitfalls in Java
With the advent of Java 8, functional programming has become a significant aspect of Java development. This paradigm allows developers to create more predictable and maintainable code, tapping into the power of higher-order functions, lambdas, and streams. However, functional programming in Java isn't without its challenges. In this blog post, we'll explore some common pitfalls developers encounter and discuss how to avoid them, ensuring that you can master functional programming in Java.
Understanding Functional Programming in Java
Before diving into common pitfalls, it’s essential to understand what functional programming entails. Functional programming is a paradigm that treats computation as the evaluation of mathematical functions, avoiding state and mutable data. In Java, this is largely implemented through:
-
Lambda Expressions: Allow you to express instances of single-method interfaces (functional interfaces) in a clear and concise manner.
-
Streams API: A powerful abstraction for manipulating sequences of data, enabling operations such as filtering, mapping, and reducing.
Let's consider a basic example that showcases these features:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FunctionalExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Using Streams and Lambdas to filter names longer than 3 characters
List<String> filteredNames = names.stream()
.filter(name -> name.length() > 3)
.collect(Collectors.toList());
System.out.println(filteredNames);
}
}
In this snippet, we create a list of names and filter them using a stream, returning only those longer than three characters. The lambda expression within the filter
method succinctly captures our intention.
However, while functional programming offers many benefits, it also has some common pitfalls. Let's explore these challenges in detail.
Pitfall 1: Overusing Streams and Lambdas
While writing concise functional-style code can be satisfying, overusing streams and lambdas can lead to code that is difficult to read and maintain.
Why It Happens
Developers sometimes overlook the complexity introduced by chaining multiple streams or using several nested lambdas. When used excessively, the clarity of the code diminishes, making it a chore for others (or even yourself) to read later on.
Avoiding the Pitfall
-
Limit Chaining: Each additional stream operation adds complexity. Where possible, consolidate operations.
-
Use Method References: Whenever applicable, prefer method references over lambdas for cleaner code.
Here’s an improved version of the previous example using method references:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FunctionalExampleRef {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> filteredNames = names.stream()
.filter(name -> isLongerThanThree(name))
.collect(Collectors.toList());
System.out.println(filteredNames);
}
private static boolean isLongerThanThree(String name) {
return name.length() > 3;
}
}
Pitfall 2: State and Side Effects
Functional programming typically espouses immutability and statelessness. However, developers often unintentionally introduce state and side effects when using lambdas and streams.
Why It Happens
When lambdas or streams act upon external state or modify shared data, they undermine the functional programming principles of predictability and reliability.
Avoiding the Pitfall
-
Use final Variables: Declare any variables used within lambdas as final to enforce immutability.
-
Avoid External State: Ensure that lambdas do not modify any external variables. Consider returning new instances of objects instead.
Here's an example that risks introducing side effects:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class RiskyLambda {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> collectedNames = new ArrayList<>();
names.forEach(name -> {
if (name.length() > 3) {
collectedNames.add(name); // Side effect!
}
});
System.out.println(collectedNames);
}
}
By avoiding side effects, you can refactor this into a functional style:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class SafeLambda {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> collectedNames = names.stream()
.filter(name -> name.length() > 3)
.collect(Collectors.toList());
System.out.println(collectedNames);
}
}
Pitfall 3: Performance Issues
While the Streams API simplifies data processing, it can lead to performance issues, particularly if not used wisely. Naive use of streams may introduce unnecessary overhead.
Why It Happens
Streams are lazy by nature, which means they defer computation. However, this can result in performance hits if the stream pipe is not optimized.
Avoiding the Pitfall
-
Avoid Creating Streams from Non-Collection Data: Using streams on arrays or collections can lead to unnecessary boxing and unboxing.
-
Use Parallel Streams Wisely: Although parallel streams can speed up operations over large data sets, they introduce thread-safety concerns and overhead associated with context switching.
Here’s an example of efficiently processing a list:
import java.util.Arrays;
import java.util.List;
public class EfficientStream {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using parallel stream to benefit from multi-core processing
int sum = numbers.parallelStream()
.mapToInt(Integer::intValue) // Ensures fewer boxing/unboxing operations!
.sum();
System.out.println("Sum: " + sum);
}
}
Pitfall 4: Not Understanding Optional
The Optional
class in Java is designed to avoid null checks and represent optional values. However, improper use can lead to more confusion and mistakes than it solves.
Why It Happens
Developers might use Optional
in cases where it’s unnecessary or may fail to use its methods correctly.
Avoiding the Pitfall
-
Use Optional as a Return Type: Rather than returning null, use
Optional
to signify the possibility of absence. -
Don’t Overuse or Nest Optionals: Keep things simple. If you find yourself nesting Optionals, consider refactoring your code.
Here’s a simple example of using Optional
correctly:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
String value = null;
// Using Optional to handle a potentially null value
Optional<String> optionalValue = Optional.ofNullable(value);
System.out.println("Value is present: " + optionalValue.isPresent());
}
}
The Bottom Line
Functional programming brings a refreshing approach to Java programming, promoting cleaner and more maintainable code. However, it also introduces its own set of pitfalls. By understanding these common challenges—overusing streams and lambdas, introducing state and side effects, performance concerns, and improper handling of Optionals—you can develop more effective functional-style Java applications.
For further reading on functional programming in Java, I recommend checking out the official Java documentation and exploring Functional Programming in Java by Venkat Subramaniam.
Incorporate these insights to elevate your Java coding, ensuring your journey into functional programming is both enjoyable and productive. Happy coding!
Checkout our other articles