Common Pitfalls When Working with Jackson in Java
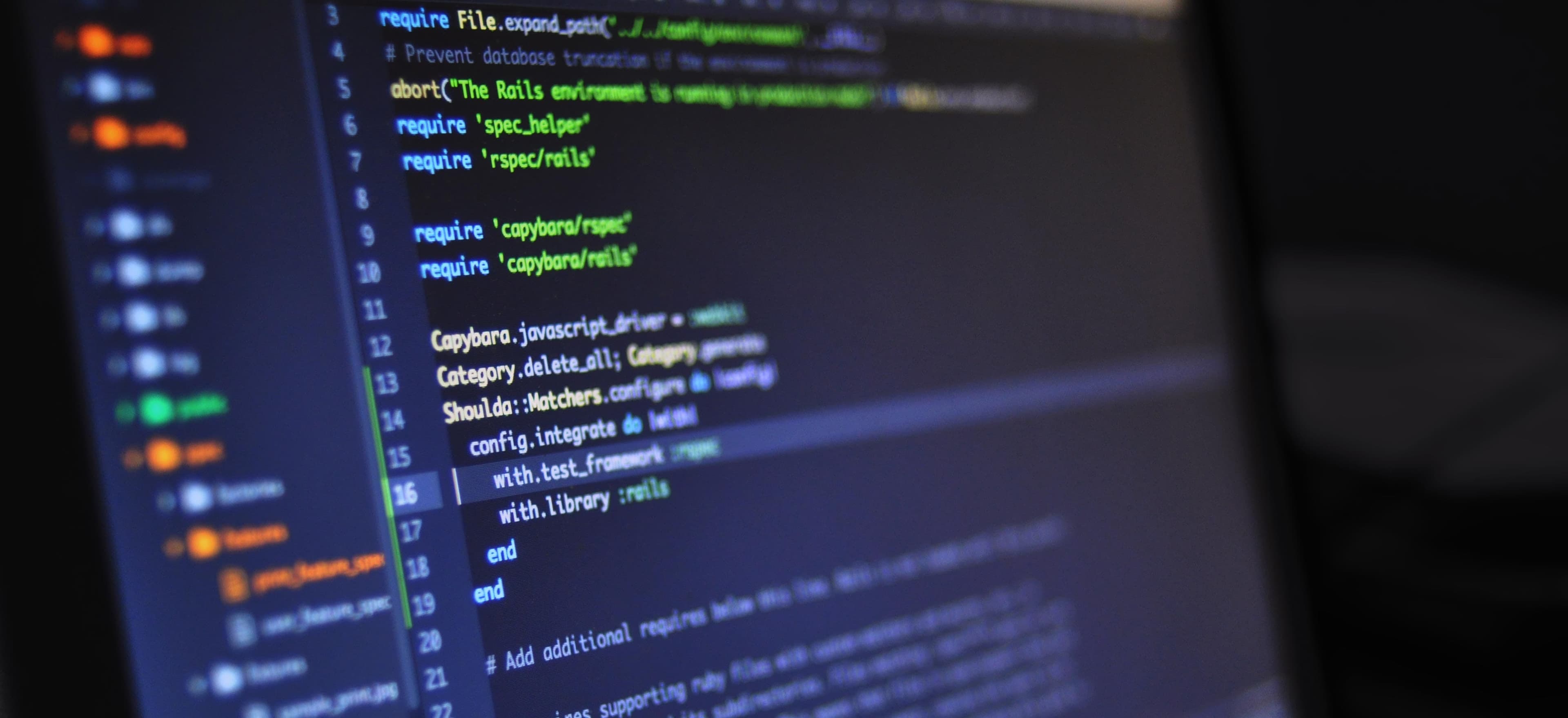
- Published on
Common Pitfalls When Working with Jackson in Java
Jackson is one of the most popular libraries for parsing JSON in Java. It is widely used in various applications, from web services to mobile apps, and is famous for its performance and versatility. However, like any powerful tool, it can come with its share of pitfalls that developers must navigate. In this blog post, we will explore some common issues developers face when working with Jackson and how to avoid them.
Table of Contents
- Understanding JSON Serialization and Deserialization
- Common Pitfall #1: Mismatched Property Names
- Common Pitfall #2: Handling Null Values
- Common Pitfall #3: Using Unrecognized Fields
- Common Pitfall #4: Circular References
- Common Pitfall #5: Date Handling
- Conclusion
Understanding JSON Serialization and Deserialization
Before diving into the pitfalls, it's essential to understand what serialization and deserialization mean in the context of Jackson.
- Serialization is converting a Java object into JSON.
- Deserialization is converting JSON back into a Java object.
Jackson uses annotations and conventions to map Java objects to JSON and vice versa. With this understanding, let’s explore some common pitfalls.
Common Pitfall #1: Mismatched Property Names
Issue
One of the most frequent issues developers encounter is mismatched property names. If the JSON keys do not align with the Java object fields, the deserialization will fail or produce incorrect values.
Solution
You can control the mapping using annotations like @JsonProperty
. The example below shows how to do this effectively:
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("username")
private String name;
@JsonProperty("user_email")
private String email;
// Getters and Setters
}
In this example, the JSON keys "username"
and "user_email"
are mapped to the fields name
and email
in the Java object. This ensures that no matter the naming convention in the JSON, it will be properly deserialized.
Why This Matters
Consistent naming conventions are crucial for code maintainability. Using annotations like @JsonProperty
gives you flexibility while avoiding confusion about what data is being represented.
Common Pitfall #2: Handling Null Values
Issue
Handling null values in JSON can lead to unexpected results in Java. Jackson has default behavior that may not suit your application's requirements—particularly when it comes to primitive types.
Solution
To manage nulls effectively, consider using wrappers like Integer
instead of int
, or simply configure the ObjectMapper for null handling.
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(SerializationFeature.WRITE_NULL_MAP_VALUES, false);
Using this configuration will prevent null values from being serialized, which keeps your JSON clean and manageable.
Why This Matters
Avoiding null values in your JSON can lead to less error-prone code and give you a clearer understanding of your application state.
Common Pitfall #3: Using Unrecognized Fields
Issue
You may encounter instances where the incoming JSON contains fields not defined in your Java class. By default, Jackson will throw an exception if it sees unrecognized fields.
Solution
To ignore these unrecognized fields, you can use:
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
This configuration allows Jackson to skip unknown fields gracefully.
Why This Matters
Ignoring unknown fields can be very beneficial when dealing with evolving APIs. It allows for backward compatibility while ensuring the existing parts of your application continue to function smoothly.
Common Pitfall #4: Circular References
Issue
Circular references occur when two objects reference each other. This can cause stack overflow errors during serialization or deserialization.
Solution
To handle circular references, use the @JsonManagedReference
and @JsonBackReference
annotations. Here’s a simplified example:
import com.fasterxml.jackson.annotation.JsonBackReference;
import com.fasterxml.jackson.annotation.JsonManagedReference;
public class User {
@JsonManagedReference
private Profile profile;
// Other properties, Getters and Setters
}
public class Profile {
@JsonBackReference
private User user;
// Other properties, Getters and Setters
}
In this example, the User
class maintains a managed reference to Profile
, allowing Jackson to serialize and deserialize them without running into circular reference issues.
Why This Matters
Circular references can lead to inefficiencies and bugs; addressing them ensures that your serialization logic remains robust.
Common Pitfall #5: Date Handling
Issue
Date and time handling in JSON and Java can be confusing. By default, Jackson can struggle with different date formats, leading to deserialization errors.
Solution
You can specify a date format using the @JsonFormat
annotation:
import com.fasterxml.jackson.annotation.JsonFormat;
public class Event {
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd HH:mm:ss")
private LocalDateTime eventDate;
// Getters and Setters
}
In this instance, the date string in the JSON must match the specified pattern to successfully deserialize into the LocalDateTime
object.
Why This Matters
Date formats may differ across various APIs, so being explicit about your expected formats will result in fewer errors and easier debugging.
Bringing It All Together
Jackson is a robust and efficient library for JSON processing in Java, but it does come with pitfalls that can disrupt your development flow. By being aware of common issues like mismatched property names, null handling, unrecognized fields, circular references, and date handling, you can avoid unnecessary headaches.
For more on Jackson and advanced use cases, consider checking out the Jackson Documentation or Baeldung's Guide on Jackson. Both resources will enrich your understanding and help you leverage Jackson more effectively in your Java applications.
Embrace these best practices, and navigate the world of JSON with confidence. Happy coding!
Checkout our other articles