Common JAXB Pitfalls in XML Processing and How to Fix Them
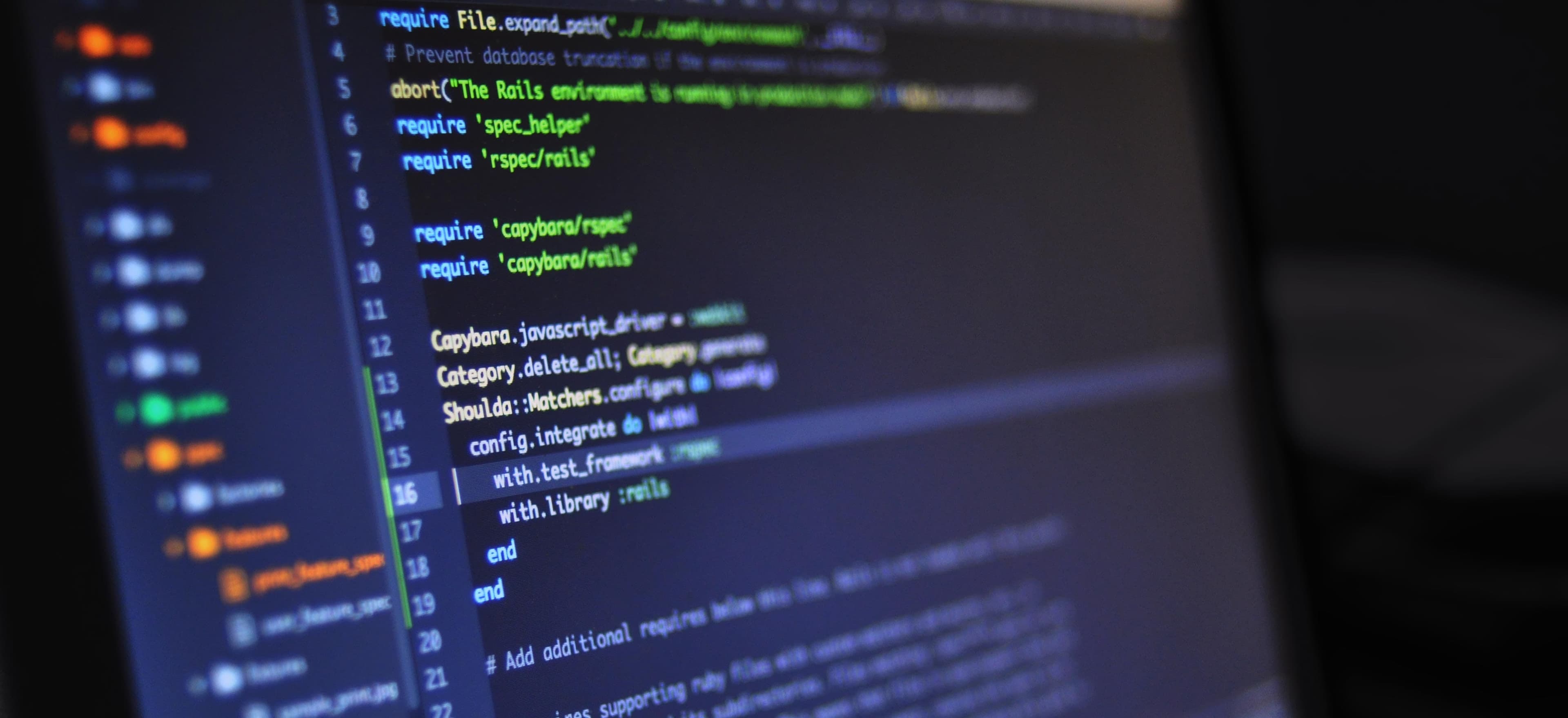
- Published on
Common JAXB Pitfalls in XML Processing and How to Fix Them
Java Architecture for XML Binding (JAXB) is a powerful framework that simplifies the process of reading and writing XML data in Java. However, while JAXB streamlines many aspects of XML processing, it is not without its pitfalls. This guide will explore some common issues developers face when using JAXB, providing solutions to ensure smoother XML data handling.
Table of Contents
- Understanding JAXB
- Common Pitfalls
- Incomplete Annotations
- Handling Namespaces
- Issues with Collections
- Unrecognized XML Types
- Validation Errors
- Solutions
- Conclusion
Understanding JAXB
JAXB allows Java developers to convert Java objects into their XML representation and vice versa. One of the most significant advantages of JAXB is that it abstracts XML parsing, serialization, and deserialization. This not only reduces boilerplate code but also simplifies the interaction between Java applications and XML data.
To start, you typically define your Java classes and annotate them with JAXB annotations such as @XmlRootElement
, @XmlElement
, and @XmlAttribute
. You can then generate XML from these classes using a Marshaller
and convert XML back to Java objects using an Unmarshaller
.
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
// Example class to demonstrate JAXB
@XmlRootElement
public class Person {
@XmlElement
private String name;
@XmlElement
private int age;
// Default constructor is necessary
public Person() {
}
// Constructor, getters, setters...
}
// Marshalling example
JAXBContext context = JAXBContext.newInstance(Person.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
// Converting Java object to XML
Person person = new Person();
person.setName("John Doe");
person.setAge(30);
marshaller.marshal(person, System.out);
This code snippet shows how straightforward it is to convert a Java object into XML format using JAXB. However, pitfalls can arise at various stages.
Common Pitfalls
Incomplete Annotations
One of the most common errors arises from incomplete or incorrect use of JAXB annotations. Each class is required to have a designated root element.
The Issue
If a class that needs to be converted to XML lacks proper annotations, you'll encounter javax.xml.bind.UnmarshalException
.
Example of the problem:
// Missing @XmlRootElement annotation
public class Product {
@XmlElement
private String id;
@XmlElement
private String name;
// Getters and setters...
}
Handling Namespaces
When your XML documents use namespaces, failure to correctly handle them can lead to issues.
The Issue
JAXB may fail to find elements if the expected namespace does not match, leading to unrecognized elements during unmarshalling.
Solution
Use the @XmlType
annotation and provide the namespace as follows:
@XmlRootElement(name = "Product")
@XmlType(namespace = "http://www.example.com/products")
public class Product {
@XmlElement(name = "Id")
private String id;
@XmlElement(name = "Name")
private String name;
// Getters and setters...
}
Issues with Collections
When dealing with lists or collections, many developers experience issues.
The Issue
JAXB may struggle with collections, throwing javax.xml.bind.UnmarshalException
if they aren't correctly annotated.
Example of the problem:
@XmlRootElement
public class Order {
private List<Product> products; // JAXB doesn't know how to handle this
// Getters and setters...
}
Solution
Use @XmlElement
on the collection:
@XmlRootElement
public class Order {
@XmlElement(name = "Product")
private List<Product> products = new ArrayList<>();
// Getters and setters...
}
Unrecognized XML Types
Sometimes, JAXB may encounter an XML type it cannot recognize, leading to an UnmarshalException
.
The Issue
This often happens when the XML provided does not match the structure of the expected Java class.
Solution
Ensure your class structure reflects the XML structure. Using @XmlSeeAlso
can help JAXB understand subclasses.
@XmlSeeAlso({DiscountedProduct.class})
@XmlRootElement
public class Product {
// Fields, getters, setters...
}
Validation Errors
Validation errors can occur if XML does not conform to the expected schema.
The Issue
Failure to validate can lead to runtime exceptions and data integrity issues.
Solution
Utilize the Schema
class in JAXB to validate XML against an XSD schema:
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = schemaFactory.newSchema(new File("schema.xsd"));
Unmarshaller unmarshaller = context.createUnmarshaller();
unmarshaller.setSchema(schema);
Solutions
Here are several strategies to mitigate these pitfalls.
- Check Annotations: Always review your Java classes for proper JAXB annotations.
- Namespace Management: Pay careful attention to namespaces when defining your XML structure.
- Collection Management: Properly annotate collections to facilitate seamless marshaling/unmarshaling.
- XML Structure Consistency: Ensure your Java classes align with the XML structure you are using.
- Validation: Implement schema validation when working with XML data to catch errors early.
Final Thoughts
While JAXB simplifies XML processing in Java applications, it presents specific challenges that developers must navigate. By being proactive about annotation completeness, namespace handling, collection management, type recognition, and validation, you can minimize common pitfalls.
For further reading on JAXB and XML processing, consider the following resources:
- Oracle's JAXB Documentation
- XML in Java: An Overview
- Java - JAXB in Action
By adopting best practices and recognizing potential pitfalls, you can effectively leverage JAXB to enhance the performance and reliability of your XML processing tasks.