Mastering Reactive Programming: Common Pitfalls in Project Reactor
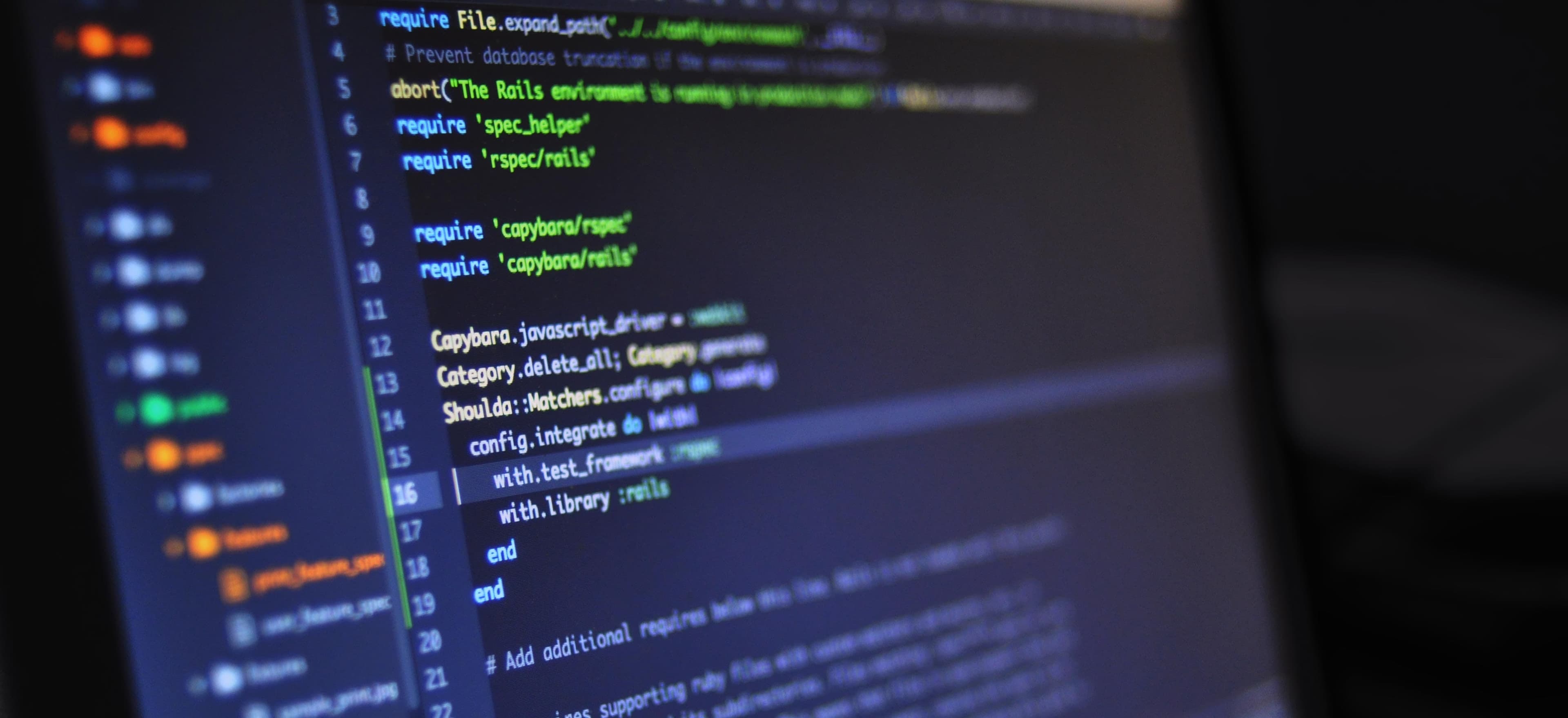
- Published on
Mastering Reactive Programming: Common Pitfalls in Project Reactor
Reactive programming has gained significant traction in modern application development, especially with the emergence of frameworks like Project Reactor. This paradigm shift allows developers to write asynchronous, non-blocking code that can handle a plethora of events efficiently. However, as with any powerful tool, there are pitfalls that can trip up even the most seasoned developers. In this post, we will explore common pitfalls in Project Reactor and how to overcome them.
Table of Contents
Understanding Reactive Programming
Before delving into the pitfalls, it's essential to grasp the fundamentals of Reactive Programming (RP). RP is centered around building systems that are resilient, responsive, and elastic. At its core, it relies on the Observer pattern, allowing one component to subscribe to events from another component.
Project Reactor provides two primary reactive types:
Mono
: Represents a single value or no value.Flux
: Represents a sequence of 0 to N values.
Both types are equipped with a rich API that makes processing streams easier. For instance, here’s a simple example:
import reactor.core.publisher.Flux;
public class SimpleFluxExample {
public static void main(String[] args) {
Flux<String> flux = Flux.just("Hello", "World");
flux.subscribe(System.out::println);
}
}
In this example, Flux.just
creates a stream of two string values, which are then printed through a subscriber. Understanding these types sets the foundation for effective reactive programming.
Common Pitfalls
1. Not Understanding the Reactive Types
A major misconception among new adopters of Project Reactor is the misuse of Mono
and Flux
. Some developers may treat them as traditional collections, failing to recognize their asynchronous and event-driven nature.
Why this is an issue?
By misunderstanding reactive types, developers may inadvertently make anti-patterns, such as attempting to iterate over a Flux
like a list.
Flux<Integer> numbers = Flux.range(1, 10);
for (Integer number : numbers) {
System.out.println(number); // This won't work!
}
To process each item, you should use operators like subscribe
, map
, or flatMap
.
2. Blocking Calls in Reactive Streams
One of the significant advantages of Project Reactor is its non-blocking nature. However, many developers inadvertently introduce blocking calls into their streams, which can severely degrade performance.
Example:
Consider the following snippet, where a blocking database call is made within the reactive chain:
Flux<User> userFlux = userRepository.findAll(); // This call is non-blocking
userFlux
.map(user -> userService.findInfo(user.getId())) // This may be a blocking call
.subscribe(System.out::println);
Solution
Always use asynchronous alternatives when possible! If your service layer needs to be reactive, make sure all calls respect the reactive paradigm.
3. Improper Error Handling
Error handling is another critical aspect of working with reactive streams. Failing to handle errors properly can lead to silent failures in the application.
Why is this problematic?
Without proper error management, exceptions in the stream can lead to unwanted behavior or application crashes.
Correct Error Handling Example:
Use onErrorReturn
, onErrorResume
, or doOnError
to manage errors effectively.
Flux<String> flux = Flux.just("1", "2", "3", "oops")
.map(Integer::parseInt)
.onErrorReturn(0); // Fallback to 0 in the case of an error
flux.subscribe(System.out::println);
4. Mismanaging Backpressure
Backpressure is a mechanism to handle stream data flow effectively. If a subscriber cannot keep up with the rate of emitted items, it can cause memory issues or system crashes.
Example of Mismanagement:
Flux<Integer> numberFlux = Flux.range(1, 100);
numberFlux
.subscribe(num -> {
try {
Thread.sleep(100); // Simulating processing time
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(num);
});
In the above code, if numberFlux
emits values faster than they can be processed, backpressure handling is overlooked.
Solution:
Use backpressure strategies like onBackpressureBuffer
or subscribeOn
for efficient resource management.
5. Ignoring Resource Management
Resources such as connections, threads, or file handles are finite. Failing to manage them in a reactive application can lead to leaks and performance degradation.
Example:
Flux<String> dataStream = Flux.create(sink -> {
while (true) {
sink.next("data");
}
});
dataStream.subscribe(System.out::println);
The above code will run indefinitely, consuming resources without bounds.
Solution:
Always ensure that reactive streams are bounded and proper cancellation is handled to avoid resource leaks.
Best Practices to Avoid Pitfalls
- Continue Learning: Familiarize yourself with the official Project Reactor documentation. Good understanding is key.
- Use the Right Operators: Leverage operators that suit your data transformation and flow requirements.
- Error Handling First: Develop a strong error handling strategy that fits your application's needs.
- Test Extensively: Use reactive testing libraries to ensure your streams behave as expected.
- Use Debugging Tools: Tools like
Hooks.onOperatorDebug()
can be beneficial for tracking errors and debugging.
To Wrap Things Up
Reactive programming with Project Reactor opens up extraordinary possibilities for building responsive, resilient, and efficient applications. However, it’s crucial to navigate the landscape wisely.
By understanding the core concepts, avoiding common pitfalls, and adhering to best practices, you can leverage the full power of Project Reactor. As you refine your reactive programming skills, continue exploring resources, and contribute to the vibrant community of developers committed to mastering this exciting paradigm.
To delve deeper into reactive programming, consider exploring other resources like ReactiveX that complement your Project Reactor proficiency. Happy coding!
Checkout our other articles