Common Pitfalls in Java Swing: How to Avoid UI Chaos
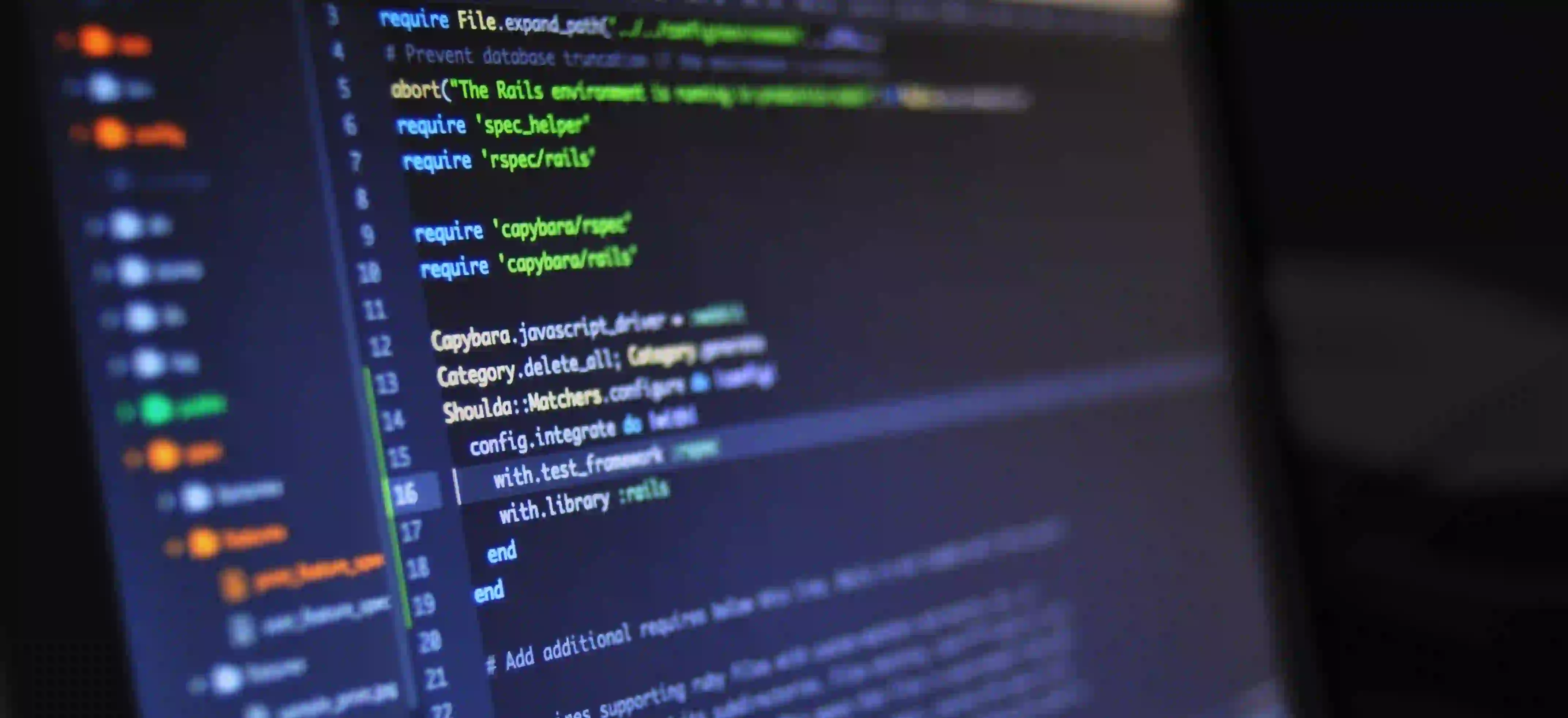
Common Pitfalls in Java Swing: How to Avoid UI Chaos
Java Swing is a powerful toolkit for creating rich graphical user interfaces (GUIs) in Java applications. While Swing provides many advanced features, it also comes with its own share of challenges. If not navigated correctly, these challenges can lead to UI chaos, resulting in a poor user experience. In this blog post, we will discuss common pitfalls in Java Swing and the best practices you can implement to avoid them.
Table of Contents
- Understanding Swing Threading Issues
- Overusing Heavyweight Components
- Neglecting UI Responsiveness
- Inefficient Layout Management
- Ignoring Accessibility Features
- Conclusion
Understanding Swing Threading Issues
One of the most common pitfalls in Java Swing is improper handling of threads. Swing is not thread-safe, which means that all updates to the GUI should be executed on the Event Dispatch Thread (EDT). Violating this rule can lead to unexpected behavior, including UI flickering and even deadlocks.
Example: Invoking Updates on the EDT
import javax.swing.*;
public class SwingExample {
public static void main(String[] args) {
// Ensure the GUI updates are executed on the EDT
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Swing Example");
JButton button = new JButton("Click Me");
button.addActionListener(e -> {
System.out.println("Button Clicked");
});
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
In this example, we use SwingUtilities.invokeLater()
to ensure that the GUI is created on the EDT. This way, we maintain thread safety while creating UI components.
Why This Matters
Calling SwingUtilities.invokeLater()
ensures that our UI remains responsive and behaves as expected. Ignoring this can lead to frustrating experiences where the UI freezes or behaves inconsistenly.
Overusing Heavyweight Components
Swing offers both lightweight and heavyweight components. Heavyweight components are tied to native screen resources and can cause performance issues. Overusing them can lead to complex UI issues, including z-order problems and rendering inconsistencies.
Example: Preference for Lightweight Components
import javax.swing.*;
public class LightweightExample {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Lightweight Component Example");
JLabel label = new JLabel("This is a lightweight label");
label.setOpaque(true); // Make it visible
frame.add(label);
frame.setSize(300, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
}
In this case, we opted for a lightweight JLabel
instead of a heavyweight component. Lightweight components tend to be more efficient and compatible with Swing’s painting model.
Why This Matters
Choosing lightweight components whenever possible eliminates render issues and increases performance. It keeps your UI clean and manageable.
Neglecting UI Responsiveness
A responsive UI enhances user experience. Long-running tasks executed on the EDT can freeze your interface, negatively affecting interaction. To avoid this, employ background threads or SwingWorker for time-consuming tasks.
Example: Using SwingWorker for Background Tasks
import javax.swing.*;
public class SwingWorkerExample {
public static void main(String[] args) {
JFrame frame = new JFrame("SwingWorker Example");
JButton button = new JButton("Perform Long Task");
button.addActionListener(e -> {
button.setEnabled(false);
// Start a background task
new SwingWorker<Void, Void>() {
@Override
protected Void doInBackground() {
// Simulated long task
try {
Thread.sleep(3000);
} catch (InterruptedException ignored) {}
return null;
}
@Override
protected void done() {
button.setEnabled(true);
}
}.execute();
});
frame.add(button);
frame.setSize(300, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why This Matters
By utilizing SwingWorker, we keep our UI responsive while offloading long tasks from the EDT. This tactical separation reduces frustration and improves user satisfaction.
Inefficient Layout Management
Poor layout management can lead to a chaotic user interface. Java Swing offers several layout managers, such as BorderLayout
, FlowLayout
, and GridBagLayout
, which help organize components effectively.
Example: Using GridBagLayout
import javax.swing.*;
import java.awt.*;
public class GridBagExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridBagLayout Example");
frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.fill = GridBagConstraints.HORIZONTAL;
JButton button1 = new JButton("Button 1");
gbc.gridx = 0; gbc.gridy = 0;
frame.add(button1, gbc);
JButton button2 = new JButton("Button 2");
gbc.gridx = 1; gbc.gridy = 0;
frame.add(button2, gbc);
JButton button3 = new JButton("Button 3");
gbc.gridx = 0; gbc.gridy = 1;
gbc.gridwidth = 2; // Spanning over two columns
frame.add(button3, gbc);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why This Matters
Efficient layout management with GridBagLayout
ensures that components are displayed correctly across different screen sizes. A well-organized layout improves usability and aesthetics.
Ignoring Accessibility Features
Creating an accessible UI should always be a priority. Accessibility features allow users with disabilities to interact with your application. Swing offers support for accessibility through the Accessible
interface.
Example: Enabling Accessibility
import javax.swing.*;
public class AccessibleExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Accessible Example");
JButton button = new JButton("Press Me");
button.setAccessibleDescription("Button to trigger an action");
button.addActionListener(e -> {
System.out.println("Button Pressed");
});
frame.add(button);
frame.setSize(300, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why This Matters
By using accessible descriptions and features, you make your application usable for a broader audience. Ignoring these features can alienate essential user bases.
Final Considerations
Avoiding these common pitfalls in Java Swing is crucial for creating a smooth and efficient user interface. Employing best practices related to threading, components, layout management, responsiveness, and accessibility is key to ensuring a positive user experience.
For more Java Swing resources, check out:
Navigating the complexities of Java Swing offers the chance to create superb applications. By considering these pitfalls and adopting the suggested practices, you will pave the way for a more organized and successful UI design. Happy coding!