How to Handle Null with Java 14's Records Effectively
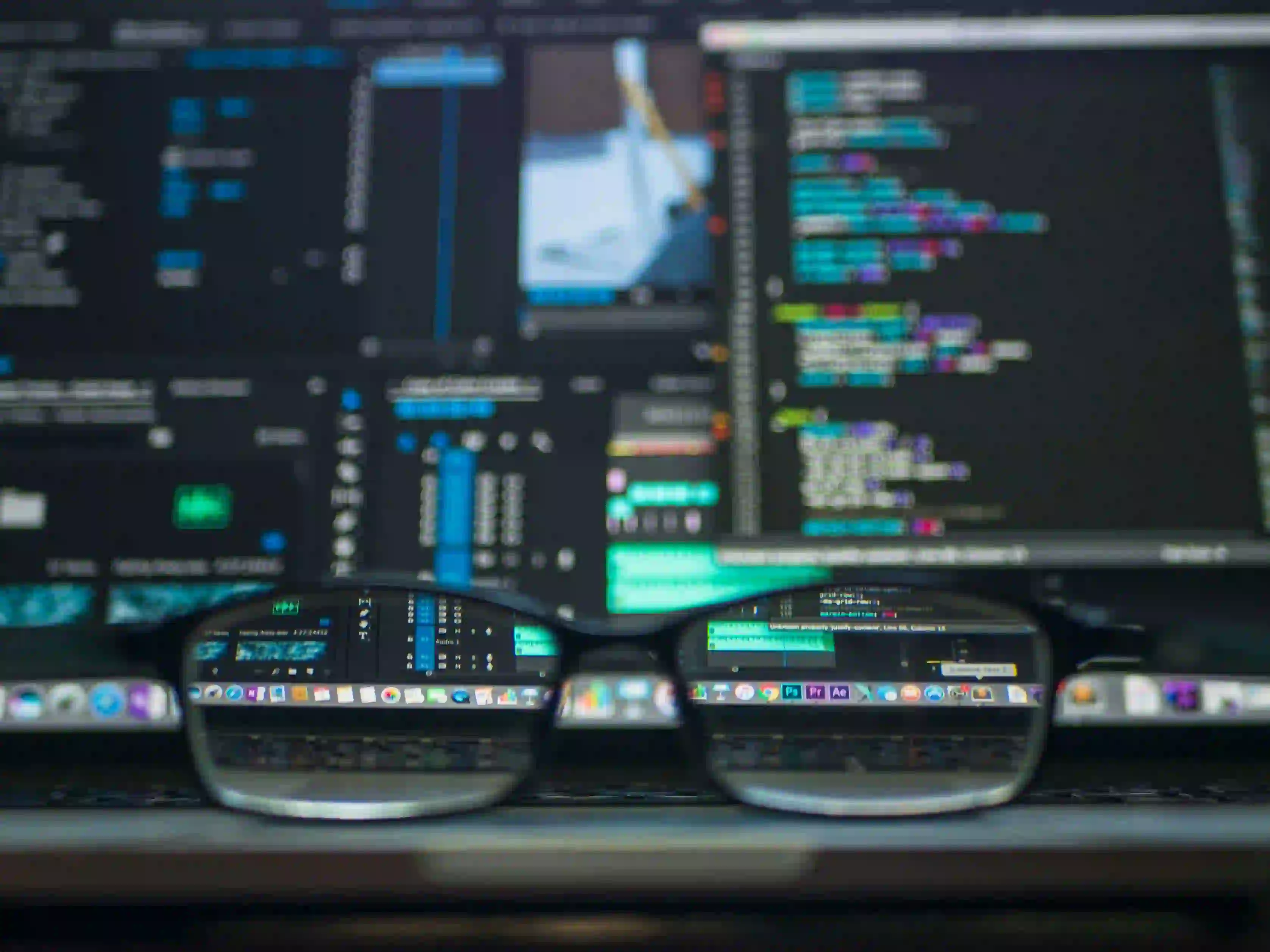
How to Handle Null with Java 14's Records Effectively
Java has come a long way since its inception in 1995. With the release of Java 14, one of the highlighted features was Records—a concise way to create data-carrier classes. Records provide numerous advantages, like reduced boilerplate code and automatic implementations of equals
, hashCode
, and toString
methods. However, one concern that comes with using Records is managing null values effectively. In this post, we will explore how to handle null in Java 14's Records effectively, offering practical code snippets and insights.
What Are Records?
Before diving into null handling, let's clarify what Records are. In essence, a Record in Java is a special kind of class designed to hold immutable data. Here’s a simple example:
public record Person(String name, int age) {}
In this record, we have defined a Person
with a name and an age. The primary use of records is to hold data, and automatically generated methods make them straightforward to use.
Why Use Records?
- Less Boilerplate: Records vastly reduce the amount of code you need to write.
- Immutability: Once created, the fields of a record cannot be changed, making your data concise and thread-safe.
- Built-in Methods:
equals
,hashCode
, andtoString
are generated automatically, offering consistency across your data types.
Now, let's explore how to handle null values when using records.
Handling Null in Records
1. Constructor Checking
One way to handle null values in records is to conduct validation checks in the constructor. While Java records come with a built-in constructor, you can create your own to enforce non-null constraints. Here’s how:
public record Person(String name, int age) {
public Person {
if (name == null) {
throw new IllegalArgumentException("Name cannot be null");
}
}
}
Why Use Constructor Checking?
The constructor enforcement ensures that any instance of Person
will always have a valid name
. Attempting to create a Person
with a null name
will throw an exception gracefully, giving developers insight into issues at the point of object creation.
2. Optional Fields
Sometimes settling for a null value simply isn't optimal. Instead, we can use the Optional
class to represent fields that may or may not be present. Here’s an updated version of our Person
record:
import java.util.Optional;
public record Person(Optional<String> name, int age) {
public Person {
// You may choose to throw an exception if 'age' is negative
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
}
}
In this example, the name
field is now an Optional
. You can use it like this:
Person person = new Person(Optional.of("Alice"), 30);
Person personWithNoName = new Person(Optional.empty(), 25);
Why Use Optional Fields?
Using Optional
provides a more descriptive way of handling uncertainty in data. It encourages developers to handle potential absence of values explicitly rather than relying on null checks.
3. Default Values
If an attribute is often null and you have a meaningful default, consider initializing it within the record definition. This approach ensures that records are always in a valid state:
public record Person(String name, int age) {
public Person {
this.name = name != null ? name : "Unknown";
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
}
}
In this adjustment, the name
will default to "Unknown" if a null value is passed. Here is how it looks in practice:
Person personWithDefaultName = new Person(null, 30);
System.out.println(personWithDefaultName);
// Output: Person[name=Unknown, age=30]
Why Use Default Values?
Default values provide a shortcut for situations where you are comfortable giving a standard fallback. They eliminate the risk of null assignments and ensure your records always return a sensible value.
4. Using Validation Libraries
While manual handling of nulls is effective, there are libraries that can streamline this process. Libraries such as Apache Commons Validator or Hibernate Validator allow for more complex validations without cluttering your codebase.
Here’s how you might use a custom validation library:
import org.hibernate.validator.constraints.NotEmpty;
import javax.validation.Valid;
import javax.validation.constraints.Min;
public record Person(@NotEmpty String name, @Min(0) int age) {}
In this scenario, you define constraints which can be validated using the Hibernate Validator engine. Here’s how it helps:
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
Set<ConstraintViolation<Person>> violations = validator.validate(person);
Why Use Validation Libraries?
These libraries provide robust, reusable validations that can significantly enhance your code's readability and maintainability. They scale your validation needs as your application grows.
Bringing It All Together
In this blog post, we have explored effective approaches to handle null values when using Java 14's Records. By implementing constructor checks, utilizing Optional
fields, establishing default values, and considering validation libraries, you can manage null situations gracefully. Each method has its own benefits and trade-offs, so it is crucial to choose the approach that best fits your application's needs.
Understanding how to handle null effectively within records can not only improve the reliability of your Java applications but also enhance the developer experience by reducing the complexity of null checks.
For further reading, check out the Java 14 Documentation on Records or dive into Using Optional Class in Java for better null handling practices.
By applying these techniques, you will write cleaner, more effective code in Java and embrace the true power of records. If you enjoyed this post or have questions about null handling in Java Records, feel free to leave a comment!
Happy coding!