Common Pitfalls When Using GraalVM with Java
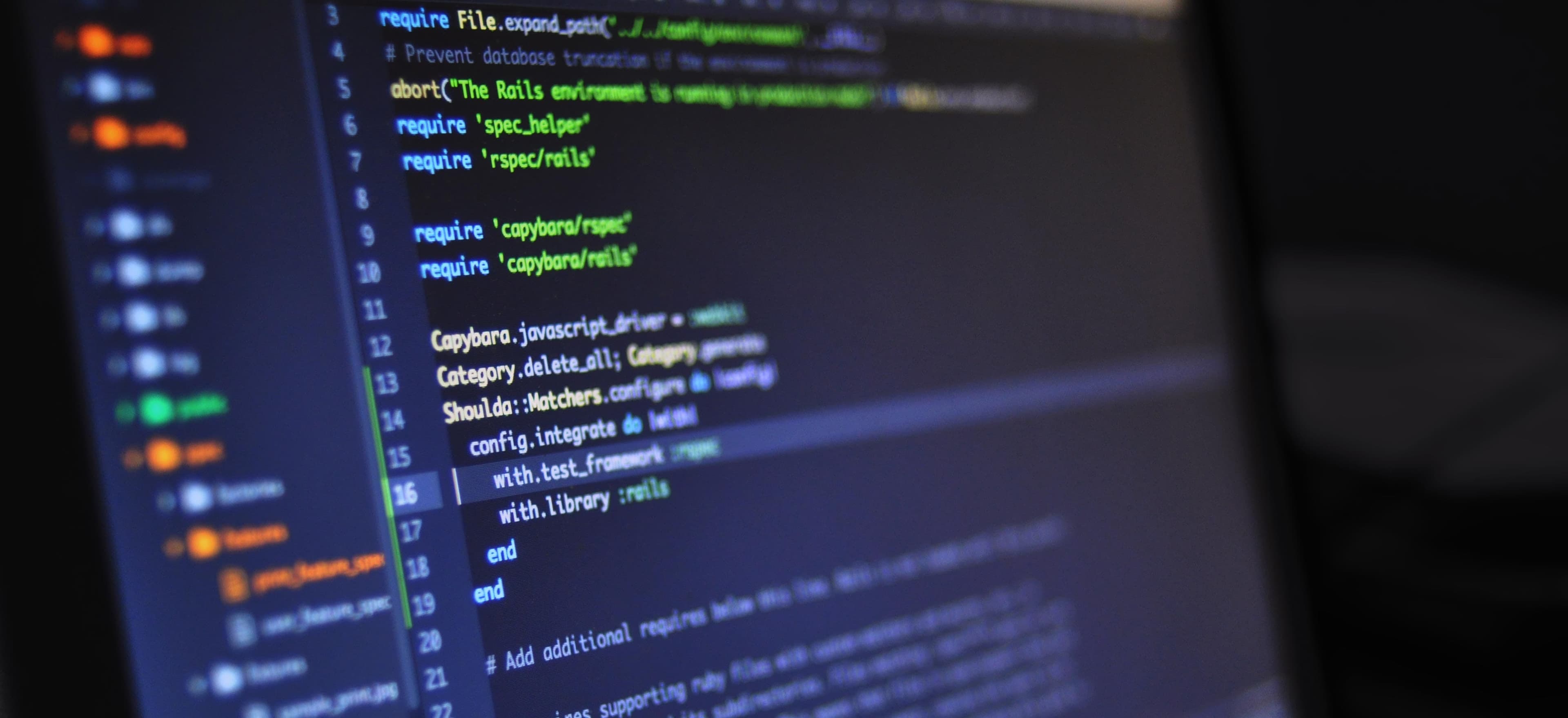
- Published on
Common Pitfalls When Using GraalVM with Java
GraalVM is an advanced, high-performance virtual machine designed to run applications written in Java and other languages. Its capability to compile Java code ahead-of-time (AOT) and support for polyglot programming makes it an attractive option for developers. However, as with any powerful tool, missteps are common. This blog post delves into the common pitfalls encountered when using GraalVM with Java, and how to navigate them effectively.
Understanding GraalVM: A Quick Overview
GraalVM builds on the Java Virtual Machine standard, improving performance with its Just-In-Time (JIT) compilation capabilities while adding support for polyglot applications. GraalVM allows you to run Java code alongside other languages like JavaScript, Ruby, and R.
Key Features of GraalVM
- AOT Compilation: Reduce startup times through ahead-of-time compilation.
- Polyglot Support: Execute multiple programming languages in a single application.
- Native Image Generation: Compile your application to a native executable for faster runtime performance.
GraalVM, while powerful, comes with challenges. Here’s a look at some common pitfalls developers face.
1. Ignoring Native Image Configuration
GraalVM provides the capability to compile Java applications into native executables. However, the configuration for this process is essential and often overlooked.
Why It Matters
When creating a native image, GraalVM must understand the types and methods used during runtime. If it cannot access all necessary code paths, you may encounter runtime exceptions after compilation.
Example
Here’s how to configure native image generation properly:
native-image -jar my-app.jar --initialize-at-build-time=my.package.ClassName
In this command:
-jar
specifies the JAR file to compile.--initialize-at-build-time
resolves class initialization at build time, making your native image more compliant and stable.
Avoiding the Pitfall
Always ensure that your configuration captures all necessary paths:
- Use the
--report-unsupported-elements-at-runtime
flag to help identify unsupported code. - Thoroughly test the application before deploying a native image.
2. Mismanaging Dependencies
GraalVM can execute a wide range of libraries, but sometimes, native images may not replicate the behavior of traditional JVM applications due to dependency issues.
Why It Matters
Certain libraries may rely on dynamic class loading or reflection, which might not be supported in the native image. This leads to exceptions and unstable behavior.
Example of Managing Dependencies
Take, for example, libraries like Spring Boot that rely heavily on reflection. You must explicitly define which classes are reachable:
@NativeImageHint
{
reflection = {
types = { "com.myapp.models.User" }
}
}
Avoiding the Pitfall
- Analyze your dependencies and remove unused libraries. Tools like Maven Dependency Plugin can help with this task.
- Use GraalVM’s
native-image-agent
to capture application behavior while running under the JVM to generate the necessary configuration files.
3. Underestimating Memory Usage
Building native images can lead to higher memory consumption than anticipated. Developers often assume that native images should be more memory-efficient without proper profiling.
Why It Matters
Certain optimizations in JIT compilation for existing JVM usage can improve memory efficiency. When compiled into a native image, your application may exhibit increased memory usage if not configured optimally.
Example Alert
Be cautious with large collection objects or heavy libraries being loaded into memory:
List<String> strings = new ArrayList<>();
for (int i = 0; i < 1000000; i++) {
strings.add("String " + i);
}
Avoiding the Pitfall
- Conduct profiling through the GraalVM VisualVM or similar tools to examine memory allocation.
- Optimize your application data structures and load necessary resources lazily.
4. Overlooking Polyglot Language Features
GraalVM excels in running polyglot applications, yet developers sometimes run into problems when integrating multiple languages due to their distinctions.
Why It Matters
Each language has different runtime characteristics and interoperability expectations. Assumptions from one language's framework may not hold true in another.
Example
Suppose you are trying to leverage Java and JavaScript together:
Value value = Context.create().eval("js", "2 + 2");
System.out.println(value.asInt());
In this code:
- We're using GraalVM's context to evaluate JavaScript code from Java.
Avoiding the Pitfall
- Familiarize yourself with the intricacies of each language to ensure smooth interoperability.
- Utilize GraalVM documentation for understanding polyglot integration better: GraalVM Documentation.
5. Skipping Exception Handling
Underestimating how exceptions are managed can lead to runtime surprises. GraalVM may handle exceptions differently when compiled into native images.
Why It Matters
Native images often reduce stack trace messages compared to JVM execution. Critical error details might be lost, making debugging problematic.
Example
You might implement simple try-catch logic:
try {
// Code that could throw an exception
} catch (Exception e) {
System.out.println("An error occurred: " + e.getMessage());
}
Avoiding the Pitfall
- Implement thorough exception logging and debugging support.
- Use GraalVM's
-Dgraalvm.PrintCompilation
during development to get more insights.
Final Thoughts
GraalVM offers Java developers unique performance and versatility opportunities. However, success hinges on awareness and management of common pitfalls.
By heeding the aforementioned warnings and strategies, developers can ensure they make the most of GraalVM while sidestepping issues that may hamper their applications.
If you want to further explore specific areas regarding GraalVM, consider checking the GraalVM Official Website or diving into community discussions through platforms like Stack Overflow. Remember, while GraalVM provides powerful tools, thorough understanding and careful configuration are pivotal for success!
Happy coding!
Checkout our other articles