Protecting Your Java Apps: Key Serialization Vulnerabilities
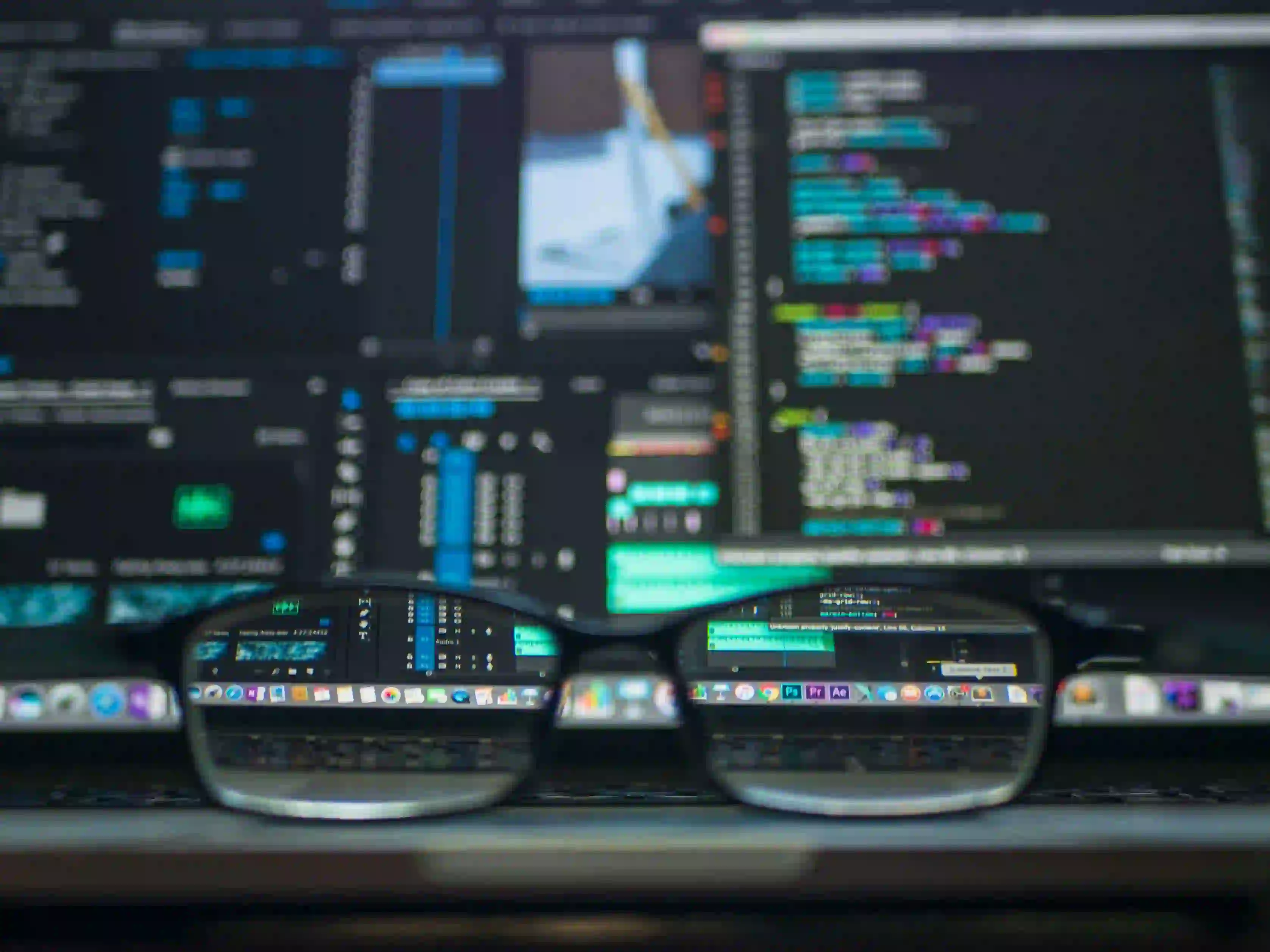
Protecting Your Java Apps: Key Serialization Vulnerabilities
Java serialization is a powerful feature that transforms Java objects into byte streams for storage or transmission. While it simplifies tasks like saving objects to files or sending them across networks, it also introduces significant security risks. In this post, we'll explore key serialization vulnerabilities in Java applications, provide you with examples, and discuss protective measures you can take.
Understanding Serialization in Java
Serialization in Java is accomplished through the Serializable
interface, which allows developers to convert an object into a format that can be easily stored or transmitted. Deserialization, on the other hand, involves reconstructing the object from this byte stream.
Here’s a simple example demonstrating serialization:
import java.io.*;
public class Person implements Serializable {
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
// Serialization example
public class SerializeDemo {
public static void main(String[] args) {
Person person = new Person("John Doe", 30);
try (FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut)) {
out.writeObject(person);
System.out.println("Serialized data is saved in person.ser");
} catch (IOException e) {
e.printStackTrace();
}
}
}
This basic code creates a Person
object and serializes it to a file.
While this demonstrates a legitimate use of serialization, it also serves as a reminder: when deserialized, an object can execute arbitrary code if not properly handled.
Common Serialization Vulnerabilities
1. Insecure Deserialization
Insecure deserialization occurs when an application accepts serialized data from untrusted sources. An attacker can manipulate this data to execute malicious code or launch a denial-of-service attack.
Example of Insecure Deserialization:
Suppose we have the following code:
import java.io.*;
public class DeserializeDemo {
public static void main(String[] args) {
Person person = null;
try (FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn)) {
person = (Person) in.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
System.out.println("Deserialized Person: " + person.getName());
}
}
Let’s say an attacker sends a modified serialized person
object that alters its attributes or methods to introduce harmful behavior.
2. Class Injection
When an attacker manipulates the type of the deserialized object, it leads to class injection vulnerabilities. Java uses the type information during deserialization, which means the attacker can control what class is instantiated.
Example of Class Injection:
By providing a malicious serialized object that references a class capable of executing harmful methods, attackers can control the application's behavior. You can learn more about preventing these vulnerabilities in depth at articles by OWASP.
3. Remote Code Execution
When deserializing an object, if the class loaded has methods that can execute arbitrary code, attackers can exploit this to run harmful code on the server.
Best Practices for Securing Serialization
To protect your Java application from these serialization vulnerabilities, consider the following best practices:
1. Avoid Serialization When Possible
The most effective solution is to avoid serialization unless necessary. If you can achieve your goal without it, do so.
2. Implement a Custom Serialization Mechanism
If serialization is necessary, implement a custom serialization mechanism. By defining writeObject
and readObject
methods, you can control what gets serialized and deserialized.
private void writeObject(ObjectOutputStream oos) throws IOException {
// Custom serialization logic
oos.defaultWriteObject();
// Do any additional logic if necessary
}
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
// Custom deserialization logic
ois.defaultReadObject();
// Validate the object state before using it
}
3. Verify Types During Deserialization
Checking the class type of deserialized objects before casting them helps ensure that the serialized data hasn't been tampered with:
Object obj = ois.readObject();
if (obj instanceof Person) {
person = (Person) obj;
} else {
throw new InvalidClassException("Invalid class type.");
}
4. Use Third-Party Libraries for Serialization
Consider leveraging libraries like Kryo or JSON-based alternatives which provide better security mechanisms compared to Java’s default serialization.
5. Secure Class Loader
Prevent using insecure class loaders that allow loading untrusted classes during the deserialization process. You may also want to restrict the classes that can be loaded by using a whitelist approach.
6. Implement Integrity Checks
When handling serialized data, implement integrity checks like checksums or digital signatures to confirm authenticity before deserialization.
Closing Remarks
Java's serialization can greatly simplify object management, but it also opens doors to potential attacks. By understanding the vulnerabilities linked to serialization and adhering to best practices, you can safeguard your applications against these threats.
To further dive into this significant topic, consider reading Java Serialization Demystified and exploring OWASP’s resources for more security measures.
Stay alerted and proactive about serialization vulnerabilities. Your application's security relies on it.
Feel free to reach out if you have any questions or wish to discuss this topic further!