Bridging the Gap: Java's Role in AI Development Challenges
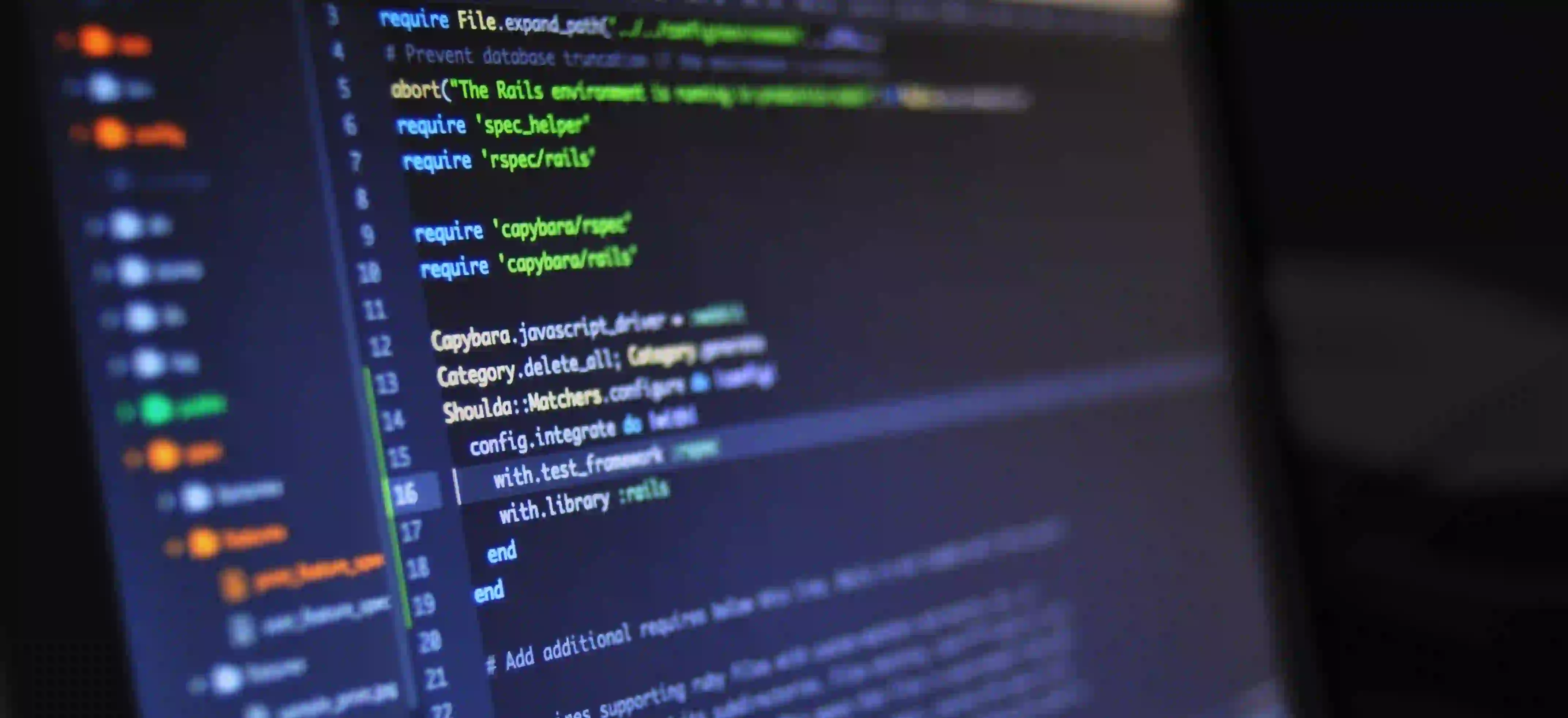
Bridging the Gap: Java's Role in AI Development Challenges
Artificial Intelligence (AI) is revolutionizing the tech landscape, pushing boundaries in various sectors from healthcare to finance. Among the myriad programming languages available, Java has carved out a unique niche within AI development. This blog post delves into how Java contributes to addressing the challenges faced in AI development and why it remains a relevant choice for AI practitioners.
Why Choose Java for AI?
Java’s robustness, portability, and ease of use provide a strong foundation for AI development. Here are some of the key reasons Java is favored in this field:
-
Platform Independence: Java’s "Write Once, Run Anywhere" (WORA) capability means that applications can run on any device equipped with the Java Virtual Machine (JVM). This flexibility is essential for AI applications, which often need to be deployed across different systems.
-
Scalability: Java’s strong concurrency support allows developers to create applications that manage multiple tasks simultaneously, a common requirement in AI for processing large datasets.
-
Rich Libraries: Java boasts a plethora of libraries that facilitate machine learning and data processing. Frameworks like Deeplearning4j and Weka streamline the creation of complex algorithms without building from scratch.
-
Strong Community Support: With a robust community of developers and extensive documentation, finding resources, resolving issues, and sharing knowledge becomes significantly easier.
Challenges in AI Development
While Java offers significant advantages, the journey of AI development is fraught with challenges. Below are some common hurdles and how Java helps address them.
1. Complexity of Algorithms
AI algorithms can often be complex, requiring a deep understanding of math and statistics. Java helps mitigate this complexity through its rich set of libraries that provide built-in implementations of these algorithms.
Example Code Snippet
Here’s an example of how you can use the Weka library to implement a simple classification algorithm:
import weka.classifiers.Classifier;
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class WekaExample {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("path/to/your/dataset.arff");
Instances data = source.getDataSet();
// Set class index to the last attribute
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
// Build a classifier
Classifier classifier = new J48(); // J48 is a type of decision tree
classifier.buildClassifier(data);
System.out.println("Classifier built successfully");
}
}
Commentary
The code above demonstrates how to utilize Weka for data classification. This approach allows developers to focus on data analysis and interpretation instead of algorithm implementation. By leveraging existing libraries, we bridge the gap between complex theoretical concepts and practical applications.
2. Data Handling and Processing
Data preparation and handling pose significant challenges in AI. Java’s capabilities shine here with libraries like Apache Spark and Java 8 Streams that facilitate large-scale data processing.
Example Code Snippet
Here’s how you can use Java Streams to filter and process data:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
// Sample data
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Filter even numbers
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
Commentary
In this code, Java Streams allow for concise and readable data filtering. Operations such as filtering, mapping, and reducing can be performed in a functional style, making the code more expressive and easier to maintain. By simplifying data manipulation, we effectively lower the barrier for developing complex AI systems.
3. Integration with Other Technologies
AI systems need to integrate seamlessly with existing applications, which can be a bottleneck for many developers. Java’s widespread use in enterprise environments makes it easier to create integrations.
Java can interact with various databases, APIs, and other services. The popularity of Java in server-side applications further ensures that AI models can be easily integrated into larger systems.
Example Code Snippet
To interact with a database for training an AI model, the JDBC API can be used:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/your_database";
String user = "username";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement()) {
String query = "SELECT * FROM your_table";
ResultSet resultSet = statement.executeQuery(query);
while (resultSet.next()) {
System.out.println("Data: " + resultSet.getString("column_name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In this snippet, we connect to a MySQL database and retrieve data using JDBC. This connection allows AI systems to access data directly, enriching the datasets used for training models. In the world of AI, where data drives insights, efficient integration is crucial.
4. Performance Optimization
AI algorithms can be resource-intensive, and optimizing performance is critical. While Java might not always be the fastest language, its performance can often be sufficient for AI applications with proper optimization techniques.
Bringing It All Together
While navigating the complexities of AI development can be challenging, Java's versatility offers viable solutions. From handling intricate algorithms to managing data and ensuring smooth integrations, Java plays a pivotal role in bridging the gap in AI development challenges.
As we continue to explore and expand AI technologies, Java remains a solid choice for developers looking to leverage its extensive libraries, community support, and robust performance. If you're interested in further exploring the intersection of Java and AI, consider reading about Deeplearning4j, a powerful framework for deep learning in Java, or Weka, which provides a comprehensive suite of machine learning algorithms.
By understanding and utilizing Java’s features effectively, developers can focus on innovation and problem-solving, rather than getting bogged down by the complexities of AI technology. Embrace Java, and let's bridge the gap in AI development challenges together!