Overcoming Common Pitfalls in Java Chatbot Development
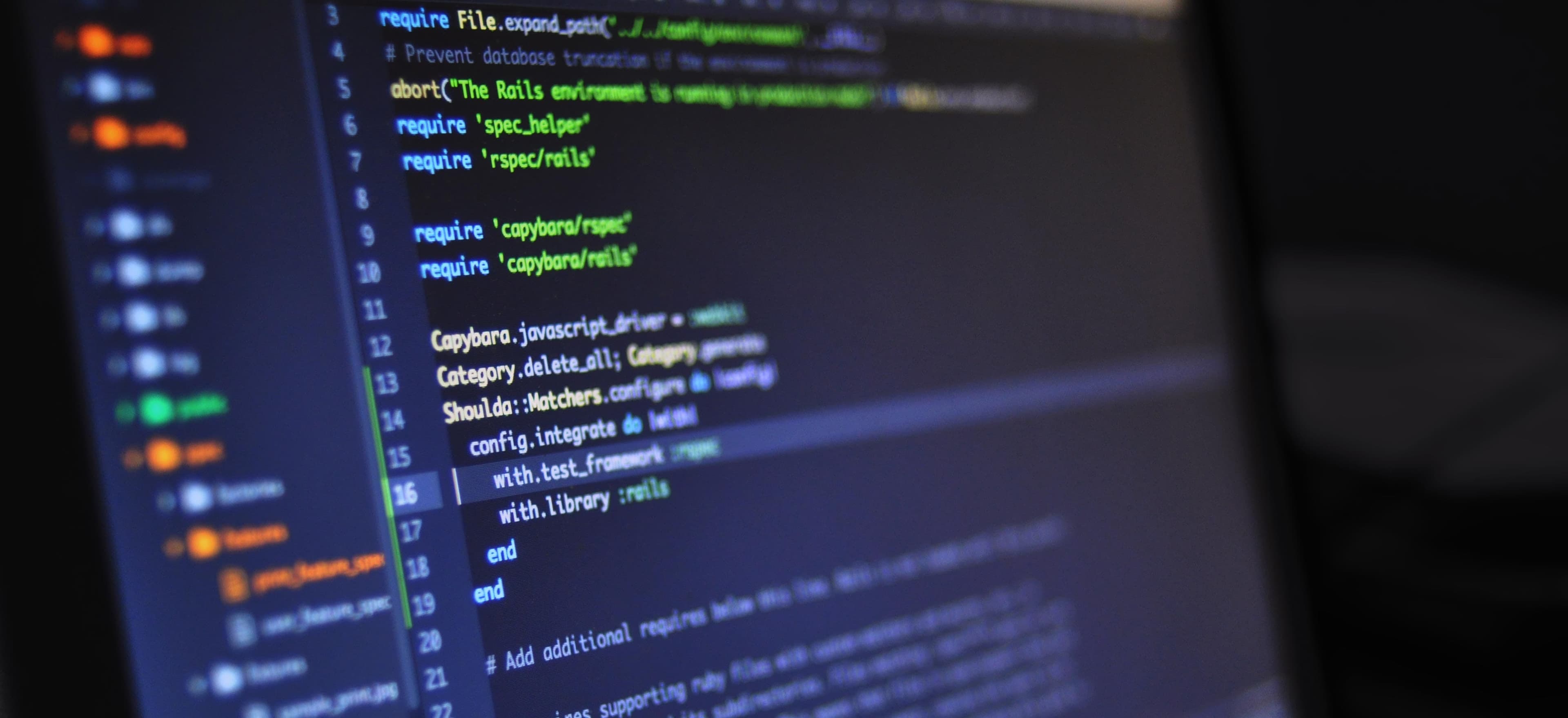
- Published on
Overcoming Common Pitfalls in Java Chatbot Development
Java has long been a preferred language for building robust applications. Its platform independence, strong community support, and rich ecosystem make it an attractive choice for developing chatbots. However, like any complex application, developing a chatbot in Java comes with its own set of challenges. In this blog post, we will explore common pitfalls in Java chatbot development and how to overcome them, providing practical solutions and code snippets along the way.
Table of Contents
- Understanding Chatbots
- Common Pitfalls
- Practical Solutions
- Conclusion
Understanding Chatbots
Before diving into the pitfalls, it’s important to understand what a chatbot is. A chatbot is an application that communicates with users using natural language processing (NLP). It can be text-based or voice-activated and is designed to carry out specific tasks or provide information.
The key components of a chatbot include:
- Input Processing: Capturing and interpreting user input.
- Response Generation: Generating appropriate responses based on user input.
- Context Management: Keeping track of the conversation context for continuity.
Common Pitfalls
1. Lack of Clear Requirements
One of the most significant mistakes developers make is jumping into development without a clear understanding of what the chatbot should accomplish. Failing to define clear objectives can lead to scope creep and inefficient designs.
Solution: Before coding, outline the chatbot’s purpose. What questions should it answer? What actions should it perform? This blueprint will guide the development process.
2. Ignoring User Experience (UX)
Many developers focus excessively on functionality, neglecting the user experience. A bot that satisfies functionality but frustrates users with poor design will fail.
Solution: Implement conversational design principles. Create user-friendly dialogues and responses.
public class Chatbot {
public String getGreeting(String userName) {
// A simple welcoming message that engages the user.
return String.format("Hello, %s! How can I assist you today?", userName);
}
}
This snippet demonstrates a simple greeting method that personalizes the interaction, enhancing user engagement right from the start.
3. Limited Natural Language Processing (NLP)
Choosing basic string matching algorithms instead of robust NLP tools can result in a bot that struggles to understand variations in user input. Relying on simple keywords may lead to misunderstandings.
Solution: Utilize NLP libraries like Stanford NLP or Apache OpenNLP that can identify intent and extract entities from user queries effectively.
4. Poor Context Management
Without effective context management, a chatbot may provide responses that are irrelevant or disconnected from earlier parts of the conversation. This often leads to a disjointed user experience.
Solution: Maintain a context object to keep track of user interactions.
import java.util.HashMap;
import java.util.Map;
public class Context {
private Map<String, String> attributes;
public Context() {
attributes = new HashMap<>();
}
// Method to set the context attributes
public void setAttribute(String key, String value) {
attributes.put(key, value);
}
// Method to get the context attributes
public String getAttribute(String key) {
return attributes.getOrDefault(key, null);
}
}
In this snippet, the Context
class allows the chatbot to remember certain attributes about the user or conversation, facilitating better continuity.
5. Insufficient Testing and Debugging
Rushed testing can leave critical bugs that impact the bot’s performance and user experience. Neglecting to conduct real-world testing can lead to a lack of reliability.
Solution: Develop a comprehensive testing strategy that includes unit tests and user acceptance tests.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class ChatbotTest {
@Test
void testGreeting() {
Chatbot chatbot = new Chatbot();
String expected = "Hello, John! How can I assist you today?";
assertEquals(expected, chatbot.getGreeting("John"));
}
}
Unit testing, demonstrated above, can ensure the chatbot’s components function as intended.
6. Not Prioritizing Scalability
As user engagement grows, the chatbot should be able to handle increased traffic. Developers often build chatbots without considering their scalability, which can lead to performance issues down the line.
Solution: Employ a scalable architecture, leveraging microservices if necessary. Using cloud platforms like AWS can also help scale your chatbot.
Practical Solutions
Having walked through the common pitfalls, let’s summarize key design and development strategies for building a successful Java chatbot:
- Define Requirements: Conduct thorough planning before coding.
- User-Centric Design: Focus on creating an engaging user interface.
- Utilize Advanced NLP: Choose effective NLP libraries for understanding user intents.
- Implement Context Management: Ensure logical conversational flow.
- Thorough Testing: Develop and execute a comprehensive testing plan.
- Plan for Scalability: Design your bot with future growth in mind.
Key Takeaways
Building a chatbot in Java can be a challenging yet rewarding endeavor. By being aware of these common pitfalls and adopting practical solutions, developers can create effective conversational agents that enhance user interaction. Always remember that the key to a successful chatbot lies in a balance between functionality and user experience.
Would you like to know more about Java chatbot frameworks? Check out Spring Boot, which can help streamline your backend development. Happy coding!