Common Pitfalls in Serverless Java with AWS Lambda
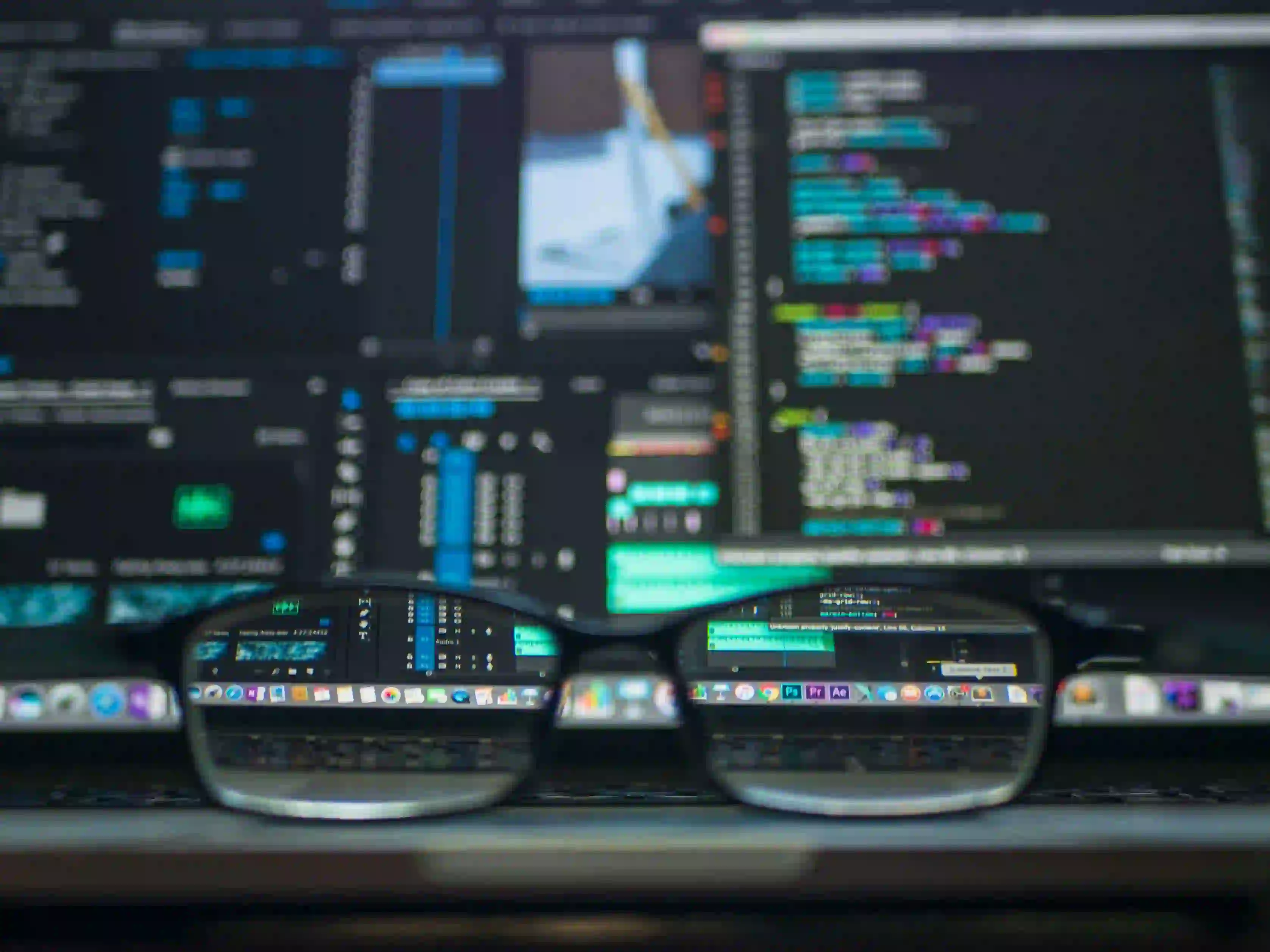
Common Pitfalls in Serverless Java with AWS Lambda
In recent years, the serverless architecture has gained immense popularity, allowing developers to build applications without the need to manage infrastructure. AWS Lambda, a leader in serverless functions, provides a seamless way to run code in response to events, scale automatically, and only pay for the compute resources you use. However, despite its advantages, developers face several common pitfalls when using Java with AWS Lambda. In this blog post, we'll explore these challenges, how to avoid them, and provide exemplary code snippets to illustrate solutions.
Understanding the Serverless Model
Before diving into the pitfalls, it's essential to understand what serverless means. With serverless computing, the cloud provider manages the infrastructure and dynamically allocates resources as needed. This allows developers to focus on writing code rather than managing servers. However, this model comes with its own set of challenges, particularly when using Java, which is traditionally not as lightweight as other languages like Python or Node.js.
Common Pitfalls in Serverless Java with AWS Lambda
Let's discuss some of the most frequent issues developers encounter when using Java in AWS Lambda.
1. Cold Start Latency
One of the most significant challenges with AWS Lambda is the "cold start" latency. Cold starts occur when a new instance of a Lambda function is created to handle requests, resulting in longer response times. This is particularly noticeable in Java due to JVM startup times.
How to Mitigate Cold Start Latency
- Use the right memory configuration: More memory not only increases the available CPU but can also reduce cold start times. Experiment with different configurations to find the optimal setting for your needs.
public class RequestHandler implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
return "Processing: " + input;
}
}
In the above code, remember, optimizing memory can lead to quicker startup time and efficient scaling.
- Reduce the package size: Minimize the size of your deployment package. Exclude unnecessary libraries and dependencies. For example, use AWS Lambda Layers to share common libraries among functions, reducing function size.
2. Managing Dependencies
Java applications often rely on numerous dependencies, which can lead to bloated deployment packages and increased cold start times.
Best Practices for Dependency Management
- Use Maven or Gradle: Utilize build tools like Maven or Gradle to manage dependencies efficiently. Ensure you are only including the necessary libraries.
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk</artifactId>
<version>1.12.80</version>
</dependency>
Using Maven's dependency management in pom.xml
, ensures only required libraries are included, reducing the overall size and improving performance.
- Dependency Injection: Consider using frameworks that support dependency injection (like Spring). This not only manages dependencies but also makes testing easier. However, keep in mind that using heavy frameworks can contribute to cold start issues.
3. Lack of Proper Exception Handling
Java's exception handling capabilities can be overlooked in serverless applications, leading to unhandled exceptions that cause Lambda functions to fail frequently.
Implementing Robust Error Handling
Always implement try-catch blocks and handle potential exceptions gracefully. Make use of structured logging to capture error details.
public String handleRequest(String input, Context context) {
try {
// process input
return "Processed: " + input;
} catch (Exception e) {
context.getLogger().log("Error processing input: " + e.getMessage());
throw new RuntimeException("Processing failed", e);
}
}
This example demonstrates the importance of controlling exceptions, which can provide insights for debugging and improve application resilience.
4. Ignoring Timeout Settings
AWS Lambda has a default timeout setting of three seconds. If your Java function takes longer than this threshold, it will terminate unexpectedly.
Setting Appropriate Timeouts
Always configure the timeout setting based on your function's specific needs. Analyze the function's performance and define a timeout that allows for sufficient processing time.
// AWS Lambda console configuration
{
"Timeout": 30 // Set to the appropriate value for your use case
}
In addition, consider breaking down longer operations into smaller functions, allowing parts of your application to respond faster.
5. Not Using Async Processing Wisely
Java provides robust threading capabilities, but AWS Lambda doesn’t support multi-threading well due to its design constraints.
How to Best Use Asynchronous Processing
-
Avoid Threading Inside Lambda: Instead of creating new threads within a Lambda function, leverage asynchronous processing through AWS services like SQS or SNS.
-
Use Lambda's Event Source Mappings: For background jobs, consider using event source mappings to trigger your Lambda from an SQS queue. This abstracts the complexity of threading.
public class QueueProcessor implements RequestHandler<SQSEvent, Void> {
@Override
public Void handleRequest(SQSEvent event, Context context) {
for (SQSEvent.SQSMessage message : event.getRecords()) {
// process each message
context.getLogger().log("Processing message: " + message.getBody());
}
return null;
}
}
Using this approach, you'll be able to scale out processing as needed and keep your Lambda simple and efficient.
6. Inefficient Handling of I/O Operations
I/O operations often result in slower Lambda execution. Java's blocking I/O can lead to performance bottlenecks.
Improving I/O Performance
-
Use Non-blocking I/O: Switch to asynchronous I/O frameworks like Vert.x or Reactor to improve throughput.
-
Leverage AWS SDK V2: The AWS SDK V2 for Java has been designed with non-blocking I/O in mind.
import software.amazon.awssdk.services.s3.S3AsyncClient;
S3AsyncClient s3 = S3AsyncClient.create();
s3.listBuckets()
.thenAccept(buckets -> buckets.buckets().forEach(bucket ->
System.out.println(bucket.name())));
Utilizing asynchronous clients can significantly reduce the latency of I/O operations, granting your Lambda function faster execution.
7. Monitoring and Debugging Challenges
Monitoring serverless applications can be challenging due to the ephemeral nature of Lambdas.
Setting Up Effective Monitoring
-
Use AWS CloudWatch: Configure logging and alarms through AWS CloudWatch. Instrument your code with sufficient logs to monitor performance and identify bottlenecks.
-
Leverage APM Tools: Consider third-party Application Performance Monitoring (APM) tools like New Relic or Datadog for more comprehensive insights into performance metrics.
Key Takeaways
Building serverless applications with Java and AWS Lambda can offer significant benefits, including reduced operational overhead and enhanced scalability. However, understanding the common pitfalls is crucial for successful implementations.
By addressing cold start latencies, managing dependencies effectively, implementing robust error handling, adjusting timeouts, using asynchronous processing wisely, optimizing I/O operations, and setting up effective monitoring, you can mitigate risks and enhance your serverless applications.
For more detailed guidance, consider checking out the AWS Lambda Documentation, which provides deeper insights into the capabilities and best practices for using Lambda.
Further Reading
- Serverless Java with AWS - Official AWS Java resource
- JavaScript vs Java in Serverless
Happy coding, and may your AWS Lambda functions run smoothly!