Common Pitfalls in Java AWS Integration and How to Avoid Them
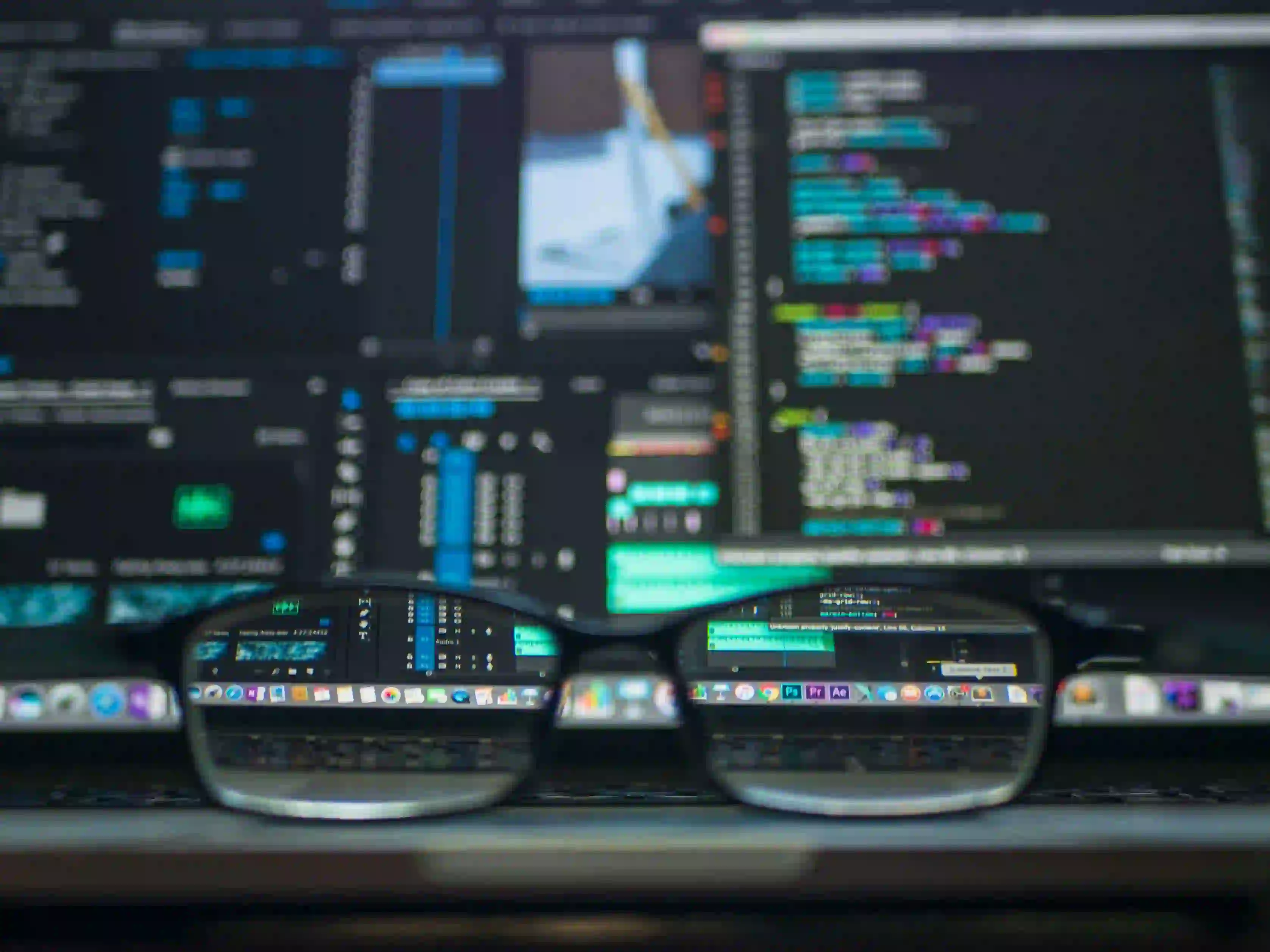
Common Pitfalls in Java AWS Integration and How to Avoid Them
Integrating Java applications with Amazon Web Services (AWS) can unleash tremendous power and scalability for your applications. However, the journey is not always smooth. In this blog post, we'll discuss common pitfalls developers encounter when performing Java AWS integration and the best practices to avoid them.
Table of Contents
- Introduction to Java AWS Integration
- Common Pitfalls
- Poor Configuration Management
- Inefficient Resource Management
- Ignoring Security Best Practices
- Neglecting Error Handling
- Overlooking SDK Versions
- Best Practices to Avoid Pitfalls
- Conclusion
The Roadmap to Java AWS Integration
AWS provides a suite of services that enhance the performance of applications, from storage to computing power. Java, being one of the most popular programming languages, is often used to build applications that interface with various AWS services. The AWS SDK for Java simplifies this integration process. However, developers often face challenges that can lead to inefficient or insecure applications.
Common Pitfalls
1. Poor Configuration Management
One common oversight is neglecting configuration management. Hardcoding AWS credentials and configurations into your application can expose your application to security risks.
Example: Hardcoded Credentials
public class AwsConfig {
private static final String AWS_ACCESS_KEY = "your-access-key";
private static final String AWS_SECRET_KEY = "your-secret-key";
public static void main(String[] args) {
// Use credentials
}
}
Why Avoid This?
Hardcoding sensitive information like access keys is a serious security risk. If the source code is exposed, anyone can access your AWS resources.
Best Practice:
Use environment variables or AWS Systems Manager Parameter Store to manage your configurations. This improves security by keeping sensitive information outside your code base.
2. Inefficient Resource Management
Another common pitfall is improper resource management.
Example: Not Closing Resources
public void connectToS3() {
AmazonS3 s3Client = AmazonS3ClientBuilder.standard().build();
// Perform operations
// Not closing the client
}
Why Avoid This?
Failing to release resources can lead to memory leaks and performance degradation.
Best Practice:
Always close your resources using try-with-resources statement in Java or explicitly close services in a finally block.
public void connectToS3() {
try (AmazonS3 s3Client = AmazonS3ClientBuilder.standard().build()) {
// Perform operations
} catch (Exception e) {
// Handle exceptions
}
}
3. Ignoring Security Best Practices
AWS services provide powerful features, but overlooking security can lead to significant vulnerabilities.
Example: Public Access to S3 Buckets
Setting up an S3 bucket without proper permissions may allow unauthorized public access.
Why Avoid This?
Public access can lead to data leaks and costs if your bucket has a large amount of data.
Best Practice:
Configure S3 bucket policies carefully and avoid enabling public access unless necessary. Utilize IAM roles and policies for granular permissions.
4. Neglecting Error Handling
Ignoring error handling in AWS service calls is a recipe for disaster.
Example: Lack of Error Handling
public void uploadToS3(InputStream inputStream, String bucketName, String key) {
AmazonS3 s3Client = AmazonS3ClientBuilder.standard().build();
s3Client.putObject(bucketName, key, inputStream, new ObjectMetadata());
// No error handling
}
Why Avoid This?
Without error handling, unanticipated issues can cause your application to crash or lead to unexpected behavior.
Best Practice:
Implement robust error handling using try-catch blocks to manage exceptions effectively.
public void uploadToS3(InputStream inputStream, String bucketName, String key) {
try {
AmazonS3 s3Client = AmazonS3ClientBuilder.standard().build();
s3Client.putObject(bucketName, key, inputStream, new ObjectMetadata());
} catch (AmazonS3Exception e) {
// Handle S3 specific exceptions
} catch (Exception e) {
// General error handling
}
}
5. Overlooking SDK Versions
The AWS SDK for Java is updated frequently, which can introduce breaking changes.
Why Avoid This?
Using outdated SDKs can expose your application to bugs or vulnerabilities that have been fixed in newer versions.
Best Practice:
Regularly check and update your AWS SDK dependencies to the latest versions. Use Maven or Gradle to manage your dependencies efficiently.
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-s3</artifactId>
<version>1.12.100</version> <!-- Always use the latest version -->
</dependency>
Best Practices to Avoid Pitfalls
-
Configuration Management: Use secure methods for managing secrets and configurations.
-
Resource Management: Always free up resources after utilization to improve efficiency.
-
Security Best Practices: Continuously evaluate your security posture and employ IAM roles judiciously.
-
Robust Error Handling: Develop a comprehensive error-handling strategy for all AWS interactions.
-
Stay Updated: Monitor SDK versions and update them regularly to ensure compatibility and security.
My Closing Thoughts on the Matter
Integrating Java applications with AWS can be greatly rewarding, provided that you are mindful of the common pitfalls that can impede progress. By focusing on configuration management, resource allocation, security practices, and error handling, you will not only avoid common mistakes but also create robust, scalable, and secure applications.
For more information on AWS and Java integration, check out the AWS SDK for Java Documentation. Your journey to cloud-native applications begins with careful consideration of these best practices.
Feel free to share your thoughts and experiences with Java and AWS integration in the comments below!