Common Pitfalls When Using OpenCV in Java for Vision Tasks
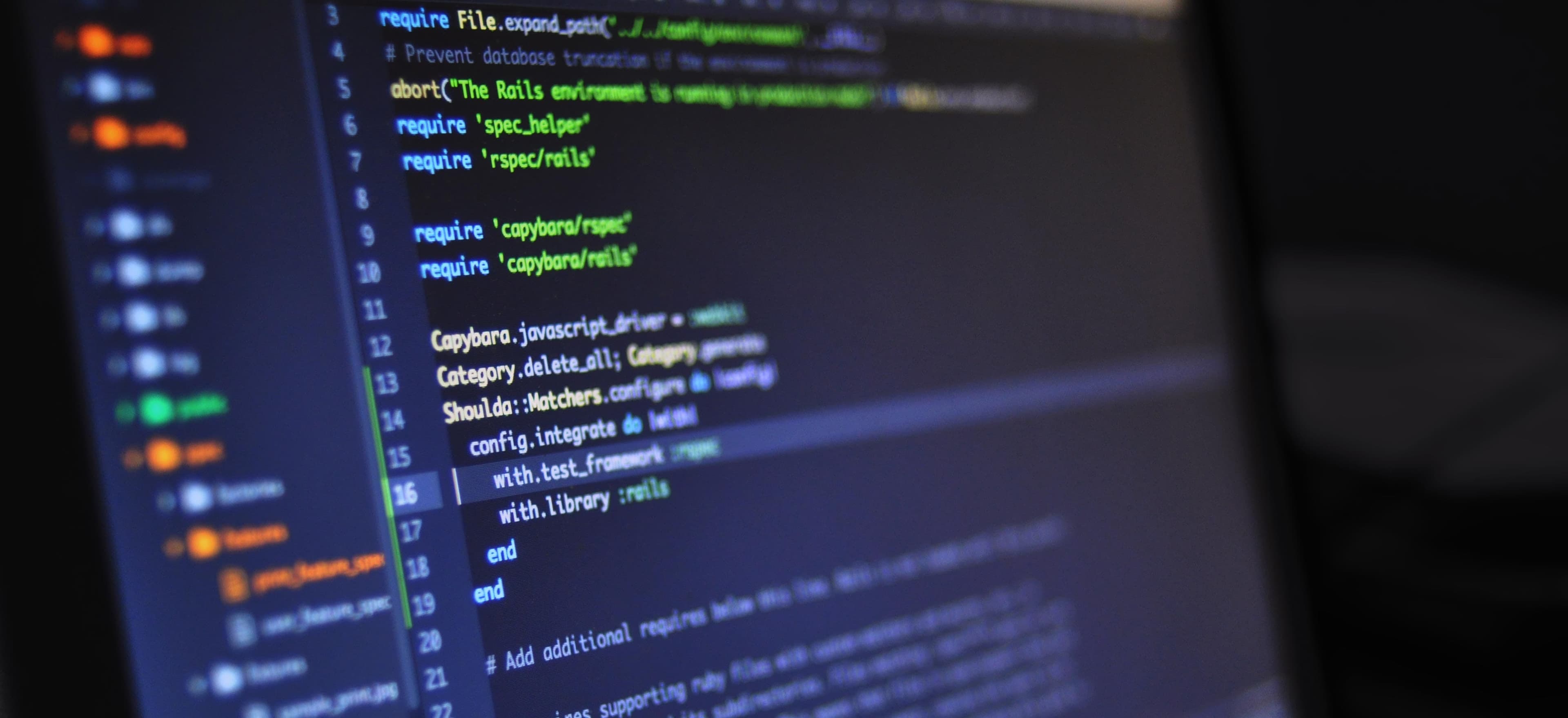
- Published on
Common Pitfalls When Using OpenCV in Java for Vision Tasks
OpenCV, an open-source computer vision and machine learning library, is one of the most popular tools available for computer vision tasks. Although it offers robust functionality, many developers encounter several common pitfalls when using OpenCV in Java. This post aims to illuminate these pitfalls while providing practical solutions and guidelines.
Let's dive into the intricacies of using OpenCV with Java, unpacking common challenges and offering actionable insights.
1. Dependency Management
The Issue
When working with OpenCV in Java, one of the first challenges is managing dependencies. OpenCV, being a native library, has a complicated setup process, especially for beginners. Many developers fail to correctly set the paths for native libraries, leading to frustrating runtime errors.
Solution
To handle dependencies effectively, you can use Maven or Gradle to manage your OpenCV library smoothly. Here's how you can set it up with Maven:
<dependency>
<groupId>org.openpnp</groupId>
<artifactId>opencv</artifactId>
<version>4.5.1-2</version>
</dependency>
To utilize OpenCV, you must also load the native library at runtime. This can be done with the following code snippet:
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
This line of code is imperative. It tells the Java Virtual Machine (JVM) where to find the OpenCV native library files, which are crucial for executing the image processing functions you intend to employ.
2. Incorrect Image Format
The Issue
Processing images incorrectly by assuming their formats can lead to unexpected results. OpenCV supports various image formats, including grayscale and color, but mistakenly handling them may result in confusing errors.
Solution
Before processing your images, it’s best to confirm their format. Here’s how to convert them properly:
Mat src = Imgcodecs.imread("image.jpg");
Mat gray = new Mat();
Imgproc.cvtColor(src, gray, Imgproc.COLOR_BGR2GRAY);
In the code above, we first read an image, which is often in BGR format, and then convert it to grayscale.
The reason for this conversion is straightforward: many algorithms, particularly those involving thresholding or edge detection, perform better and faster using a grayscale format.
3. Memory Management Issues
The Issue
Java developers sometimes forget that OpenCV uses native memory through its C++ backend. This can escalate to significant memory leaks if not managed correctly, particularly when large images are processed in loops.
Solution
A good practice is to explicitly release unused Mat objects as shown below:
Mat image = Imgcodecs.imread("large_image.jpg");
// Process image
image.release(); // Free memory
Manual memory management ensures that the native resources allocated by OpenCV are freed up, preventing potential memory leaks. Be vigilant about releasing Mat objects when they are no longer needed.
4. Misunderstanding Coordinate Systems
The Issue
One common pitfall that remains overlooked by many developers is the difference in coordinate systems. OpenCV uses a (x, y) coordinate framework where (0, 0) is at the top-left corner.
Solution
Using the OpenCV functions like rectangle()
and circle()
, always double-check coordinates. Here’s an example:
Imgproc.rectangle(image, new Point(50, 50), new Point(150, 150), new Scalar(0, 255, 0), 2);
In this code, we draw a rectangle. The points (50, 50)
and (150, 150)
specify the top-left and bottom-right corners, respectively. Understanding this detail can save developers from visualizing incorrect placements on the image.
5. Poor Performance with High-Resolution Images
The Issue
Using high-resolution images may also affect performance. Some algorithms may not be optimized for large resolutions, causing slow processing speeds.
Solution
To avoid performance drops, consider resizing images before processing:
Mat resized = new Mat();
Size size = new Size(image.width() / 2, image.height() / 2);
Imgproc.resize(image, resized, size);
In the above code snippet, we reduce the dimensions of the original image by half. Resizing images can drastically improve performance, especially in real-time applications like video processing, where latency is critical.
6. Neglecting OpenCV's Documentation
The Issue
OpenCV has extensive documentation, yet many developers tend to overlook it. This can lead to incorrect usage of functions and algorithms, resulting in suboptimal code.
Solution
Always refer back to the official OpenCV documentation for guidance, examples, and detailed explanations of functions. Moreover, using forums like Stack Overflow can be valuable to troubleshoot issues you may encounter in your coding journey.
7. Ineffective Debugging
The Issue
Debugging OpenCV-related code can be challenging, especially if errors occur within the native layer. Many developers only rely on Java exceptions, which can obscure the root cause of the problem.
Solution
To implement effective debugging, log relevant information, such as image sizes or interim processing states. Use print statements or logging frameworks like SLF4J to capture essential variables throughout your workflow.
System.out.println("Image Size: " + src.size().width + "x" + src.size().height);
By incorporating logging, you can efficiently trace the flow of your application, ultimately leading to more efficient debugging.
Final Thoughts
While OpenCV is an incredibly powerful library for computer vision tasks in Java, the pitfalls noted above can hinder progress and lead to critical errors. By understanding these issues, you can avoid common mistakes and streamline your image processing tasks.
Make sure to manage your dependencies using Maven or Gradle, correctly handle image formats, practice efficient memory management, comprehend the coordinate system, resize images when necessary, consult the documentation, and effectively debug your applications.
By keeping these recommendations top of mind, you'll navigate OpenCV in Java with more competence and ease, resulting in cleaner, more maintainable code.
For additional insights on leveraging OpenCV for Java applications, check out resources like the OpenCV Java Tutorials or participate in community forums to keep learning. Happy coding!
Checkout our other articles