Common Vaadin Missteps: Avoid These Web App Pitfalls
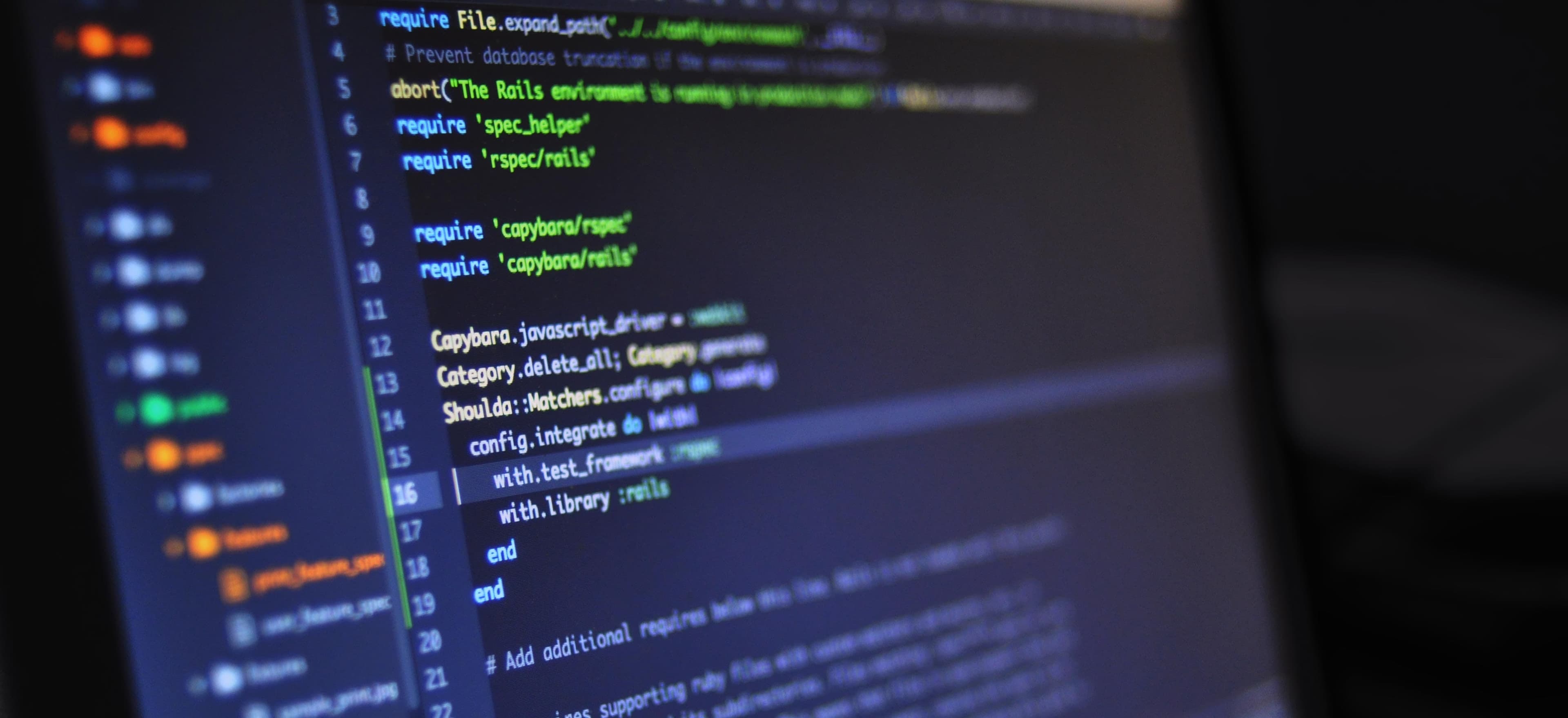
- Published on
Common Vaadin Missteps: Avoid These Web App Pitfalls
Vaadin is a popular framework used for building modern web applications in Java. Its component-based architecture allows developers to create rich, responsive user interfaces with minimal effort. However, even experienced developers can fall into common traps. In this post, we will explore typical missteps in Vaadin development and how to avoid them. By learning from these pitfalls, you can enhance your application's performance and user experience.
1. Ignoring the Component Lifecycle
Why It Matters
Vaadin components have a lifecycle that includes creation, initialization, and destruction phases. Understanding this lifecycle is crucial for efficiently managing resources and enhancing performance.
Common Mistake
Developers often initialize or manipulate components in the wrong lifecycle phase, leading to wasted resources or unexpected behavior.
Example
@Route("")
public class MainView extends VerticalLayout {
public MainView() {
Button button = new Button("Click Me");
button.addClickListener(e -> Notification.show("Button Clicked!"));
add(button);
}
}
In this example, we create a button in the constructor. While this may seem straightforward, if you had complex data binding or initialization code, it might not work as expected since the component is not fully initialized.
Solution
Utilize the @PostConstruct
annotation or extend lifecycle methods to ready your components. This ensures all dependencies are initialized before interaction.
@Component
public class MyComponent extends VerticalLayout {
private final SomeService someService;
@Autowired
public MyComponent(SomeService someService) {
this.someService = someService;
}
@PostConstruct
public void init() {
// Initialize UI components here
}
}
2. Overloading the UI Thread
Why It Matters
Vaadin operates on a single-threaded model. All component updates and interactions happen in the UI thread. Overloading this thread can lead to sluggish user experiences.
Common Mistake
Developers frequently perform long-running tasks on the UI thread, causing the application to freeze.
Example
public void loadData() {
// Long-running database call; DO NOT DO THIS!
List<Data> data = dataService.getData();
updateGrid(data);
}
Solution
Use background threads for long-running processes and then use UI.access()
to update components when complete. This keeps the UI thread responsive:
public void loadData() {
new Thread(() -> {
List<Data> data = dataService.getData();
UI.getCurrent().access(() -> updateGrid(data));
}).start();
}
3. Lack of Proper Error Handling
Why It Matters
In any web application, errors are inevitable. Proper error handling not only prevents undesirable crashes but also provides a better user experience.
Common Mistake
Developers often ignore exceptions or fail to provide user-friendly feedback when something goes wrong.
Example
try {
someService.doSomething();
} catch (Exception e) {
// Error ignored
}
Solution
Implement a centralized error handling mechanism by using @ErrorHandler
:
@ErrorHandler
public class CustomErrorHandler implements ErrorHandler {
@Override
public void error(Event event) {
// Log the error and show a friendly message to the user
Notification.show("An error occurred: " + event.getThrowable().getMessage());
}
}
This approach simplifies your code and maintains a consistent error-handling strategy across the application.
4. Neglecting Responsive Design
Why It Matters
The world is increasingly mobile-first. If your Vaadin application does not adapt to different screen sizes, you risk alienating users.
Common Mistake
Developers often build applications that look perfect on desktop but fail miserably on mobile devices.
Solution
Leverage Vaadin’s built-in support for responsive layouts. Use features like CSS Grid or Flexbox to create flexible designs.
Example
VerticalLayout layout = new VerticalLayout();
layout.setSizeFull();
layout.add(new Text("Responsive Text"));
Also, consider using custom CSS:
@media (max-width: 600px) {
.responsive-text {
font-size: 14px;
}
}
5. Underutilizing Vaadin Features
Why It Matters
Vaadin provides many out-of-the-box features like data binding, validation, and navigation. Many developers fail to leverage these strengths, often reinventing the wheel.
Common Mistake
Opting for manual handling of tasks already managed by Vaadin.
Example
Implementing validation manually rather than using Vaadin's built-in validators.
TextField filter = new TextField();
filter.addValueChangeListener(event -> {
if (!event.getValue().matches("[A-Za-z]*")) {
Notification.show("Invalid input!");
}
});
Solution
Utilize the validators provided by Vaadin:
filter.setRequiredIndicatorVisible(true);
filter.addValidator(new RegexpValidator("[A-Za-z]*", "Invalid input!"));
This simplifies your code and maintains consistency throughout your application.
6. Poor State Management
Why It Matters
Maintaining application state is crucial in web applications, especially those using Vaadin’s server-side architecture. Poor state management can result in a confusing user experience.
Common Mistake
Failing to preserve or manage user state effectively.
Solution
Utilize Vaadin's built-in sessions or HTTP sessions to manage user-specific state:
VaadinSession.getCurrent().setAttribute("myData", myData);
Retrieve this attribute later as needed:
MyData myData = (MyData) VaadinSession.getCurrent().getAttribute("myData");
The Last Word
Building applications with Vaadin can facilitate a smooth and efficient development experience if done correctly. By being mindful of common missteps, you can avoid pitfalls and improve the quality and maintainability of your application.
Fostering a habit of understanding the framework's capabilities, embracing best practices, and implementing a robust error and state management strategy will help you build high-quality applications that stand the test of time.
For further reading and resources, check Vaadin's official documentation and explore community tutorials to deepen your understanding of this vibrant framework.
Additional Resources
- Vaadin Extensions - Enhance your application with community-built components.
- Vaadin DevTalk - Join the conversation and get insights directly from other developers.
By steering clear of these pitfalls, you’re on your way to creating top-notch Vaadin applications that not only impress users but also stand the test of evolving web standards. Happy coding!
Checkout our other articles