Top 5 Java Vulnerabilities and How to Fix Them
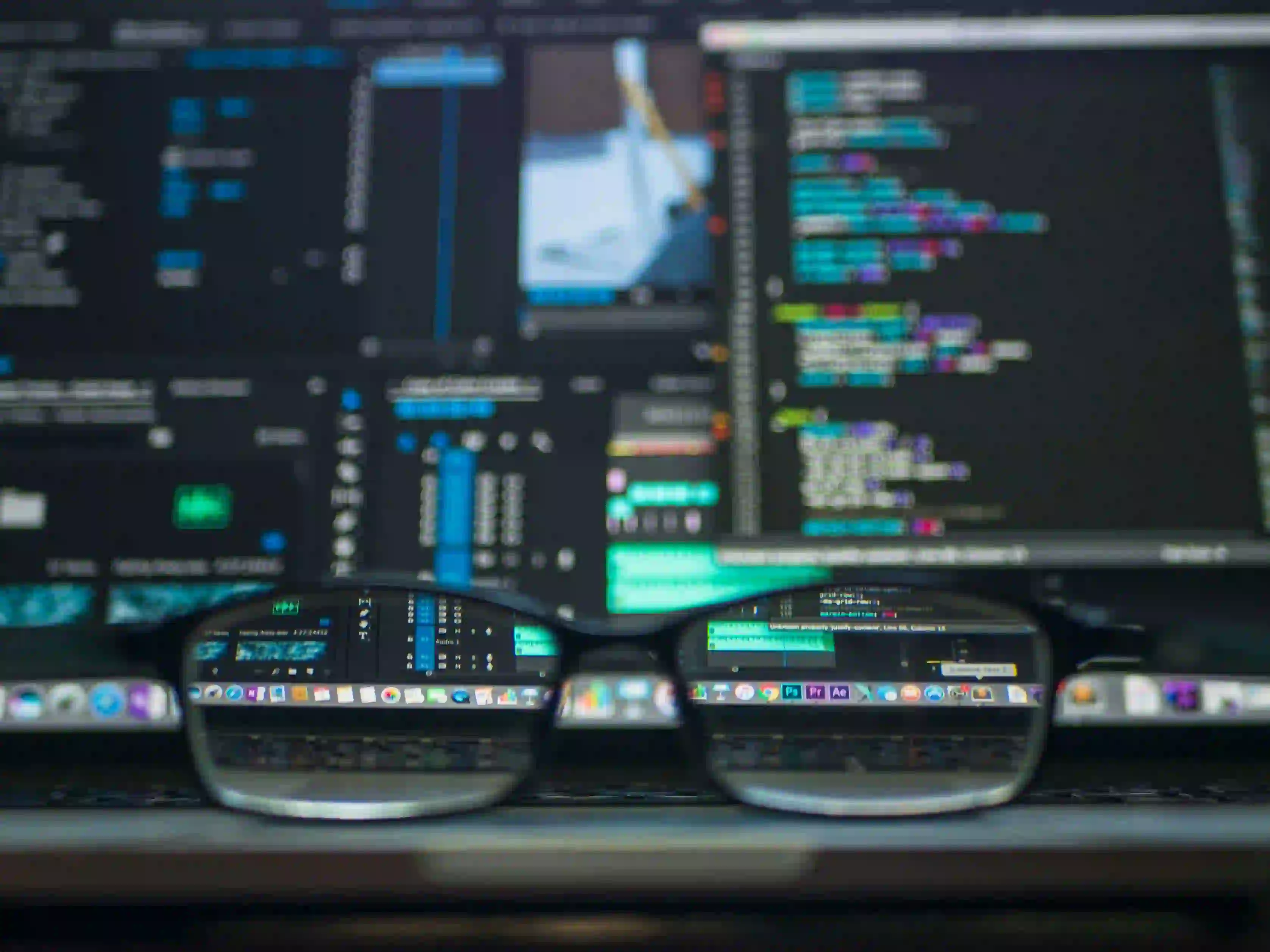
Top 5 Java Vulnerabilities and How to Fix Them
Java is one of the most widely used programming languages today. Its portability, security features, and rich ecosystem make it a favorite among developers for building robust applications. However, like any technology, Java is not immune to vulnerabilities. Understanding these weaknesses is crucial for developers who aim to create secure applications. In this blog post, we will discuss the top five vulnerabilities in Java and suggest effective ways to mitigate them.
Why Security in Java Matters
Java applications are often targets for cyber attacks due to their widespread use, from web applications to enterprise software. According to the OWASP Top Ten, Java projects are particularly vulnerable to security threats. Hence, understanding and fixing these vulnerabilities is not just an option but a necessity for any serious developer.
Vulnerability #1: SQL Injection
What is SQL Injection?
SQL Injection occurs when an attacker executes arbitrary SQL code on a database, usually through input fields in an application. This can allow them to read sensitive information, manipulate or delete data, and even take full control of the database.
Code Example
Here's an example of a vulnerable piece of code:
String query = "SELECT * FROM users WHERE username = '" + username + "' AND password = '" + password + "'";
Why It's Vulnerable
In this code, user inputs directly concatenate into a SQL query without any form of validation or sanitization. This leaves the door wide open for SQL Injection attacks.
How to Fix
To prevent SQL Injection, always use Prepared Statements. Here’s how:
String query = "SELECT * FROM users WHERE username = ? AND password = ?";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, username);
preparedStatement.setString(2, password);
ResultSet resultSet = preparedStatement.executeQuery();
Why This Works
Prepared statements pre-compile the SQL query, thus separating SQL code from user input. This method prevents attackers from manipulating queries, effectively diminishing the risk of SQL injection.
Vulnerability #2: Insecure Deserialization
What is Insecure Deserialization?
Insecure deserialization happens when untrusted data is used to create objects in a Java application. Attackers can send malformed data to a server, leading to code execution, data tampering, or denial of service.
Code Example
Consider this deserialization code:
ObjectInputStream in = new ObjectInputStream(inputStream);
MyObject obj = (MyObject) in.readObject();
Why It's Vulnerable
If the input stream is controlled by an attacker, they can send malicious serialized objects that can exploit your application.
How to Fix
To secure your application against insecure deserialization, do not blindly deserialize objects. Here’s a safer approach:
if (inputStream instanceof ByteArrayInputStream) {
ObjectInputStream in = new ObjectInputStream(inputStream);
Object obj = in.readObject();
if (obj instanceof MyObject) {
MyObject myObj = (MyObject) obj;
// Process myObj
}
}
Why This Works
By checking the type of the object being deserialized, you add a layer of authentication against potentially mischievous input. You should also consider using non-serialized formats like JSON or XML unless you absolutely need to use Java serialization.
Vulnerability #3: Cross-Site Scripting (XSS)
What is XSS?
XSS is a vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. While Java is primarily a backend language, when it interacts with web technologies, XSS can still be a significant threat.
Code Example
Here’s an example of code that could be vulnerable to XSS:
response.getWriter().println("<h1>" + userInput + "</h1>");
Why It's Vulnerable
This code directly renders user input without validating or escaping it, which allows an attacker to inject scripts.
How to Fix
Always sanitize and escape user inputs:
String safeInput = StringEscapeUtils.escapeHtml4(userInput);
response.getWriter().println("<h1>" + safeInput + "</h1>");
Why This Works
Using libraries like Apache Commons Text's StringEscapeUtils
ensures that any HTML tags in the input are converted to escape characters, thus preventing scripts from executing.
Vulnerability #4: Misconfiguration of Security Settings
What is Misconfiguration?
Misconfiguration refers to the failure to properly configure security controls, which can lead to exposure of sensitive data or vulnerabilities within an application.
Code Example
An example of misconfigured security might look like this:
public class SecurityConfig {
public boolean isDebugEnabled() {
return true; // Debugging enabled
}
}
Why It's Vulnerable
Having debugging enabled in production code can reveal sensitive information about your application.
How to Fix
Always set configurations based on the environment. For example, utilize properties files to manage different settings.
public class SecurityConfig {
private boolean debugEnabled = Boolean.parseBoolean(System.getProperty("debug", "false"));
public boolean isDebugEnabled() {
return debugEnabled;
}
}
Why This Works
By using system properties, you can change settings according to the deployment environment, preventing sensitive data from being exposed unintentionally in production.
Vulnerability #5: Weak Password Management
What is Weak Password Management?
Weak password management occurs when an application uses insecure methods for storing and validating user passwords. This vulnerability makes it easier for attackers to crack user accounts.
Code Example
Here's a problematic method of storing passwords:
String hashedPassword = userInput.getPassword();
saveToDatabase(username, hashedPassword);
Why It's Vulnerable
Storing passwords as plain text or using weak hashing algorithms makes them susceptible to attacks like brute force or dictionary attacks.
How to Fix
Always use a strong hashing algorithm such as BCrypt:
String hashedPassword = BCrypt.hashpw(userInput.getPassword(), BCrypt.gensalt());
saveToDatabase(username, hashedPassword);
Why This Works
BCrypt is designed specifically for password hashing. It incorporates salting and adaptive hashing, making it significantly harder to crack.
Final Thoughts
Java security vulnerabilities can have dire consequences if left unaddressed. By understanding the common vulnerabilities and adopting best practices for mitigation, developers can ensure their applications remain secure.
From SQL Injection through prepared statements to Weak Password Management using BCyrpt, every step taken in securing Java applications aids in safeguarding user data and enhancing overall integrity.
Remember, security is a continuous process, not a one-time task. Regularly update your skills and keep abreast of the latest security standards to develop robust and secure applications.
For more resources on Java security best practices, consider exploring Java Security Documentation or participating in courses on security software development.
By keeping these vulnerabilities in mind, you can contribute significantly to the security of your Java applications, ensuring a safer experience for users and developers alike.