Common Pitfalls When Implementing WebSockets in Java
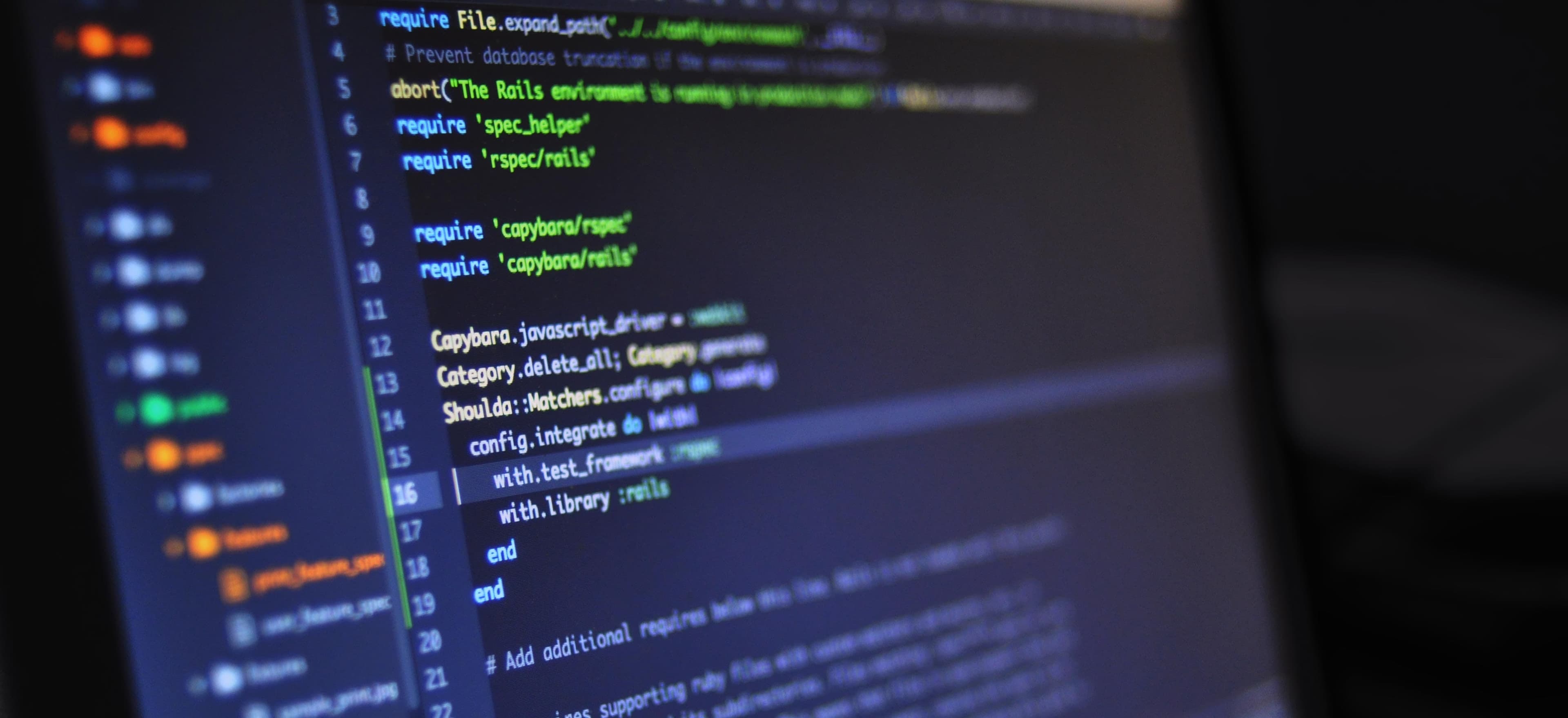
- Published on
Common Pitfalls When Implementing WebSockets in Java
WebSockets have revolutionized the way we build real-time web applications by providing a persistent connection between the client and server. While they offer numerous advantages such as reduced latency and reduced overhead compared to traditional HTTP requests, implementing WebSockets in Java is not without its challenges. In this blog post, we’ll explore some common pitfalls you may encounter and how to effectively avoid them.
1. Server Configuration Issues
Problem
One of the first hurdles developers encounter is server configuration. Many Java application servers, such as Tomcat, Jetty, or GlassFish, require specific configurations to support WebSocket protocols effectively. This may lead to unexpected behavior or performance degradation.
Solution
Always ensure that your server is properly set up to handle WebSockets. When using a server like Tomcat, for instance, you need to be running version 7.0.47 or higher for WebSocket support. Here’s a simple Java class for a WebSocket endpoint:
import javax.websocket.OnMessage;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/websocket")
public class MyWebSocket {
@OnMessage
public void onMessage(String message, Session session) {
// Echo the received message back to the client
try {
session.getBasicRemote().sendText("Server received: " + message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why? This snippet demonstrates how you can set up a simple WebSocket that echoes back messages to the client. Ensure you check your server's documentation for any specific configuration needs.
2. Not Handling Connection Lifecycle Properly
Problem
Handling the connection lifecycle incorrectly can lead to memory leaks or unhandled exceptions. For instance, forgetting to close sessions or manage user states can clutter your application’s memory.
Solution
Ensure that you implement methods to handle the session lifecycle correctly. Here’s an enhanced version of the earlier example:
import javax.websocket.OnClose;
import javax.websocket.OnError;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/websocket")
public class MyWebSocket {
@OnOpen
public void onOpen(Session session) {
System.out.println("New connection: " + session.getId());
}
@OnClose
public void onClose(Session session) {
System.out.println("Closed connection: " + session.getId());
// Perform any cleanup here
}
@OnError
public void onError(Session session, Throwable throwable) {
System.err.println("Error on session: " + session.getId() + " - " + throwable.getMessage());
}
@OnMessage
public void onMessage(String message, Session session) {
try {
session.getBasicRemote().sendText("Server received: " + message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why? This code now logs open and close events, allowing you to track the session lifecycle effectively and cleanly manage resources.
3. Poor Scalability Approach
Problem
Scalability is a significant concern when implementing WebSockets. Relying on in-memory data to track active connections can lead to complications when scaling your application across multiple nodes.
Solution
Consider using a Redis-backed pub/sub mechanism or similar solutions to manage session states. This ensures that all instances of your application can communicate effectively.
Here’s a simple example of how to publish messages in a Redis setup:
import redis.clients.jedis.Jedis;
public class RedisPublisher {
private Jedis jedis;
public RedisPublisher(String host, int port) {
jedis = new Jedis(host, port);
}
public void publish(String channel, String message) {
jedis.publish(channel, message);
}
}
Why? This enables you to manage messages across multiple instances of your WebSocket server, thus maintaining performance as your application grows.
4. Neglecting Security Measures
Problem
WebSockets can be vulnerable to various attacks if not adequately secured. Attack vectors such as Cross-Site WebSocket Hijacking (CSWSH) or Man-in-the-Middle (MitM) attacks can compromise user data.
Solution
Implement strong security measures such as:
- Use the
wss://
protocol to encrypt WebSocket traffic. - Verify origins to prevent unauthorized connections.
- Authenticate users before establishing WebSocket connections.
Here’s an example of how you might enforce origin checks:
import javax.websocket.server.ServerEndpoint;
import javax.websocket.server.HandshakeRequest;
import javax.websocket.server.server.EndpointConfig;
@ServerEndpoint(value = "/websocket", configurator = MyEndpointConfigurator.class)
public class MyWebSocket {
// Existing methods ...
public static class MyEndpointConfigurator extends ServerEndpointConfig.Configurator {
@Override
public void beforeRequest(Map<String, List<String>> headers) {
String origin = headers.get("Origin").get(0);
// Implement your origin check logic here
if (!isValidOrigin(origin)) {
throw new RuntimeException("Invalid Origin");
}
}
}
private static boolean isValidOrigin(String origin) {
// Add logic to validate the origin
return true; // example placeholder
}
}
Why? The above code showcases how to enforce origin checks, thereby adding a layer of security to your WebSocket implementation.
5. Ignoring Protocol Compatibility
Problem
Compatibility issues arise when clients use different versions of WebSocket protocols or when you combine WebSocket with other technologies not designed to work seamlessly with real-time applications.
Solution
Ensure that your WebSocket implementation adheres to the current RFC 6455 protocol. Use libraries that handle cross-browser compatibility issues, like Jetty or Tyrus.
Additionally, testing is key. Use tools like SocketTest to validate your WebSocket server under real conditions.
6. Inadequate Error Handling
Problem
WebSocket connections can drop unexpectedly due to a variety of reasons, including network issues or server restarts. Inadequate error handling can lead to confusion and frustration for end users.
Solution
Implement reconnection logic on the client side. Below is a simple JavaScript example that tries to reconnect when a connection is lost:
function connect() {
let socket = new WebSocket("wss://yourserver.com/websocket");
socket.onopen = function() {
console.log("Connected to server");
};
socket.onclose = function(event) {
console.error("Connection closed, attempting to reconnect...");
setTimeout(connect, 1000); // Try to reconnect after 1 second
};
socket.onerror = function(error) {
console.error("WebSocket error: " + error.message);
};
socket.onmessage = function(event) {
console.log("Message from server: " + event.data);
};
}
connect();
Why? This script ensures a robust connection strategy, aiming to maintain user experience seamlessly even in the event of connection loss.
Lessons Learned
Implementing WebSockets in Java can bring significant benefits to your real-time applications, but it's crucial to be aware of common pitfalls. By addressing server configuration, connection lifecycle management, scalability, security, protocol compatibility, and error handling, you can create a robust WebSocket application.
For further reading, consider checking out these resources:
By staying informed and proactive, you’ll be well on your way to mastering WebSockets in Java! Happy coding!