Common Pitfalls When Implementing OAuth 2.0 in Java Apps
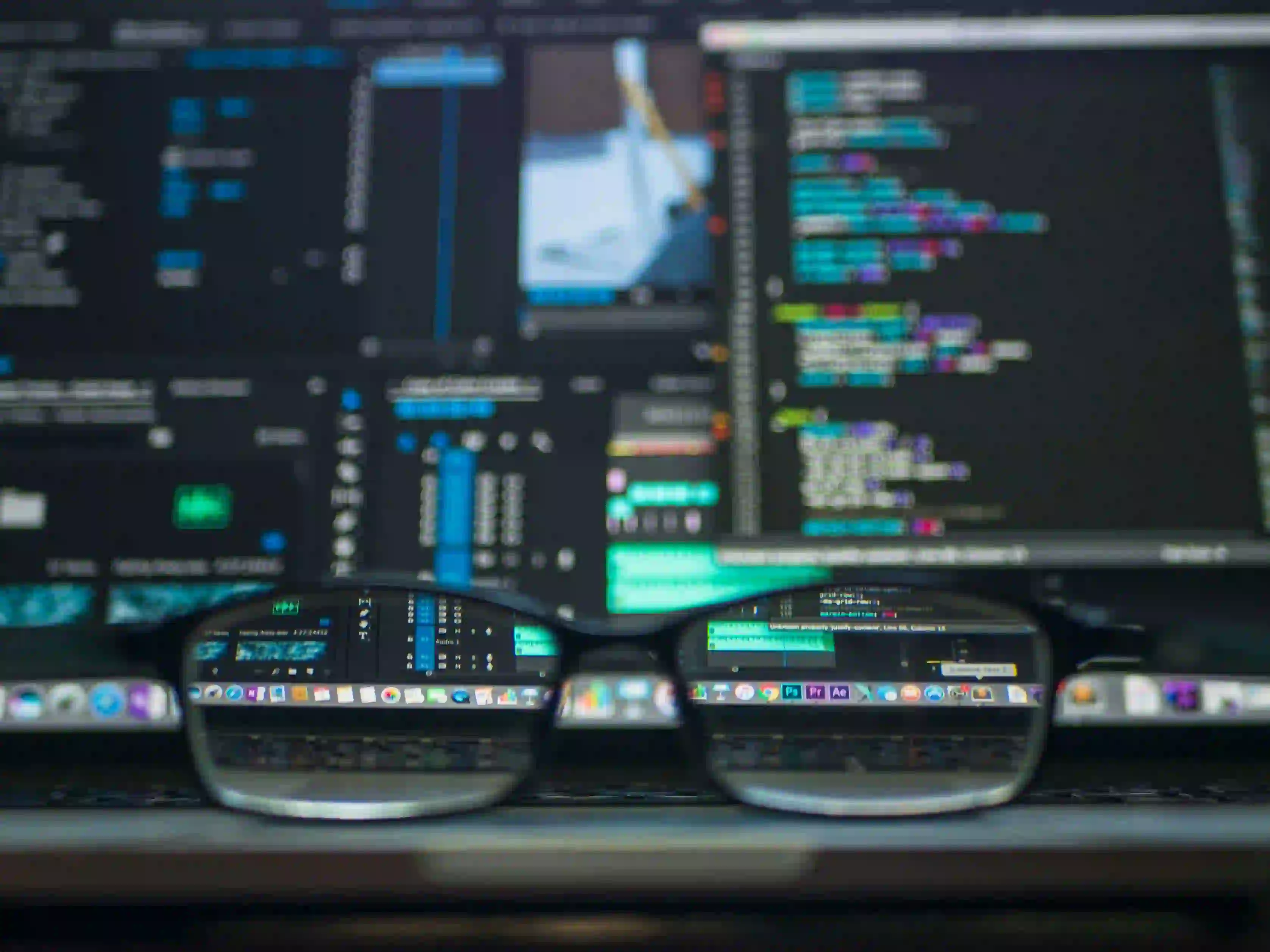
Common Pitfalls When Implementing OAuth 2.0 in Java Apps
OAuth 2.0 has revolutionized how web applications manage authentication and authorization. By allowing users to grant third-party apps limited access to their data without revealing passwords, it enhances security and user experience. However, implementing OAuth 2.0 in Java applications isn't without challenges. This blog post highlights common pitfalls developers encounter and offers best practices for navigating these issues effectively.
Understanding OAuth 2.0
Before diving into common pitfalls, it is crucial to understand what OAuth 2.0 is. According to OAuth.net, OAuth 2.0 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service. It consists of four main roles:
- Resource Owner: Typically, the end-user who owns the data.
- Client: The application that wants to access the user’s data.
- Authorization Server: The server responsible for authenticating the user and issuing access tokens.
- Resource Server: The server hosting the user's data.
Using these roles, OAuth 2.0 creates a secure mechanism for granting access that minimizes security risks.
Common Pitfalls
1. Poorly Configured Redirect URIs
Issue: One of the most critical aspects of OAuth 2.0 is defining redirect URIs correctly. A misconfigured URI can lead to redirection vulnerabilities, which can be exploited by attackers.
Solution: Ensure that redirect URIs match exactly with the registered URIs on the Authorization Server. Use absolute URIs and avoid using wildcard URIs to restrict access.
String redirectUri = "https://yourapp.com/callback";
// Validate URI
if (!redirectUri.equals(registeredRedirectUri)) {
throw new IllegalArgumentException("Invalid redirect URI");
}
2. Failing to Validate State Parameter
Issue: The state parameter is a critical security feature that helps prevent CSRF (Cross-Site Request Forgery) attacks. Not validating this parameter can allow an attacker to exploit your application.
Solution: Always generate and validate a unique state for each OAuth request.
String state = UUID.randomUUID().toString(); // Generate unique state
// Store the state in session for later validation
// After redirect
if (!state.equals(request.getParameter("state"))) {
throw new SecurityException("Invalid state parameter");
}
3. Ignoring Token Expiration
Issue: OAuth 2.0 access tokens are meant to have limited lifespans. Ignoring token expiration can compromise your application's security.
Solution: Implement a token renewal strategy and handle expired tokens gracefully.
if (isTokenExpired(accessToken)) {
accessToken = refreshAccessToken(refreshToken);
}
Utilizing refresh tokens is essential for maintaining a seamless user experience without requiring them to log in repeatedly. For more detail on token management, refer to the OAuth 2.0 documentation.
4. Storing Token Info Insecurely
Issue: Developers may store sensitive token information in insecure locations (e.g., local storage or memory). This can lead to token theft and unauthorized access.
Solution: Always store tokens securely. For server-side applications, consider utilizing database encryption or secure environments to safeguard sensitive information.
// Example of secure storage
String encryptedToken = encrypt(token);
database.saveEncryptedToken(userId, encryptedToken);
5. Overreliance on Third-Party Libraries
Issue: While third-party libraries can streamline OAuth implementation, overreliance can lead to issues if libraries become outdated or insecure.
Solution: Review library dependencies regularly. Understand how they work and ensure they are kept up-to-date. It can also be beneficial to write tests around these libraries to verify their expected behavior.
// Example of using a reliable library
OAuth2AccessToken token = oAuth2Client.getAccessToken();
// Always handle exceptions and validate results
6. Failing to Implement Proper Scopes
Issue: OAuth 2.0 allows developers to request specific permissions (scopes) from users. Insufficient scoping can lead to over-privileged access.
Solution: Define and request the least amount of permissions necessary for your application to function. This reduces risk and enhances user trust.
// Requesting scopes during authorization
String scopes = "read write"; // Define required scopes
7. Neglecting Error Handling
Issue: Lack of proper error handling can lead to poor user experience and security vulnerabilities. Uncaught errors can expose sensitive information about your application.
Solution: Implement robust error handling and provide meaningful user feedback to users about failures.
try {
// OAuth process
} catch (OAuthException e) {
log.error("OAuth error: " + e.getMessage());
// Show friendly error message to the user
}
A Final Look
Implementing OAuth 2.0 in Java applications is a powerful way to enhance security and user experience but requires careful attention to detail. By being aware of common pitfalls such as poorly configured redirect URIs, inadequate state parameter validation, and insecure token management, you can build a robust application that users can trust. Remember, security isn't a checkbox; it requires continuous monitoring and improvement.
For more advanced techniques and topics, consider reviewing the Spring Security documentation or exploring the broader Java security landscape. By leveraging OAuth 2.0 effectively, you can ensure your Java application remains secure and user-friendly.
Feel free to ask questions or share your experiences with OAuth 2.0 in the comments below!