Mastering Apache Spark: Common Java Pitfalls to Avoid
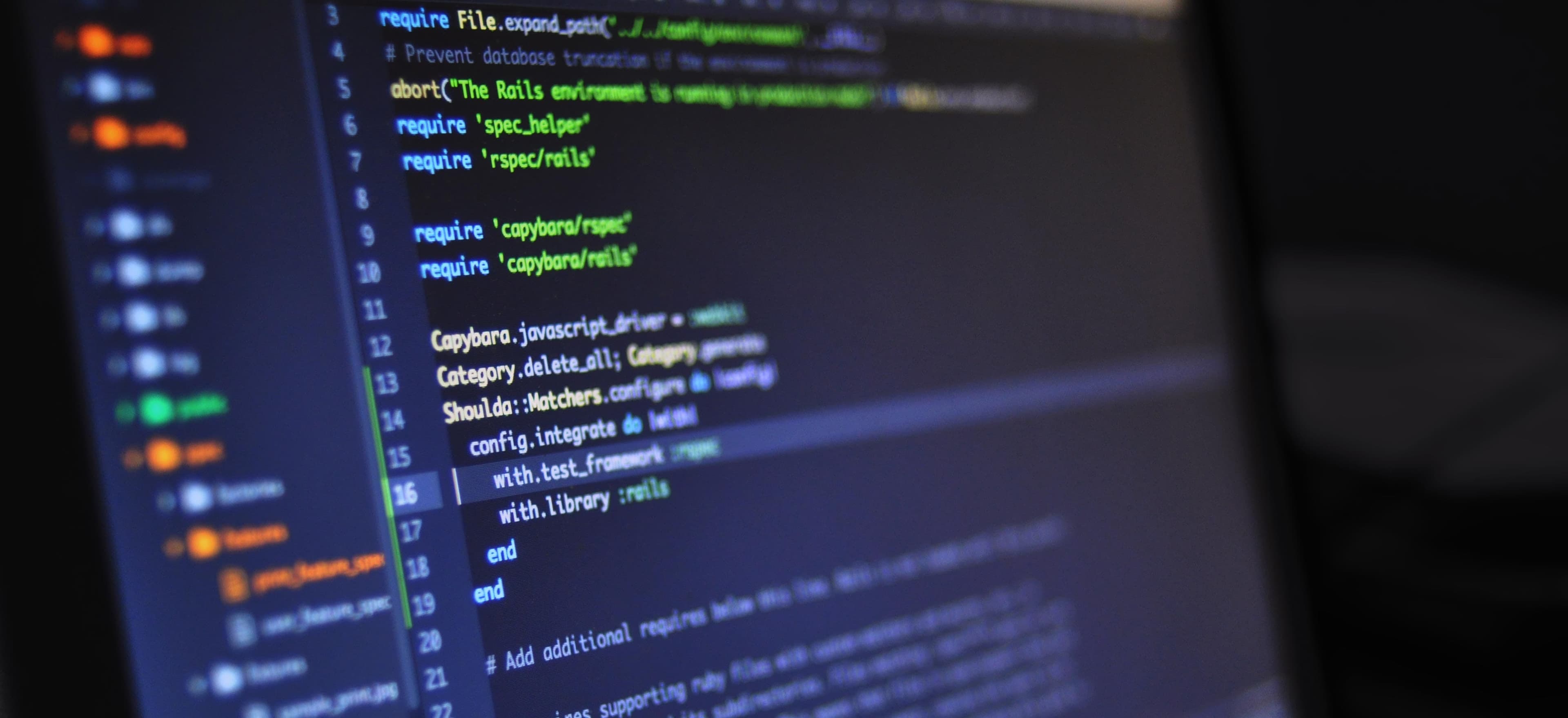
- Published on
Mastering Apache Spark: Common Java Pitfalls to Avoid
Apache Spark is a powerhouse for big data processing, enabling users to conduct fast data analysis and a myriad of ETL tasks. However, when utilizing Spark with Java, there are some common pitfalls developers can easily stumble into. This blog will explore these pitfalls and provide solutions, keeping you on the path to efficient and error-free Spark applications.
Understanding Apache Spark and Its Importance
Apache Spark is a unified analytics engine designed for large-scale data processing. With its ability to perform in-memory computations, Spark accelerates processing times significantly compared to traditional disk-based processing. Java, being one of Spark's native languages, allows developers to seamlessly integrate Spark's capabilities into their Java applications.
For a great introduction to what Spark can do, refer to Apache Spark - Official Documentation.
Common Pitfalls When Using Apache Spark with Java
1. Poor Dataset Design
Pitfall: Ignoring the importance of partitioning can lead to inefficient data processing.
A poorly designed dataset can result in data skew, where one partition has significantly more data than others. This, in turn, can cause performance bottlenecks.
Solution: Ensure optimal partitioning of datasets. When loading data into Spark, consider using specific partitioning strategies to evenly distribute the workload.
JavaSparkContext sparkContext = new JavaSparkContext(sparkConf);
JavaRDD<String> lines = sparkContext.textFile("hdfs://path/to/large_file.txt");
// Repartition the RDD for better performance
JavaRDD<String> repartitionedLines = lines.repartition(100); // Adjust the number based on your cluster
Why: Using repartition()
helps in evenly distributing the data across the partitions, improving performance during transformations and actions.
2. Ineffective Use of Caching
Pitfall: Failing to cache or incorrectly caching datasets.
Developers sometimes forget to cache recurrently accessed RDDs, leading to unnecessary data recomputation. On the other hand, caching too many datasets might lead to excessive memory usage.
Solution: Use caching smartly.
JavaRDD<String> cachedData = lines.cache(); // Cache the RDD for better performance
long count = cachedData.count(); // Count will use the cached result.
Why: The .cache()
method stores the RDD in memory, so future actions don't require recomputation from scratch, significantly improving performance.
3. Not Utilizing Spark SQL
Pitfall: Sticking to RDDs when you could simplify your code with DataFrames or SQL queries.
RDDs provide more granular control but can lead to more complex and verbose code. Utilizing Spark SQL provides a high-level API that is easier to use and understand.
Solution: Utilize DataFrame
and Dataset
APIs for better performance and simplicity.
// Creating a DataFrame from an existing RDD
Dataset<Row> df = sparkContext.read().json("hdfs://path/to/json_file");
// Running SQL queries on DataFrames
df.createOrReplaceTempView("table");
Dataset<Row> result = sparkContext.sql("SELECT * FROM table WHERE age > 30");
Why: The DataFrame API is optimized for performance and allows for complex queries to be written in a concise manner, enhancing code maintainability.
4. Not Handling Serialization Issues
Pitfall: Overlooking serialization when passing objects across the Spark cluster can lead to runtime exceptions.
When Spark needs to send data between nodes, it serializes Java objects. If the objects are not serializable, your application will throw an exception.
Solution: Ensure that all classes used within RDDs or DataFrames implement Serializable
.
public class MySerializableClass implements Serializable {
private String data;
public MySerializableClass(String data) {
this.data = data;
}
// getters and other methods
}
Why: Implementing Serializable
on classes ensures Spark can serialize and send them without failing during distribution across the cluster.
5. Ignoring Error Handling
Pitfall: Neglecting error handling can result in failures that crash the entire Spark job.
Not anticipating potential failures may leave your Spark applications vulnerable. Failure to manage exceptions can lead to lost jobs and wasted computational resources.
Solution: Implement comprehensive error handling and checkpoints.
try {
long result = repartitionedLines.map(line -> {
// Processing code here
return process(line);
}).reduce((a, b) -> a + b);
} catch (Exception e) {
System.err.println("Error processing data: " + e.getMessage());
}
Why: By wrapping functional code inside a try-catch block, you ensure that specific errors can be caught and handled, significantly improving the robustness of your application.
6. Configuring Spark Properties Inefficiently
Pitfall: Using defaults or configuring Spark properties without understanding their impact can lead to suboptimal performance.
Spark provides numerous configuration settings that influence memory usage, shuffle behavior, and overall execution efficiency.
Solution: Review and adjust Spark properties based on your workload.
SparkConf config = new SparkConf()
.set("spark.executor.memory", "4g")
.set("spark.driver.memory", "2g")
.set("spark.sql.shuffle.partitions", "200");
JavaSparkContext sparkContext = new JavaSparkContext(config);
Why: Customizing Spark configurations to fit your workload helps exploit available resources, leading to better computations.
A Final Look: Avoiding the Java Pitfalls in Spark
Mastering Apache Spark with Java involves understanding both the API and best practices. By avoiding these common pitfalls—such as poor dataset design, ineffective caching, and neglecting serialization—you can enhance the performance and reliability of your Spark applications.
Always keep learning and exploring the features of Apache Spark. Don’t hesitate to delve deeper into Spark's capabilities, explore its ecosystem, and leverage its extensive documentation found on the Apache Spark Documentation.
By implementing the strategies discussed in this blog, you will enhance your efficiency and control within your Apache Spark workflows, ultimately leading to more effective data processing applications. Happy coding!