Mastering Event-Driven Microservices: Common Pitfalls to Avoid
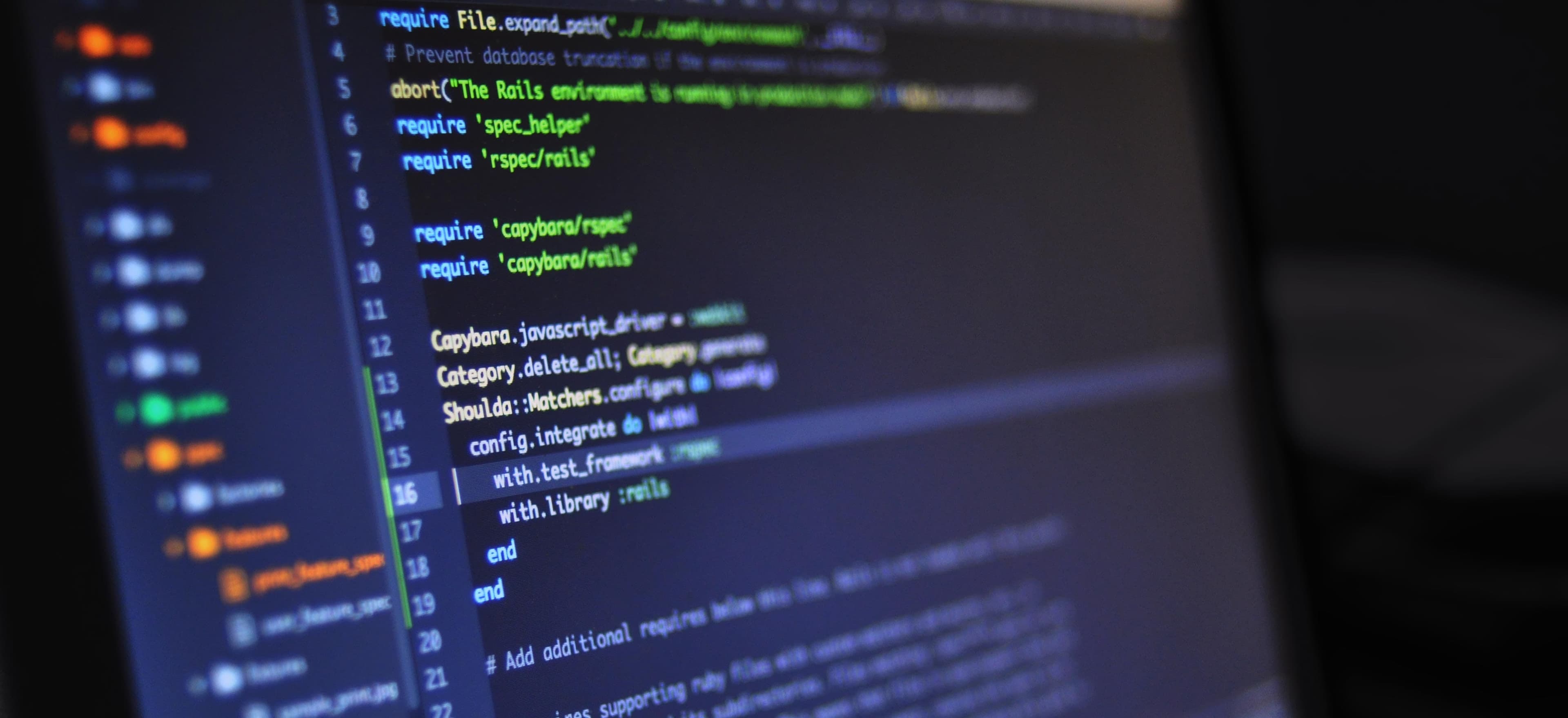
- Published on
Mastering Event-Driven Microservices: Common Pitfalls to Avoid
The rise of microservices has transformed how we design and build software systems. Among the architectural styles emerging from this evolution, event-driven microservices have gained particular prominence. This approach embraces the power of events to notify components of an application about changes in the state, allowing them to react accordingly. However, building an effective event-driven architecture comes with its own set of challenges. In this blog post, we will discuss common pitfalls to avoid when developing event-driven microservices, along with valuable insights and code examples to help you on your journey.
What are Event-Driven Microservices?
Event-driven microservices use events as the driving force for communication between different microservice components. Each service listens for specific events and reacts, allowing for a decoupled system where individual microservices can evolve independently.
Why Choose an Event-Driven Architecture?
- Scalability: Event-driven microservices scale better since services are decoupled and can be developed and deployed independently.
- Resilience: Systems become more fault-tolerant. If one service fails, others can continue to operate, handling events when the service recovers.
- Flexibility: New features can be added with minimal disruption to existing services by introducing new event handlers.
Common Pitfalls to Avoid
1. Overcomplicating the Event Model
Issue: One of the primary challenges in event-driven systems is designing an event model that is simple yet sufficiently expressive.
Tip: Stick to the principle of keeping events simple and focused. Each event should represent a single outcome or change in state.
Example:
public class UserRegisteredEvent {
private String userId;
private String email;
private LocalDate registrationDate;
public UserRegisteredEvent(String userId, String email, LocalDate registrationDate) {
this.userId = userId;
this.email = email;
this.registrationDate = registrationDate;
}
// Getters and additional methods...
}
Here, the UserRegisteredEvent
focuses solely on the registration action. Avoid merging unrelated events into a single model; this leads to confusion and can complicate event processing logic.
2. Ignoring Event Schema Evolution
Issue: The need for changes in event structures will arise as your application evolves. Ignoring schema evolution can lead to difficulties in data compatibility.
Tip: Utilize techniques such as versioning events, backwards compatibility, and event processing libraries to manage changes gracefully.
Example of Versioning:
public class UserRegisteredEventV1 {
private String userId;
private String email;
public UserRegisteredEventV1(String userId, String email) {
this.userId = userId;
this.email = email;
}
}
public class UserRegisteredEventV2 extends UserRegisteredEventV1 {
private LocalDate registrationDate;
public UserRegisteredEventV2(String userId, String email, LocalDate registrationDate) {
super(userId, email);
this.registrationDate = registrationDate;
}
}
Versioning helps in maintaining compatibility. Consumers of the event can choose which version they wish to handle without breaking existing functionality.
3. Not Handling Event Ordering
Issue: Frequently, microservices need to process events in a specific order, especially when the events are interdependent.
Tip: Implement a sequence or partitioning logic based on your use case to manage order effectively. Using message brokers that support ordered delivery can also help.
Code Snippet for Ordering:
@KafkaListener(topics = "user-events", groupId = "user-handler")
public void listen(ConsumerRecord<String, UserRegisteredEvent> record) {
// Processing the event while maintaining order
processEvent(record.value());
}
This code leverages Kafka's partitioning features. Events in the same partition will be processed in the order they arrive, reducing the risk of out-of-order processing.
4. Neglecting Security
Issue: Security is a crucial aspect often overlooked, especially with multiple services interacting over events.
Tip: Implement robust authentication and authorization mechanisms for event producers and consumers. Consider using signed events to validate their origin.
Security Implementation Example:
public class SecureEventProducer {
private String secretKey;
public SecureEventProducer(String secretKey) {
this.secretKey = secretKey;
}
public String signEvent(String eventJson) {
// Sign the event
return HmacUtils.hmacSha256Hex(secretKey, eventJson);
}
}
This code implements a simple signing mechanism for events. By signing events, you ensure their integrity, and consumers can verify that they haven't been tampered with.
5. Underestimating Monitoring and Logging
Issue: Events can be lost or mishandled, leading to silent failures that are difficult to diagnose.
Tip: Implement thorough monitoring and logging mechanisms to track both successful and failed event flows.
Effective Logging Example:
@KafkaListener(topics = "user-events", groupId = "user-handler")
public void listen(UserRegisteredEvent event) {
try {
processEvent(event);
logger.info("Processed event: {}", event);
} catch (Exception e) {
logger.error("Failed to process event: {}", event, e);
}
}
In this example, we log the processing of events, including failures. This helps in immediate diagnosis and remediation of issues. For advanced systems, consider linking logs to a centralized logging solution for better insights.
6. Ignoring Eventual Consistency
Issue: Event-driven systems often operate under eventual consistency, which might confuse developers accustomed to strong consistency models.
Tip: Educate your team about eventual consistency and design your system accordingly, ensuring that users are aware of potential temporary states.
Example Explanation:
Using a payment system as an example, a user may register their details and trigger a fund withdrawal. Due to the asynchronous nature, users may see their account status update, but the transaction may take a bit longer to reflect, leading to scenarios where the user perceives inconsistency.
7. Not Planning for Retries and Failures
Issue: Events may fail to be delivered due to system errors or network issues, leading to potential data loss if not handled.
Tip: Implement proper retry and dead-letter queue mechanisms to ensure events that couldn't be processed do not get lost.
Retry Logic Example:
@Retryable(
value = { ProcessingException.class },
maxAttempts = 5,
backoff = @Backoff(delay = 2000)
)
public void processEvent(UserRegisteredEvent event) {
// Processing logic
}
This example utilizes Spring's @Retryable
annotation to retry the processing of the event up to five times with a specified delay, enhancing reliability.
The Last Word
Transitioning to an event-driven microservices architecture is a rewarding but complex journey. By avoiding common pitfalls—like overcomplicating the event model, ignoring event schema evolution, and neglecting security—you can build robust and efficient systems that take full advantage of the benefits this architecture offers.
As you implement your solutions, don't forget the importance of monitoring, retries, and eventual consistency. By adhering to these best practices, you will set your development practices on a path toward sustainable growth.
For further reading on event-driven architectures, consider checking out Martin Fowler’s guide to Event-Driven Architecture and Dan Woods' insights on microservices. These resources can provide valuable insights and deeper dives into the subject.
Feel free to share your experiences or ask questions in the comments below! Happy coding!