Overcoming Common JPackage Pitfalls in Java Apps
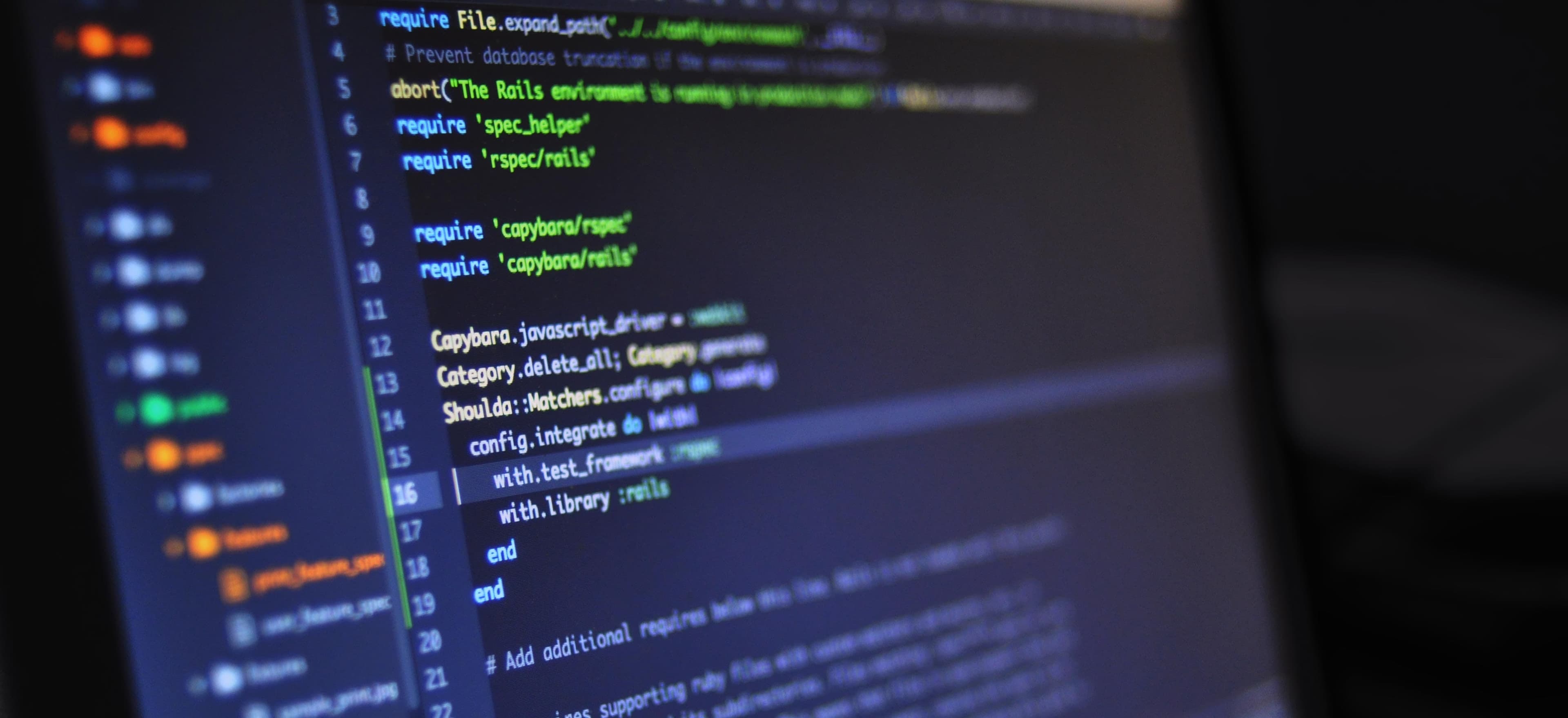
- Published on
Overcoming Common JPackage Pitfalls in Java Apps
Java's modular system introduced a revolutionary way to manage dependencies and package applications. With tools like jlink
and jpackage
, developers can easily bundle and distribute their Java applications. However, despite the benefits, many developers encounter pitfalls while using jpackage
. In this blog post, we will explore these common issues, present solutions, and share code snippets that illustrate best practices.
What is JPackage?
Before diving into pitfalls, let's clarify what jpackage
does. Introduced in Java 14 as an incubating feature, jpackage
is a command-line tool that packages a Java application into a native installer. It allows applications to be bundled with their dependencies, making distribution and installation seamless for end users.
Common JPackage Pitfalls
1. Ignoring the Module Path
One common pitfall is neglecting the module path versus the classpath. With the introduction of the Java Platform Module System (JPMS), using the wrong path can lead to runtime errors.
Why It Matters: The module path is essential for identifying module dependencies correctly. If your application uses modules, ensure you set the module path correctly when running jpackage
.
Example Code:
jpackage --input input-dir \
--output output-dir \
--name MyApp \
--main-jar myapp.jar \
--module-path lib:libs \
--main-class com.example.Main
In this example, --module-path
specifies where the required modules are located.
2. Bundle Size
Another frequent issue is the size of the packaged application. Including unnecessary libraries can bloat your installer.
Why It Matters: A large bundle can deter users from downloading your application. It's important to include only what's necessary.
Solution: Before packaging, analyze and minimize dependencies. Tools like Maven Dependency Plugin can help identify and exclude unnecessary libraries.
Example Code:
<dependency>
<groupId>com.example</groupId>
<artifactId>unnecessary-library</artifactId>
<version>1.0</version>
<scope>provided</scope>
</dependency>
Setting the scope to provided
indicates that the dependency is available in the runtime environment but should not be included in the bundle.
3. Missing Icons and Resources
Omitting application icons and resources during packaging is another common mistake. Users appreciate visually appealing applications, and icons significantly enhance user experience.
Why It Matters: A missing icon can lead to a professional appearing app being perceived as low-quality.
Example Code:
jpackage --input input-dir \
--output output-dir \
--name MyApp \
--main-jar myapp.jar \
--icon app-icon.png
Including the --icon
option ensures that your application has the necessary branding.
4. Runtime Issues Due to Incomplete Dependencies
Your application might compile fine, but runtime issues can arise due to missing dependencies in the final build.
Why It Matters: Users expect your application to work seamlessly without errors. If it fails to start or runs into errors, it affects your reputation.
Solution: Always test your package on a clean machine that does not have your development environment installed.
Example Testing Steps:
- Build your application.
- Transfer the packaged application to a new system.
- Run it and address any missing dependencies.
5. Invalid JVM Options
Sometimes developers include JVM options that work fine in development but fail in a packaged environment.
Why It Matters: The native installer may have different configurations than the development environment, leading to unexpected behavior.
Solution:
Use --java-options
with care and validate configurations.
Example Code:
jpackage --input input-dir \
--output output-dir \
--name MyApp \
--main-jar myapp.jar \
--java-options "-Xmx512m -Xms256m"
This ensures that your application starts with the specified memory settings.
Best Practices for Using JPackage
Ensure Version Compatibility
Always verify that your Java version matches what your application requires. Inconsistencies can cause subtle bugs that are difficult to diagnose.
Leverage Native Packaging
If you plan to distribute over multiple platforms (Windows, Mac, Linux), consider creating a native installer for each. This approach ensures better integration and a user-friendly experience.
Example Code for Linux Build:
jpackage --type deb \
--input input-dir \
--output output-dir \
--name MyApp \
--main-jar myapp.jar
Use Custom Installer Options
Customizing your installer with options such as banners, additional files, or installation directories can significantly enhance the user experience. For example:
jpackage --app-version 1.0.0 \
--description "My Java Application" \
--input input-dir \
--output output-dir \
--name MyApp \
--main-jar myapp.jar
Include a README and License
Distributing your application with a README file and license agreement increases transparency and compliance with open source licenses. This is especially important when using third-party libraries.
Final Considerations
Navigating the world of jpackage
can feel daunting, but understanding and addressing these common pitfalls will streamline your application packaging and enhance user experience. By paying attention to the module path, scrutinizing bundle sizes, ensuring visual elements are in place, validating dependencies and options, and adhering to best practices, you can avoid the common hurdles that arise in Java application packaging.
For further insights into packaging Java applications, consider exploring the official JPackage documentation.
Keep learning and fine-tuning your approach, and your Java applications will not only function well but will also shine when delivered to users. Happy coding!