Mastering Java Thread Pools: Common Pitfalls to Avoid
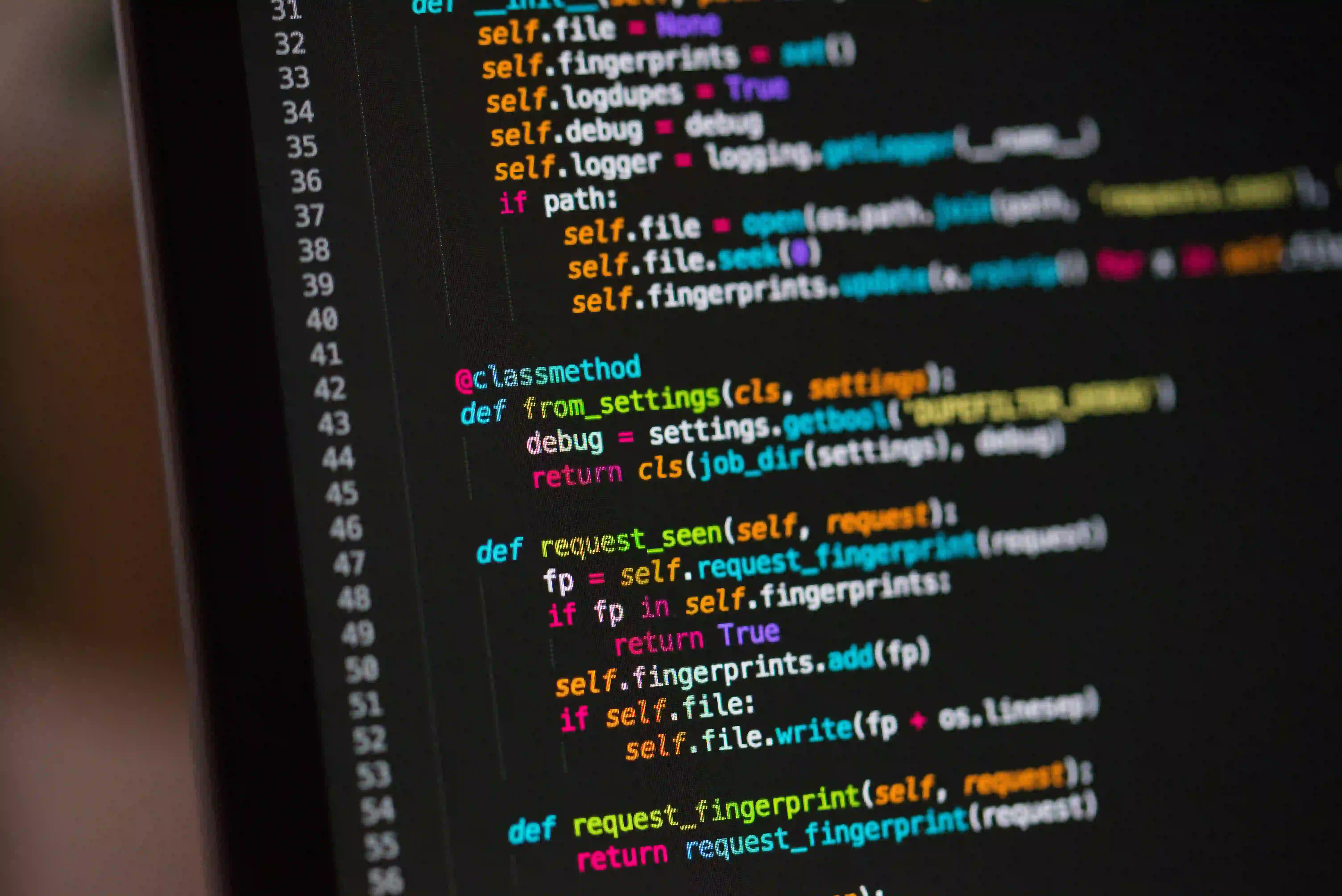
Mastering Java Thread Pools: Common Pitfalls to Avoid
When diving into multithreading in Java, one of the essential aspects developers encounter is thread pools. Managed correctly, they can significantly enhance the performance and efficiency of your applications. However, there are several pitfalls that can lead you down a road of inefficiency and bugs. In this post, we will explore these pitfalls in detail, providing best practices and code snippets to illustrate effective usage.
Understanding Thread Pools
Before diving into the common pitfalls, it's crucial to understand what thread pools are. A thread pool is a collection of pre-initialized threads that can be reused to handle multiple tasks. The main advantages of thread pools include:
- Performance Improvement: Reduces the overhead of thread creation.
- Resource Management: Provides better control over the number of concurrent threads and system resources.
- Task Management: Handles task queues efficiently.
To use thread pools in Java, the ExecutorService
interface provides various implementations, primarily through the Executors
factory class.
ExecutorService executorService = Executors.newFixedThreadPool(5);
In this example, we've created a thread pool with a maximum of five threads. This ensures that no more than five threads will run concurrently, thereby managing resource consumption.
Common Pitfalls in Using Java Thread Pools
1. Not Shutting Down the Executor
One of the most common mistakes developers make is not shutting down the ExecutorService
. If you fail to shut down your thread pool properly, you risk memory leaks and unresponsive applications.
Why This Matters: Unused threads linger in the background, consuming resources and potentially leading to memory exhaustion.
How to Do It:
executorService.shutdown();
This call gracefully shuts down the pool, allowing previously submitted tasks to execute before terminating the service.
2. Overloading the Pool
Another common pitfall is overloading the thread pool. Setting a thread pool size too high can lead to contention and a decrease in performance.
Why This Matters: When too many threads are vying for CPU and memory resources, context switching overhead increases, leading to reduced throughput.
Example Code:
ExecutorService executorService = Executors.newFixedThreadPool(20); // A number too high based on resources
for (int i = 0; i < 100; i++) {
executorService.submit(() -> {
// Simulate some work
performTask();
});
}
executorService.shutdown();
In this scenario, 20 threads may be excessive for the available resources. A careful assessment based on the system's capabilities is essential.
3. Ignoring Rejected Execution Handling
When tasks are submitted to a ExecutorService
and it's full, the default behavior will throw a RejectedExecutionException
. Ignoring this exception can lead to lost tasks.
Why This Matters: Failed submissions can lead to data loss or missed processing opportunities.
How to Handle:
You can customize the behavior when tasks are rejected by providing a RejectedExecutionHandler
.
ExecutorService executorService = new ThreadPoolExecutor(
5, // core pool size
10, // maximum pool size
60L, // keep-alive time
TimeUnit.SECONDS,
new ArrayBlockingQueue<>(5), // work queue
new ThreadPoolExecutor.AbortPolicy() // This is the default
);
You can replace AbortPolicy
with other strategies such as CallerRunsPolicy
, which provides feedback by running the rejected task on the thread that invokes execute
.
4. Not Using the Right Thread Pool Type
Choosing the wrong type of thread pool can lead to performance issues. Java's Executors
class provides different implementations such as newFixedThreadPool
, newCachedThreadPool
, and newScheduledThreadPool
, each serving different use cases.
Why This Matters: Using a CachedThreadPool
for tasks that require high predictability can lead to excessive thread creation and destruction, while a FixedThreadPool
is best for high-load, long-running tasks.
Example:
ExecutorService cachedThreadPool = Executors.newCachedThreadPool();
// Use for short-lived tasks
cachedThreadPool.submit(() -> {
// Short task
});
// Fixed thread pool for long running tasks
ExecutorService fixedThreadPool = Executors.newFixedThreadPool(2);
fixedThreadPool.submit(() -> {
// Long task
});
5. Deadlocks in Shared Resources
Using shared resources (like data structures) can lead to deadlocks if multiple threads attempt to access them simultaneously. Proper synchronization or utilizing thread-safe collections is vital to mitigate this risk.
Why This Matters: Deadlocks freeze your application, making it unresponsive.
Example Code:
Lock lock1 = new ReentrantLock();
Lock lock2 = new ReentrantLock();
Runnable task1 = () -> {
lock1.lock(); // Lock 1
try {
Thread.sleep(50);
lock2.lock(); // Lock 2
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock2.unlock();
lock1.unlock();
}
};
Runnable task2 = () -> {
lock2.lock(); // Lock 2
try {
Thread.sleep(50);
lock1.lock(); // Lock 1
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock1.unlock();
lock2.unlock();
}
};
In this code, two tasks could lead to a deadlock situation. Avoiding this and ensuring proper locking order can help mitigate the problem.
6. Not Handling Exceptions in Threads
When exceptions occur in a thread, they can sometimes terminate the thread silently without proper logging or handling, leading to harder-to-trace bugs later.
Why This Matters: Failing to catch exceptions means that important errors will go unmissed.
How to Catch Exceptions:
Runnable task = () -> {
try {
// Task logic
performCriticalOperation();
} catch (Exception e) {
System.err.println("Error occurred: " + e.getMessage());
}
};
executorService.submit(task);
By wrapping your task in a try-catch block, you can log or handle exceptions effectively.
7. Skipping the Use of Thread Pools Altogether
Despite the pitfalls, many developers shy away from using thread pools out of fear of complexity. This can lead to inefficient, blocking I/O operations that degrade application performance.
Why This Matters: Avoiding thread pools can lead to high resource consumption and suboptimal performance in multi-threaded applications.
Closing Remarks
Mastering Java Thread Pools involves understanding both their advantages and the common pitfalls associated with them. By avoiding issues like failing to shut down executors, overloading pools, and neglecting error handling, you can create robust and efficient multithreaded applications.
By proactively addressing these pitfalls, you will not only enhance the performance of your applications but also ensure better resource management, leading to a richer, more efficient experience for your users.
For further reading on Java multithreading, consider checking out Oracle's multithreaded programming guide or resources on thread safety to reinforce best practices in your development process.
Happy coding!