JNI vs JNA: Choosing the Right Tool for Your Project
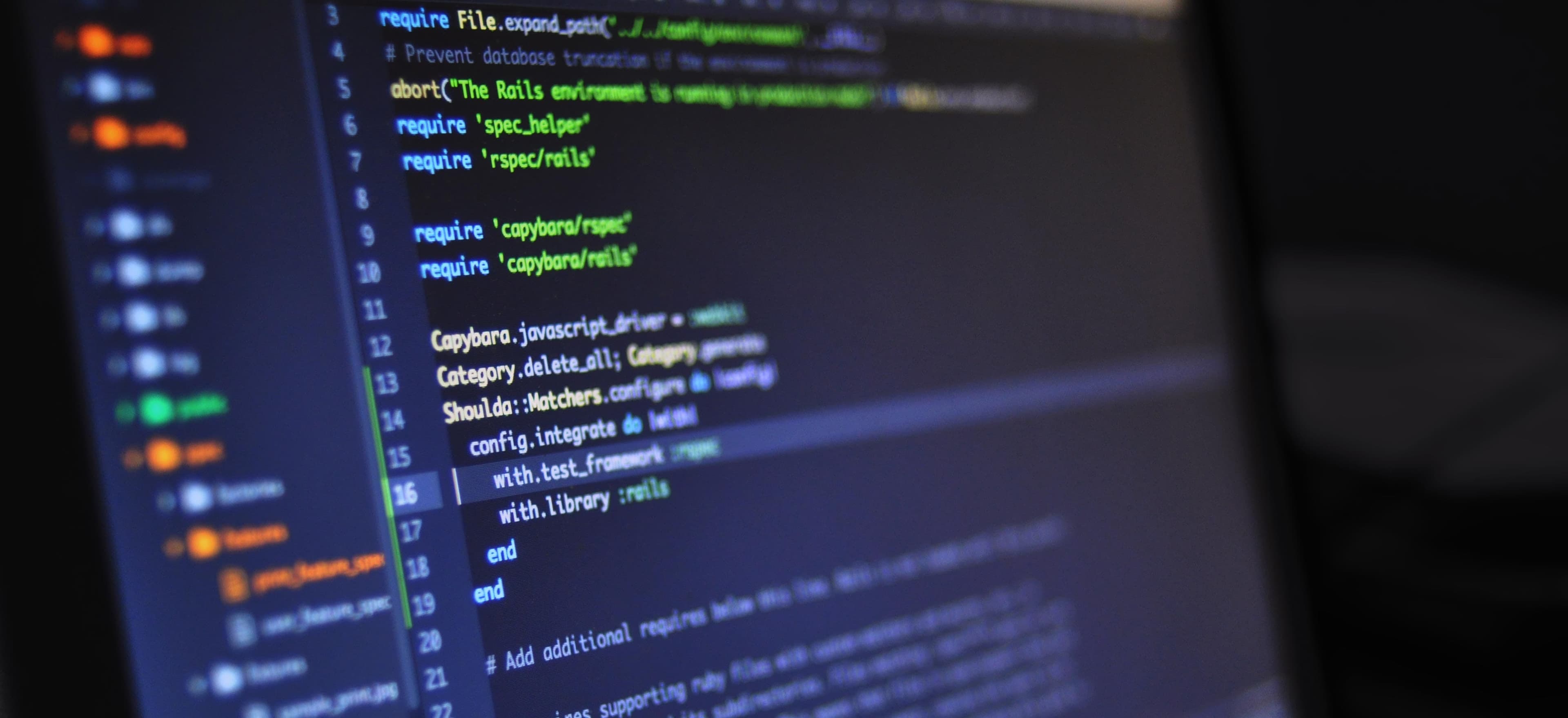
- Published on
JNI vs JNA: Choosing the Right Tool for Your Project
When developing Java applications that require native code integration, developers often face the choice between Java Native Interface (JNI) and Java Native Access (JNA). Both technologies serve the purpose of invoking native methods written in languages like C or C++, but they differ significantly in their approaches, complexity, performance, and use cases. This article aims to provide a comprehensive overview of both JNI and JNA, guiding you to choose the right tool for your project.
Understanding JNI and JNA
What is JNI?
The Java Native Interface (JNI) is a programming framework that allows Java code to interface with native applications and libraries written in other languages, most commonly C and C++. It is part of the Java platform and provides a mechanism to call native functions and utilize native resources directly within Java code.
Pros of JNI:
- Performance: JNI is generally faster than JNA because it is more closely integrated with the Java Virtual Machine (JVM).
- Control: JNI provides more control over the native interactions, allowing developers to optimize their code.
Cons of JNI:
- Complexity: Writing JNI code can be complicated, requiring a good understanding of both Java and native programming,
- Error-Prone: The complexity also makes it more prone to errors, which can lead to crashes.
What is JNA?
Java Native Access (JNA) is a simpler alternative that allows Java code to call native functions without writing any native code. JNA provides Java programs easy access to native shared libraries by providing a Java interface that maps to the native function signatures.
Pros of JNA:
- Ease of Use: JNA is simpler and requires less boilerplate code compared to JNI.
- Dynamic Loading: JNA can dynamically load native libraries without having to pre-compile any Java bindings to them.
Cons of JNA:
- Reduced Performance: While it is generally easier to use, JNA might result in slightly lower performance compared to JNI.
- Less Control: It abstracts much of the underlying complexity, which may not be suitable for all scenarios.
When to Use JNI
Performance-Critical Applications
If your application demands high performance and low latency, JNI might be the better choice. Native code can be optimized for performance in ways that may be necessary for certain applications, like graphics rendering, video processing, or real-time data processing.
Here is an example of JNI code that demonstrates how to call a simple native method from Java:
// Java code
public class HelloWorld {
// Load the native library
static {
System.loadLibrary("HelloWorld");
}
// Declare the native method
public native void sayHello();
public static void main(String[] args) {
new HelloWorld().sayHello(); // Call the native method
}
}
// C code (HelloWorld.c)
#include <jni.h>
#include <stdio.h>
#include "HelloWorld.h"
JNIEXPORT void JNICALL Java_HelloWorld_sayHello(JNIEnv *env, jobject obj) {
printf("Hello from C!\n"); // Print hello message
}
In this example:
- A Java class declares a native method.
- The native C implementation of this method prints a message.
System.loadLibrary
imports the native library at runtime.
Advanced Use-Cases
Developers who need to work with complex data structures or require fine-grained control over memory management should opt for JNI. JNI allows for intricate operations that may be complicated to achieve through JNA.
When to Use JNA
Rapid Prototyping and Development
For many applications, especially those that are in the early stages or require quick deployment, JNA is a more suitable option. If your goal is to rapidly prototype or if the native calls are relatively simple, JNA offers a much friendlier developer experience. The following example demonstrates how to access a native library using JNA:
import com.sun.jna.Library;
import com.sun.jna.Native;
public class HelloWorld {
// Define an interface for the native library
public interface CLibrary extends Library {
CLibrary INSTANCE = (CLibrary) Native.load("HelloWorld", CLibrary.class);
void sayHello();
}
public static void main(String[] args) {
CLibrary.INSTANCE.sayHello(); // Call the native method
}
}
In this example:
- The
CLibrary
interface defines the native methods. - The
Native.load
function automatically handles the loading of the native library. - The native method
sayHello
is invoked just like a regular Java method.
Applications with Light Native Requirements
If your application integrates with existing native libraries that have simple functions, then JNA is probably sufficient and will save you time. Also, since JNA requires no specialized knowledge beyond Java, it can be a good choice for teams that may not have experience with native code.
Performance Considerations
As a rule of thumb, you should always measure the performance impact of JNI vs JNA for your particular use case. In performance-critical applications, JNI typically outperforms JNA, especially when numerous calls are made to native code. That said, the differences may not be significant for the majority of applications.
My Closing Thoughts on the Matter: Making the Right Choice
The choice between JNI and JNA ultimately comes down to your specific project requirements. Use JNI for performance-critical applications where control and efficiency are paramount. Opt for JNA when your priority is rapid development and ease of use.
Quick Comparison Table
| Feature | JNI | JNA | |------------------|---------------------------|----------------------------| | Complexity | High | Low | | Performance | High | Moderate | | Control | Fine-grained | Abstracted | | Use Case | Advanced applications | Rapid prototyping | | Error-Prone | More susceptible to errors | Generally safer |
Further Reading
To enhance your understanding, you may want to check out the official documentation for JNI and JNA reference documentation.
In the end, choosing between JNI and JNA is a matter of weighing benefits against your project’s limitations and needs. Evaluate your requirements carefully, and make the choice that aligns best with your development goals.