Common JNA Pitfalls and How to Avoid Them
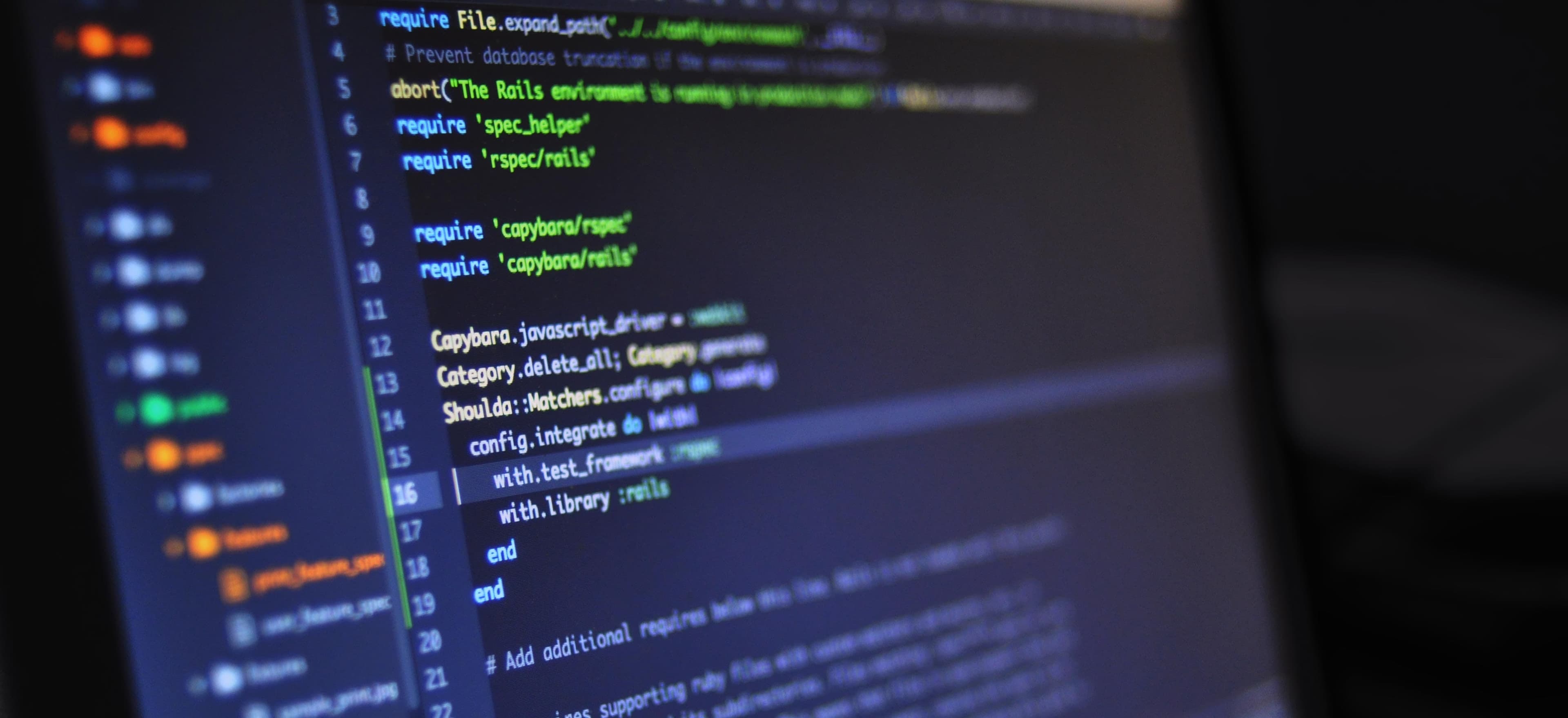
- Published on
Common JNA Pitfalls and How to Avoid Them
Java Native Access (JNA) is a fantastic tool for Java developers who need to interface with native code in an efficient and user-friendly manner. It allows developers to call native libraries directly from Java without needing to write extensive JNI (Java Native Interface) code. However, while JNA simplifies many tasks, it also comes with its share of pitfalls that can lead to frustrating debugging sessions and unintended consequences.
In this blog post, we'll explore common JNA pitfalls and provide solutions to avoid them. Whether you are new to JNA or looking to refine your existing skills, this guide will help bolster your understanding and effectiveness in working with native libraries in Java.
Understanding JNA Basics
Before diving into pitfalls, let's briefly understand what JNA is. JNA provides Java programs easy access to native shared libraries (DLLs on Windows or .so files on Linux). It is achieved by creating interface definitions in Java that match the native function signatures.
For example:
import com.sun.jna.Library;
import com.sun.jna.Native;
public interface MyCLibrary extends Library {
MyCLibrary INSTANCE = (MyCLibrary) Native.loadLibrary("myc", MyCLibrary.class);
int myNativeFunction(int number);
}
In this code snippet, we define a Java interface that maps to the native function from our C library. With this setup, we can directly call myNativeFunction
from Java.
Pitfall 1: Misunderstanding Data Types
One of the most common pitfalls in JNA is misunderstanding the data type mappings between Java and C. JNA provides mappings for most common data types, but not all.
Example:
public interface MyCLibrary extends Library {
byte[] myNativeByteArrayFunction(int size);
}
Here, we expect to receive a byte array directly. However, if the C function returns a pointer to an array, the JNI garbage collector might intervene, leading to unexpected results.
Solution:
Always verify the C data types and their Java equivalents. For pointers or arrays, use Pointer
or define specific wrappers to guarantee proper memory handling. Here's how to safely retrieve an array:
public interface MyCLibrary extends Library {
Pointer myNativeByteArrayFunction(int size);
}
public byte[] getByteArray(int size) {
Pointer pointer = MyCLibrary.INSTANCE.myNativeByteArrayFunction(size);
byte[] array = pointer.getByteArray(0, size);
return array;
}
Pitfall 2: Memory Management Misconceptions
In JNA, memory management becomes critical. While JNA handles a lot of behind-the-scenes memory allocation, misusing this can lead to memory leaks or segmentation faults.
Example:
public interface MyCLibrary extends Library {
Pointer createNativeString(String str);
void freeNativeString(Pointer ptr);
}
If you forget to free up allocated memory, the application may suffer from memory leaks.
Solution:
Recognize when you need to manually release resources and ensure you always call free functions. Implementing a wrapper can help avoid memory leaks.
public class NativeString {
private final Pointer ptr;
public NativeString(String str) {
this.ptr = MyCLibrary.INSTANCE.createNativeString(str);
}
public String getValue() {
return ptr.getString(0);
}
public void cleanup() {
MyCLibrary.INSTANCE.freeNativeString(ptr);
}
}
Always invoke cleanup
to avoid leaks.
Pitfall 3: Thread Safety Issues
JNA is not inherently thread-safe. Many native libraries, especially older C libraries, may not handle concurrent access effectively, leading to race conditions or corrupted data.
Solution:
If you need to access native libraries from multiple threads, ensure proper synchronization. Utilize Java's synchronized blocks or consider Java's ReentrantLock
for more complex scenarios.
Example:
public class SynchronizedLibraryAccess {
private final MyCLibrary library = MyCLibrary.INSTANCE;
public synchronized int safeNativeFunction(int number) {
return library.myNativeFunction(number);
}
}
By using synchronized
, we ensure that only one thread can access safeNativeFunction
at any given time.
Pitfall 4: Not Handling Native Exceptions
Native code can throw exceptions or return error codes that Java won’t handle automatically. This can lead to silent failures in your application.
Example:
public interface MyCLibrary extends Library {
int myNativeFunction(int number);
int getLastError();
}
If myNativeFunction
fails, we need a way to check the error:
Solution:
Always check return values and implement a structured error-handling mechanism.
public int callNativeFunction(int input) {
int result = MyCLibrary.INSTANCE.myNativeFunction(input);
if (result < 0) {
int errorCode = MyCLibrary.INSTANCE.getLastError();
throw new RuntimeException("Native function failed with error code: " + errorCode);
}
return result;
}
Additional Resources
For further reading on error handling in JNA, you might find JNA's Official Documentation helpful.
Pitfall 5: Ignoring Platform Compatibility
Using native libraries often means dealing with platform-specific nuances. A library compiled for Windows may not work on macOS or Linux.
Solution:
Be explicit about the library being loaded and accommodate platform checks in your code.
public class NativeLibraryLoader {
public static MyCLibrary loadLibrary() {
String osName = System.getProperty("os.name").toLowerCase();
if (osName.contains("win")) {
return (MyCLibrary) Native.loadLibrary("myc.dll", MyCLibrary.class);
} else if (osName.contains("mac")) {
return (MyCLibrary) Native.loadLibrary("libmyc.dylib", MyCLibrary.class);
} else {
return (MyCLibrary) Native.loadLibrary("libmyc.so", MyCLibrary.class);
}
}
}
This code will ensure that the appropriate library is loaded based on the OS.
The Closing Argument
While JNA is a powerful tool that grants Java developers the ability to easily access native libraries, it is essential to understand the common pitfalls that come along with its use. By carefully watching out for data type mismatches, ensuring proper memory management, implementing thread safety, handling native exceptions, and confirming platform compatibility, developers can harness the full potential of JNA without falling into its traps.
By applying the tips laid out in this article, you will be better positioned to create robust and efficient applications that integrate seamlessly with native code. Happy coding!
Checkout our other articles