Common Pitfalls When Integrating Cassandra with Java
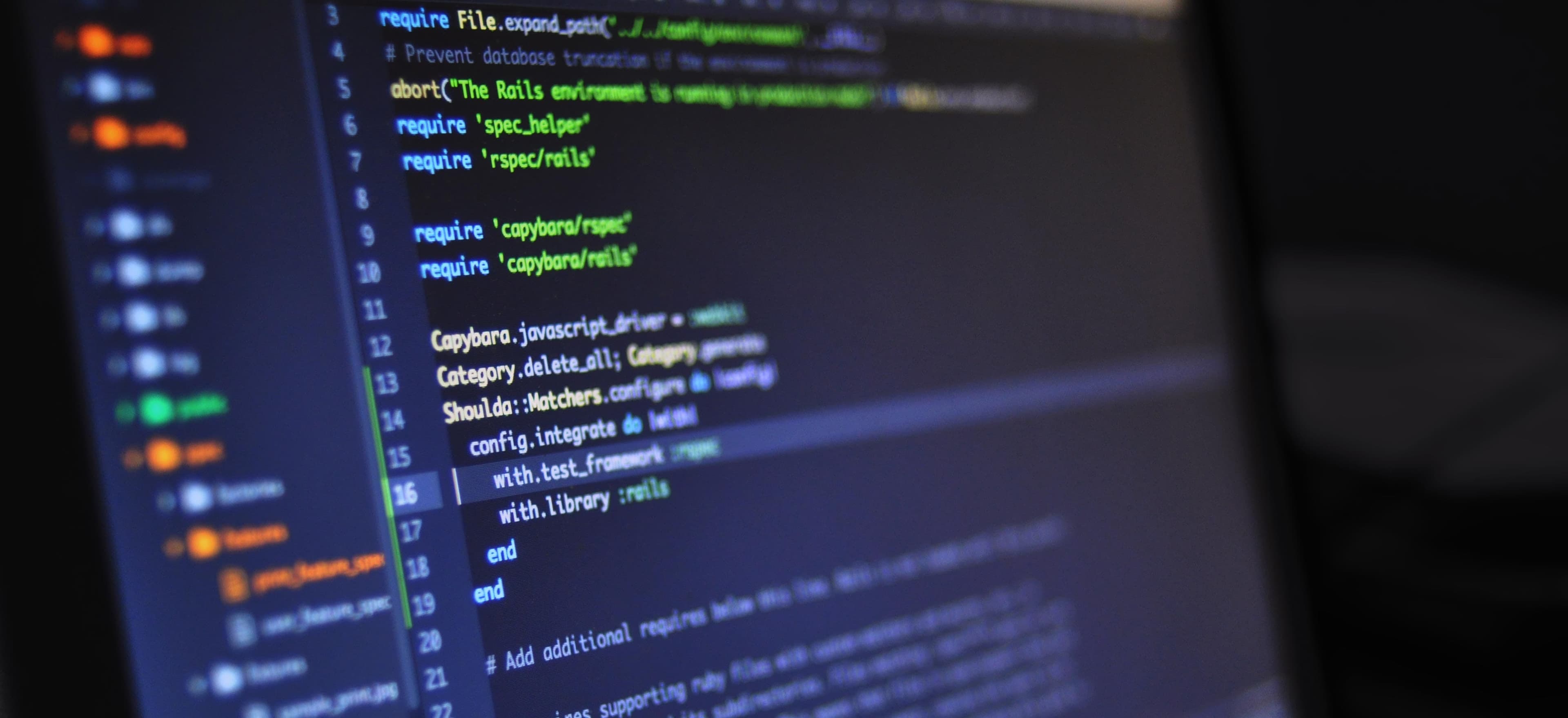
- Published on
Common Pitfalls When Integrating Cassandra with Java
Apache Cassandra is an open-source, distributed database management system designed to handle large amounts of data across many servers while providing high availability with no single point of failure. Its ability to manage vast volumes of data makes it a favorite among developers. However, integrating Cassandra with Java is not without its challenges. Understanding these common pitfalls will enable developers to navigate this powerful tool more efficiently and effectively.
In this blog post, we will discuss several common pitfalls that developers encounter when integrating Cassandra with Java, along with best practices and practical code examples to help you avoid them.
1. Ignoring Data Modeling Principles
Why Data Modeling is Important
Cassandra is a NoSQL database that offers flexibility in schema design. However, that flexibility can lead to poorly designed data models if not approached thoughtfully. Because data is not normalized, it can lead to redundancy and inefficiencies if developers don't understand how to leverage Cassandra’s strengths.
Best Practices
Prioritize understanding your data access patterns before designing your schema. The data model should be optimized for queries, and you might need to denormalize your data to achieve your performance goals.
Code Example
Here is a simple example demonstrating the importance of modeling:
CREATE TABLE user_activity (
user_id UUID,
activity_time TIMESTAMP,
activity_description text,
PRIMARY KEY (user_id, activity_time)
);
In this schema:
user_id
acts as your partition key for efficient querying.activity_time
is the clustering key, allowing for sorting activities over time.
This structure allows for efficient access patterns when querying user activities by time.
2. Overlooking Connection Management
Connection Management is Key
Cassandra uses a more complex driver setup compared to traditional databases. Not properly managing connections can lead to performance bottlenecks.
Best Practices
Utilize a connection pool to manage Cassandra sessions effectively. The Datastax Java driver allows you to easily set up a connection pool.
Code Example
Here's how to create a connection pool for Cassandra:
import com.datastax.oss.driver.api.core.CassandraSession;
import com.datastax.oss.driver.api.core.CassandraCluster;
import com.datastax.oss.driver.api.core.config.DefaultDriverConfig;
import com.datastax.oss.driver.api.core.config.DriverConfigLoader;
public class CassandraConnector {
private CassandraSession session;
public void connect(String node, Integer port) {
session = CassandraCluster.builder()
.addContactPoint(new InetSocketAddress(node, port))
.withPoolingOptions(new PoolingOptions()
.setMaxRequestsPerConnection(ProtocolVersion.DEFAULT, 1))
.build()
.connect();
}
public void close() {
session.close();
}
}
This code demonstrates how to create a connection pool by utilizing the CassandraCluster
to manage connections effectively. Make sure to close sessions appropriately to prevent memory leaks.
3. Not Handling Exceptions Correctly
The Importance of Exception Handling
Cassandra interactions can lead to various exceptions; overlooking these can cause the application to crash or function unpredictably.
Best Practices
Always include robust exception handling to manage common errors like timeouts, unavailable nodes, or write failures.
Code Example
This code snippet demonstrates how to handle exceptions gracefully:
try {
ResultSet rs = session.execute("SELECT * FROM users");
for (Row row : rs) {
System.out.println(row.getString("name"));
}
} catch (QueryExecutionException e) {
System.err.println("Query failed: " + e.getMessage());
} catch (NoHostAvailableException e) {
System.err.println("No available hosts: " + e.getMessage());
} finally {
close();
}
In this example, we clearly handle potential exceptions that may arise during query execution. It's essential to have fallback mechanisms and create clear logs for debugging.
4. Failing to Tune Performance Parameters
Performance Tuning is Crucial
Cassandra offers numerous tunable parameters that can drastically affect the performance of your application. Failing to optimize these settings may lead to underperformance.
Best Practices
Understand and experiment with configurations like readiness timeouts, connection pooling, and batch sizes to optimize performance.
Code Example
Here’s how to set some performance configurations:
ClusterBuilder builder = Cluster.builder();
builder.withSocketOptions(
new SocketOptions().setReadTimeoutMillis(5000)
);
This simple adjustment minimizes read timeouts, helping reduce latency in high-volume applications.
5. Inefficient Use of Batches
Avoiding Batch Misuse
While batching can optimize the performance of your write operations, misusing it can lead to poor performance results and increased latency.
Best Practices
Keep your batch sizes small and avoid using them for a large number of records across different partitions. Batching should be used to group together related writes.
Code Example
Consider the following snippet, illustrating an efficient use of batches for related updates:
BatchStatement batch = BatchStatement.newInstance(BatchType.UNLOGGED);
for (User user : usersToInsert) {
Statement insert = SimpleStatement.newInstance("INSERT INTO users (id, name, age) VALUES (?, ?, ?)",
user.getId(), user.getName(), user.getAge());
batch.add(insert);
}
session.execute(batch);
In this example:
- We only group related user insert statements together, keeping performance in check by choosing UNLOGGED batches.
6. Failing to Monitor and Analyze Performance
Why Monitoring Matters
Once integrated, monitoring the performance and health of your Cassandra cluster is vital. Failing to do so can cause unnoticed degradation over time.
Best Practices
Utilize tools like DataStax OpsCenter for monitoring critical metrics and setting up alerts for abnormal behaviors.
Code Example
Integrate a simple health check in your Java application:
public boolean isClusterAlive() {
try {
session.execute("SELECT now() FROM system.local");
return true;
} catch (Exception e) {
return false;
}
}
Regular health checks ensure that your application runs smoothly and that issues are identified early on.
The Closing Argument
Integrating Cassandra with Java can be extremely beneficial but comes with its challenges. By acknowledging and addressing these common pitfalls — data modeling, connection management, exception handling, performance tuning, batching, and monitoring — you can avoid unnecessary complications and utilize the full potential of both technologies.
With diligent attention to these practices, your applications will be robust, efficient, and scalable.
If you're interested in diving deeper into Cassandra, check out the official Apache Cassandra Documentation. Happy coding!