Common Pitfalls When Building Command-Line Apps in Java
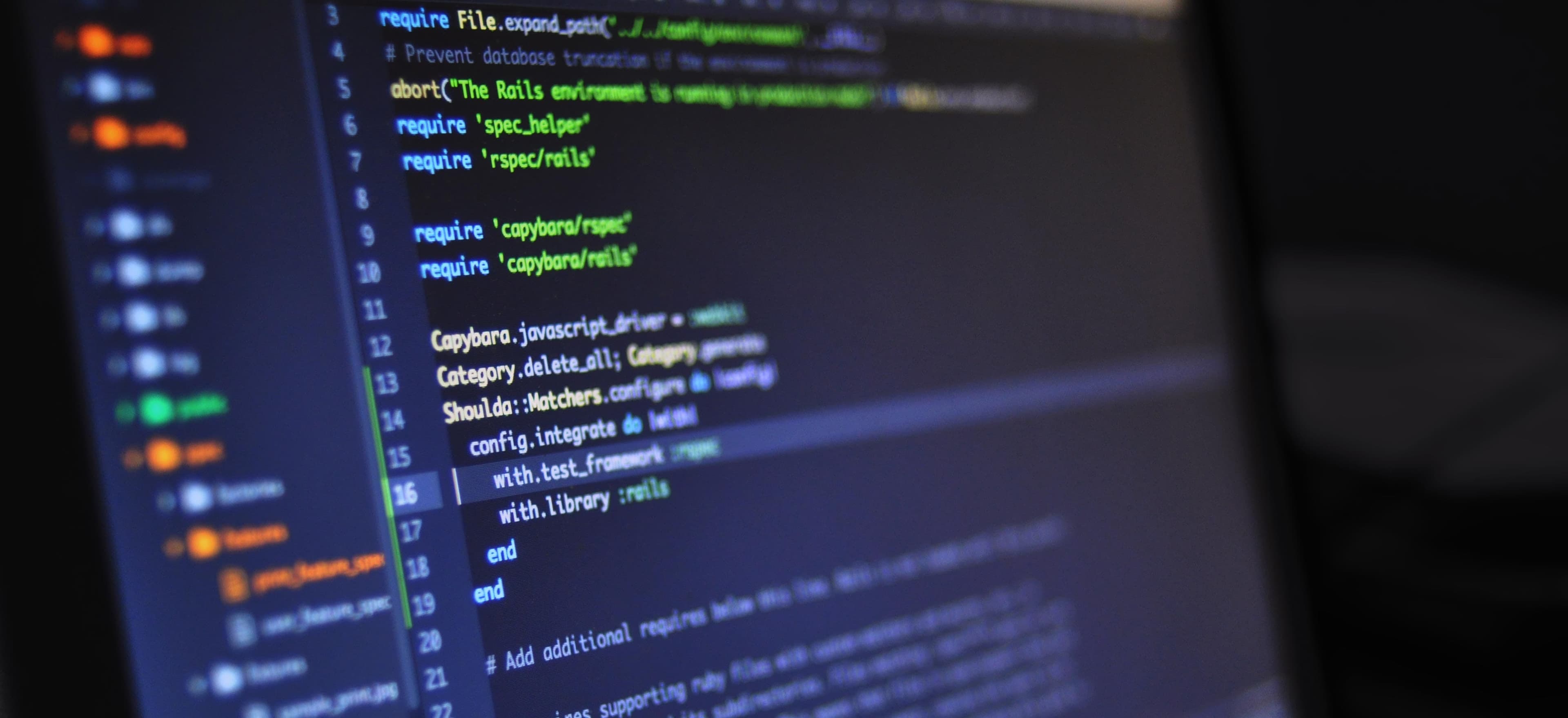
- Published on
Common Pitfalls When Building Command-Line Apps in Java
Command-line applications in Java are a powerful way to interact with users and empower them to perform tasks through a terminal interface. However, building such applications can lead to a handful of pitfalls that can derail your entire project. This blog post seeks to illuminate those pitfalls and provide guidance on how to avoid them.
Understanding Command-Line Applications
Command-line applications (CLIs) are programs that can be started from a text-only interface, typically a terminal or console. They are beneficial for automating repetitive tasks, managing system configurations, or running scripts. While Java is not the first language you might think of for this purpose, its rich ecosystem and libraries make it a strong candidate.
Why Java?
Java is known for its cross-platform compatibility, robustness, and extensive libraries. The language is an excellent choice for building scalable and maintainable applications, even those that run from a command line. However, as with any tool, there are common pitfalls to avoid.
Common Pitfalls in CLI Development
1. Neglecting Argument Parsing
A common pitfall is to neglect proper argument parsing. Command-line applications frequently make use of command-line arguments. Failing to parse them correctly can lead to unexpected behaviors.
For example, consider this snippet where we simply print out command-line arguments:
public class CommandLineArgs {
public static void main(String[] args) {
for (String arg : args) {
System.out.println(arg);
}
}
}
While this works, it doesn't handle the various nuances of input. You might want to include argument validation to avoid errors. A more robust solution is to use a library like Apache Commons CLI or JCommander, allowing you to define options more clearly.
Why Use Libraries?
Libraries provide structure and error handling out-of-the-box, allowing you to concentrate on your business logic rather than reinventing the wheel. For more information on Apache Commons CLI, check out the Apache Commons CLI documentation.
2. Ignoring User Input Validation
User input validation is crucial in any application. Command-line apps often receive various types of input from users, including strings, integers, and file paths. Ignoring validation can lead to runtime errors or insecure code.
import java.util.Scanner;
public class InputValidation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your age: ");
String input = scanner.nextLine();
try {
int age = Integer.parseInt(input);
if (age < 0) {
System.out.println("Age cannot be negative.");
} else {
System.out.println("Your age is: " + age);
}
} catch (NumberFormatException e) {
System.out.println("Invalid age input. Please enter a number.");
}
}
}
Why Validate?
A well-structured validation mechanism leads to a better user experience. It also makes your code more robust and easier to maintain.
3. Failing to Offer Help and Documentation
One of the most significant oversights in CLI development is the lack of help documentation. Users should be able to enter a simple command like --help
or -h
to get information about how to use the app.
You can accomplish this by adding a method that displays usage instructions:
public static void displayHelp() {
System.out.println("Usage: command [options]");
System.out.println("Options:");
System.out.println(" -h, --help Display help");
System.out.println(" -o, --option Example of an option");
}
Why Help Matters
Good help documentation can serve as both a user guide and a troubleshooting aid. It reduces user frustration, promotes adoption, and is a sign of professionalism.
4. Not Handling Exceptions Properly
Exception handling is crucial for maintaining a stable command-line application. Consider how your application should respond when it encounters an error: should it crash, or is there a way to recover gracefully?
Here’s a simple example:
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
// Simulated code that can throw an exception
riskyOperation();
} catch (Exception e) {
System.out.println("An error has occurred: " + e.getMessage());
// Implement any additional recovery or logging mechanisms
}
}
public static void riskyOperation() throws Exception {
throw new Exception("Simulated exception");
}
}
Why Handle Exceptions?
Proper exception handling ensures your application remains user-friendly even when unexpected situations occur. It allows you to log issues for debugging while providing meaningful feedback to the user.
5. Poorly Structured Code
When building a command-line application, keeping your code modular and structured should be a priority. Avoid having a monolithic main method. Instead, separate concerns into classes and methods.
Here's a simple example of good structure:
public class Calculator {
public double add(double a, double b) {
return a + b;
}
public double subtract(double a, double b) {
return a - b;
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
System.out.println("Sum: " + calculator.add(5, 3));
}
}
Why Structure Code?
Well-structured code is easier to maintain, test, and expand. It promotes code reusability and makes it easier for other developers to contribute.
6. Ignoring Cross-Platform Compatibility
Remember that Java is designed for cross-platform compatibility, but your application could be affected by the environment it is executed in. For instance, commands and paths can differ across operating systems.
Therefore, always use platform-independent structures, such as:
- Use
File.separator
for file paths. - Avoid system-specific commands when possible.
Why Cross-Platform Compatibility is Important
Users will likely run your application in various environments. Ignoring this can alienate a part of your user base.
Final Thoughts
Building command-line applications in Java can be an enriching experience, but being mindful of common pitfalls is crucial for success. From proper argument parsing and input validation to structured code and exception handling, acknowledging these challenges will set you on the path to creating user-friendly, robust, and maintainable applications.
Further Reading
For a deeper understanding of effective CLI development practices, you can check out resources like:
Understanding and avoiding the common pitfalls discussed in this blog can significantly enhance the quality of your Java command-line applications. Happy coding!