Why Java Developers Struggle with MongoDB Integration
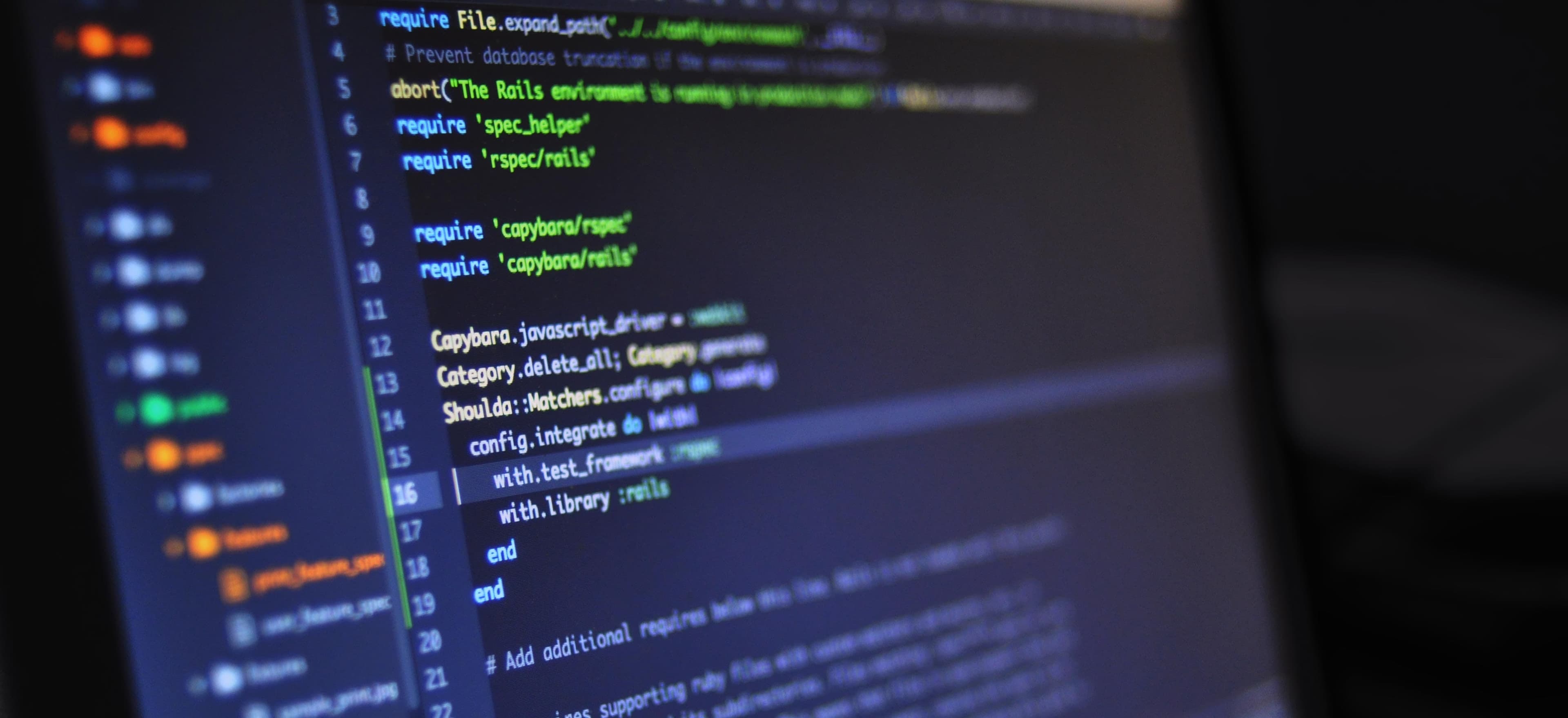
- Published on
Why Java Developers Struggle with MongoDB Integration
In the rapidly evolving landscape of web technologies, Java continues to be a prominent backend programming language. However, when it comes to integrating with NoSQL databases like MongoDB, many Java developers face challenges. This blog aims to explore the core reasons why this struggle exists, while also providing practical solutions and insights that can streamline the integration process.
Understanding MongoDB and Its Adoption
MongoDB is a NoSQL document database known for its flexibility, scalability, and robust performance. Unlike traditional SQL databases, MongoDB stores data in a JSON-like format, conducive to hierarchical data structures. Developers embrace MongoDB for its ease of scaling and handling unstructured data, which has become increasingly essential in modern web applications.
However, despite its advantages, Java developers often find integrating MongoDB into their applications daunting. Let’s delve into the factors contributing to this phenomenon.
1. Different Paradigms
Java is often associated with a rigid object-oriented paradigm, focusing heavily on explicit data structures and type declarations. Conversely, MongoDB embraces a dynamic schema environment. While the flexibility of MongoDB is liberating, it can be counterintuitive for Java developers who are used to the strict data typologies of relational databases.
Code Snippet: Mapping Java Objects to MongoDB Documents
Here’s a simple example of mapping a Java object to a MongoDB document with the MongoDB Java Driver.
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public Document toDocument() {
return new Document("name", name).append("age", age);
}
// Additional getters and setters can be defined here
}
// Usage
MongoDatabase database = mongoClient.getDatabase("testdb");
MongoCollection<Document> collection = database.getCollection("users");
User user = new User("Alice", 30);
collection.insertOne(user.toDocument());
Why This Matters: Java developers need to adapt their thinking to embrace a more dynamic structure, which can feel foreign. The toDocument
method clearly illustrates how a Java object can be transformed into a MongoDB document. Understanding this conversion is pivotal.
2. Lack of Strong Typing
Java's strong type system is one of its core strengths. However, the type system isn't as robust in MongoDB. This mismatch can lead to runtime errors that are often hard to debug.
For example, when storing or retrieving data, there may be discrepancies between expected and actual types. In some cases, data might not be present or might appear in an unexpected format, leading to Null Pointer Exceptions.
Handling Data with Type Safety
To enhance type safety with MongoDB, it is recommended to use libraries like Morphia:
import org.mongodb.morphia.annotations.Entity;
import org.mongodb.morphia.annotations.Id;
@Entity
public class Car {
@Id
private String id;
private String make;
private String model;
// Constructors, Getters, Setters
}
// Usage
Datastore datastore = morphia.createDatastore(mongoClient, "testdb");
Car car = new Car("1", "Tesla", "Model S");
datastore.save(car);
Why This Matters: Libraries like Morphia abstract away some of the integration complexities and help with mapping entities to documents, which can lead to safer, less error-prone code.
3. Query Language Differences
Java developers are often used to SQL (Structured Query Language) for executing database queries. SQL has a defined structure, making it easy to read and write queries. On the other hand, MongoDB uses its own query language, which may be less intuitive for developers accustomed to SQL.
MongoDB Query Example
Here’s a basic example of querying a MongoDB collection:
import static com.mongodb.client.model.Filters.eq;
MongoCollection<Document> collection = database.getCollection("users");
Document foundUser = collection.find(eq("name", "Alice")).first();
if (foundUser != null) {
System.out.println("Found user: " + foundUser.toJson());
}
Why This Matters: Learning to navigate these differences is crucial. A solid understanding of MongoDB’s querying capabilities is essential for effective database interactions, allowing Java developers to utilize the strengths of MongoDB efficiently.
4. Learning Curve of Asynchronous Programming
As Java continues to grow, the importance of asynchronous programming has emerged, especially with frameworks like Spring WebFlux. MongoDB’s drivers are often designed to handle asynchronous operations, which can be a steep learning curve for developers used to synchronous programming models.
Example of Asynchronous Call
Here is an example of an asynchronous call using the MongoDB driver:
MongoCollection<Document> collection = database.getCollection("users");
collection.find(eq("name", "Alice"))
.forEach(doc -> System.out.println(doc.toJson()),
(result, t) -> {
if (t != null) {
System.err.println("Error: " + t.getMessage());
} else {
System.out.println("Query completed successfully");
}
});
Why This Matters: Embracing asynchronous operations is critical for achieving optimal performance and responsiveness in applications. Developers must invest time in learning how asynchronous models work, particularly in combination with MongoDB.
5. Absence of Comprehensive Documentation
While MongoDB does have considerable documentation, many Java developers feel it lacks comprehensive examples tailored specifically for Java integrations. The vast array of drivers and libraries can also create confusion, making it difficult to choose the right tool for specific use cases.
To supplement this, developers can refer to resources like MongoDB Java Driver documentation for in-depth guides.
Lessons Learned
Integrating MongoDB with Java presents a unique set of challenges. From differing paradigms and learning curves to the requirement for understanding NoSQL query syntax and handling asynchronous programming, Java developers must adjust their approach significantly.
However, with suitable tools and thorough learning, they can overcome these hurdles. Adopting abstraction libraries, investing time in understanding the core concepts of NoSQL, and practicing converting data structures can substantially ease the integration process.
Ultimately, embracing new paradigms and overcoming the integration challenges can lead to the development of highly scalable and responsive applications. Whether you are an experienced Java developer or just starting out, there's ample opportunity to master the art of MongoDB integration.
For more resources, consider reading about successful Java and MongoDB projects or exploring libraries like Morphia or Spring Data MongoDB for efficient database management. Happy coding!