Maximizing Java Code Coverage: Common Mistakes to Avoid
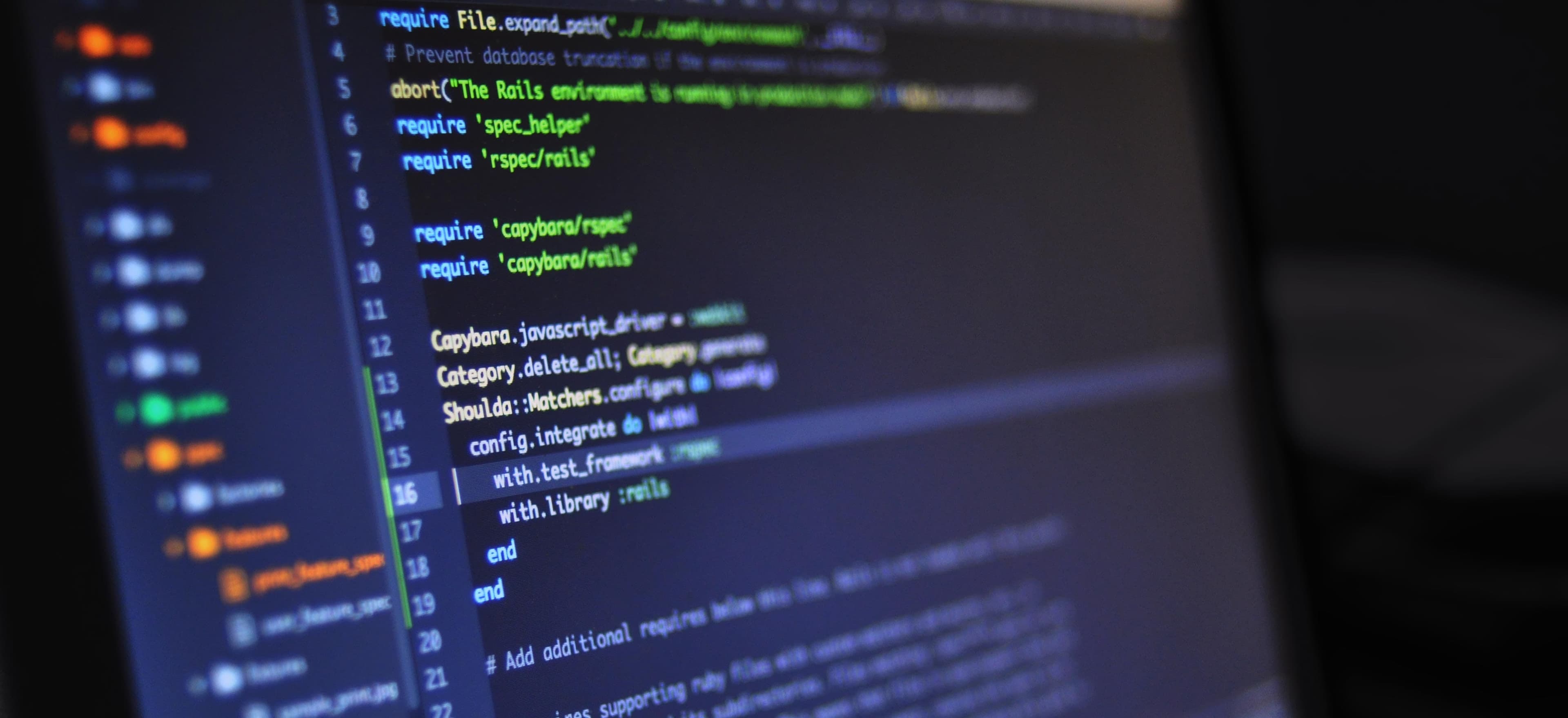
- Published on
Maximizing Java Code Coverage: Common Mistakes to Avoid
When it comes to software development, ensuring the quality of your code is paramount. High code coverage is often associated with high-quality software. In Java, achieving optimal code coverage is crucial, yet several common mistakes can hinder your efforts. In this blog post, we'll explore these pitfalls and how to avoid them, ensuring your code achieves maximum coverage efficiently.
What is Code Coverage?
Code coverage is a measure used to describe the degree to which the source code of a program is executed during testing. Essentially, it helps you identify untested parts of your codebase. In Java, code coverage can be achieved through unit tests, integration tests, and others. Tools like JaCoCo, Cobertura, or IntelliJ IDEA provide visual feedback on which parts of your code are covered by tests.
For more on code coverage tools, check out JaCoCo or Cobertura.
Why is Code Coverage Important?
Code coverage is important for several reasons:
- Quality Assurance: Higher coverage can indicate fewer defects.
- Refactoring Confidence: With extensive tests, you can refactor and improve code without fear.
- Documentation: Tests act as a form of documentation, indicating how the code is expected to behave.
Common Mistakes to Avoid
1. Assuming 100% Coverage Equals No Bugs
One of the most prevalent myths in software engineering is that achieving 100% code coverage guarantees a bug-free application. This assumption is faulty. Coverage measures only how much of the code has been executed during testing, not whether the tests effectively validate business logic.
Solution:
Focus not just on quantity but also on quality. Write meaningful tests that assert expected behaviors, not just dummy tests that increase coverage metrics.
@Test
public void testUserCreation() {
User user = new User("john.doe@example.com");
assertNotNull(user);
assertEquals("john.doe@example.com", user.getEmail());
}
In this example, we check that the User object is created as expected while asserting that the email is stored correctly, validating the logic behind the code.
2. Ignoring Edge Cases
Developers often write tests for the "happy path," neglecting edge cases. These are inputs and scenarios that might not occur frequently but can cause the application to fail.
Solution:
Identify edge cases early and ensure they are included in your test cases. Use parameterized tests to cover multiple scenarios.
@RunWith(Parameterized.class)
public class LoginTest {
private String username;
private String password;
private boolean expectedOutcome;
public LoginTest(String username, String password, boolean expectedOutcome) {
this.username = username;
this.password = password;
this.expectedOutcome = expectedOutcome;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ "user1", "pass1", true },
{ "", "pass2", false }, // edge case: empty username
{ "user3", "", false }, // edge case: empty password
{ "user4", "wrongPass", false } // edge case: wrong password
});
}
@Test
public void testLogin() {
assertEquals(expectedOutcome, LoginService.authenticate(username, password));
}
}
Using parameterized tests helps ensure that your test suite doesn't miss edge cases.
3. Relying Solely on Coverage Tools
While tools like JaCoCo and Cobertura help you measure code coverage, relying on them alone can lead to complacency. Code can be covered but not meaningfully tested.
Solution:
Use coverage reports to pinpoint areas lacking tests but validate the results with peer code reviews. Collaborate with your team to write meaningful tests informed by real-world use cases.
4. Not Testing Exception Handling
Exception handling can often be ignored in tests, yet failures in this area can lead to application crashes.
Solution:
Write unit tests that deliberately trigger exceptions to ensure your application behaves as expected during failures.
@Test(expected = IllegalArgumentException.class)
public void testInvalidEmail() {
new User("invalid-email");
}
This test ensures that when an invalid email is used, the application throws an exception, thus ensuring robustness.
5. Overlooking Integration Tests
Unit tests are vital, but they don't simulate how components interact. Omitting integration tests can prevent you from uncovering issues that only manifest during interoperability.
Solution:
Implement integration tests that assess the application as a whole, ensuring various parts function together seamlessly.
@SpringBootTest
public class UserIntegrationTest {
@Autowired
UserService userService;
@Test
public void testUserRegistration() {
User user = new User("jane.doe@example.com");
User savedUser = userService.registerUser(user);
assertNotNull(savedUser.getId());
assertEquals("jane.doe@example.com", savedUser.getEmail());
}
}
This integration test checks that when a user is registered, they are correctly saved in the database, highlighting interactions between components.
6. Writing Tests for Test Coverage Only
Crafting tests simply to inflate coverage metrics can backfire. These tests often lack meaningful assertions and provide no value.
Solution:
Instead, write tests with clear intentions, focusing on the critical functionalities first. Prioritize quality over quantity.
7. Neglecting Refactoring of Tests
Code evolves, and so should your tests. Old tests can become brittle or irrelevant as the codebase changes.
Solution:
Regularly review and refactor tests to align them with the latest code modifications. Treat tests like production code; they should be maintained and improved.
8. Failing to Review Test Cases
Test cases should undergo the same level of scrutiny as production code. Failing to peer-review them can lead to subpar testing coverage.
Solution:
Implement code review processes specifically for tests, ensuring they meet quality standards and effectively cover all intended scenarios.
A Final Look
Maximizing Java code coverage is an essential goal for any software development team aiming for high-quality applications. By avoiding these common mistakes—misunderstanding coverage metrics, neglecting edge cases, blindly relying on tools, and failing to test exception handling—you can develop a robust test suite that genuinely protects your code.
Ultimately, remember that high code coverage is a means to an end, not the end itself. Prioritize comprehensive, high-quality tests that not only cover code but also ensure the application behaves correctly in all scenarios.
For a deeper dive into testing strategies and methodologies, consider reading The Art of Unit Testing by Roy Osherove, which provides extensive insights into writing effective tests.
Start implementing these practices today, and watch your Java applications become more robust and reliable over time!
Checkout our other articles