Common Quarkus Pitfalls: Avoid These Beginner Mistakes!
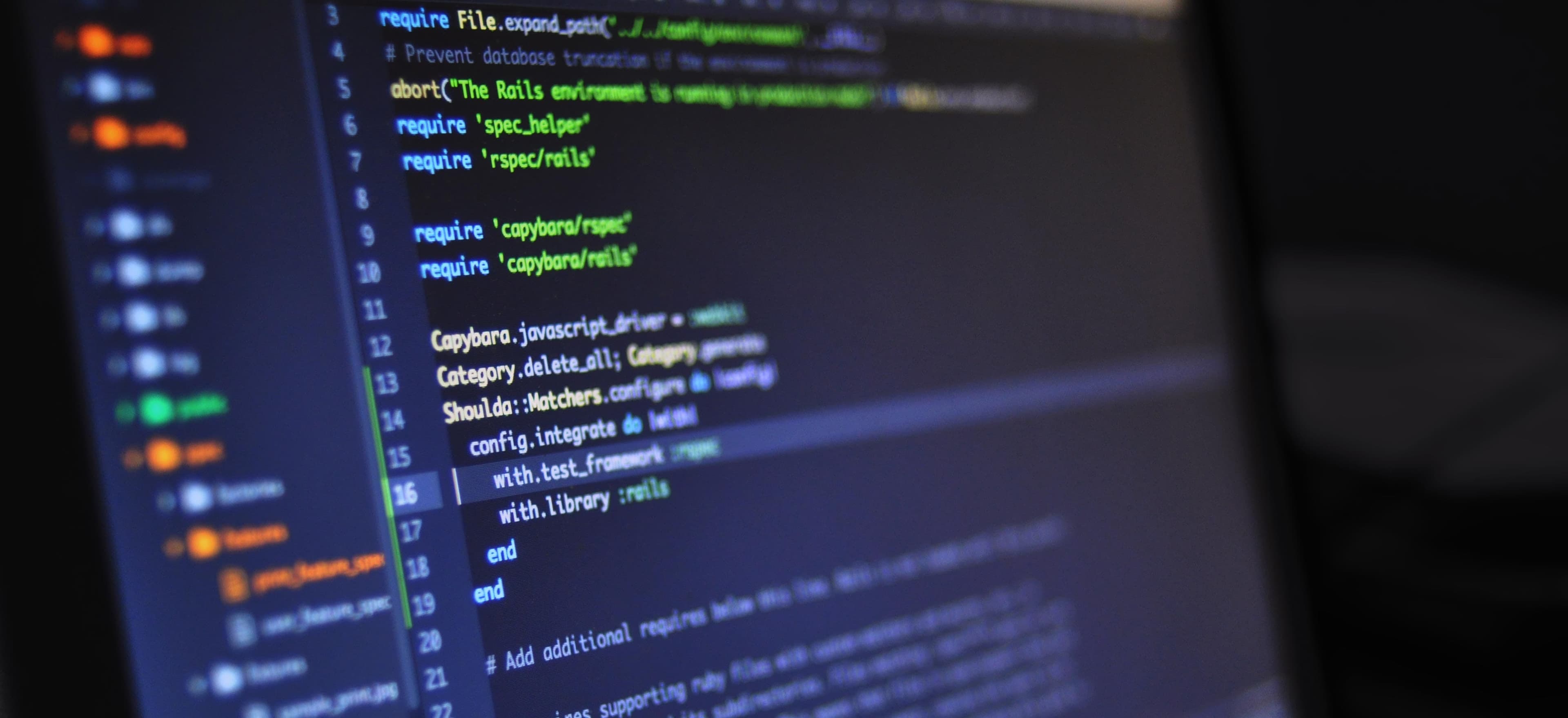
- Published on
Common Quarkus Pitfalls: Avoid These Beginner Mistakes!
Quarkus is rapidly gaining traction as a popular Java framework designed for cloud-native applications. While its capabilities are impressive, beginners often stumble upon common pitfalls that can lead to frustration. In this post, we'll delve into these missteps and provide solutions to help you leverage Quarkus more effectively. By the end, you’ll be well-equipped to build robust applications and avoid the traps that many newcomers fall into.
What is Quarkus?
Quarkus is a Kubernetes-native Java framework tailored for GraalVM and OpenJDK HotSpot. Its design philosophy is centered around making Java a leading platform for cloud environments. It optimizes Java specifically for Kubernetes with a focus on low memory consumption and fast startup times.
For more information about Quarkus, check the official Quarkus website.
1. Insufficient Understanding of Dependency Injection
The Pitfall: Many beginners approach Quarkus with a Spring mindset, which can lead to misunderstandings regarding dependency injection.
The Solution
Quarkus uses CDI (Contexts and Dependency Injection) for managing dependencies. Understanding the lifecycle of objects and the various scopes available in Quarkus (like @ApplicationScoped
, @RequestScoped
, etc.) is crucial.
import javax.enterprise.context.ApplicationScoped;
@ApplicationScoped
public class GreetingService {
public String greet(String name) {
return "Hello, " + name + "!";
}
}
Why?: By using @ApplicationScoped
, you ensure that there is only one instance of GreetingService
throughout your application, optimizing memory usage and performance.
Additional Resources
- CDI in Java EE
2. Ignoring the Quarkus Extension Ecosystem
The Pitfall: A common mistake is not taking full advantage of Quarkus's extensive ecosystem of extensions.
The Solution
Quarkus provides various extensions for integrating with databases, messaging systems, and APIs. Use the command-line interface (CLI) or Maven to add extensions as needed.
./mvnw quarkus:add-extension -Dextensions="jdbc-postgresql"
Why?: This command adds PostgreSQL support seamlessly, allowing you to focus on business logic rather than configuration overhead.
Additional Resources
3. Misunderstanding Configuration
The Pitfall: Beginners often mishandle the configuration files, leading to runtime issues.
The Solution
Use the application.properties
or application.yml
files to manage configurations. Quarkus allows for easy overrides for different environments, such as dev, test, and prod.
quarkus.datasource.db-kind=postgresql
quarkus.datasource.username=myuser
quarkus.datasource.password=mypassword
Why?: Properly managing your configurations enhances the maintainability of your application across different environments.
Additional Resources
4. Overlooking Testing Strategies
The Pitfall: Many newcomers skip writing tests or use the wrong frameworks, resulting in less confidence about their code.
The Solution
Quarkus supports JUnit 5 by default and provides several testing utilities tailored specifically for Quarkus applications.
import io.quarkus.test.junit.QuarkusTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.is;
@QuarkusTest
public class GreetingServiceTest {
@Inject
GreetingService greetingService;
@Test
public void testGreeting() {
String greeting = greetingService.greet("World");
assertThat(greeting, is("Hello, World!"));
}
}
Why?: Writing tests ensures that your application works as expected and that future changes do not break existing functionality.
Additional Resources
- Testing in Quarkus
5. Failing to Utilize Live Reload
The Pitfall: Not taking full advantage of Quarkus's live-reload feature results in slower development cycles.
The Solution
When running your application in development mode using:
./mvnw quarkus:dev
Quarkus automatically detects file changes and reloads the application.
Why?: This feature streamlines the development process, allowing for immediate feedback and reducing the need for constant restarts.
6. Misconfigured Resource Handling
The Pitfall: Beginners may underestimate how Quarkus handles resources such as assets, static files, and templates.
The Solution
Carefully manage static resources in the src/main/resources/META-INF/resources
folder for web applications.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloWorldResource {
@GET
@Produces(MediaType.TEXT_HTML)
public String hello() {
return "<h1>Hello World from Quarkus!</h1>";
}
}
Why?: Proper management of your resources ensures that they are served correctly and enhances user experience.
Additional Resources
7. Overlooking Performance Optimizations
The Pitfall: Developers often neglect the various performance tweaks Quarkus offers.
The Solution
Use the Quarkus build options to customize the build process, target native images for faster performance, and reduce memory footprint.
./mvnw package -Pnative
Why?: Creating a native image ensures faster startup times and lower runtime memory consumption—a core feature of Quarkus.
In Conclusion, Here is What Matters
By avoiding these common pitfalls, you can effectively harness the full potential of Quarkus for your Java applications. Don't let these beginner mistakes impede your growth as a developer. Instead, leverage the resources available, engage with the community, and continually test your understanding of the framework.
As you sharpen your skills, you may find that Quarkus not only enhances your productivity but also evolves your approach to building applications.
For more information and community support, check out the Quarkus Community Forum.
Happy coding!