Mastering SAX vs. DOM: Choosing the Right XML Parser
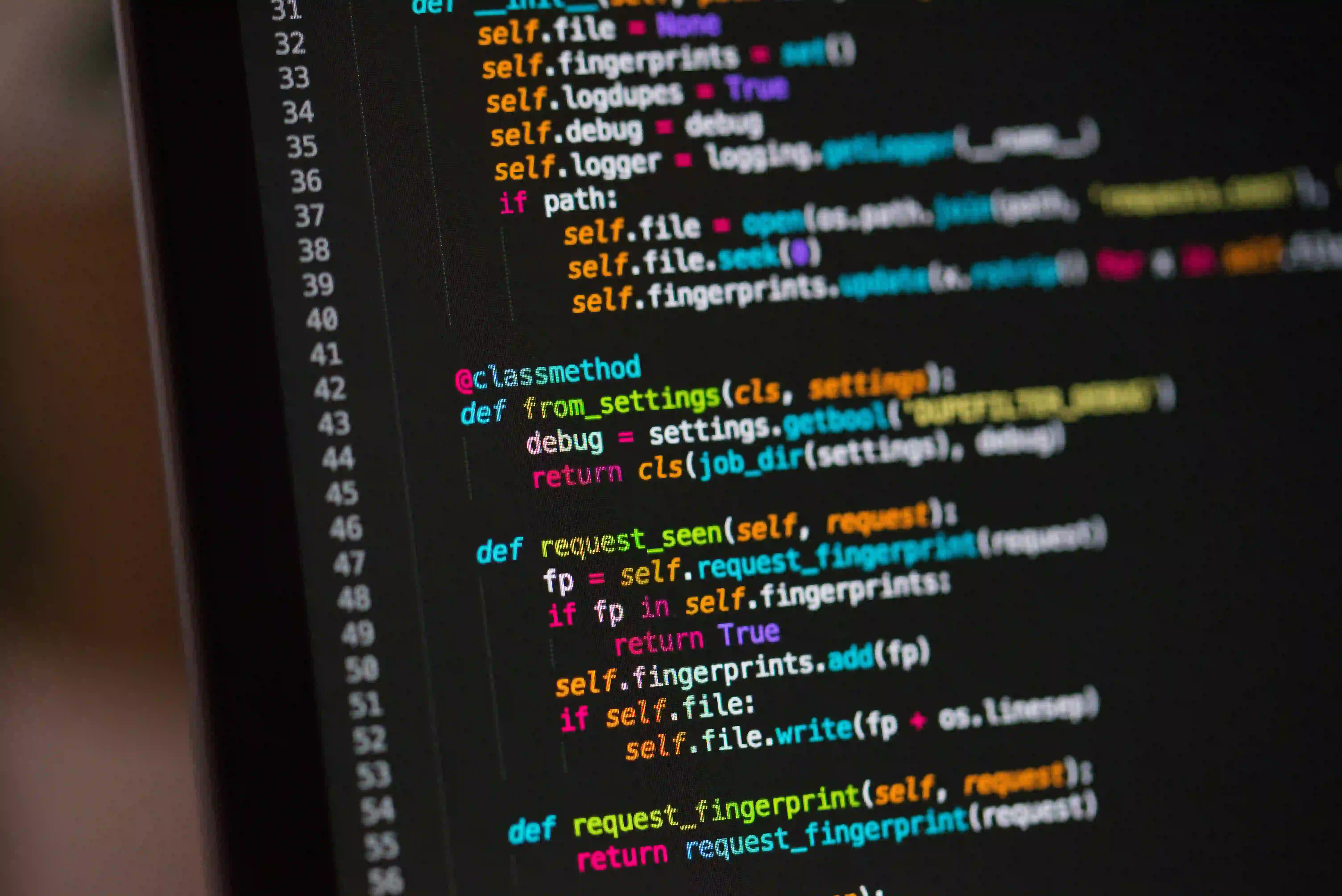
Mastering SAX vs. DOM: Choosing the Right XML Parser
XML (eXtensible Markup Language) is an integral part of modern web development and data interchange. It allows for the structured representation of information in a text-based format, making it easy for both humans and machines to read and understand. When it comes to parsing XML in Java, two of the most widely used options are SAX (Simple API for XML) and DOM (Document Object Model). Each parser has its strengths and weaknesses, making the choice between them a critical decision for developers.
In this post, we will delve into the characteristics of SAX and DOM, explore when to use each parser, and provide practical examples with code snippets.
Understanding SAX and DOM
Before we dive into the comparisons, let's define each parsing method:
SAX (Simple API for XML)
SAX is an event-driven, serial-access mechanism for parsing XML documents. As the parser reads the XML file, it triggers events as it encounters elements and attributes. This "push" model allows for linear reading, which is memory efficient as it does not load the entire document into memory.
Key Characteristics of SAX:
- Memory Efficiency: SAX parsers do not store the entire XML document in memory. They process the document node by node.
- Event-driven: You write handler methods that respond to specific events, such as the start or end of an element.
- Single Pass: SAX reads the document from start to finish in a single pass.
Use Case for SAX: SAX is ideal for parsing large XML files or streams where low memory usage is essential, such as when processing logs or real-time data feeds.
DOM (Document Object Model)
DOM, on the other hand, reads the entire XML document into memory and represents it as a tree structure. This allows for more intuitive data manipulation since you can navigate through and access different parts of the XML tree.
Key Characteristics of DOM:
- Tree Structure: DOM loads the complete XML document into memory, allowing you to traverse and manipulate the data more easily.
- Random Access: Once the document is loaded, you can access any element or attribute at any time.
- Modification Capabilities: You can easily add, update, or delete nodes in the XML structure.
Use Case for DOM: DOM is suitable for smaller XML documents where random access and manipulation are required, such as configuration files or documents where the structure is frequently changed.
Comparing SAX vs. DOM
To make an informed choice between SAX and DOM, let’s highlight their differences across various dimensions.
1. Memory Consumption
SAX is designed to handle large files while consuming minimal memory because it doesn’t keep the entirety of the XML document in memory. Conversely, DOM requires significant memory as it loads the complete structure into memory.
2. Parsing Speed
SAX is generally faster when parsing large documents since it does not involve building a complex data structure. However, for smaller documents where you might need to navigate back and forth, DOM can be more convenient despite the extra overhead.
3. Ease of Use
For developers, DOM offers an easier interface, allowing straightforward navigation through elements and attributes. SAX, while efficient, requires more boilerplate code, making it harder to implement for complex documents.
4. Situational Best Fit
- Use SAX for large XML files, where performance and memory usage are critical.
- Use DOM for smaller XML documents where you need to manipulate the data.
Practical Examples
SAX Parsing Example
Here is how to implement a simple SAX parser in Java:
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
public class SAXParserExample {
public static void main(String[] args) {
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
DefaultHandler handler = new DefaultHandler() {
boolean isTitle = false;
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (qName.equalsIgnoreCase("title")) {
isTitle = true;
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
if (isTitle) {
System.out.println("Title: " + new String(ch, start, length));
isTitle = false;
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
// Optional: Handle end of element if needed
}
};
saxParser.parse("example.xml", handler);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Code Explanation
- SAXParserFactory: Creates a SAXParser instance.
- DefaultHandler: Implemented to handle SAX events.
- startElement: Identifies the start of an XML element.
- characters: Processes the character data when the title element is encountered.
In this example, we extract the title from an XML file. SAX is memory efficient, making it suitable for large XML files.
DOM Parsing Example
Now, let's look at a DOM parser implementation:
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
public class DOMParserExample {
public static void main(String[] args) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("example.xml");
doc.getDocumentElement().normalize();
NodeList titleList = doc.getElementsByTagName("title");
for (int i = 0; i < titleList.getLength(); i++) {
Element titleElement = (Element) titleList.item(i);
System.out.println("Title: " + titleElement.getTextContent());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Code Explanation
- DocumentBuilderFactory: Creates a factory for document builders.
- DocumentBuilder: Parses XML and creates a Document object.
- normalize(): Normalizes the XML structure, merging adjacent text nodes.
- getElementsByTagName(): Retrieve node elements by name.
This parser reads the entire XML file and can easily traverse back and forth, making it better for smaller XML documents or where modifications are needed.
The Bottom Line
Choosing between SAX and DOM in Java hinges on your specific needs. If you're dealing primarily with large XML files where performance and memory consumption are pivotal, SAX is the right choice. On the other hand, if you require functionality that allows for easy navigation and manipulation of a smaller XML document, DOM provides a more straightforward solution.
Regardless of your choice, both parsing methods are powerful tools in the Java ecosystem. For more information on XML parsing and best practices, consider checking out the Java XML Tutorial.
Now that you have an understanding of SAX and DOM, you can make more informed decisions when handling XML in your Java applications. Happy coding!