Common Pitfalls When Building Java WebSocket Chat Apps
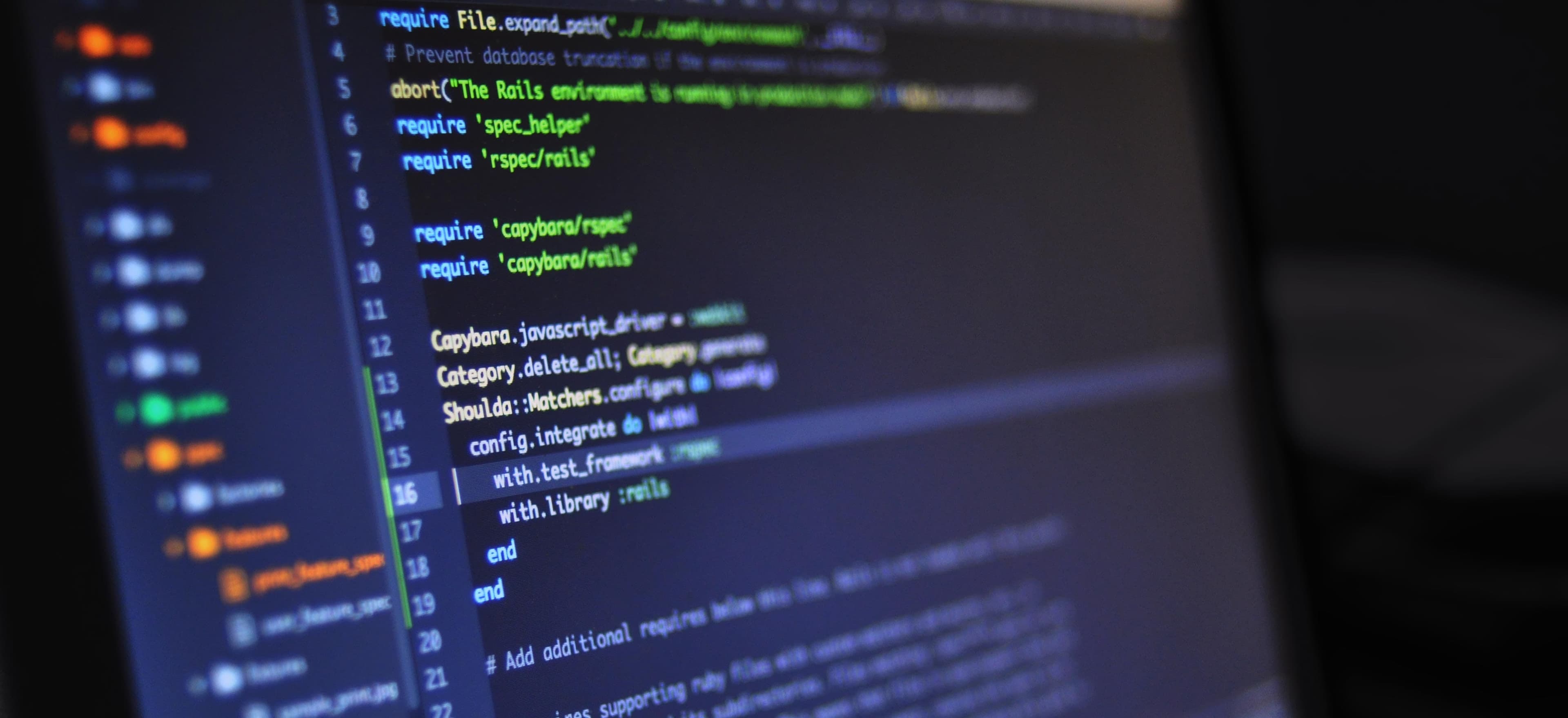
- Published on
Common Pitfalls When Building Java WebSocket Chat Apps
WebSocket technology is revolutionizing real-time web applications, allowing for two-way communication between the client and the server. In Java, building applications with WebSocket can be straightforward, but pitfalls abound for the unprepared. Let's discuss some common mistakes you can encounter when building Java WebSocket chat applications and how to avoid them.
Understanding WebSocket Basics
Before diving into the pitfalls, it's crucial to understand what WebSocket is. WebSocket is a protocol that enables persistent connections with low latency. Unlike traditional HTTP, where a request-response cycle can delay information transfer, WebSocket keeps the connection open, allowing both the server and client to send messages at any time.
Setting Up a Basic WebSocket Server
Let's start with a simple WebSocket server. We will use the Java EE API to create our WebSocket application.
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/chat")
public class ChatServer {
@OnOpen
public void onOpen(Session session) {
System.out.println("New connection: " + session.getId());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Message from client: " + message);
// Here you would typically broadcast the message to other sessions.
}
}
Why This Code Is Important
- @ServerEndpoint: This annotation defines the WebSocket endpoint.
- onOpen: This method is triggered when a new connection is established.
- onMessage: This handles incoming messages from the client. You will want to use this to manage broadcasts to other clients.
Now that we've established a basic structure, let's explore common pitfalls.
Common Pitfalls
1. Ignoring Thread Safety
One of the most common mistakes is overlooking thread safety in the WebSocket server. Sessions in WebSocket are not inherently thread-safe. You must ensure that shared resources are handled properly.
Solution
Use synchronization techniques or rely on concurrent collections like ConcurrentHashMap
.
import java.util.concurrent.ConcurrentHashMap;
public class ChatServer {
private static final ConcurrentHashMap<String, Session> activeSessions = new ConcurrentHashMap<>();
@OnOpen
public void onOpen(Session session) {
activeSessions.put(session.getId(), session);
System.out.println("New connection: " + session.getId());
}
}
2. Poor Message Handling
Another frequent issue is inadequate message handling. Many developers fail to sanitize or validate messages before broadcasting them.
Solution
Ensure you validate incoming messages:
@OnMessage
public void onMessage(String message, Session session) {
if (message == null || message.trim().isEmpty()) {
System.err.println("Invalid message");
return; // Ignore empty messages
}
// Broadcast the message to other sessions
}
3. Not Implementing Error Handling
WebSocket applications can experience unexpected disconnects or unhandled exceptions. Not implementing proper error handling can lead to a poor user experience.
Solution
Add error handling to manage exceptions and disconnections gracefully:
@OnError
public void onError(Session session, Throwable throwable) {
System.err.println("Error in session " + session.getId() + ": " + throwable.getMessage());
// Cleanup or notify other clients as necessary
}
4. Failure to Scale
As your application grows, the initial design may not accommodate scaling. A common pitfall is relying solely on a single server instance for handling connections.
Solution
Use a scalable architecture such as:
- Load Balancers: Distribute incoming connections across multiple instances.
- Message Brokers: Use something like RabbitMQ or Kafka to handle message distribution efficiently.
5. Not Handling Disconnects Properly
Ignoring user disconnects can lead to stale connections and memory leaks. Expired sessions should be cleaned up efficiently.
Solution
Implement @OnClose
to remove sessions accordingly:
@OnClose
public void onClose(Session session) {
activeSessions.remove(session.getId());
System.out.println("Connection closed: " + session.getId());
}
6. Lack of User Authentication
Failing to implement user authentication can expose your application to unauthorized access. Incorporate authentication mechanisms to ensure only legitimate users can join chat rooms.
Solution
Consider using JWT (JSON Web Tokens) for a stateless authentication mechanism. This can validate users upon their initial connection and maintain session integrity without storing session state on the server.
7. Not Testing with Real-World Scenarios
Testing WebSocket applications under real-world conditions is crucial. Some developers only test their applications in ideal conditions, neglecting various users joining and leaving simultaneously.
Solution
Use tools like Apache JMeter or Locust to simulate multiple users interacting with the WebSocket server to ensure it can handle various conditions.
Key Takeaways
Building a Java WebSocket chat application is rewarding, but avoiding common pitfalls is essential for creating a robust, scalable solution. Prioritize thread safety, implement proper error handling, scale effectively, and ensure security through user authentication. Regular testing under realistic conditions will prepare your application for the demands of real-world usage.
For further reading, consider reviewing the official Java WebSocket API documentation, which provides a more in-depth understanding of additional features and best practices.
Happy Coding! You are now equipped with the knowledge to avoid the common pitfalls when building Java WebSocket chat applications.