Mastering Java Bytecode Manipulation: Common Pitfalls and Fixes
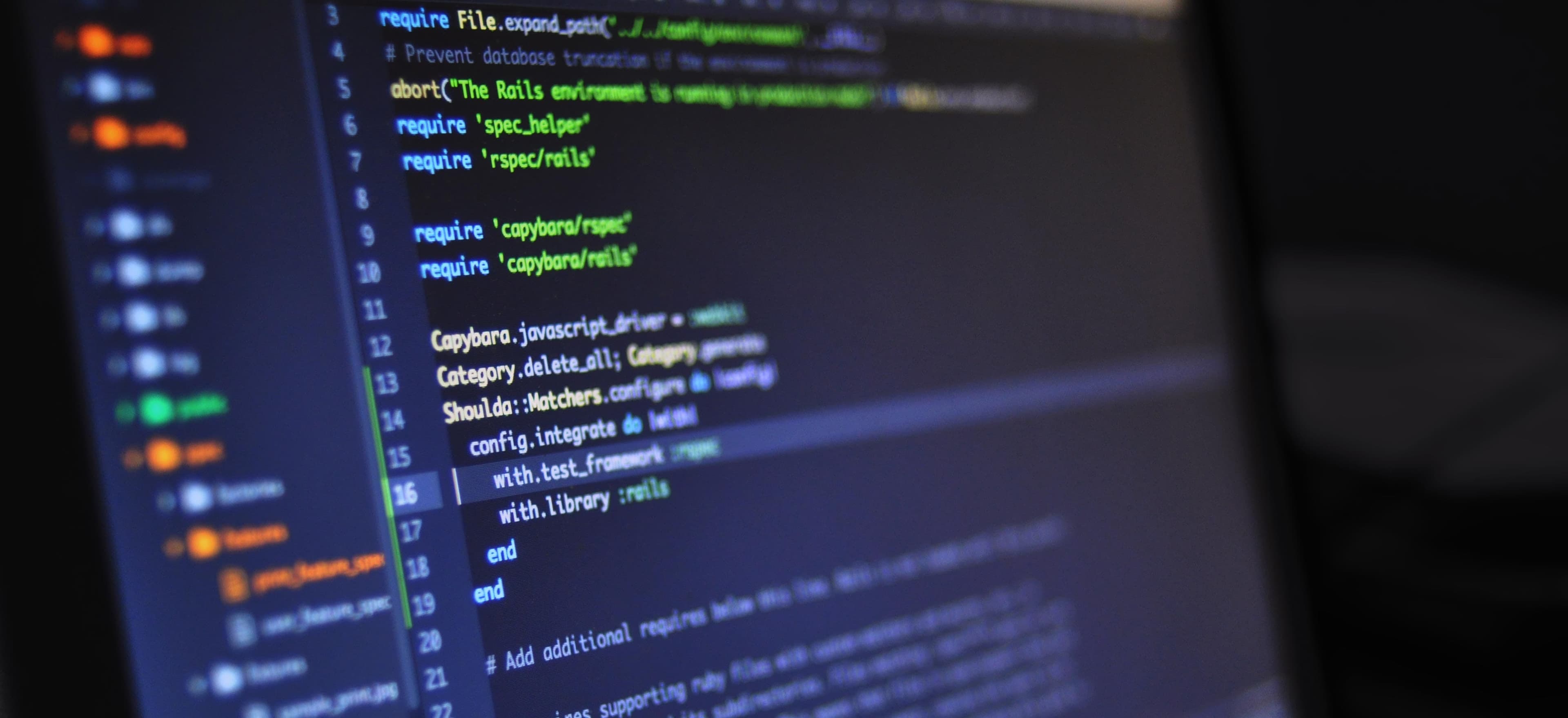
- Published on
Mastering Java Bytecode Manipulation: Common Pitfalls and Fixes
Java, a highly versatile programming language, compiles source code into bytecode, which the Java Virtual Machine (JVM) interprets. While this abstraction adds portability and security benefits, it also offers a playground for developers looking to manipulate the behavior of Java applications at runtime. Bytecode manipulation can unlock powerful features such as dynamic class generation, behavior modification, and improved performance. However, it is easy to encounter pitfalls on this journey. In this post, we'll explore common mistakes and their solutions when working with Java bytecode manipulation.
Understanding Java Bytecode
Before diving into the pitfalls, let’s clarify what Java bytecode is. When you compile a Java class, the Java compiler generates bytecode in the form of a .class
file. This bytecode is a set of instructions that the JVM executes. Understanding this layer of abstraction allows developers to modify and enhance existing behaviors, improving versatility.
Why Manipulate Bytecode?
Bytecode manipulation can be beneficial for several reasons:
- Dynamic Proxy: Creating proxies at runtime for interfaces.
- Performance Optimization: Adjusting methods for better performance without changing the source.
- Aspect-Oriented Programming (AOP): Injecting common behavior (like logging) across various parts of an application.
To manipulate Java bytecode effectively, libraries like ASM, Javassist, and ByteBuddy are commonly used. Let's look at how we often misstep when using these tools.
Common Pitfalls in Bytecode Manipulation
1. Not Understanding the Class Loader
The class loader is a critical piece of the JVM ecosystem. If you manipulate bytecode without clear knowledge of class loading, important classes may not be found at runtime.
Fix: Understand the hierarchy of class loaders - Bootstrap, Extension, and Application class loaders. Use the appropriate loader when loading your modified classes.
ClassLoader classLoader = MyClass.class.getClassLoader();
Class<?> modifiedClass = classLoader.loadClass("com.example.ModifiedClass");
2. Altering the Structure of Classes Unintentionally
While bytecode libraries allow you to modify fields and methods, failing to maintain the integrity of the class can lead to NoSuchMethodError
or NoSuchFieldError
.
Fix: Always review the structure of the existing class before attempting modifications. Utilize the ClassVisitor
in ASM, for example, to examine the structure.
public class MyClassVisitor extends ClassVisitor {
public MyClassVisitor() {
super(Opcodes.ASM9);
}
@Override
public void visit(int version, int access, String name, String signature, String superName, String[] interfaces) {
// Analyze class structure
}
}
3. Failing to Handle Method Overriding Properly
When overriding methods, it is crucial to maintain the correct method signature (name, return type, parameters). A mismatch can lead to runtime errors.
Fix: Ensure the new method matches the original closely. Use MethodVisitor
methods for accurate override:
@Override
public void visitMethod(int access, String name, String descriptor, String signature, String[] exceptions) {
if ("myMethod".equals(name)) {
// Ensure the descriptor matches the original
}
}
4. Ignoring Performance Implications
While modifying bytecode can enhance performance, improper changes can lead to inefficiencies or higher memory consumption.
Fix: Profile your application pre- and post-manipulation to identify performance impacts. Use tools like VisualVM or Java Flight Recorder to monitor performance.
5. Lack of Testing and Version Control
Manipulating bytecode dynamically can introduce subtle regressions. Skipping tests might lead to fragile code.
Fix: Implement a solid testing strategy. Ensure unit tests cover the modified methods comprehensively. Use a version control system to track changes.
@Test
public void testModifiedMethod() {
// Assume we've modified MyClass
MyClass instance = new MyClass();
assertEquals(expected, instance.modifiedMethod());
}
Best Practices to Avoid Pitfalls
1. Use High-Level Libraries
Consider using libraries like ByteBuddy that abstract the complexities of bytecode manipulation. Higher-level constructs often prevent the low-level pitfalls associated with direct ASM usage.
2. Follow the SOLID Principles
When modifying classes, try to adhere to SOLID principles. This will help maintain code readability and prevent breakages.
3. Document Your Changes
Documentation serves as your guide when revisiting your modifications. Keep the reasons for changes and expected implications well-documented.
4. Practice Incremental Changes
Instead of making multiple large changes in one go, make small, incremental adjustments. This minimizes the chances of introducing complex bugs and eases troubleshooting.
The Bottom Line
Bytecode manipulation provides an opportunity to take Java applications to new heights; however, one must tread carefully to avoid common pitfalls. By understanding the class loading mechanisms, maintaining the integrity of class structure, and prioritizing testing and version control, you can successfully leverage bytecode manipulation.
For further reading, consider looking at:
By mastering these techniques, you can craft fine-tuned Java applications tailored to your specific needs while maintaining stability and performance. Happy coding!