Common SSL/TLS Pitfalls in Java Applications and How to Avoid Them
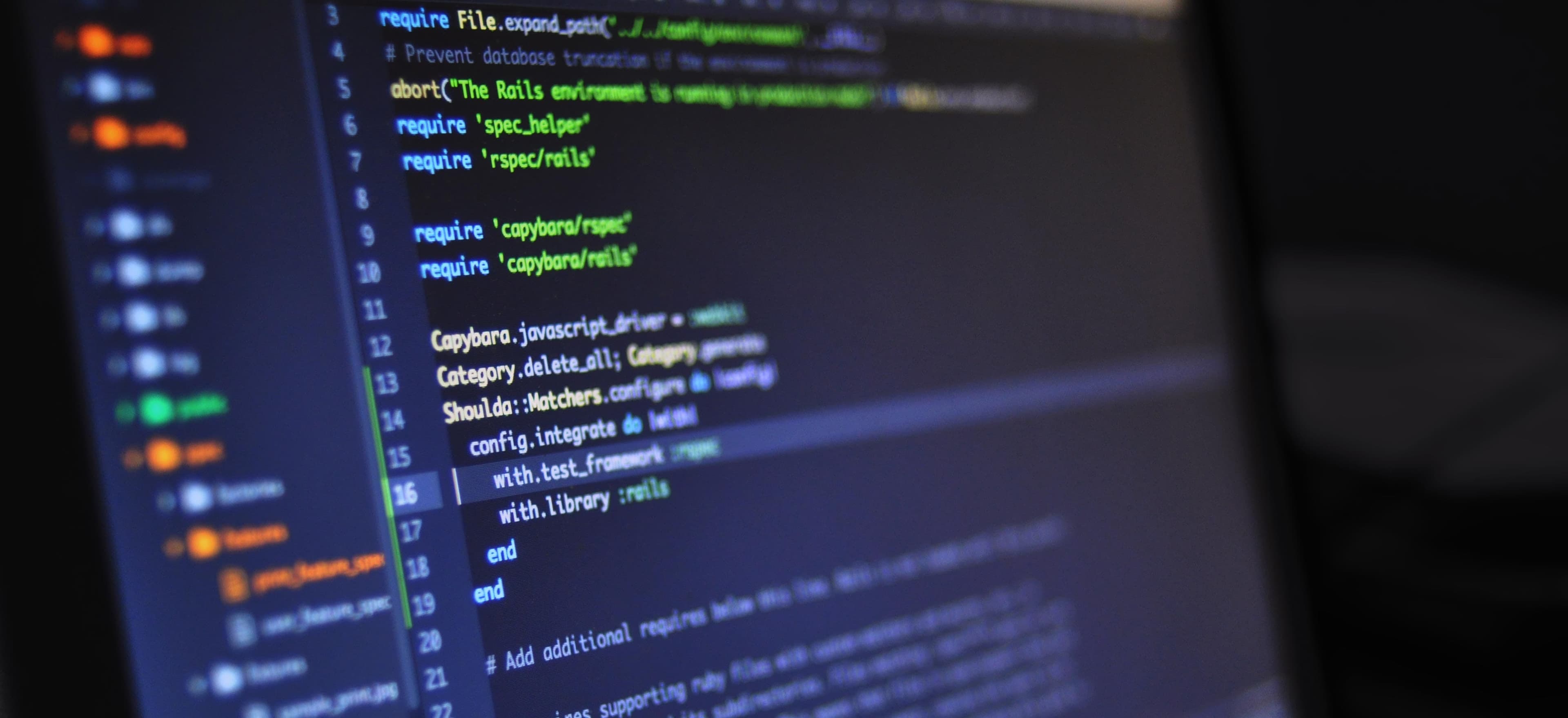
- Published on
Common SSL/TLS Pitfalls in Java Applications and How to Avoid Them
In today's digital world, securing data transactions is imperative. Secure Sockets Layer (SSL) and its successor, Transport Layer Security (TLS), are cryptographic protocols designed to provide communication security over a computer network. In Java applications, improper implementation of SSL/TLS can lead to vulnerabilities, potentially compromising sensitive data. This post explores common pitfalls developers encounter while implementing SSL/TLS in Java applications and provides strategic measures to avoid them.
1. Not Validating SSL Certificates
One of the most egregious mistakes is failing to validate SSL certificates. The validation process assures that the server the application is communicating with is indeed genuine and not a malicious imitation.
Why This Matters
Not validating SSL certificates exposes your application to Man-in-the-Middle (MitM) attacks. This means that an attacker could intercept and alter communications, leading to data leaks or corruption.
Example Code Snippet
import javax.net.ssl.*;
import java.security.cert.X509Certificate;
public class CustomTrustManager {
public static void main(String[] args) {
try {
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, new TrustManager[]{new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] certs, String authType) {}
public void checkServerTrusted(X509Certificate[] certs, String authType) {}
public X509Certificate[] getAcceptedIssuers() { return new X509Certificate[0]; }
}}, new java.security.SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(sslContext.getSocketFactory());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on the Code
The above code demonstrates how to establish an SSLContext
with a custom TrustManager
. While this example disables validation, it’s crucial to implement proper validation here. Use checkServerTrusted
for the server SSL certificate validation effectively. This code highlights what not to do.
How to Avoid This Pitfall
- Always validate certificates.
- Use libraries like Bouncy Castle to enhance security features for validating certificates.
- Implement hostname verification to ensure that the hostname matches the certificate.
2. Using Weak Cipher Suites
Many developers inadvertently use outdated or weak cipher suites for SSL/TLS connections. This oversight can make your application susceptible to eavesdropping and snooping.
Why This Matters
Weak cipher suites (like DES or RC4) have known vulnerabilities and can be easily exploited by attackers. It’s essential to use robust and up-to-date cipher suites to secure data effectively.
Example Code Snippet
import javax.net.ssl.*;
public class SSLConfiguration {
public static void main(String[] args) {
try {
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, null, null);
SSLServerSocketFactory factory = sslContext.getServerSocketFactory();
SSLServerSocket serverSocket = (SSLServerSocket) factory.createServerSocket(8443);
// Specify strong cipher suites
serverSocket.setEnabledCipherSuites(new String[]{
"TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384",
"TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256"
});
// Continue with server socket operations
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on the Code
This code demonstrates how to create an SSLServerSocket
and specify strong cipher suites. It not only ensures a more secure connection but also facilitates communication with modern clients.
How to Avoid This Pitfall
- Regularly review your cipher suite configurations. (Use resources like Mozilla's SSL Configuration Generator).
- Ensure you are disabling deprecated versions like SSLv2, SSLv3, and even TLSv1.0.
- Apply strict server configurations that enforce secure communication protocols.
3. Ignoring Security Updates
Java and the Java Dev Kit (JDK) are updated consistently, which often involves security patches related to SSL/TLS protocols. Ignoring these updates can introduce vulnerabilities over time.
Why This Matters
The longer you wait to update, the greater your exposure becomes. Cyber threats are evolving, and consistent updates are critical to maintaining robust security.
How to Avoid This Pitfall
- Set up regular checks for updates in your development and deployment environments.
- Use tools like Jenkins for continuous integration that can automatically alert developers to newer versions of Java and third-party libraries.
4. Improper Handling of SSL Exceptions
Carelessly managing SSL-related exceptions could leak sensitive information or lead to application logic flaws.
Example Code Snippet
import javax.net.ssl.*;
import java.io.IOException;
import java.net.URL;
public class SSLConnection {
public static void main(String[] args) {
try {
URL url = new URL("https://example.com");
HttpsURLConnection conn = (HttpsURLConnection) url.openConnection();
conn.connect();
} catch (SSLHandshakeException e) {
System.err.println("SSL handshake failed: " + e.getMessage());
// Implement proper error handling
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary on the Code
In this snippet, an SSLHandshakeException
is caught, but it only outputs the message without properly managing the error. This could leak sensitive information about the server that could be exploited.
How to Avoid This Pitfall
- Implement comprehensive logging that does not expose sensitive stack traces.
- Instead of printing the entire stack trace, log structured error messages that aid in debugging without compromising security.
- Consider using a proper logging framework like SLF4J or Log4j for better control over logging.
Lessons Learned
Implementing SSL/TLS in Java applications is essential for safeguarding sensitive data. By understanding common pitfalls—such as failing to validate SSL certificates, using weak cipher suites, ignoring updates, and improperly handling exceptions—developers can avoid serious security flaws.
For further reading on SSL/TLS and secure application development practices in Java, consider visiting OWASP's SSL/TLS Cheat Sheet or the Java Secure Socket Extension (JSSE) Reference Guide.
By continuously educating ourselves on security practices and remaining vigilant with updates and configurations, we can fortify our Java applications against potential threats and enhance the trust of our users.
Checkout our other articles